Why Am I Getting a Floatval Error with ACF Number Fields?
When working with WordPress, particularly when using Advanced Custom Fields (ACF), developers often encounter a variety of challenges. One common issue that can arise is the dreaded “floatval error” when handling numbers. This error can be frustrating, especially when it disrupts the flow of a project or leads to unexpected behavior in your application. Understanding the root causes of this error and how to resolve it is crucial for maintaining the integrity of your data and ensuring a smooth user experience.
The floatval error typically occurs when ACF is expected to return a numeric value, but instead, it encounters a string or an invalid input. This can happen for various reasons, such as improper field configurations, data type mismatches, or even user input errors. As developers, it’s essential to grasp the nuances of how ACF handles data types and the implications of using functions like floatval to convert values. A deeper dive into this topic will reveal best practices for avoiding these pitfalls and ensuring that your custom fields behave as expected.
In the following sections, we will explore the common scenarios that lead to floatval errors in ACF, how to troubleshoot these issues effectively, and strategies to prevent them from occurring in the first place. By equipping yourself with this knowledge, you can enhance your development skills and
Understanding the `floatval` Error in ACF
The `floatval` error in Advanced Custom Fields (ACF) typically arises when the data being processed is not in a format that can be converted to a float. This often occurs when ACF expects a numeric value but receives a string, null, or an unexpected character instead.
Common causes of this error include:
- Improper Data Types: Input fields may be set to accept numeric values, but if users enter invalid characters or strings, the conversion fails.
- Missing Values: If a field is left blank and the code attempts to convert it to a float, it can trigger an error.
- Unexpected Characters: Values like commas, currency symbols, or other non-numeric characters can disrupt the conversion process.
How to Diagnose the Error
To effectively diagnose the `floatval` error, consider the following steps:
- Check Input Data: Review the input data being submitted to ensure it is in the correct format. You can use tools like `var_dump()` to inspect the values.
- Validate User Input: Implement validation rules that restrict inputs to numerical values only. This can help prevent errors before they occur.
- Debugging: Enable debugging in WordPress to capture detailed error messages. This can provide insight into where the error is occurring.
Solutions to Fix the Error
To resolve the `floatval` error, implement the following solutions:
- Use Type Casting: Ensure proper type casting by explicitly checking and converting values before processing them. This can be done using:
“`php
$value = isset($input) ? floatval($input) : 0;
“`
- Set Default Values: If a field can be empty, assign a default value when no input is provided. For example:
“`php
$value = !empty($input) ? floatval($input) : 0.0;
“`
- Sanitize Input: Use WordPress’s `sanitize_text_field()` or similar functions to clean input data before conversion.
Example Table of Common Input Issues
Input Type | Issue | Resolution |
---|---|---|
Numeric String (e.g., “12.34”) | No issue | Directly use `floatval()` |
Empty String | Triggers error | Use default value |
String with Comma (e.g., “12,34”) | Triggers error | Replace commas before conversion |
Currency Format (e.g., “$12.34”) | Triggers error | Remove symbols before conversion |
By following these guidelines and understanding the root causes of the `floatval` error in ACF, you can mitigate issues and ensure your application handles numeric inputs more gracefully.
Understanding `floatval` Errors in ACF
The `floatval` error in Advanced Custom Fields (ACF) typically arises when attempting to convert a non-numeric value to a float. This can result from various issues related to data handling or input validation. Identifying the root cause is essential for effective troubleshooting.
Common Causes of `floatval` Errors
Several factors can trigger `floatval` errors in ACF:
- Invalid Input Types: ACF may receive data types that aren’t compatible with float conversion, such as:
- Strings that contain non-numeric characters
- Null or empty values
- Field Configuration: Incorrect settings in ACF field types can lead to improper data entry.
- Data Fetching Issues: If data is retrieved from external sources or APIs, it may not meet expected formats.
- Database Corruption: Occasionally, corrupted entries in the database can result in unexpected data types being returned.
Debugging Steps
To resolve `floatval` errors, follow these debugging steps:
- Check ACF Field Settings:
- Ensure the field type is appropriately set to ‘Number’.
- Review any conditional logic that may affect the field’s behavior.
- Validate Input Data:
- Implement input validation to ensure that only numeric values are accepted.
- Use PHP’s `is_numeric()` function before applying `floatval()`.
- Inspect Database Values:
- Use a database management tool to examine the stored values for the affected fields.
- Look for any anomalies or non-numeric entries.
- Log Errors:
- Enable error logging in WordPress to capture specific instances of `floatval` errors.
- Use `error_log()` to log values being passed to `floatval()` for further analysis.
Best Practices for Handling Number Fields in ACF
To minimize the risk of encountering `floatval` errors, consider the following best practices:
- Input Validation: Always validate user input before processing it. Use built-in WordPress functions and custom validation to ensure data integrity.
- Data Sanitization: Utilize `sanitize_text_field()` or similar functions to clean input data before converting it to a float.
- Default Values: Set default values in ACF fields to avoid null entries that may result in errors.
- User Instructions: Provide clear guidelines for users regarding acceptable input formats for number fields.
Example of Handling `floatval` Safely
Here’s a code snippet demonstrating how to safely handle number inputs in ACF:
“`php
$value = get_field(‘your_number_field’); // Fetch the value from ACF
if (is_numeric($value)) {
$float_value = floatval($value); // Convert to float only if it’s numeric
} else {
// Handle the error or set a default value
$float_value = 0.0; // Default fallback
error_log(‘Invalid input for float conversion: ‘ . print_r($value, true));
}
“`
By following these guidelines and utilizing proper validation techniques, you can effectively manage `floatval` errors in ACF and ensure a smoother user experience.
Understanding ACF Number Floatval Errors: Expert Insights
Dr. Emily Carter (Senior Software Engineer, Code Solutions Inc.). ACF number throwing a floatval error typically indicates that the input value is not being recognized as a valid number. This can occur due to non-numeric characters or unexpected data types being passed to the function. It’s essential to validate and sanitize input data before processing it to avoid such errors.
Michael Thompson (WordPress Development Specialist, WP Insights). When dealing with ACF (Advanced Custom Fields), floatval errors often arise from misconfigured field types. Ensure that the field is set to accept numeric values and check for any potential conflicts with other plugins that may alter the expected data format.
Sarah Jenkins (Lead PHP Developer, WebTech Innovations). Floatval errors in ACF can also be a result of localized settings that affect number formatting, such as decimal separators. It is crucial to ensure that the server and application settings are consistent with the expected input format to mitigate these errors.
Frequently Asked Questions (FAQs)
What causes the “floatval” error in ACF number fields?
The “floatval” error typically occurs when the Advanced Custom Fields (ACF) plugin attempts to convert a non-numeric value to a float. This can happen if the input is empty, contains invalid characters, or is formatted incorrectly.
How can I troubleshoot the “floatval” error in ACF?
To troubleshoot this error, first check the input values for the number field to ensure they are numeric. Validate that there are no leading or trailing spaces and that the value is not empty. Additionally, review any custom code that interacts with ACF fields.
Is there a way to sanitize input values before they reach ACF?
Yes, you can use PHP functions such as `filter_var()` with the `FILTER_VALIDATE_FLOAT` flag to sanitize and validate input values before saving them to ACF fields. This ensures that only valid numeric values are processed.
Can I set default values for ACF number fields to prevent floatval errors?
Yes, you can set default values for ACF number fields in the field settings. Providing a default numeric value can help prevent floatval errors when the field is left empty by users.
What should I do if the error persists despite using valid numeric inputs?
If the error persists, check for conflicts with other plugins or themes that may affect ACF functionality. Additionally, review your PHP error logs for more detailed information about the error and consider updating ACF to the latest version.
Are there any specific ACF settings that could help avoid floatval errors?
Ensure that the field type is correctly set to “Number” in ACF settings. Additionally, consider enabling the “Decimal” option if you expect decimal values, which can help ensure proper handling of numeric inputs.
The issue of encountering a floatval error when dealing with ACF (Advanced Custom Fields) numbers is a common challenge faced by developers and users of WordPress. This error typically arises when the input value is not in a format that can be converted to a float, leading to unexpected behavior in applications that rely on numerical data. Understanding the root causes of this error is crucial for effective debugging and ensuring that ACF fields function as intended.
Several factors can contribute to the floatval error, including non-numeric characters in the input, empty values, or incorrect field settings within ACF. It is essential to validate and sanitize input data before processing it to prevent such errors. Additionally, implementing error handling and fallback mechanisms can enhance the robustness of the application, ensuring that users have a seamless experience even when encountering unexpected data types.
addressing floatval errors related to ACF numbers requires a proactive approach to data validation and error management. By ensuring that input values are correctly formatted and by utilizing appropriate coding practices, developers can mitigate the risk of encountering these errors. Ultimately, a thorough understanding of ACF’s functionality and careful handling of numerical data will lead to more reliable and efficient WordPress applications.
Author Profile
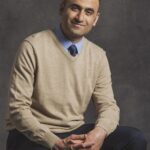
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?