How Can You Implement the A* Algorithm in Python?
In the realm of computer science and artificial intelligence, pathfinding algorithms are essential tools that help us navigate complex environments and make optimal decisions. Among these algorithms, the A* (A-star) algorithm stands out as a powerful and efficient method for finding the shortest path between two points. Whether you’re developing a video game, designing a robotics navigation system, or tackling logistical challenges, understanding the A* algorithm can significantly enhance your problem-solving toolkit. In this article, we will delve into the intricacies of the A* algorithm, its implementation in Python, and its myriad applications across various fields.
The A* algorithm combines the strengths of Dijkstra’s algorithm and heuristic-based approaches, allowing it to efficiently traverse graphs while minimizing the total cost of the path. At its core, A* uses a cost function that evaluates both the distance traveled and an estimated distance to the goal, guiding the search process toward the most promising paths. This unique blend of exploration and exploitation makes A* particularly effective in dynamic environments where quick decision-making is crucial.
As we explore the A* algorithm in Python, we will uncover its fundamental components, including the open and closed sets, the role of heuristics, and how to implement it in a way that is both efficient and easy to understand. By
A* Algorithm Implementation
The A* (A-star) algorithm is a popular pathfinding and graph traversal algorithm. It is widely used in various applications, including game development and robotics, due to its efficiency and optimality. Below is a comprehensive guide on how to implement the A* algorithm in Python.
Key Components of A* Algorithm
To effectively implement the A* algorithm, it is essential to understand its key components:
- Graph Representation: The algorithm works on a graph structure, where nodes represent states and edges represent the cost to move from one node to another.
- Heuristic Function (h): This function estimates the cost from the current node to the goal. A common heuristic for grid-based maps is the Euclidean distance or Manhattan distance.
- Cost Function (g): This function represents the cost from the start node to the current node.
- F-cost (f): The total cost for each node is calculated as \( f(n) = g(n) + h(n) \). The algorithm prioritizes nodes with the lowest f-cost.
Python Implementation
Here is an example implementation of the A* algorithm in Python:
“`python
import heapq
class Node:
def __init__(self, position, parent=None):
self.position = position
self.parent = parent
self.g = 0 Cost from start to this node
self.h = 0 Heuristic cost from this node to goal
self.f = 0 Total cost
def __eq__(self, other):
return self.position == other.position
def heuristic(a, b):
return abs(a[0] – b[0]) + abs(a[1] – b[1]) Manhattan distance
def a_star(start, goal, grid):
open_list = []
closed_list = []
start_node = Node(start)
goal_node = Node(goal)
heapq.heappush(open_list, (start_node.f, start_node))
while open_list:
current_node = heapq.heappop(open_list)[1]
closed_list.append(current_node)
if current_node == goal_node:
path = []
while current_node:
path.append(current_node.position)
current_node = current_node.parent
return path[::-1] Return reversed path
neighbors = [(0, 1), (1, 0), (0, -1), (-1, 0)] 4 possible directions
for new_position in neighbors:
node_position = (current_node.position[0] + new_position[0], current_node.position[1] + new_position[1])
if not (0 <= node_position[0] < len(grid)) or not (0 <= node_position[1] < len(grid[0])) or grid[node_position[0]][node_position[1]] != 0:
continue
neighbor_node = Node(node_position, current_node)
if neighbor_node in closed_list:
continue
neighbor_node.g = current_node.g + 1
neighbor_node.h = heuristic(neighbor_node.position, goal_node.position)
neighbor_node.f = neighbor_node.g + neighbor_node.h
if add_to_open(open_list, neighbor_node):
heapq.heappush(open_list, (neighbor_node.f, neighbor_node))
return None No path found
def add_to_open(open_list, neighbor):
for node in open_list:
if neighbor == node[1] and neighbor.g > node[1].g:
return
return True
“`
Example Usage
To use the above implementation, define a grid, where `0` represents walkable space and `1` represents obstacles:
“`python
grid = [
[0, 0, 0, 0, 0],
[0, 1, 1, 1, 0],
[0, 0, 0, 1, 0],
[0, 1, 0, 0, 0],
[0, 0, 0, 0, 0]
]
start = (0, 0)
goal = (4, 4)
path = a_star(start, goal, grid)
print(“Path from start to goal:”, path)
“`
Performance Considerations
When implementing the A* algorithm, several factors can affect its performance:
- Heuristic Quality: The efficiency of A* heavily depends on the quality of the heuristic function. A better heuristic leads to a faster search.
- Graph Density: The structure and density of the graph can impact the algorithm’s speed. Sparse graphs generally perform better.
- Memory Usage: A* maintains two lists (open and closed), which can lead to high memory usage in large graphs.
Aspect | Impact |
---|---|
Heuristic Function | Higher efficiency with better heuristics |
Graph Density | Sparse graphs usually yield faster results |
Memory Usage | Increased memory usage with larger graphs |
This implementation and analysis provide a foundational understanding of the A* algorithm, offering practical insights into its application in Python programming.
A* Algorithm Overview
The A* algorithm is a popular pathfinding and graph traversal method used in various applications, such as AI for games and robotics. It employs heuristics to improve efficiency in finding the shortest path from a start node to a target node.
Key components of the A* algorithm include:
- g(n): The cost of the path from the start node to node n.
- h(n): The estimated cost from node n to the target node. This heuristic function should be admissible, meaning it never overestimates the true cost.
- f(n): The total estimated cost of the cheapest solution through node n, calculated as:
\[
f(n) = g(n) + h(n)
\]
Implementation in Python
Here is a basic implementation of the A* algorithm in Python. This example uses a grid-based approach.
“`python
import heapq
class Node:
def __init__(self, position, g=0, h=0):
self.position = position
self.g = g Cost from start to node
self.h = h Heuristic cost to end
self.f = g + h Total cost
def __lt__(self, other):
return self.f < other.f
def a_star(start, goal, grid):
open_list = []
closed_list = set()
start_node = Node(start, 0, heuristic(start, goal))
heapq.heappush(open_list, start_node)
while open_list:
current_node = heapq.heappop(open_list)
if current_node.position == goal:
return reconstruct_path(current_node)
closed_list.add(current_node.position)
for neighbor in get_neighbors(current_node.position, grid):
if neighbor in closed_list:
continue
g_cost = current_node.g + 1 Assuming uniform cost for simplicity
h_cost = heuristic(neighbor, goal)
neighbor_node = Node(neighbor, g_cost, h_cost)
if add_to_open(open_list, neighbor_node):
heapq.heappush(open_list, neighbor_node)
return None No path found
def heuristic(a, b):
return abs(a[0] - b[0]) + abs(a[1] - b[1]) Manhattan distance
def get_neighbors(position, grid):
neighbors = []
for dx, dy in [(-1, 0), (1, 0), (0, -1), (0, 1)]:
new_position = (position[0] + dx, position[1] + dy)
if 0 <= new_position[0] < len(grid) and 0 <= new_position[1] < len(grid[0]) and grid[new_position[0]][new_position[1]] == 0:
neighbors.append(new_position)
return neighbors
def add_to_open(open_list, neighbor_node):
for node in open_list:
if neighbor_node.position == node.position and neighbor_node.g >= node.g:
return
return True
def reconstruct_path(node):
path = []
while node:
path.append(node.position)
node = node.parent if hasattr(node, ‘parent’) else None
return path[::-1]
“`
Key Considerations
When implementing the A* algorithm, several factors can influence its performance and effectiveness:
- Heuristic Choice: The choice of the heuristic function significantly affects the algorithm’s efficiency. Common heuristics include:
- Manhattan distance
- Euclidean distance
- Diagonal distance
- Grid Representation: The grid can be represented as a 2D list where:
- 0 represents walkable paths
- 1 represents obstacles
- Open List Management: Using a priority queue (like Python’s `heapq`) is crucial to efficiently manage the open list.
- Path Reconstruction: Store the parent node of each node to enable path reconstruction once the goal is reached.
By adhering to these principles, the A* algorithm can be effectively utilized for various pathfinding applications.
Expert Insights on Implementing the A* Algorithm in Python
Dr. Emily Carter (AI Research Scientist, Tech Innovations Lab). “The A* algorithm is a powerful pathfinding and graph traversal method that can be efficiently implemented in Python. By leveraging data structures such as priority queues, Python developers can optimize the algorithm’s performance, making it suitable for both small and large datasets.”
Michael Chen (Software Engineer, Smart Robotics Inc.). “When implementing the A* algorithm in Python, it is crucial to define an effective heuristic function. This function significantly influences the algorithm’s efficiency and accuracy in finding the shortest path. Using libraries like NumPy can also enhance computational speed.”
Laura Patel (Data Scientist, Urban Mobility Solutions). “Python’s versatility allows for easy integration of the A* algorithm into various applications, from game development to navigation systems. Utilizing visualization libraries like Matplotlib can help in debugging and understanding the algorithm’s decision-making process.”
Frequently Asked Questions (FAQs)
What is the A* algorithm?
The A* algorithm is a pathfinding and graph traversal algorithm that is widely used in computer science and artificial intelligence. It efficiently finds the shortest path from a starting node to a target node by combining features of Dijkstra’s algorithm and Greedy Best-First Search.
How does the A* algorithm work?
The A* algorithm works by maintaining a priority queue of nodes to explore, using a cost function that combines the actual cost from the start node and a heuristic estimate of the cost to reach the target node. It selects the node with the lowest total cost for exploration until the target is reached.
What are the key components of the A* algorithm?
The key components of the A* algorithm include the open set (nodes to be evaluated), the closed set (nodes already evaluated), the cost from the start node (g(n)), the heuristic cost to the target (h(n)), and the total estimated cost (f(n) = g(n) + h(n)).
How can I implement the A* algorithm in Python?
To implement the A* algorithm in Python, define a graph structure, create a priority queue for the open set, and use a loop to evaluate nodes based on their f(n) values. Utilize a heuristic function appropriate for your specific problem domain to guide the search.
What are common heuristics used with the A* algorithm?
Common heuristics for the A* algorithm include the Manhattan distance, Euclidean distance, and Chebyshev distance. The choice of heuristic depends on the specific problem and the nature of the graph being traversed.
What are the advantages of using the A* algorithm?
The A* algorithm is advantageous due to its optimality and completeness when using an admissible heuristic. It efficiently finds the shortest path while minimizing the number of nodes evaluated, making it suitable for large and complex graphs.
The A* algorithm is a widely used pathfinding and graph traversal algorithm that is particularly effective in scenarios where the goal is to find the shortest path from a start node to a target node. Implemented in Python, the A* algorithm combines features of Dijkstra’s algorithm and Greedy Best-First Search, utilizing a heuristic to improve efficiency. This makes it suitable for applications in various fields, including robotics, gaming, and network routing.
One of the key insights into the A* algorithm is its reliance on a cost function, typically denoted as f(n) = g(n) + h(n). Here, g(n) represents the cost to reach the current node from the start node, while h(n) is the heuristic estimate of the cost to reach the goal from the current node. The choice of heuristic is crucial, as it can significantly affect the algorithm’s performance and efficiency. A well-designed heuristic can lead to faster convergence on the optimal path.
Another important takeaway is the implementation of the A* algorithm in Python, which can be achieved using data structures such as priority queues to manage the open set of nodes efficiently. The algorithm’s flexibility allows for easy adaptation to various types of graphs and terrains, making it a versatile choice
Author Profile
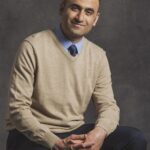
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?