Is ‘A Course in Python: The Core of the Language’ the Key to Mastering Python?
In the ever-evolving landscape of programming languages, Python stands out as a beacon of simplicity and versatility. Whether you are a seasoned developer or a curious beginner, understanding the core of Python is essential for harnessing its full potential. “A Course in Python: The Core of the Language” serves as your gateway to mastering the fundamental concepts that make Python not just a tool, but a powerful ally in your coding journey. This article will guide you through the essential elements that define Python, equipping you with the knowledge to tackle complex problems with elegance and efficiency.
Python’s design philosophy emphasizes readability and simplicity, making it an ideal language for newcomers while still being robust enough for experienced programmers. At the heart of Python lies a set of core principles that govern its syntax, data structures, and execution model. By delving into these foundational aspects, learners will discover how Python’s unique features facilitate a smooth learning curve and foster creativity in problem-solving.
As we explore the core components of Python, we will uncover the language’s dynamic typing, object-oriented nature, and extensive standard library. These elements not only enhance the programming experience but also empower developers to write clean, maintainable code. Join us as we embark on this enlightening journey through the core of Python, where each
Understanding Python’s Data Types
Python’s strength lies in its rich set of built-in data types, which allows developers to handle data effectively and efficiently. The primary data types in Python can be categorized as follows:
- Numeric Types: Includes integers (`int`), floating-point numbers (`float`), and complex numbers (`complex`).
- Sequence Types: Comprises strings (`str`), lists (`list`), and tuples (`tuple`).
- Mapping Type: The dictionary (`dict`), which is a collection of key-value pairs.
- Set Types: The `set` and `frozenset`, allowing for collection of unique elements.
- Boolean Type: Represents truth values using `True` and “.
Each of these types comes with specific methods and operations that can be performed on them, making Python a versatile language for data manipulation.
Data Type | Description | Example |
---|---|---|
int | Whole numbers, both positive and negative | 42 |
float | Numbers with a decimal point | 3.14 |
str | Textual data enclosed in quotes | “Hello, World!” |
list | Ordered collection of items | [1, 2, 3] |
dict | Collection of key-value pairs | {“name”: “Alice”, “age”: 30} |
Control Structures in Python
Control structures are essential for managing the flow of a Python program. They include conditional statements and loops, which allow for decision-making and repeated execution of code blocks.
- Conditional Statements: The `if`, `elif`, and `else` statements enable branching logic in code. For example:
“`python
if condition:
execute this block
elif another_condition:
execute this block
else:
execute this block
“`
- Loops: Python supports both `for` and `while` loops.
- For Loop: Iterates over a sequence (like a list or string).
“`python
for item in sequence:
process item
“`
- While Loop: Continues execution as long as a specified condition is true.
“`python
while condition:
execute block
“`
These control structures allow developers to write dynamic and responsive code tailored to various scenarios.
Functions and Scope
Functions in Python are defined using the `def` keyword and are essential for organizing code into reusable blocks. A function can take parameters and return values, enhancing modularity and readability.
- Defining a Function:
“`python
def function_name(parameters):
function body
return value
“`
- Scope: In Python, the scope determines the visibility of variables. There are four types of scopes:
- Local Scope: Variables defined within a function.
- Enclosing Scope: Variables in the local scope of enclosing functions.
- Global Scope: Variables defined at the top level of a script or module.
- Built-in Scope: Names pre-defined in Python’s built-in namespace.
Understanding these scopes is crucial for preventing variable name conflicts and managing state effectively in larger applications.
Key Concepts in Python
Python is a versatile programming language that emphasizes readability and simplicity. Understanding its core concepts is crucial for effective coding. Here are some foundational elements:
- Variables and Data Types: Variables are used to store data, and Python supports multiple data types:
- Integers (`int`)
- Floating-point numbers (`float`)
- Strings (`str`)
- Lists (`list`)
- Tuples (`tuple`)
- Dictionaries (`dict`)
- Control Structures: Python utilizes control structures to manage the flow of execution:
- Conditional Statements:
- `if`, `elif`, `else`
- Loops:
- `for` loops
- `while` loops
Functions and Modules
Functions encapsulate code for reusability and modularity. They can take parameters and return values. Key aspects include:
- Defining Functions:
“`python
def function_name(parameters):
function body
return value
“`
- Modules: Modules allow for code organization and reuse. You can create a module by saving a Python file with a `.py` extension. Importing a module can be done using:
“`python
import module_name
“`
Object-Oriented Programming (OOP)
Python supports OOP, enabling developers to create classes and objects. Essential concepts include:
- Classes and Objects:
- A class is a blueprint for creating objects.
- An object is an instance of a class.
- Key Features:
- Encapsulation: Bundling data and methods that operate on the data.
- Inheritance: Creating new classes from existing ones to promote code reuse.
- Polymorphism: Allowing methods to do different things based on the object it is acting upon.
Error Handling and Exceptions
Effective error handling is essential for robust applications. Python provides a mechanism to catch and handle exceptions:
- Try and Except Blocks:
“`python
try:
code that may cause an exception
except ExceptionType:
code to handle the exception
“`
- Finally Clause: This block executes regardless of whether an exception occurred:
“`python
finally:
code that runs after try/except blocks
“`
Data Structures in Python
Python includes built-in data structures that facilitate efficient data management:
Data Structure | Description | Use Cases |
---|---|---|
List | Ordered, mutable collection | Storing sequences of items |
Tuple | Ordered, immutable collection | Storing fixed collections |
Set | Unordered collection of unique items | Removing duplicates |
Dictionary | Key-value pairs | Storing related data |
File Handling
Reading from and writing to files is straightforward in Python:
- Opening a File:
“`python
with open(‘filename.txt’, ‘r’) as file:
content = file.read()
“`
- Writing to a File:
“`python
with open(‘filename.txt’, ‘w’) as file:
file.write(‘Hello, World!’)
“`
This ensures proper resource management by automatically closing the file after operations.
Expert Insights on “A Course in Python: The Core of the Language”
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “Understanding the core of Python is essential for any programmer. A course that focuses on these fundamentals not only enhances coding skills but also fosters a deeper appreciation for the language’s design philosophy, making it easier to adapt to advanced concepts.”
Michael Chen (Lead Software Engineer, CodeCraft Academy). “A comprehensive course on Python should emphasize its core features, such as data types, control structures, and functions. Mastering these elements is crucial for building robust applications and for transitioning to more complex frameworks and libraries.”
Sarah Lopez (Education Director, Python for Everyone Initiative). “Courses that delve into the core of Python empower learners to think critically about problem-solving. By focusing on the foundational aspects of the language, students can develop a strong base that supports lifelong learning and adaptability in the tech field.”
Frequently Asked Questions (FAQs)
What is “A Course in Python: The Core of the Language”?
This course focuses on the fundamental aspects of the Python programming language, covering essential concepts, syntax, and best practices for effective coding.
Who is the target audience for this course?
The course is designed for beginners with no prior programming experience, as well as intermediate programmers looking to strengthen their understanding of Python’s core features.
What topics are typically covered in this course?
Key topics include data types, control structures, functions, modules, error handling, and object-oriented programming, providing a comprehensive foundation in Python.
What are the prerequisites for enrolling in this course?
There are no formal prerequisites; however, familiarity with basic programming concepts may enhance the learning experience.
How is the course delivered?
The course is typically delivered through a combination of video lectures, interactive coding exercises, and quizzes to reinforce learning and assess understanding.
Will I receive a certification upon completion of the course?
Most courses offer a certificate of completion, which can be a valuable addition to your resume or LinkedIn profile, demonstrating your proficiency in Python.
“A Course in Python: The Core of the Language” serves as an essential resource for both beginners and experienced programmers seeking to deepen their understanding of Python. The course meticulously covers the fundamental concepts, syntax, and structures that form the backbone of the language. By emphasizing practical applications and real-world examples, it equips learners with the necessary skills to effectively utilize Python in various programming scenarios.
Moreover, the course highlights the importance of mastering core principles such as data types, control flow, functions, and object-oriented programming. These foundational elements are crucial for developing robust and efficient Python applications. The structured approach of the course allows learners to progressively build their knowledge and confidence, ensuring a comprehensive grasp of the language.
Key takeaways from the course include the significance of writing clean and maintainable code, the value of leveraging Python’s extensive libraries, and the advantages of understanding Python’s unique features. These insights not only enhance a programmer’s technical capabilities but also foster a mindset geared towards continuous learning and improvement in the ever-evolving field of software development.
Author Profile
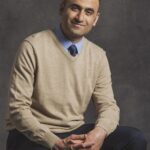
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?