How Can You Add a Class When Scrolling to an Element?
Have you ever scrolled through a webpage and noticed how certain elements seem to come alive as you reach them? This captivating effect isn’t just a happy coincidence; it’s a powerful technique used by web developers to enhance user experience and engagement. By adding classes when you scroll to specific elements, you can create dynamic interactions that not only draw attention but also guide users through your content seamlessly. In this article, we’ll explore the art of implementing scroll-triggered class additions, unlocking the potential to transform static pages into interactive experiences that keep visitors captivated.
As users navigate through your site, the way elements respond to scrolling can significantly impact their journey. By strategically adding classes when users scroll to particular sections, you can trigger animations, change styles, or reveal hidden content, making the browsing experience more immersive. This technique not only enhances visual appeal but also serves practical purposes, such as highlighting important information or guiding users toward call-to-action buttons.
Moreover, the implementation of this feature is more accessible than ever, thanks to modern JavaScript libraries and frameworks. Whether you’re a seasoned developer or just starting, understanding how to add classes based on scroll position can elevate your web design skills. In the following sections, we will delve into the methods and best practices for achieving this effect, ensuring
Add Class When Scroll to Element
When implementing a feature that adds a class to an element upon scrolling, it is essential to leverage JavaScript efficiently. This technique enhances user experience by highlighting elements as they come into view. Below are the steps and considerations for executing this functionality.
JavaScript Implementation
To add a class when an element is scrolled into view, you can use the Intersection Observer API or the traditional scroll event listener. Below is a basic implementation using the scroll event.
“`javascript
window.addEventListener(‘scroll’, function() {
const element = document.querySelector(‘.target-element’);
const position = element.getBoundingClientRect();
if (position.top < window.innerHeight && position.bottom >= 0) {
element.classList.add(‘active’);
} else {
element.classList.remove(‘active’);
}
});
“`
This code snippet listens for the scroll event and checks the position of the target element relative to the viewport. If the element is visible, it adds the ‘active’ class; otherwise, it removes it.
Performance Considerations
Using the scroll event can lead to performance issues, especially if the page contains multiple elements or if the scroll event fires rapidly. To mitigate this, consider the following strategies:
- Throttling: Limit the rate at which the scroll event handler runs.
- Debouncing: Execute the scroll handler after a specified delay from the last event.
- Using Intersection Observer: This API provides a more efficient way to observe changes in the intersection of a target element with an ancestor element or the viewport.
Example Using Intersection Observer
Here’s how you can implement the same functionality using the Intersection Observer API:
“`javascript
const observer = new IntersectionObserver((entries) => {
entries.forEach(entry => {
if (entry.isIntersecting) {
entry.target.classList.add(‘active’);
} else {
entry.target.classList.remove(‘active’);
}
});
});
const targetElement = document.querySelector(‘.target-element’);
observer.observe(targetElement);
“`
This approach is more efficient, as it reduces the number of function calls during scrolling, improving performance.
Styling the Active Class
When adding a class dynamically, it is crucial to define its styles in your CSS. Here’s an example of styling the ‘active’ class:
“`css
.target-element {
transition: transform 0.3s ease, opacity 0.3s ease;
opacity: 0.5;
}
.target-element.active {
transform: scale(1.1);
opacity: 1;
}
“`
This CSS snippet creates a smooth transition effect when the class is added or removed.
Table of Key Considerations
Method | Pros | Cons |
---|---|---|
Scroll Event | Easy to implement | Can cause performance issues |
Intersection Observer | More efficient, better performance | Requires modern browser support |
By employing these methods and considerations, you can effectively manage class additions based on scroll position, enhancing the interactivity and responsiveness of your web applications.
Understanding the Scroll Event
The scroll event is triggered when the user scrolls the document or an element. This can be utilized to detect when a specific element is in view, allowing for dynamic changes to the webpage, such as adding a class to an element.
- Event Listener: You can attach a scroll event listener to the window or a specific element.
- Debouncing: To improve performance, especially with frequent scroll events, debouncing techniques can be applied.
Detecting Element Visibility
To determine if an element is in the viewport, the `getBoundingClientRect()` method can be utilized. This method returns the size of an element and its position relative to the viewport.
“`javascript
function isElementInViewport(el) {
const rect = el.getBoundingClientRect();
return (
rect.top >= 0 &&
rect.left >= 0 &&
rect.bottom <= (window.innerHeight || document.documentElement.clientHeight) &&
rect.right <= (window.innerWidth || document.documentElement.clientWidth)
);
}
```
Implementing the Class Addition
Once the visibility of the element is determined, a class can be added to it. This can be performed within the scroll event listener.
“`javascript
const targetElement = document.querySelector(‘.target-element’);
window.addEventListener(‘scroll’, function() {
if (isElementInViewport(targetElement)) {
targetElement.classList.add(‘active’);
} else {
targetElement.classList.remove(‘active’);
}
});
“`
Performance Optimization Techniques
When implementing scroll events, performance can be a concern. To mitigate potential lag, consider the following techniques:
– **Debouncing**: Limit the rate at which the scroll event handler is called.
– **Throttling**: Execute the handler at regular intervals instead of on every scroll event.
“`javascript
function debounce(func, wait) {
let timeout;
return function executedFunction(…args) {
const later = () => {
clearTimeout(timeout);
func(…args);
};
clearTimeout(timeout);
timeout = setTimeout(later, wait);
};
}
window.addEventListener(‘scroll’, debounce(function() {
if (isElementInViewport(targetElement)) {
targetElement.classList.add(‘active’);
} else {
targetElement.classList.remove(‘active’);
}
}, 100));
“`
Cross-Browser Compatibility
When working with scroll events and element visibility, ensure compatibility across different browsers. Most modern browsers support the methods used, but testing is essential.
- Feature Detection: Use libraries like Modernizr to check for support.
- Polyfills: Implement polyfills for older browsers that may not support certain methods.
Browser | Support for `getBoundingClientRect()` | Notes |
---|---|---|
Chrome | Yes | |
Firefox | Yes | |
Safari | Yes | |
Internet Explorer | Partial (IE9+) | Limited support for older versions |
Styling and Animation Considerations
When adding or removing classes based on scroll position, consider the following styling and animation tips:
- CSS Transitions: Use CSS transitions to create smooth changes when the class is added or removed.
- Animation Libraries: Consider using libraries like Animate.css for predefined animations.
“`css
.target-element {
opacity: 0;
transition: opacity 0.5s ease;
}
.target-element.active {
opacity: 1;
}
“`
Implementing scroll-based class additions can significantly enhance user experience and engagement on a website. By leveraging efficient detection and performance techniques, developers can create dynamic and responsive web applications.
Expert Insights on Adding Classes When Scrolling to an Element
Emily Chen (Front-End Development Specialist, CodeCraft). “Implementing a class addition upon scrolling to a specific element enhances user experience significantly. It allows for dynamic visual feedback, which can guide users’ attention to important content on the page.”
Michael Thompson (Web Performance Analyst, SpeedyWeb Solutions). “From a performance standpoint, adding classes based on scroll position can be optimized to ensure minimal impact on load times. Using efficient event listeners and throttling techniques can help maintain a smooth user experience.”
Sarah Patel (User Interface Designer, Creative Minds Studio). “The strategic use of class additions during scrolling not only improves interactivity but also allows for tailored animations that can make a website feel more engaging. It is essential to balance aesthetics with functionality to achieve the best results.”
Frequently Asked Questions (FAQs)
What does it mean to add a class when scrolling to an element?
Adding a class when scrolling to an element refers to the process of dynamically applying a CSS class to an HTML element as the user scrolls the page, typically to trigger visual effects or animations.
How can I implement adding a class on scroll using JavaScript?
You can implement this by using the `scroll` event listener in JavaScript. When the user scrolls, check the position of the target element and add or remove the class based on its visibility in the viewport.
What libraries can assist with adding classes on scroll events?
Libraries such as jQuery, Intersection Observer API, and ScrollMagic can simplify the process of detecting scroll events and managing classes based on element visibility.
Is it possible to add multiple classes when scrolling to an element?
Yes, you can add multiple classes by using the `classList.add()` method in JavaScript, allowing you to apply several styles or effects simultaneously when the scroll condition is met.
What are some common use cases for adding a class on scroll?
Common use cases include triggering animations, changing styles for sticky navigation bars, revealing hidden content, and creating parallax scrolling effects.
Can adding a class on scroll affect website performance?
Yes, excessive use of scroll event listeners can lead to performance issues. It is advisable to debounce the scroll events or use the Intersection Observer API for better efficiency and performance.
the process of adding a class when scrolling to an element is a widely utilized technique in web development that enhances user experience and interactivity. This method typically involves using JavaScript or jQuery to detect the user’s scroll position and determine when a specific element comes into view. By applying a class to that element, developers can trigger visual changes, animations, or other effects that engage users as they navigate through a page.
Key takeaways from this discussion include the importance of performance optimization when implementing scroll-based effects. Developers should ensure that their scripts are efficient to avoid any lag or jank during scrolling. Additionally, the use of debouncing or throttling techniques can help manage the frequency of function calls, thus improving overall responsiveness. Furthermore, it is essential to consider accessibility and usability, ensuring that such effects do not detract from the content’s readability or navigation.
Ultimately, leveraging the ability to add classes upon scrolling can significantly enhance the aesthetic appeal and functionality of a website. By thoughtfully implementing these techniques, developers can create dynamic and engaging experiences that captivate users and encourage them to explore the content further. This approach not only showcases technical skills but also demonstrates a commitment to delivering high-quality web experiences.
Author Profile
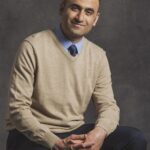
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?