How Can You Master Linux Commands, Editors, and Shell Programming with This Practical Guide?
In the ever-evolving landscape of technology, mastering the command line can feel like unlocking a hidden realm of possibilities. For those venturing into the world of Linux, understanding commands, editors, and shell programming is not just a skill—it’s a gateway to enhanced productivity and control over your computing environment. Whether you’re a budding developer, a system administrator, or simply a curious learner, this practical guide will illuminate the essential tools and techniques that can elevate your Linux experience.
Navigating the command line may initially seem daunting, but it empowers users to perform tasks with speed and precision. From file manipulation to system monitoring, the myriad of commands available in Linux provides a robust toolkit for managing your system efficiently. This guide will introduce you to the most commonly used commands, helping you build a solid foundation that will serve you well in various scenarios.
Moreover, the choice of text editors can significantly influence your workflow. With options ranging from lightweight editors like Nano to powerful IDEs like Vim, understanding their features and functionalities is crucial for effective coding and scripting. As we delve into shell programming, you’ll discover how to automate tasks, streamline processes, and harness the full potential of your Linux environment. Prepare to embark on a journey that will transform the way you interact with your system, making you
Linux Command Line Basics
The Linux command line is a powerful interface that allows users to interact with the operating system. Understanding basic commands is essential for efficient navigation and task execution. Here are some of the fundamental commands:
- `ls`: Lists directory contents.
- `cd`: Changes the current directory.
- `pwd`: Displays the current working directory.
- `cp`: Copies files or directories.
- `mv`: Moves or renames files or directories.
- `rm`: Removes files or directories.
To further illustrate how these commands work, consider the following table:
Command | Description | Example |
---|---|---|
ls | List files in the current directory | ls -l |
cd | Change directory | cd /home/user/Documents |
pwd | Print working directory | pwd |
cp | Copy a file | cp file.txt /home/user/Documents/ |
mv | Move or rename a file | mv oldname.txt newname.txt |
rm | Remove a file | rm file.txt |
Text Editors in Linux
Linux offers various text editors, each catering to different user preferences. The most widely used editors include:
- Nano: A user-friendly command-line text editor ideal for beginners.
- Vim: A powerful editor with a steeper learning curve but extensive capabilities.
- Emacs: An extensible editor that can be customized and is particularly favored by programmers.
For example, to create and edit a file using Nano, you would use the command:
“`bash
nano filename.txt
“`
In Vim, you would enter:
“`bash
vim filename.txt
“`
To save changes in Nano, press `CTRL + O`, followed by `CTRL + X` to exit. In Vim, you would type `:wq` to save and quit.
Shell Programming Basics
Shell programming allows users to automate tasks through scripts. A shell script is a file that contains a series of commands that the shell can execute. Here are some key components of shell scripts:
- Shebang: The first line of the script, indicating the interpreter to be used. For example, `!/bin/bash`.
- Variables: Used to store data. For example, `name=”John”`.
- Control Structures: Conditional statements and loops allow for decision-making and repetition.
Here’s a simple shell script example:
“`bash
!/bin/bash
echo “Hello, $name”
“`
To execute a shell script, you must first make it executable using:
“`bash
chmod +x script.sh
“`
Then run it with:
“`bash
./script.sh
“`
File Permissions in Linux
Understanding file permissions is crucial for system security. Each file in Linux has associated permissions that dictate who can read, write, or execute the file. Permissions are represented as follows:
- r: Read
- w: Write
- x: Execute
Permissions are displayed when using the `ls -l` command. The output will show the permissions in a format like `-rwxr-xr–`, where the first character indicates the file type, followed by permissions for the owner, group, and others.
To change file permissions, the `chmod` command is used. For example, to grant execute permissions to the owner, you would use:
“`bash
chmod u+x filename
“`
Understanding these components of Linux commands, text editors, shell programming, and file permissions is essential for effective system management and programming.
Understanding Linux Commands
Linux commands are the backbone of interacting with the operating system. Knowing how to effectively use these commands can significantly enhance productivity.
Basic Command Structure:
- Command: The instruction to be executed.
- Options: Modifiers that alter the command’s behavior (often preceded by a dash).
- Arguments: The files or directories the command acts upon.
Examples:
- `ls -l /home/user`:
- `ls`: command to list directory contents.
- `-l`: option for long listing format.
- `/home/user`: argument specifying the directory.
Common Commands:
- `pwd`: Print working directory.
- `cd`: Change directory.
- `cp`: Copy files and directories.
- `mv`: Move or rename files and directories.
- `rm`: Remove files or directories.
Text Editors in Linux
Linux offers several text editors, each with unique features. The choice of editor can depend on user preference and the specific task at hand.
Popular Editors:
- nano: A simple, user-friendly editor.
- vim: A powerful editor with a steep learning curve, ideal for advanced users.
- emacs: Highly customizable and extensible, suitable for programming.
Basic Commands for Editors:
Editor | Command | Description |
---|---|---|
nano | `Ctrl + O` | Save file |
nano | `Ctrl + X` | Exit editor |
vim | `:w` | Save file |
vim | `:q` | Quit editor |
emacs | `Ctrl + X, Ctrl + S` | Save file |
emacs | `Ctrl + X, Ctrl + C` | Exit editor |
Shell Programming Basics
Shell programming allows users to write scripts that automate tasks and enhance efficiency.
Key Components:
- Shebang: `!/bin/bash` indicates the script’s interpreter.
- Comments: Lines starting with “ are ignored by the interpreter.
- Variables: Store data for later use, e.g., `myVar=”Hello World”`.
Basic Script Structure:
“`bash
!/bin/bash
This is a comment
echo “Hello, World!” Output text to the terminal
“`
Control Structures:
- Conditional Statements:
“`bash
if [ condition ]; then
commands
fi
“`
- Loops:
“`bash
for i in {1..5}; do
echo “Number $i”
done
“`
Best Practices:
- Use descriptive variable names.
- Comment your code for clarity.
- Test scripts in a safe environment before deploying.
Getting Help with Commands
Linux provides several ways to access help for commands, which is essential for learning and troubleshooting.
Help Options:
- `man [command]`: Displays the manual page for the command.
- `command –help`: Provides a summary of command options.
- `info [command]`: Accesses more detailed documentation.
Example:
- `man ls`: Opens the manual for the `ls` command, detailing its usage and options.
By mastering these elements, users can significantly improve their efficiency and proficiency in Linux environments.
Expert Insights on Mastering Linux Commands and Shell Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Understanding Linux commands and shell programming is essential for any developer. Mastery of these tools not only enhances productivity but also provides a deeper understanding of system operations, which is crucial in today’s technology landscape.”
Mark Thompson (Linux Systems Administrator, Open Source Solutions). “A practical guide to Linux commands should emphasize hands-on practice. The best way to learn is by using the terminal for real tasks, which helps in solidifying concepts and improving efficiency in shell scripting.”
Linda Garcia (Technical Writer, Linux Journal). “When creating a guide for Linux editors and shell programming, clarity is key. Providing clear examples and use cases can bridge the gap between theory and practice, making it easier for newcomers to grasp these powerful tools.”
Frequently Asked Questions (FAQs)
What are the essential Linux commands for beginners?
Essential Linux commands for beginners include `ls` for listing directory contents, `cd` for changing directories, `cp` for copying files, `mv` for moving or renaming files, and `rm` for removing files. These commands form the foundation for navigating and managing files in a Linux environment.
How do I choose a text editor for Linux?
Choosing a text editor depends on user preference and task requirements. Popular choices include `nano` for simplicity, `vim` for advanced features and efficiency, and `gedit` for a graphical interface. Each editor has its strengths, so users should try a few to determine which best suits their workflow.
What is shell programming, and why is it important?
Shell programming involves writing scripts to automate tasks in a Unix/Linux shell environment. It is important because it allows users to execute complex sequences of commands, manage system operations, and streamline repetitive tasks, significantly enhancing productivity and efficiency.
How can I create and run a shell script in Linux?
To create a shell script, use a text editor to write your commands and save the file with a `.sh` extension. Make the script executable with the command `chmod +x script.sh`. Run the script by typing `./script.sh` in the terminal. Ensure the script has the appropriate shebang (e.g., `!/bin/bash`) at the top.
What are some common errors encountered in shell scripting?
Common errors in shell scripting include syntax errors, incorrect file permissions, and issues with variable scope. Additionally, forgetting to use the correct shebang line or misusing command substitutions can lead to script failures. Debugging tools such as `bash -x script.sh` can help identify errors.
Where can I find resources to learn more about Linux commands and shell programming?
Resources for learning Linux commands and shell programming include online tutorials, documentation such as the Linux man pages, and books like “The Linux Command Line” by William Shotts. Websites like Linux Journey and Codecademy also offer interactive learning experiences.
In summary, a practical guide to Linux commands, editors, and shell programming serves as an essential resource for both beginners and experienced users. It emphasizes the importance of mastering fundamental Linux commands, which are the building blocks for effective system navigation and file management. Understanding these commands not only enhances productivity but also empowers users to perform complex tasks with ease.
Furthermore, the guide delves into various text editors available in Linux, such as Vim, Nano, and Emacs. Each editor offers unique features and functionalities that cater to different user preferences and workflows. By familiarizing oneself with these editors, users can efficiently manipulate text files and scripts, making the editing process more streamlined and effective.
Lastly, the exploration of shell programming highlights the significance of scripting in automating tasks and improving system management. Learning how to write and execute shell scripts enables users to perform repetitive tasks with minimal effort, thus optimizing their workflow. Overall, this guide equips users with the necessary skills to navigate the Linux environment confidently and effectively.
Author Profile
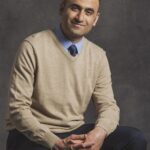
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?