How Do You Create an A Href Link to JavaScript?
Introduction
In the ever-evolving landscape of web development, the interplay between HTML and JavaScript plays a pivotal role in creating dynamic, interactive user experiences. One of the fundamental elements of this interaction is the anchor tag, or `` tag, which traditionally serves to link users to different pages or resources. However, as developers seek to push the boundaries of what’s possible on the web, the concept of linking directly to JavaScript has emerged as a powerful tool. This article will delve into the intriguing world of using the `` tag to trigger JavaScript functions, exploring its implications, benefits, and best practices.
As we navigate through this topic, we will uncover how the simple act of clicking a link can transform into a sophisticated event that enhances user engagement. By leveraging the capabilities of JavaScript within anchor tags, developers can create seamless transitions, interactive elements, and even complex applications that respond to user actions in real-time. This approach not only streamlines the user experience but also opens up a realm of possibilities for creative web solutions.
Moreover, we will discuss the importance of understanding the underlying mechanics of this technique. From improving accessibility to optimizing performance, knowing how to effectively implement JavaScript within anchor tags can significantly impact the overall functionality of a website. Join us
Understanding the `a href` Attribute in JavaScript
The `a` (anchor) tag in HTML is primarily used to create hyperlinks. The `href` attribute specifies the URL of the page the link goes to. However, when integrating JavaScript, the `href` attribute can also be leveraged to execute JavaScript code directly when the link is clicked. This mechanism allows developers to create interactive web applications that respond to user actions without requiring a full page reload.
To create a link that executes JavaScript, you can set the `href` attribute to `javascript:` followed by the code you want to execute. Here’s a basic example:
When a user clicks this link, an alert box will pop up displaying “Hello World!”.
Best Practices for Using `javascript:` in Links
While using `javascript:` in the `href` attribute can be convenient, it is generally not recommended for various reasons, including maintainability, accessibility, and separation of concerns. Here are some best practices to consider:
- Use Event Listeners: Instead of embedding JavaScript in the `href`, use event listeners in JavaScript to handle clicks. This keeps HTML and JavaScript separate.
- Accessibility Considerations: Ensure that using JavaScript does not hinder accessibility. Screen readers may not interpret `javascript:` links correctly. Use semantic HTML elements like buttons for actions, which are inherently more accessible.
- Avoid Inline JavaScript: Inline JavaScript can lead to security risks, such as Cross-Site Scripting (XSS). Always sanitize any input or data that could be manipulated.
Common Use Cases for `javascript:` Links
Despite the caveats, there are scenarios where using `javascript:` links can be beneficial. Here are some common use cases:
- Dynamic Content Loading: You can use `javascript:` to load content dynamically without refreshing the page.
- Quick Navigation: For quick actions, like toggling visibility of elements, `javascript:` links can provide instant feedback.
- Bookmarklets: These are small JavaScript programs stored as the URL of a bookmark in a web browser, often initiated via `javascript:` links.
Use Case | Example Code | Benefits |
---|---|---|
Alert Message | <a href="javascript:alert('Hello!');">Alert</a> |
Simple feedback |
Dynamic Content | <a href="javascript:loadContent();">Load Content</a> |
Improves user experience |
Quick Form Submission | <a href="javascript:submitForm();">Submit</a> |
Streamlined process |
By understanding the implications of using `javascript:` in `href`, developers can make informed decisions that enhance both functionality and user experience.
Understanding `href` Attributes in JavaScript
The `href` attribute is commonly associated with anchor (``) tags in HTML, defining the link’s destination. However, JavaScript can dynamically manipulate these attributes, allowing for interactive web applications. This section will explore how to leverage JavaScript for handling `href` attributes effectively.
Modifying `href` Attributes with JavaScript
JavaScript can be used to change the `href` attribute of anchor elements on-the-fly. This can be achieved through the Document Object Model (DOM). Below are methods to modify `href` attributes:
- Using `getElementById`:
javascript
document.getElementById(‘myLink’).href = ‘https://www.example.com’;
- Using `querySelector`:
javascript
document.querySelector(‘.myClass’).href = ‘https://www.example.com’;
- Using `getElementsByClassName`:
javascript
var links = document.getElementsByClassName(‘myClass’);
for (var i = 0; i < links.length; i++) {
links[i].href = 'https://www.example.com';
}
Creating Dynamic Links
Dynamic links can be generated in response to user actions or events. Here’s how to create a link programmatically:
javascript
var link = document.createElement(‘a’);
link.href = ‘https://www.example.com’;
link.innerText = ‘Visit Example’;
document.body.appendChild(link);
This code snippet creates a new anchor element, sets its `href`, and appends it to the body of the document.
JavaScript Event Handling for Links
Event handling can add interactivity to links. You can prevent the default action of a link and execute JavaScript instead:
javascript
document.getElementById(‘myLink’).addEventListener(‘click’, function(event) {
event.preventDefault(); // Prevent the default link behavior
alert(‘Link clicked!’); // Execute custom code
});
This example alerts the user when a link is clicked, rather than navigating to the link’s destination.
Linking to JavaScript Functions
You can link anchor tags directly to JavaScript functions by using the `onclick` attribute in HTML:
Alternatively, you can attach the event listener in JavaScript:
javascript
document.getElementById(‘myLink’).onclick = myFunction;
function myFunction() {
// Custom function logic
alert(‘Function executed!’);
}
Best Practices for Using `href` with JavaScript
When working with `href` attributes in JavaScript, consider the following best practices:
- Always Use `event.preventDefault()`: Prevent default actions when necessary to avoid unwanted navigation.
- Provide Fallbacks: Ensure that if JavaScript is disabled, your links still lead to the correct destinations.
- Semantic HTML: Use appropriate tags and attributes for accessibility, ensuring that links are recognizable as such.
- Performance Optimization: Minimize DOM manipulations for better performance, especially in larger applications.
Common Use Cases for JavaScript Links
Use Case | Description |
---|---|
Modal Triggers | Use links to open modal dialogs without navigation. |
AJAX Loading | Link actions that load content dynamically via AJAX. |
Single Page Applications | Update view without refreshing the page. |
Form Submissions | Redirect after form submission using JavaScript. |
By following these guidelines, developers can create robust, interactive web experiences that effectively utilize the `href` attributes in conjunction with JavaScript.
Expert Insights on Using `a href` with JavaScript
Emily Carter (Senior Web Developer, Tech Innovations Inc.). “Using `a href` with JavaScript is a powerful technique that allows developers to create interactive web applications. However, it is crucial to ensure that the links are accessible and provide meaningful navigation for all users.”
Michael Chen (JavaScript Framework Specialist, CodeCraft Solutions). “When integrating JavaScript with `a href`, one must consider the implications for SEO and user experience. Properly managing `href` attributes can enhance both the visibility of a site and its usability.”
Sarah Patel (UX/UI Designer, Creative Web Agency). “The combination of `a href` and JavaScript can significantly improve user engagement. However, it is essential to maintain a balance between functionality and design to avoid overwhelming users with too many interactive elements.”
Frequently Asked Questions (FAQs)
What is an “a href” in HTML?
An “a href” is an anchor tag in HTML used to create hyperlinks. The “href” attribute specifies the URL of the page the link goes to.
How can I link to a JavaScript function using “a href”?
You can link to a JavaScript function by using the “href” attribute with “javascript:void(0);” and adding an “onclick” event to the anchor tag to call the function.
Is it possible to execute JavaScript code directly in an “a href”?
Yes, you can execute JavaScript code directly in an “a href” by using the “javascript:” protocol followed by the code you want to execute. However, this is generally discouraged for security and readability reasons.
What are the best practices for using “a href” with JavaScript?
Best practices include avoiding inline JavaScript, using event listeners instead of “onclick” attributes, and ensuring that links are accessible and usable even when JavaScript is disabled.
Can I prevent the default action of an “a href” link in JavaScript?
Yes, you can prevent the default action of an “a href” link by using the event.preventDefault() method within an event handler.
What are the security implications of using “a href” with JavaScript?
Using “a href” with JavaScript can expose your site to security risks such as cross-site scripting (XSS) if not handled properly. Always validate and sanitize any user input and avoid executing untrusted code.
In summary, the use of the `` tag in conjunction with JavaScript serves as a powerful tool for web developers seeking to enhance user interaction and navigation within web applications. By utilizing JavaScript to manipulate the behavior of anchor links, developers can create dynamic content, manage single-page applications, and improve the overall user experience. This integration allows for a seamless transition between different sections of a webpage or even different web pages without the need for full page reloads.
Moreover, it is essential to understand the implications of using JavaScript with anchor tags, particularly regarding accessibility and SEO. Developers should ensure that any JavaScript-driven navigation is still accessible to users relying on assistive technologies. Additionally, proper implementation of the `` tag with JavaScript can contribute positively to search engine optimization by maintaining a clear structure and hierarchy within the website’s content.
Author Profile
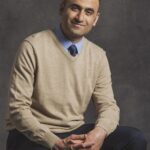
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?