How Can You Effectively Implement A/B Testing in Python?
In the fast-paced world of digital marketing and product development, making data-driven decisions is crucial for success. One of the most effective methods to optimize user experience and enhance engagement is through A/B testing. This powerful technique allows businesses to compare two versions of a webpage, app feature, or any other user interaction to determine which one performs better. But how can you harness the power of A/B testing using Python, a language renowned for its simplicity and versatility? In this article, we will explore the fundamentals of A/B testing in Python, equipping you with the knowledge to implement this strategy effectively and drive meaningful results.
A/B testing is not just about running experiments; it’s about understanding user behavior and making informed choices based on statistical evidence. By leveraging Python’s robust libraries and frameworks, you can streamline the process of designing, executing, and analyzing A/B tests. From setting up your test environment to interpreting the results, Python provides a suite of tools that can simplify complex tasks and enhance your analytical capabilities. Whether you’re a seasoned data scientist or a marketer looking to dive into the world of experimentation, mastering A/B testing in Python can unlock new opportunities for growth and innovation.
As we delve deeper into this topic, we will cover essential concepts, best practices, and
A/B Testing Frameworks in Python
A/B testing in Python can be efficiently conducted using several frameworks that simplify the implementation of experiments. Popular libraries include:
- SciPy: Offers statistical functions to analyze A/B test results.
- Statsmodels: Provides tools for statistical modeling and hypothesis testing.
- PyAB: A lightweight library tailored specifically for A/B testing.
- ABBA: A simple A/B testing framework that allows for easy integration and analysis.
These libraries allow users to set up experiments, collect data, and perform statistical analysis to determine the effectiveness of variations.
Implementing A/B Testing with SciPy
Using SciPy for A/B testing involves several steps: designing the experiment, collecting data, and analyzing results. Here’s a basic example of how to perform a t-test to compare two groups:
“`python
import numpy as np
from scipy import stats
Sample data for two groups
control_group = np.random.normal(100, 10, 1000)
experiment_group = np.random.normal(110, 10, 1000)
Perform t-test
t_statistic, p_value = stats.ttest_ind(control_group, experiment_group)
print(f’T-statistic: {t_statistic}, P-value: {p_value}’)
“`
In this code snippet, we generate normally distributed data for two groups and conduct a t-test to compare their means.
Data Collection and Analysis
To conduct a successful A/B test, it is crucial to collect data accurately. This includes defining key metrics, determining sample sizes, and setting up data collection methods. Once the data is collected, it can be analyzed using statistical tests to ascertain the significance of the results.
Key metrics might include:
- Conversion rate
- Average order value
- Bounce rate
- Engagement level
The following table summarizes common metrics used in A/B testing:
Metric | Description | Importance |
---|---|---|
Conversion Rate | Percentage of users completing a desired action | Directly measures the effectiveness of the variant |
Average Order Value | Average revenue generated per user | Indicates profitability changes |
Bounce Rate | Percentage of visitors leaving without interacting | Helps assess user engagement |
Engagement Level | Metrics like time spent on site or pages viewed | Shows how well users are interacting with content |
Interpreting Results
Interpreting the results of an A/B test involves understanding the significance of the p-value obtained from statistical tests. A common threshold for significance is a p-value of 0.05. If the p-value is less than this threshold, the null hypothesis (that there is no difference between the groups) can be rejected.
Additionally, confidence intervals should be calculated to understand the range within which the true effect lies. This provides insight into the reliability of the results obtained.
In practice, results may also be visualized using libraries like Matplotlib or Seaborn to create compelling graphs that illustrate the differences between variants.
By following these steps and utilizing the appropriate tools, practitioners can effectively conduct A/B testing in Python to optimize their products and marketing strategies.
A/B Testing in Python
A/B testing, also known as split testing, is a method used to compare two versions of a webpage or app against each other to determine which one performs better. Implementing A/B testing in Python involves several steps, including setting up the environment, designing the test, analyzing results, and drawing conclusions.
Setting Up the Environment
To begin A/B testing in Python, you need to install the necessary libraries. The following libraries are commonly used:
- NumPy: For numerical operations.
- Pandas: For data manipulation and analysis.
- SciPy: For statistical tests.
- Matplotlib/Seaborn: For data visualization.
You can install these libraries using pip:
“`bash
pip install numpy pandas scipy matplotlib seaborn
“`
Designing the Test
When designing an A/B test, consider the following components:
- Objective: Clearly define what you want to test (e.g., conversion rates, click-through rates).
- Target Audience: Identify who will be part of the test.
- Sample Size: Calculate the required sample size to achieve statistically significant results.
- Variations: Develop the two versions (A and B) of the webpage or app feature.
Example of sample size calculation:
“`python
import statsmodels.api as sm
Define parameters
alpha = 0.05 significance level
power = 0.8 desired power
p1 = 0.1 conversion rate for group A
p2 = 0.15 conversion rate for group B
Calculate sample size
effect_size = sm.stats.proportion_effectsize(p1, p2)
sample_size = sm.stats.NormalIndPower().solve_power(effect_size, power=power, alpha=alpha)
print(“Sample size per group:”, sample_size)
“`
Running the A/B Test
Once you have your test designed, you can run the test and collect data. This can be done through web analytics tools or custom tracking.
Here’s a basic structure for data collection:
“`python
import pandas as pd
Simulate data collection
data = {
‘group’: [‘A’] * 1000 + [‘B’] * 1000,
‘conversion’: [1 if i < 100 else 0 for i in range(1000)] + [1 if i < 150 else 0 for i in range(1000)]
}
df = pd.DataFrame(data)
```
Analyzing Results
To analyze the results, use statistical tests to determine if the difference in performance is significant.
- Chi-Squared Test: For categorical data.
- t-Test: For comparing means between two groups.
Example of a Chi-Squared test:
“`python
from scipy.stats import chi2_contingency
Create a contingency table
contingency_table = pd.crosstab(df[‘group’], df[‘conversion’])
Perform Chi-Squared test
chi2, p, dof, expected = chi2_contingency(contingency_table)
print(“p-value:”, p)
“`
Visualizing the Results
Visualization aids in comprehending the results of your A/B test. Use Matplotlib or Seaborn for this purpose.
Example of a bar plot:
“`python
import matplotlib.pyplot as plt
import seaborn as sns
Calculate conversion rates
conversion_rates = df.groupby(‘group’)[‘conversion’].mean().reset_index()
Create bar plot
sns.barplot(x=’group’, y=’conversion’, data=conversion_rates)
plt.title(‘Conversion Rates for A/B Test’)
plt.xlabel(‘Group’)
plt.ylabel(‘Conversion Rate’)
plt.show()
“`
Interpreting the Results
Interpreting the results requires a clear understanding of statistical significance:
- If the p-value is less than the significance level (typically 0.05), you reject the null hypothesis, indicating a significant difference between groups.
- Consider the practical significance in addition to statistical significance to ensure the findings are actionable.
By following these guidelines, you can effectively implement A/B testing in Python, enabling data-driven decision-making to enhance user experience and optimize performance.
Expert Insights on A/B Testing in Python
Dr. Emily Chen (Data Scientist, Tech Innovations Inc.). “A/B testing in Python is a powerful approach for data-driven decision-making. Utilizing libraries such as SciPy and Statsmodels allows practitioners to conduct rigorous statistical analyses and ensure the validity of their results. The flexibility of Python enables seamless integration with data pipelines, making it an ideal choice for modern experimentation.”
Michael Thompson (Lead Software Engineer, Analytics Solutions Group). “Implementing A/B testing in Python requires a solid understanding of both the technical and statistical aspects. Tools like Pytest for testing and Matplotlib for visualization can significantly enhance the process. It is crucial to define clear hypotheses and metrics to measure success accurately.”
Sarah Patel (Marketing Analyst, Digital Strategies LLC). “Python’s extensive ecosystem provides marketers with the resources to conduct effective A/B tests. Libraries such as Pandas for data manipulation and Seaborn for visual representation can simplify the analysis process. Understanding user behavior through A/B testing can lead to substantial improvements in conversion rates.”
Frequently Asked Questions (FAQs)
What is A/B testing in Python?
A/B testing in Python refers to a statistical method used to compare two versions of a variable to determine which one performs better. It involves running experiments where users are randomly assigned to either group A or group B, and their responses are measured to assess performance.
How can I implement A/B testing in Python?
You can implement A/B testing in Python using libraries such as SciPy for statistical analysis, Pandas for data manipulation, and Matplotlib or Seaborn for visualization. You would typically collect data, perform statistical tests, and analyze the results to draw conclusions.
What libraries are commonly used for A/B testing in Python?
Common libraries for A/B testing in Python include SciPy for statistical functions, Statsmodels for more advanced statistical modeling, and PyAB for a dedicated A/B testing framework. Additionally, Pandas and NumPy are often used for data handling and manipulation.
How do I analyze the results of an A/B test in Python?
To analyze A/B test results, calculate metrics such as conversion rates for both groups, perform statistical tests (e.g., t-tests or chi-squared tests) to determine significance, and visualize the results using plots. This helps in understanding the impact of changes made.
What statistical tests are suitable for A/B testing in Python?
Suitable statistical tests for A/B testing include the t-test for comparing means, chi-squared test for categorical data, and z-test for proportions. The choice of test depends on the data type and distribution.
How can I ensure my A/B test is valid in Python?
To ensure validity, randomize user assignment to groups, maintain a sufficient sample size, control for external variables, and run the test for an adequate duration. Additionally, ensure that the metrics used are relevant to the objectives of the test.
A/B testing in Python is a powerful method for comparing two or more variations of a product, feature, or marketing strategy to determine which one performs better. By utilizing statistical analysis, A/B testing helps businesses make data-driven decisions that enhance user experience and optimize conversion rates. Python, with its rich ecosystem of libraries such as SciPy, Statsmodels, and Pandas, provides robust tools for implementing and analyzing A/B tests effectively. This allows users to conduct experiments, analyze results, and draw meaningful conclusions with relative ease.
Key takeaways from the discussion on A/B testing in Python include the importance of proper experimental design, including sample size determination and randomization, to ensure valid results. Additionally, understanding statistical significance and confidence intervals is crucial for interpreting the outcomes of A/B tests accurately. Python’s capabilities enable practitioners to automate the testing process, visualize data, and conduct post-test analyses, making it an invaluable resource for marketers and data scientists alike.
A/B testing in Python not only facilitates the systematic evaluation of different strategies but also empowers organizations to leverage data for continuous improvement. By embracing this approach, businesses can enhance their decision-making processes, ultimately leading to increased customer satisfaction and higher revenue. As the field of data science continues
Author Profile
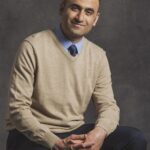
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?