How Can You Resolve ‘A Generic Error Occurred in GDI+’ When Working with Graphics?
In the world of software development and graphic design, encountering errors can be a frustrating yet common experience. One such error that has puzzled many developers and users alike is the cryptic message: “A generic error occurred in GDI+.” This seemingly vague notification often appears when working with image processing or rendering in .NET applications, leaving many to wonder about its origins and potential solutions. Understanding this error is crucial for anyone involved in graphics programming or application development, as it can hinder productivity and lead to unexpected downtime. In this article, we will delve into the intricacies of this error, exploring its causes, implications, and the strategies you can employ to resolve it effectively.
The GDI+ library, a core component of the .NET framework, is responsible for rendering graphics and handling image files. When this error surfaces, it typically indicates an underlying issue that could stem from a variety of sources, such as file permissions, invalid file paths, or resource management problems. Developers may encounter this error during tasks like saving images, manipulating graphics, or even when attempting to display images on a user interface. The ambiguity of the error message can make troubleshooting a daunting task, but understanding its context is the first step toward resolution.
As we navigate through the potential triggers of the ”
Understanding GDI+ Errors
GDI+ is a graphics device interface in Windows that allows for the rendering of graphics and images. When working with GDI+, various errors can occur, one of the most common being the generic error message: “A generic error occurred in GDI+”. This error can arise from multiple sources, often related to file access permissions, incorrect file paths, or issues with the image format.
Common causes of this error include:
- File Permissions: The application does not have permission to write to the specified file location.
- Invalid Paths: The path to the file may not exist, or the filename may be incorrect.
- File Format Issues: The image format may not be supported or corrupted.
- Resource Limitations: The system may be running low on resources, which can hinder GDI+ operations.
Troubleshooting Steps
To resolve the “generic error occurred in GDI+” message, consider the following troubleshooting steps:
- Check File Permissions: Ensure that the application has the necessary permissions to access the file or directory.
- Verify File Path: Double-check the file path for accuracy, ensuring it exists and is correctly formatted.
- Inspect Image Formats: Confirm that the image file is in a supported format and not corrupted.
- Monitor System Resources: Use system monitoring tools to check if there are sufficient resources available for GDI+ operations.
Common Solutions
Here are some effective solutions to address the GDI+ error:
- Run as Administrator: If file permissions are an issue, running the application with administrator privileges may help.
- Change Output Path: Try saving the file to a different directory, preferably one with guaranteed write permissions, such as the user’s Documents folder.
- Use Correct File Extensions: Ensure that the file extension matches the image format being saved (e.g., .png for PNG files).
- Image Disposal: When working with images, make sure to dispose of image objects properly after use to free up resources.
Error Handling Example
Implementing proper error handling can help manage GDI+ errors effectively. Here is a simple Cexample:
“`csharp
try
{
using (Image img = Image.FromFile(“path_to_image”))
{
img.Save(“output_path”, ImageFormat.Png);
}
}
catch (System.Runtime.InteropServices.ExternalException ex)
{
Console.WriteLine(“GDI+ Error: ” + ex.Message);
}
“`
Summary of Error Causes and Solutions
Cause | Solution |
---|---|
File Permissions | Run as administrator or change directory permissions |
Invalid File Path | Verify and correct the file path |
Unsupported Image Format | Use a supported format and check for corruption |
System Resource Limitations | Close other applications or restart the system |
By addressing these common issues, users can effectively troubleshoot and resolve GDI+ errors, ensuring smoother application performance and image handling.
Understanding the GDI+ Error
The “generic error occurred in GDI+” is an exception that arises from the Graphics Device Interface Plus (GDI+) in .NET applications. This error typically indicates issues related to file handling, permissions, or memory management during image processing tasks.
Common scenarios that lead to this error include:
- Attempting to save an image to a file path that does not exist or is invalid.
- Insufficient permissions to write to the specified directory.
- The image being used is already open in another process, preventing modifications.
- Issues with memory allocation or corruption during image manipulation.
Troubleshooting Steps
To resolve the GDI+ error, consider the following troubleshooting steps:
- Check File Path:
Ensure the file path used for saving or loading images is correct. Use a valid, fully qualified path.
- Inspect Permissions:
Verify that the application has the necessary permissions to access the file system where the image is being saved or loaded. This may involve:
- Running the application as an administrator.
- Adjusting folder permissions to allow write access.
- Close Open Streams:
Make sure that any image files or streams are closed before trying to save or modify them. Use the `Dispose()` method on image objects to release resources.
- Validate Image Format:
Confirm that the image format you are trying to save is supported by GDI+. Unsupported formats can trigger this error.
- Check for Memory Issues:
Monitor memory usage and ensure your application is not running out of memory. Optimize image processing to handle large images more efficiently.
Code Examples
When working with GDI+, the following code snippets can help prevent common errors:
Saving an Image Safely:
“`csharp
using (Bitmap bitmap = new Bitmap(100, 100))
{
// Drawing operations here
try
{
bitmap.Save(@”C:\Images\image.png”, ImageFormat.Png);
}
catch (Exception ex)
{
Console.WriteLine($”Error: {ex.Message}”);
}
}
“`
Loading an Image with Validation:
“`csharp
Image img = null;
try
{
img = Image.FromFile(@”C:\Images\image.png”);
}
catch (FileNotFoundException)
{
Console.WriteLine(“File not found.”);
}
catch (OutOfMemoryException)
{
Console.WriteLine(“Not a valid image format.”);
}
finally
{
img?.Dispose();
}
“`
Best Practices
To minimize the chances of encountering GDI+ errors, adhere to the following best practices:
- Use Exception Handling: Implement robust exception handling to capture and log errors gracefully.
- Optimize Image Size: Work with appropriately sized images to reduce memory usage.
- Dispose Resources: Always dispose of image objects and streams to free up system resources.
- Regular Updates: Keep your .NET framework and GDI+ library updated to benefit from the latest fixes and improvements.
By following these guidelines and understanding the underlying causes of the “generic error occurred in GDI+,” developers can effectively troubleshoot and prevent issues in their applications.
Understanding the GDI+ Generic Error: Expert Insights
Dr. Emily Carter (Senior Software Engineer, GraphicsTech Solutions). “The ‘generic error occurred in GDI+’ typically arises from improper file access permissions or issues with the image file itself. Ensuring that the application has the necessary rights to read and write files can often resolve this issue.”
Mark Thompson (Lead Developer, Visual Graphics Innovations). “In many cases, this error is linked to memory issues or resource constraints. It is crucial to monitor system resources and optimize image handling in your application to prevent such errors from occurring.”
Linda Garcia (IT Support Specialist, TechHelp Inc.). “Users frequently encounter the ‘generic error occurred in GDI+’ when dealing with unsupported image formats or corrupted files. Implementing robust error handling and validating file formats before processing can significantly mitigate these problems.”
Frequently Asked Questions (FAQs)
What does “a generic error occurred in GDI+” mean?
This error typically indicates a problem with the Graphics Device Interface (GDI+) in Windows, often arising from issues related to file access, permissions, or resource availability when attempting to manipulate images or graphics.
What are common causes of the GDI+ generic error?
Common causes include insufficient permissions to access the file, the file being in use by another process, attempting to save to a read-only location, or issues with the image format or corruption.
How can I resolve the GDI+ generic error?
To resolve this error, ensure that the application has the necessary permissions, check if the file is not in use, verify the file path is correct, and confirm that the image format is supported and not corrupted.
Does this error occur in specific programming environments?
Yes, this error is frequently encountered in .NET applications, particularly when using classes like `Bitmap`, `Image`, or `Graphics` for image processing tasks.
Can antivirus software cause the GDI+ generic error?
Yes, antivirus software can sometimes interfere with file access, leading to the GDI+ generic error. Temporarily disabling the antivirus or adding exceptions for specific applications may help resolve the issue.
Is there a way to prevent the GDI+ generic error in future applications?
To prevent this error, implement proper error handling, ensure file paths are valid, manage file access appropriately, and validate image formats before processing them in your applications.
The error message “A generic error occurred in GDI+” is a common issue encountered in applications that utilize the Graphics Device Interface (GDI+) for rendering graphics in .NET environments. This error can arise from various underlying causes, including file access permissions, invalid file paths, or issues related to memory resources. Understanding the context in which the error occurs is crucial for effective troubleshooting and resolution.
One of the primary factors contributing to this error is file access permissions. When an application attempts to write to a file without the necessary permissions, GDI+ generates this generic error. Additionally, attempting to access a file that is already open or locked by another process can lead to similar issues. Therefore, ensuring that the application has the appropriate permissions and that files are not in use is essential for preventing this error.
Another important aspect to consider is the format and validity of the files being processed. GDI+ supports various image formats, but if an application tries to load a corrupted or unsupported file, it may trigger the generic error. Developers should implement error handling mechanisms to catch such exceptions and provide meaningful feedback to users. Moreover, checking the integrity of image files before processing can help mitigate this issue.
In summary, the “A
Author Profile
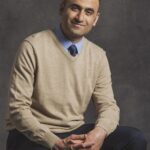
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?