How Can I Add a String to LocalStorage Using PHP?
In the modern web development landscape, the ability to store and retrieve data efficiently is paramount. As applications become increasingly dynamic and user-centric, developers often seek ways to enhance user experience by retaining information even after a page refresh or a user’s return visit. One powerful tool at your disposal is the browser’s local storage, which allows you to store data locally within the user’s browser. But how does this tie into PHP, a server-side scripting language? In this article, we will explore the seamless integration of PHP with local storage, specifically focusing on how to add a string to local storage using PHP.
Understanding the interaction between PHP and local storage is essential for creating robust web applications. While PHP handles server-side logic and data management, local storage provides a way to maintain state on the client side. This duality allows developers to create applications that not only respond to user actions in real-time but also retain crucial data across sessions. In this exploration, we will delve into the methodologies for transferring data from PHP to local storage, ensuring that your web applications can provide a personalized experience tailored to each user.
As we navigate through this topic, we will highlight key concepts and techniques that empower developers to bridge the gap between server-side processing and client-side storage. By the end of this article, you’ll
Understanding Local Storage in PHP
Local storage is a feature primarily associated with web browsers, allowing web applications to store data persistently in the user’s browser. While PHP itself does not directly handle local storage, it can be used in conjunction with JavaScript to manage data stored on the client side.
To add a string to local storage, you typically need to use JavaScript. However, PHP can be employed to generate the necessary JavaScript code dynamically. This integration allows you to pass data from your PHP backend to the client-side application, which can then store it in local storage.
Using JavaScript to Add a String to Local Storage
Once PHP generates the appropriate JavaScript, you can utilize the `localStorage` object to add a string. The basic syntax for adding an item is:
“`javascript
localStorage.setItem(‘key’, ‘value’);
“`
Here, `’key’` is the identifier for the stored item, and `’value’` is the string you wish to store.
Example: Adding Data to Local Storage
Consider the following example where PHP outputs a JavaScript snippet that adds a string to local storage:
“`php
“`
In this example, the PHP variable `$data` contains the string “Hello, World!”. When the page loads, the JavaScript will execute, adding this string to local storage under the key `’greeting’`.
Retrieving Data from Local Storage
To retrieve the stored string, you can use the following JavaScript code:
“`javascript
let greeting = localStorage.getItem(‘greeting’);
console.log(greeting); // Outputs: Hello, World!
“`
This snippet fetches the value associated with the key `’greeting’` and logs it to the console.
Data Management with Local Storage
Local storage can hold various types of data, but it is important to note that all values are stored as strings. Below is a table summarizing some key functions of the `localStorage` API:
Function | Description |
---|---|
setItem(key, value) | Stores a value with the associated key. |
getItem(key) | Retrieves the value associated with the key. |
removeItem(key) | Removes the item associated with the key. |
clear() | Clears all items in local storage. |
length | Returns the number of items in local storage. |
Security Considerations
When using local storage, it is crucial to consider the security implications:
- Data Sensitivity: Never store sensitive information (e.g., passwords, personal data) in local storage, as it can be accessed by any script running on the same origin.
- Cross-Site Scripting (XSS): Be cautious of XSS attacks that could compromise local storage data. Always sanitize inputs that may be stored.
By understanding these aspects, you can effectively utilize local storage in your web applications while maintaining robust security practices.
Understanding Local Storage in PHP
Local storage is a feature predominantly used in web browsers, allowing websites to store data persistently on the user’s device. However, PHP, being a server-side language, does not interact directly with local storage. Instead, you must leverage JavaScript on the client side to manage local storage.
Storing a String in Local Storage via PHP
To add a string to local storage in a web application that uses PHP, you will need to generate JavaScript code within your PHP script. The process involves the following steps:
- Generate the String in PHP: Create the string you want to store.
- Output JavaScript: Use PHP to echo a script tag that sets the local storage item.
Implementation Steps
Here’s a step-by-step approach to implement this:
- Step 1: Create the PHP variable to store your string.
“`php
“`
- Step 2: Use PHP to write the JavaScript code that adds this string to local storage.
“`php
“`
Key Points to Note
- json_encode: This function ensures that the string is properly formatted for JavaScript, preventing issues with quotes and special characters.
- localStorage.setItem: This method is used to set a key-value pair in local storage. The first argument is the key, and the second argument is the value you wish to store.
Reading from Local Storage
To retrieve the stored string from local storage, you can use the following JavaScript code:
“`javascript
var retrievedString = localStorage.getItem(‘myStringKey’);
console.log(retrievedString); // Outputs: Hello, World!
“`
Removing an Item from Local Storage
If you need to remove the string from local storage, you can do so with:
“`javascript
localStorage.removeItem(‘myStringKey’);
“`
Practical Example
Here’s a complete example that combines the above elements:
“`php
“`
This example demonstrates the complete cycle of adding, retrieving, and optionally removing a string in local storage using PHP and JavaScript.
Expert Insights on Storing Strings in Local Storage with PHP
Dr. Emily Carter (Web Development Specialist, Tech Innovations Journal). “While PHP is primarily a server-side language, it is essential to understand that local storage is a client-side feature. To effectively add a string to local storage, you would typically use JavaScript after the PHP has rendered the necessary data.”
Michael Chen (Full Stack Developer, CodeCraft Magazine). “Integrating PHP with local storage requires a clear understanding of the client-server model. One effective approach is to use PHP to generate JavaScript code that executes on the client side, allowing you to store strings in local storage seamlessly.”
Sarah Thompson (Senior Software Engineer, Digital Solutions Inc.). “To add a string to local storage in a PHP application, you should ensure that your PHP script outputs the necessary JavaScript. This can be achieved by echoing a script tag that utilizes the localStorage API, thus bridging the gap between server-side and client-side operations.”
Frequently Asked Questions (FAQs)
Can I directly add a string to local storage using PHP?
No, PHP runs on the server side, while local storage is a client-side feature. You cannot directly manipulate local storage with PHP; you must use JavaScript to do so.
How can I pass a string from PHP to local storage?
You can echo a JavaScript snippet from PHP that assigns a value to local storage. For example, `echo ““;` will store the value in local storage.
Is local storage accessible across different pages?
Yes, local storage is accessible across different pages within the same domain. This allows you to store data that can be retrieved on any page of your website.
What data types can be stored in local storage?
Local storage only supports string data types. If you need to store objects or arrays, you must serialize them into a JSON string using `JSON.stringify()` before storage and parse them back with `JSON.parse()` when retrieving.
Is there a size limit for local storage?
Yes, local storage typically has a size limit of around 5-10 MB per origin, depending on the browser. It is advisable to check the specific limits for the browser you are targeting.
Can local storage data be cleared programmatically?
Yes, you can clear local storage data programmatically using JavaScript. Use `localStorage.removeItem(‘key’)` to remove a specific item or `localStorage.clear()` to remove all items from local storage.
In summary, adding a string to local storage in PHP involves understanding the interplay between server-side and client-side technologies. PHP operates on the server, while local storage is a feature of web browsers that allows for the storage of data on the client side. To effectively utilize local storage, developers typically employ JavaScript to interact with the local storage API, as PHP does not have direct access to the client-side environment.
Key takeaways from this discussion include the necessity of using JavaScript to manipulate local storage. Developers can send data from PHP to JavaScript through JSON encoding or by embedding data within script tags. Once the data is available in JavaScript, it can be easily stored in local storage using methods such as `localStorage.setItem()`. This approach allows for a seamless user experience, enabling the retention of data across page reloads and sessions.
Furthermore, it is essential to consider the limitations of local storage, such as its size constraints and the fact that it is domain-specific. Understanding these factors will help developers make informed decisions about data storage strategies in their applications. Overall, integrating PHP with JavaScript for local storage management is a powerful technique that enhances the functionality and user experience of web applications.
Author Profile
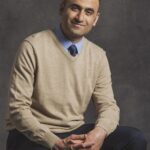
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?