How Can I Format Dates in SQL to dd/mm/yyyy?
In the world of databases, managing and manipulating dates is a fundamental skill that can significantly impact the integrity and usability of your data. Whether you’re developing a complex application or simply querying a database, understanding how to format dates correctly is crucial. One of the most widely used formats is the `dd/mm/yyyy` format, which is favored in many regions around the globe. This article delves into the nuances of working with date formats in SQL, specifically focusing on how to effectively utilize the `dd/mm/yyyy` format in your queries and data management practices.
When dealing with dates in SQL, it’s essential to recognize that different database systems may have varying default formats and functions. This can lead to confusion and errors if not properly handled. The `dd/mm/yyyy` format, while intuitive for many users, requires specific functions and conversions to ensure that your SQL queries return the correct results. Understanding how to convert and manipulate date formats will not only enhance your ability to retrieve accurate data but also improve the overall efficiency of your database operations.
In this exploration, we will cover the best practices for formatting dates in SQL, including how to store, retrieve, and manipulate dates in the `dd/mm/yyyy` format. By the end of this article, you’ll be equipped with the knowledge to
Date Formatting in SQL
When dealing with date values in SQL, it is crucial to understand how to format dates correctly to ensure accurate data manipulation and retrieval. SQL databases typically use a standard date format, but when you need to display or input dates in the `dd/mm/yyyy` format, specific functions and methods can help achieve this.
Converting Dates to dd/mm/yyyy Format
To convert a date to the `dd/mm/yyyy` format, SQL provides various functions depending on the database management system in use. Here are some common examples:
- SQL Server: You can use the `FORMAT` function or `CONVERT` function.
- MySQL: The `DATE_FORMAT` function is used.
- Oracle: The `TO_CHAR` function can be applied.
Examples by Database
Below are examples of how to format a date in `dd/mm/yyyy` for different SQL databases.
Database | SQL Command | Output Example |
---|---|---|
SQL Server | SELECT FORMAT(GETDATE(), ‘dd/MM/yyyy’) AS FormattedDate; | 25/10/2023 |
MySQL | SELECT DATE_FORMAT(NOW(), ‘%d/%m/%Y’) AS FormattedDate; | 25/10/2023 |
Oracle | SELECT TO_CHAR(SYSDATE, ‘DD/MM/YYYY’) AS FormattedDate FROM dual; | 25/10/2023 |
Inserting Dates in dd/mm/yyyy Format
When inserting dates in the `dd/mm/yyyy` format, it is essential to ensure that the database recognizes this format correctly. For most databases, the standard format is `yyyy-mm-dd`, so you may need to convert the input date before insertion.
- For SQL Server:
“`sql
INSERT INTO YourTable (DateColumn)
VALUES (CONVERT(DATETIME, ’25/10/2023′, 103));
“`
- For MySQL:
“`sql
INSERT INTO YourTable (DateColumn)
VALUES (STR_TO_DATE(’25/10/2023′, ‘%d/%m/%Y’));
“`
- For Oracle:
“`sql
INSERT INTO YourTable (DateColumn)
VALUES (TO_DATE(’25/10/2023′, ‘DD/MM/YYYY’));
“`
Retrieving Dates in dd/mm/yyyy Format
Retrieving dates in the `dd/mm/yyyy` format can be done using similar functions as mentioned earlier. This ensures that users or applications interacting with the database can easily read the date.
- Example for SQL Server:
“`sql
SELECT FORMAT(DateColumn, ‘dd/MM/yyyy’) AS FormattedDate FROM YourTable;
“`
- Example for MySQL:
“`sql
SELECT DATE_FORMAT(DateColumn, ‘%d/%m/%Y’) AS FormattedDate FROM YourTable;
“`
- Example for Oracle:
“`sql
SELECT TO_CHAR(DateColumn, ‘DD/MM/YYYY’) AS FormattedDate FROM YourTable;
“`
By following these examples and guidelines, you can effectively manage date formats in your SQL databases, ensuring the correct presentation and manipulation of date values according to the `dd/mm/yyyy` standard.
Date Formatting in SQL
In SQL, formatting dates to a specific string representation, such as the `dd/mm/yyyy` format, can be essential for both data retrieval and display. Various SQL database systems provide functions that allow you to format dates as needed. Below are examples for some of the most common SQL databases.
MySQL
In MySQL, you can use the `DATE_FORMAT()` function to format a date. Here’s how to format a date to `dd/mm/yyyy`:
“`sql
SELECT DATE_FORMAT(NOW(), ‘%d/%m/%Y’) AS formatted_date;
“`
- Function: `DATE_FORMAT(date, format)`
- Format Specifiers:
- `%d` – Day of the month, numeric (01 to 31)
- `%m` – Month, numeric (01 to 12)
- `%Y` – Year, numeric, four digits
SQL Server
For SQL Server, you can utilize the `FORMAT()` function or `CONVERT()` function to achieve the desired date format:
Using `FORMAT()`:
“`sql
SELECT FORMAT(GETDATE(), ‘dd/MM/yyyy’) AS formatted_date;
“`
Using `CONVERT()`:
“`sql
SELECT CONVERT(VARCHAR, GETDATE(), 103) AS formatted_date;
“`
- Function: `FORMAT(value, format_string)`
- Function: `CONVERT(data_type, expression, style)`
- Style: `103` is the style code for `dd/mm/yyyy`.
PostgreSQL
In PostgreSQL, the `TO_CHAR()` function is used for formatting dates. You can format a date as follows:
“`sql
SELECT TO_CHAR(NOW(), ‘DD/MM/YYYY’) AS formatted_date;
“`
- Function: `TO_CHAR(date, format)`
- Format Specifiers:
- `DD` – Day of the month (01 to 31)
- `MM` – Month (01 to 12)
- `YYYY` – Year (four digits)
Oracle
Oracle SQL also provides the `TO_CHAR()` function for date formatting:
“`sql
SELECT TO_CHAR(SYSDATE, ‘DD/MM/YYYY’) AS formatted_date FROM dual;
“`
- Function: `TO_CHAR(date, format)`
- Format Specifiers:
- `DD` – Day of the month
- `MM` – Month
- `YYYY` – Year
SQLite
In SQLite, you can format dates using the `strftime()` function:
“`sql
SELECT strftime(‘%d/%m/%Y’, ‘now’) AS formatted_date;
“`
- Function: `strftime(format_string, time)`
- Format Specifiers:
- `%d` – Day of the month
- `%m` – Month
- `%Y` – Year
Understanding how to format dates in SQL is crucial for effective database management and reporting. The above examples illustrate how to convert dates to the `dd/mm/yyyy` format across various SQL databases, ensuring consistency and clarity in your data presentation.
Understanding Date Formats in SQL: Expert Insights
Dr. Emily Carter (Database Architect, Tech Solutions Inc.). “When working with SQL, it’s crucial to understand how date formats can affect data retrieval and manipulation. The dd/mm/yyyy format is not universally supported across all SQL databases, which can lead to inconsistencies. Always check the documentation specific to your SQL dialect.”
Michael Chen (Senior SQL Developer, DataWise Analytics). “To effectively use the dd/mm/yyyy format in SQL, one must utilize the appropriate conversion functions. For instance, in SQL Server, using CONVERT with style 103 can help achieve this format, ensuring that date comparisons are accurate and reliable.”
Sarah Johnson (Data Management Consultant, Insight Partners). “Adopting a consistent date format across your database is essential for data integrity. While dd/mm/yyyy is common in many regions, consider the implications of using different formats in multi-regional applications. Standardizing on ISO 8601 (yyyy-mm-dd) may prevent potential issues.”
Frequently Asked Questions (FAQs)
How can I format a date in dd/mm/yyyy in SQL?
You can format a date in SQL using the `FORMAT()` function in SQL Server or `TO_CHAR()` in Oracle. For example, in SQL Server, you can use `FORMAT(your_date_column, ‘dd/MM/yyyy’)`.
Is it possible to store dates in dd/mm/yyyy format in SQL databases?
SQL databases typically store dates in a standard format (e.g., YYYY-MM-DD). However, you can format the output for display purposes without changing the underlying storage format.
What SQL function can I use to convert a string to a date in dd/mm/yyyy format?
You can use `STR_TO_DATE()` in MySQL to convert a string to a date. For example, `STR_TO_DATE(’31/12/2023′, ‘%d/%m/%Y’)` will convert the string to a date type.
How do I retrieve dates in dd/mm/yyyy format from a SQL query?
To retrieve dates in dd/mm/yyyy format, you can use the `CONVERT()` function in SQL Server or `TO_CHAR()` in Oracle. For example, `SELECT CONVERT(varchar, your_date_column, 103)` for SQL Server.
Can I set the default date format for my SQL session?
Yes, you can set the default date format for your SQL session using the `SET DATEFORMAT` command in SQL Server or by configuring the NLS_DATE_FORMAT parameter in Oracle.
What should I consider when using dd/mm/yyyy format in SQL?
When using dd/mm/yyyy format, be cautious about regional settings and ensure consistency across your application to avoid misinterpretation of date values.
In SQL, handling dates in the dd/mm/yyyy format requires an understanding of how different database systems interpret date formats. The standard SQL date format is typically yyyy-mm-dd, which can lead to challenges when working with data that is formatted differently. To effectively manage dates in the dd/mm/yyyy format, it is crucial to utilize the appropriate functions and methods specific to the SQL dialect being used, such as MySQL, SQL Server, or PostgreSQL.
For instance, in MySQL, the STR_TO_DATE function can be employed to convert a string in dd/mm/yyyy format into a date type. In SQL Server, the CONVERT function allows for similar conversions, enabling users to specify the format they are working with. PostgreSQL offers the TO_DATE function for this purpose. Understanding these functions not only aids in data manipulation but also ensures data integrity and accuracy when performing date-related queries.
Moreover, it is essential to consider regional settings and the implications of date formats in applications. Misinterpretation of date formats can lead to errors in data processing and reporting. Therefore, developers and database administrators should standardize date formats across their systems and ensure that all users are aware of the expected format to prevent confusion and maintain consistency.
Author Profile
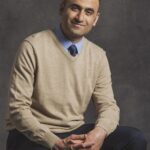
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?