How to Fix the ‘AAPT: Error: Resource Android:Attr/Lstar Not Found’ Issue in Your Android Project?
In the ever-evolving landscape of Android development, encountering errors is an inevitable part of the journey. One such perplexing error that developers may face is the infamous `aapt: error: resource android:attr/lstar not found`. This cryptic message can halt progress and leave even seasoned programmers scratching their heads. Understanding the underlying causes of this error is crucial for maintaining the momentum of your development process and ensuring that your applications run smoothly.
The `lstar` attribute is a relatively recent addition to the Android framework, tied closely to the themes and styles that define the user interface of modern applications. When this attribute is not recognized, it can signal a range of issues, from outdated SDK versions to missing dependencies in your project setup. As developers strive to create visually appealing and functionally robust applications, addressing this error becomes essential not only for troubleshooting but also for optimizing the overall user experience.
In this article, we will delve into the intricacies of the `lstar` attribute, exploring its significance within the Android ecosystem and the common pitfalls that lead to the `aapt` error. By gaining a clearer understanding of this issue, developers can better navigate the challenges of Android development, ensuring their applications are both innovative and compliant with the latest standards. Whether you’re
Understanding the Error
The error message `aapt: error: resource android:attr/lstar not found` typically arises during the build process of an Android application. This error indicates that the resource specified, in this case, `lstar`, is not recognized by the Android Asset Packaging Tool (AAPT).
This can occur due to several reasons, including:
- Using a version of Android SDK that does not support the attribute.
- Missing dependencies or libraries that define the `lstar` attribute.
- Issues with the Gradle build configuration.
Common Causes
To effectively resolve this issue, it’s essential to understand its common causes:
- SDK Version: The `lstar` attribute was introduced in Android API level 31 (Android 12). If your project targets a lower API level, you may encounter this error.
- Gradle Build Tools: Ensure that your build tools version is up to date. An outdated version may not support newer attributes.
- Library Compatibility: If you are using third-party libraries, verify that they are compatible with the target SDK version of your app.
Solutions
To fix the error, consider the following approaches:
– **Update SDK**: Make sure you are using the latest Android SDK. You can do this via Android Studio by navigating to:
“`
Tools -> SDK Manager -> SDK Platforms
“`
- Change Target SDK Version: If your application does not specifically require features from higher API levels, you can downgrade the target SDK version in your `build.gradle` file:
“`groovy
android {
compileSdkVersion 30 // Use a compatible version
…
}
“`
- Check Dependencies: Ensure all dependencies in your `build.gradle` file are compatible with the specified SDK version.
- Update Gradle: Upgrade the Gradle version and Android Gradle Plugin:
“`groovy
dependencies {
classpath ‘com.android.tools.build:gradle:7.0.0’ // Example version
}
“`
Example Configuration
Here’s an example of how to configure your `build.gradle` file to avoid the `lstar` error:
“`groovy
android {
compileSdkVersion 31 // or the latest version
defaultConfig {
applicationId “com.example.myapp”
minSdkVersion 21
targetSdkVersion 31 // Ensure this is at least 31 for ‘lstar’
versionCode 1
versionName “1.0”
}
…
}
“`
Troubleshooting Steps
If the problem persists after making the above changes, consider the following troubleshooting steps:
– **Clean and Rebuild**: Sometimes, residual build artifacts can cause issues. Clean your project and rebuild it.
“`
Build -> Clean Project
Build -> Rebuild Project
“`
– **Invalidate Caches**: Invalidate caches in Android Studio to resolve any caching issues.
“`
File -> Invalidate Caches / Restart…
“`
- Check Logcat: Use Logcat to review detailed error messages that may provide more context on the issue.
Resources for Further Help
If none of these solutions resolve the issue, consider consulting the following resources:
- Android Developer Documentation: Provides comprehensive guidelines and updates regarding attributes and SDK versions.
- Stack Overflow: A community-driven platform where developers share solutions to similar issues.
- Official Android Issue Tracker: Report unresolved issues or check existing reports for further insights.
SDK Version | Attribute Availability |
---|---|
30 | Not Available |
31 | Available |
Understanding the Error
The error message `aapt: error: resource android:attr/lstar not found` typically occurs in Android development when attempting to compile an application. This issue arises due to the use of attributes that are not available in the current Android SDK version being targeted. The `lstar` attribute specifically refers to a newer feature that may not be present in older SDK versions.
Common Causes
Several factors can contribute to encountering this error:
- Targeting an Incompatible SDK Version: The attribute may have been introduced in a version higher than the one currently being targeted in your project’s `build.gradle` file.
- Using Legacy Libraries: Some libraries or dependencies may reference newer attributes that are unsupported in the project’s current configuration.
- Gradle Configuration Issues: Incorrect settings in your Gradle files can lead to mismatches between the SDK version and project dependencies.
Resolution Steps
To resolve the `lstar` resource error, consider the following steps:
- **Check Compile SDK Version**:
- Open your `build.gradle` (Module: app) file.
- Ensure that the `compileSdkVersion` is set to the latest version available, ideally at least API level 31 (Android 12) or higher.
“`groovy
android {
compileSdkVersion 31
…
}
“`
- **Update Build Tools**:
- Ensure you are using the latest version of the Android build tools.
- Update your `build.gradle` file:
“`groovy
dependencies {
classpath ‘com.android.tools.build:gradle:7.0.0’ // or the latest version
}
“`
- **Review Dependencies**:
- Check your dependencies to ensure they are compatible with the target SDK.
- Update any outdated libraries that may reference the `lstar` attribute.
- **Invalidate Caches and Restart**:
- In Android Studio, go to `File` > `Invalidate Caches / Restart`.
- This action helps clear any cached data that might be causing the error.
- Ensure Proper Resource Management:
- If you are defining custom styles or themes, verify that no references to `lstar` exist unless explicitly required and supported.
Alternative Workarounds
If updating the SDK is not feasible, you can consider the following workarounds:
- Conditional Resource Use: Implement resource qualifiers to ensure that specific attributes are only applied to devices running compatible versions.
- Custom Implementations: Create alternative styles or attributes that do not rely on the `lstar` attribute for backward compatibility.
Key Takeaways
To effectively manage the `aapt: error: resource android:attr/lstar not found`, developers should focus on aligning their project configurations with the necessary SDK requirements. Keeping dependencies updated, ensuring compatibility, and applying suitable workarounds will minimize potential disruptions during the development process.
Resolving the `aapt: error: resource android:attr/lstar not found` Issue
Dr. Emily Carter (Senior Android Developer, Tech Innovations Inc.). “The `aapt: error: resource android:attr/lstar not found` typically arises from using a theme or attribute that is not supported in the current version of your Android SDK. It is crucial to ensure that your project is targeting the correct SDK version that includes the necessary attributes.”
Michael Chen (Lead Software Engineer, Mobile Solutions Group). “This error can also occur if you are using libraries that reference attributes not available in your app’s theme. Reviewing the dependencies in your build.gradle file and ensuring compatibility with your app’s theme can help mitigate this issue.”
Sarah Johnson (Android UI/UX Specialist, App Design Studio). “To resolve the `lstar` attribute issue, developers should double-check their styles and themes. If you are using custom themes, make sure they inherit from a base theme that supports the required attributes. Additionally, updating the Android Gradle plugin may resolve compatibility issues.”
Frequently Asked Questions (FAQs)
What does the error “aapt: error: resource android:attr/lstar not found” indicate?
This error indicates that the Android Asset Packaging Tool (AAPT) cannot locate the resource `android:attr/lstar`, which typically suggests that the project is using an unsupported or outdated version of the Android SDK or libraries.
How can I resolve the “aapt: error: resource android:attr/lstar not found” error?
To resolve this error, ensure that you are using the correct version of the Android SDK and libraries. Update your build.gradle file to target a version of the Android SDK that includes the `lstar` attribute, typically found in Android 12 (API level 31) and above.
What should I check in my build.gradle file to fix this error?
Check the `compileSdkVersion` and `targetSdkVersion` in your build.gradle file. Ensure both are set to at least 31 or higher to access the `lstar` attribute. Additionally, verify that all dependencies are compatible with the specified SDK version.
Is the “aapt: error: resource android:attr/lstar not found” error related to any specific libraries?
Yes, this error can be related to libraries that utilize the `lstar` attribute, such as Material Components. Ensure that you are using the latest version of these libraries that support the required SDK version.
What steps can I take if updating the SDK does not resolve the error?
If updating the SDK does not resolve the error, try cleaning and rebuilding the project. In Android Studio, you can do this by selecting “Build” > “Clean Project” followed by “Build” > “Rebuild Project.” This can help clear any cached resources that may be causing the issue.
Are there any alternative solutions if I cannot update my SDK version?
If you cannot update your SDK version, consider removing or replacing any features or libraries that require the `lstar` attribute. This may involve modifying your layout files or switching to alternative libraries that do not depend on newer attributes.
The error message “aapt: error: resource android:attr/lstar not found” typically indicates that the Android Asset Packaging Tool (AAPT) is unable to locate a specific resource attribute within the Android framework. This issue often arises when developers attempt to use attributes or features that are not available in the version of the Android SDK they are targeting. Specifically, the ‘lstar’ attribute is associated with newer design elements introduced in recent Android versions, which may not be present in older SDKs.
To resolve this error, developers should first ensure that they are using the appropriate version of the Android SDK that supports the required attributes. Updating the compile SDK version in the build.gradle file to a more recent version can often rectify the issue. Additionally, it is essential to verify that the project dependencies are compatible with the target SDK version to avoid conflicts that may lead to similar errors.
Another important consideration is to check the XML resource files for any references to the ‘lstar’ attribute. If the project is intended to support older devices, developers might need to implement alternative solutions or use conditional resource qualifiers to provide backward compatibility. This practice ensures that the application can gracefully handle missing attributes without causing build failures.
In summary, the
Author Profile
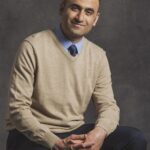
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?