Why Am I Getting Blocked by CORS Policy When Trying to Access XMLHttpRequest?
In today’s interconnected digital landscape, web applications often rely on seamless communication between servers and clients. However, developers frequently encounter a perplexing hurdle known as the CORS (Cross-Origin Resource Sharing) policy, which can block access to resources like XMLHttpRequest. This security feature, designed to protect users from malicious attacks, can leave even the most seasoned programmers scratching their heads when they see the dreaded error message. Understanding the intricacies of CORS is essential not only for troubleshooting but also for building robust web applications that adhere to modern security standards.
As web technologies evolve, so do the challenges associated with cross-origin requests. When a web application attempts to fetch resources from a different domain, the browser enforces CORS policies to mitigate potential risks. This mechanism ensures that only trusted sources can interact with sensitive data, but it can also lead to frustrating roadblocks for developers who are trying to integrate APIs or access external resources. The implications of these restrictions are significant, as they can impact everything from user experience to application performance.
In this article, we will delve into the fundamental concepts of CORS and the XMLHttpRequest mechanism, exploring how they work together to maintain security while allowing for necessary data exchanges. We’ll also discuss common pitfalls and best practices for navigating CORS-related issues, empowering
CORS Policy Overview
Cross-Origin Resource Sharing (CORS) is a security feature implemented by web browsers to prevent malicious websites from making requests to a different domain than the one that served the web page. It allows servers to specify who can access their resources and how. When a web application makes a request to a different origin, the browser checks the CORS policy set by the server. If the policy does not allow the request, the browser blocks it, leading to the common error: “Access to XMLHttpRequest at [URL] from origin [origin] has been blocked by CORS policy.”
Key points regarding CORS include:
- Same-Origin Policy: This is the foundation of web security, ensuring that scripts can only access data from the same origin.
- Preflight Requests: For certain types of requests (like those that modify data), browsers send a “preflight” request using the OPTIONS method to check if the actual request is safe to send.
- CORS Headers: The server must include specific headers in its response to allow cross-origin requests, such as `Access-Control-Allow-Origin`.
Common CORS Headers
Several headers are crucial for CORS functionality. Below is a table outlining these headers and their purposes:
Header | Description |
---|---|
Access-Control-Allow-Origin | Specifies which origins are allowed to access the resource. A wildcard (*) can be used to allow all. |
Access-Control-Allow-Methods | Indicates the HTTP methods that are permitted when accessing the resource (e.g., GET, POST, PUT). |
Access-Control-Allow-Headers | Lists the HTTP headers that can be used when making the actual request. |
Access-Control-Allow-Credentials | Indicates whether the browser should include credentials (like cookies) in the request. |
Access-Control-Max-Age | Specifies how long the results of a preflight request can be cached. |
Handling CORS Issues
When a CORS issue arises, it can be frustrating for developers. Here are common strategies to address CORS errors:
- Server Configuration: Ensure that the server is correctly configured to send the appropriate CORS headers. This may involve modifying server settings or using middleware in web frameworks.
- Using a Proxy: In development, using a proxy server can help bypass CORS restrictions. The client makes requests to the proxy, which then forwards them to the target server.
- JSONP: For GET requests, JSONP (JSON with Padding) can be used as a workaround, although it is less common due to security concerns and limitations.
- Browser Extensions: Some developers use browser extensions to disable CORS for testing purposes. However, this should never be used in production environments.
Debugging CORS Errors
To effectively debug CORS issues, developers can follow these steps:
- Check the Network Tab: Use the browser’s developer tools to inspect the network requests and see the CORS headers being sent and received.
- Review Server Logs: Check server logs for any errors or indications that requests are being denied.
- Test with cURL: Use cURL commands to simulate requests and observe the headers returned by the server.
By understanding CORS and its configuration, developers can effectively manage cross-origin requests and avoid CORS-related errors.
Understanding CORS Policy
Cross-Origin Resource Sharing (CORS) is a security feature implemented in web browsers to prevent malicious websites from making requests to a different domain than the one that served the web page. When a web application tries to access resources on a different origin, the browser checks the CORS policy of the target server.
Key points regarding CORS policy:
- Same-Origin Policy: This policy restricts web pages from making requests to a different domain, protocol, or port.
- Preflight Requests: For certain types of requests (like those that modify data), browsers send an OPTIONS request before the actual request to determine if the server permits the cross-origin request.
- HTTP Headers: Servers must include specific headers to allow cross-origin requests, such as:
- `Access-Control-Allow-Origin`: Specifies which origins are permitted to access the resource.
- `Access-Control-Allow-Methods`: Lists the HTTP methods the server supports for cross-origin requests.
- `Access-Control-Allow-Headers`: Defines which headers can be used in the actual request.
Common Causes of CORS Policy Errors
When a web application encounters a CORS policy error, it typically stems from the following issues:
- Missing CORS Headers: The server does not include the required CORS headers in its response.
- Incorrect Origin: The `Access-Control-Allow-Origin` header does not match the requesting origin.
- Preflight Request Failure: The server does not handle the OPTIONS preflight request correctly.
- Credentials Issue: If credentials (like cookies or HTTP authentication) are included, the server must explicitly allow them by setting `Access-Control-Allow-Credentials` to true.
How to Resolve CORS Policy Issues
To resolve CORS policy errors, consider the following strategies:
- Modify Server Configuration: Ensure the server is configured to send the correct CORS headers.
- Use a Proxy: Implement a server-side proxy to handle requests and relay them from the client to the desired endpoint.
- Browser Extensions: For development purposes, use browser extensions to disable CORS enforcement temporarily.
Example of appropriate CORS headers in a server response:
“`http
HTTP/1.1 200 OK
Access-Control-Allow-Origin: https://example.com
Access-Control-Allow-Methods: GET, POST, OPTIONS
Access-Control-Allow-Headers: Content-Type
“`
Testing CORS Configuration
To verify that CORS is configured correctly, you can use several tools:
Tool | Description |
---|---|
Browser Console | Use the Developer Tools console to check for CORS errors. |
Postman | Simulate requests with custom headers to test CORS. |
Curl | Command-line tool to make requests with specific headers. |
Example Curl command to test CORS headers:
“`bash
curl -i -X OPTIONS https://api.example.com/resource -H “Origin: https://yourwebsite.com”
“`
By understanding CORS policies and their configurations, developers can effectively troubleshoot and resolve issues related to XMLHttpRequests being blocked.
Understanding CORS Policy and XMLHttpRequest Access Issues
Dr. Emily Chen (Web Security Researcher, CyberSafe Institute). “The CORS (Cross-Origin Resource Sharing) policy is a critical security feature in web browsers that prevents malicious websites from accessing resources from another domain without permission. When you encounter an error stating that access to XMLHttpRequest is blocked by CORS policy, it typically indicates that the server hosting the resource has not been configured to allow requests from your domain.”
Mark Thompson (Lead Software Engineer, Tech Innovations Corp). “To resolve CORS issues, developers can implement specific headers on the server, such as ‘Access-Control-Allow-Origin’, which explicitly defines which origins are permitted to access the resource. It’s essential to ensure that these headers are correctly set up to facilitate cross-origin requests without compromising security.”
Lisa Patel (Frontend Development Specialist, WebDev Solutions). “When debugging CORS-related errors, using tools like browser developer consoles can be invaluable. They provide insights into the request and response headers, allowing developers to identify whether the appropriate CORS headers are present and if the request is being blocked due to policy restrictions.”
Frequently Asked Questions (FAQs)
What does it mean when access to XMLHttpRequest is blocked by CORS policy?
Access to XMLHttpRequest being blocked by CORS policy indicates that the web application is attempting to make a request to a different origin (domain, protocol, or port) than its own, and the server has not permitted this action through proper CORS (Cross-Origin Resource Sharing) headers.
What is CORS and why is it important?
CORS is a security feature implemented by web browsers that restricts web pages from making requests to a different domain than the one that served the web page. It is important because it helps protect users from malicious websites attempting to access sensitive data from other domains.
How can I resolve CORS issues in my web application?
To resolve CORS issues, you can modify the server-side configuration to include appropriate CORS headers, such as `Access-Control-Allow-Origin`, which specifies which origins are allowed to access the resources. Alternatively, you can use a proxy server to handle requests.
Are there any tools available to test CORS configurations?
Yes, there are several tools available to test CORS configurations, including browser developer tools, Postman, and online CORS testing services. These tools can help verify if the correct headers are being sent by the server.
Can CORS issues occur in local development environments?
Yes, CORS issues can occur in local development environments, especially when using localhost for the frontend and a different domain or port for the backend. Developers can use tools like browser extensions or modify their server settings to bypass CORS during development.
What are the security implications of misconfigured CORS settings?
Misconfigured CORS settings can expose sensitive data to unauthorized domains, leading to potential security vulnerabilities such as data theft or cross-site request forgery (CSRF). It is crucial to carefully configure CORS to allow only trusted origins.
The issue of “access to XMLHttpRequest at blocked by CORS policy” primarily revolves around the Cross-Origin Resource Sharing (CORS) mechanism, which is a security feature implemented by web browsers. CORS restricts web pages from making requests to a different domain than the one that served the web page. This is designed to prevent malicious sites from reading sensitive data from another site without permission. When a web application attempts to make a cross-origin request, the browser checks for the appropriate CORS headers in the response from the server. If these headers are missing or do not allow the requesting origin, the browser blocks the request, resulting in the CORS policy error message. Understanding this mechanism is crucial for developers working with APIs and web services.
To resolve CORS-related issues, developers can take several approaches. They can configure the server to include the necessary CORS headers, such as ‘Access-Control-Allow-Origin’, which specifies which domains are permitted to access resources. Additionally, using proxy servers or modifying the request method can help bypass CORS restrictions during development. It is also important to note that while workarounds exist, they should be implemented with caution to avoid compromising security. Properly managing CORS settings is essential for ensuring both functionality and safety in web
Author Profile
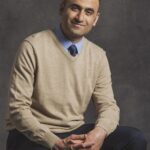
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?