What Should You Do When a Fatal JavaScript Error Occurs?
### Introduction
In the world of web development, JavaScript reigns supreme as one of the most widely used programming languages. However, as with any technology, it is not without its pitfalls. One of the most alarming issues a developer can encounter is a fatal JavaScript error. This seemingly innocuous phrase can send shivers down the spine of even the most seasoned coder, as it often signals a critical failure that can disrupt functionality and compromise user experience. Understanding the nature of these errors, their causes, and how to effectively troubleshoot them is essential for anyone looking to maintain robust and reliable web applications.
A fatal JavaScript error occurs when the interpreter encounters a problem so severe that it cannot continue executing the script. This can stem from a variety of sources, including syntax issues, type mismatches, or even external factors like network failures. When such an error arises, it not only halts the execution of the current script but can also lead to a cascade of failures across the application, affecting everything from user interactions to data processing. As web applications become increasingly complex, the potential for these errors to occur grows, making it crucial for developers to be equipped with the right knowledge and tools to tackle them head-on.
In the following sections, we will delve deeper into the common causes of
Understanding the Fatal JavaScript Error
A fatal JavaScript error indicates a critical issue that disrupts the execution of a script. These errors can arise from several factors, such as syntax mistakes, reference errors, or runtime exceptions. When a fatal error occurs, it typically halts the execution of the entire script, preventing any subsequent code from running. This can lead to significant disruptions, especially in web applications where user interaction is critical.
Common causes of fatal JavaScript errors include:
- Syntax Errors: Mistakes in the code structure, such as missing brackets or incorrect variable declarations.
- Type Errors: Occur when a value is not of the expected type, such as attempting to call a method on an undefined variable.
- Reference Errors: Happen when the code tries to access a variable that has not been declared.
- Out of Memory Errors: Occur when the script consumes too much memory, often due to infinite loops or excessive data processing.
Identifying Fatal JavaScript Errors
Detecting fatal JavaScript errors can be accomplished through various methods. Developers should make use of browser developer tools, which provide a console for viewing errors and debugging information. The following steps are essential for identifying issues:
- Open Developer Tools: Typically accessible via F12 or right-clicking on the page and selecting “Inspect.”
- View the Console: This tab displays error messages and warnings that occur during script execution.
- Check Network Requests: Ensure that all necessary resources, such as scripts and stylesheets, are properly loaded.
- Use Debugging Tools: Set breakpoints in the code to step through execution and observe variable states.
Common Debugging Techniques
When faced with a fatal JavaScript error, employing effective debugging strategies is crucial. Here are some widely used techniques:
- Console Logging: Utilize `console.log()` to track the values of variables at different points in the code.
- Try-Catch Blocks: Wrap potentially problematic code in try-catch statements to gracefully handle errors.
- Linting Tools: Employ tools like ESLint to catch syntax and potential runtime errors before running the code.
Error Handling Strategies
Implementing robust error handling can mitigate the impact of fatal JavaScript errors. Here are strategies to consider:
- Graceful Degradation: Ensure that applications can still function, albeit with reduced functionality, when errors occur.
- User Notifications: Inform users when an error occurs, providing options to retry or report the issue.
- Logging Mechanisms: Record errors in a log for later analysis, helping to identify patterns or recurring issues.
Error Type | Common Causes | Debugging Techniques |
---|---|---|
Syntax Error | Missing brackets, incorrect keywords | Linting, code review |
Type Error | Invalid operations on types | Console logging, type checking |
Reference Error | Undefined variables | Debugging tools, variable tracking |
Out of Memory | Excessive data processing | Performance profiling, optimization |
By employing these methods and strategies, developers can effectively navigate the challenges posed by fatal JavaScript errors, ensuring a smoother experience for users and maintaining application integrity.
Understanding the Causes of Fatal JavaScript Errors
Fatal JavaScript errors can arise from various sources, often resulting in a disruption of script execution. Key causes include:
- Syntax Errors: Mistakes in code structure, such as missing brackets or semicolons.
- Type Errors: Attempting to operate on an incompatible data type, such as treating a string as a number.
- Reference Errors: Accessing variables or functions that are not defined in the current scope.
- Network Issues: Failure to load external scripts due to connectivity problems or incorrect URLs.
Common Symptoms of Fatal JavaScript Errors
Identifying the presence of a fatal JavaScript error can be facilitated by recognizing specific symptoms:
- Browser Crashes: The web page becomes unresponsive.
- Error Messages: Messages appearing in the console, such as “Uncaught SyntaxError” or “Uncaught TypeError.”
- Incomplete Functionality: Features relying on JavaScript fail to execute as intended.
- Visual Artifacts: User interface elements may not render correctly.
Troubleshooting Steps for Fatal JavaScript Errors
When dealing with a fatal JavaScript error, follow these troubleshooting steps:
- Check the Console: Open the browser’s developer tools and inspect the console for error messages.
- Review the Code: Look for syntax errors or typos in your JavaScript code.
- Isolate the Error: Comment out sections of code to identify the specific line causing the issue.
- Debugging Tools: Utilize debugging tools, such as breakpoints, to step through your code.
- Check Dependencies: Ensure all external libraries and resources are correctly linked and loaded.
Best Practices to Prevent Fatal JavaScript Errors
Implementing best practices can significantly reduce the likelihood of encountering fatal JavaScript errors:
- Code Linting: Use tools like ESLint to automatically identify potential errors in your code.
- Modular Code: Organize code into modules to improve readability and maintainability.
- Error Handling: Employ try-catch blocks to manage exceptions gracefully.
- Regular Testing: Conduct thorough testing using various browsers and devices to catch errors early.
Resources for Further Learning
For those seeking to deepen their understanding of JavaScript error handling and debugging, the following resources may be beneficial:
Resource Type | Name/Link | Description |
---|---|---|
Documentation | [MDN Web Docs](https://developer.mozilla.org/) | Comprehensive JavaScript reference. |
Online Courses | [Codecademy](https://www.codecademy.com/) | Interactive JavaScript tutorials. |
Books | “JavaScript: The Good Parts” by Douglas Crockford | In-depth exploration of JavaScript. |
Community Forums | [Stack Overflow](https://stackoverflow.com/) | Q&A platform for troubleshooting help. |
Handling Fatal JavaScript Errors in Production
In a production environment, addressing fatal JavaScript errors requires a structured approach:
- Error Logging: Implement logging mechanisms to capture and analyze errors in real time.
- User Notifications: Design user-friendly error messages that inform users without overwhelming them.
- Fallback Strategies: Consider providing alternative content or functionality when JavaScript fails to execute.
- Monitoring Tools: Use tools like Sentry or LogRocket to monitor application performance and capture errors proactively.
Understanding the Causes of Fatal JavaScript Errors
Dr. Emily Carter (Lead Software Engineer, Tech Innovations Inc.). “A fatal JavaScript error typically occurs due to issues such as syntax errors, reference errors, or exceeding memory limits. These errors can halt script execution, leading to significant disruptions in web applications. Developers must implement robust error handling and debugging techniques to mitigate such risks.”
James Patel (Senior Web Developer, CodeCraft Solutions). “When encountering a fatal JavaScript error, it is crucial to analyze the stack trace provided by the browser’s developer tools. This can pinpoint the exact line of code causing the issue, enabling developers to address the root cause effectively. Regular code reviews and adherence to best practices can also prevent these errors from occurring in the first place.”
Linda Chen (JavaScript Consultant, WebDev Experts). “Fatal JavaScript errors can significantly impact user experience and application performance. It is essential for developers to utilize modern frameworks that offer built-in error handling and logging capabilities. Additionally, thorough testing across different environments can help identify potential issues before they reach production.”
Frequently Asked Questions (FAQs)
What does “a fatal JavaScript error occurred” mean?
A fatal JavaScript error indicates a critical issue in the execution of JavaScript code, causing the script to terminate unexpectedly. This often results from syntax errors, reference errors, or issues with external libraries.
What are common causes of fatal JavaScript errors?
Common causes include syntax mistakes, calling undefined variables or functions, incorrect API usage, and issues with asynchronous code execution. Additionally, conflicts with browser extensions or outdated libraries can contribute to these errors.
How can I troubleshoot a fatal JavaScript error?
To troubleshoot, use the browser’s developer tools to inspect the console for error messages. Review the code for syntax errors, check variable and function definitions, and ensure that all libraries are correctly loaded and compatible with your code.
Can fatal JavaScript errors be prevented?
Yes, they can be minimized by following best coding practices, such as using strict mode, validating input, employing error handling techniques, and regularly testing code in various environments to catch issues early.
What tools can help identify and fix fatal JavaScript errors?
Tools such as linters (e.g., ESLint), debuggers (e.g., Chrome DevTools), and integrated development environments (IDEs) with built-in error detection can assist in identifying and resolving fatal JavaScript errors effectively.
Is it possible to recover from a fatal JavaScript error?
While a fatal error stops the execution of the script, recovery can be achieved by implementing error handling mechanisms, such as try-catch blocks, which allow the program to continue running or to fail gracefully without crashing the entire application.
In summary, a fatal JavaScript error typically indicates a critical issue within a web application that prevents it from executing properly. Such errors can arise from various sources, including syntax errors, reference errors, or issues with external libraries. Identifying the root cause of these errors is essential for developers, as they can lead to significant disruptions in user experience and application functionality. Proper debugging techniques and tools are vital for diagnosing and resolving these issues effectively.
Moreover, understanding the context in which these errors occur can aid developers in implementing better error handling and prevention strategies. By utilizing modern development practices, such as thorough testing, code reviews, and the adoption of robust frameworks, developers can minimize the occurrence of fatal JavaScript errors. Additionally, leveraging browser developer tools can provide insights into error messages and stack traces, facilitating quicker resolutions.
Ultimately, addressing fatal JavaScript errors not only enhances the reliability of web applications but also improves overall user satisfaction. Developers are encouraged to stay informed about best practices and emerging technologies that can help mitigate these errors. By fostering a proactive approach to coding and debugging, developers can ensure a smoother and more efficient development process.
Author Profile
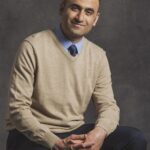
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?