How Can You Implement AG Grid Tables Across Multiple Tabs Effectively?
In the world of web development, creating dynamic and interactive user interfaces is paramount, and one tool that has gained immense popularity is AG Grid. This powerful data grid component allows developers to display and manipulate large datasets with ease. However, as applications grow in complexity, so does the need for efficient data organization. Enter the concept of implementing AG Grid tables across multiple tabs. This innovative approach not only enhances user experience but also streamlines data management, making it easier for users to navigate through different datasets without feeling overwhelmed.
Imagine a sleek application where users can effortlessly switch between tabs, each housing its own AG Grid table, tailored to specific data sets or functionalities. This setup not only improves accessibility but also allows for a more organized presentation of information. By leveraging AG Grid’s robust features, developers can create a seamless experience that caters to diverse user needs, from viewing detailed reports to performing complex data analyses.
As we delve into the intricacies of integrating AG Grid tables across multiple tabs, we will explore the benefits, best practices, and potential challenges developers may face. Whether you’re a seasoned developer or just starting, understanding how to effectively implement this feature can significantly enhance the usability and functionality of your web applications. Get ready to unlock the full potential of AG Grid as we guide
Integrating AG Grid with Multiple Tabs
To effectively manage data in a user-friendly manner, integrating AG Grid tables across multiple tabs can enhance the user experience significantly. This approach allows users to switch between different datasets seamlessly, while maintaining the benefits of AG Grid’s powerful data handling capabilities.
When implementing AG Grid within tabbed interfaces, consider the following best practices:
- Isolation of State: Each tab should maintain its own state, including sorting, filtering, and pagination. This can be achieved by assigning unique grid options for each tab.
- Dynamic Data Loading: Load data dynamically based on the active tab to optimize performance and reduce initial load times.
- Consistent UI/UX: Ensure that the grid layout and functionalities remain consistent across all tabs to avoid confusing the user.
Example Structure for Multiple Tabs with AG Grid
Here’s a simple example of how you might structure a tabbed interface with AG Grid tables.
“`html
“`
For each tab, initialize the AG Grid separately:
“`javascript
function openTab(evt, tabName) {
var i, tabcontent, tablinks;
// Hide all tab content
tabcontent = document.getElementsByClassName(“tabcontent”);
for (i = 0; i < tabcontent.length; i++) {
tabcontent[i].style.display = "none";
}
// Remove the "active" class from all tabs
tablinks = document.getElementsByClassName("tablink");
for (i = 0; i < tablinks.length; i++) {
tablinks[i].className = tablinks[i].className.replace(" active", "");
}
// Show the current tab and add an "active" class to the button that opened the tab
document.getElementById(tabName).style.display = "block";
evt.currentTarget.className += " active";
// Initialize AG Grid for the active tab
if (tabName === 'Tab1') {
new agGrid.Grid(document.getElementById('myGrid1'), gridOptions1);
} else {
new agGrid.Grid(document.getElementById('myGrid2'), gridOptions2);
}
}
```
Managing Data for AG Grid in Tabs
To effectively manage the data displayed in each AG Grid, consider using a structured approach to organize your data sources. Below is an example table showcasing how data might be categorized for different tabs.
Tab Name | Data Source | Key Features |
---|---|---|
Tab 1 | Data API 1 | Sorting, Filtering, Pagination |
Tab 2 | Data API 2 | Inline Editing, Custom Cell Renderers |
This table demonstrates the differentiation of data sources and key functionalities available in each tab. By following these guidelines, developers can create an efficient multi-tab interface that leverages the full capabilities of AG Grid, ultimately leading to a more organized and intuitive application for end-users.
Implementing AG Grid in Multiple Tabs
When working with AG Grid in applications that require multiple tabs, it is essential to manage the state and lifecycle of the grid effectively. This ensures that each tab can maintain its own grid settings, data, and user interactions without interference from others.
Grid Initialization per Tab
Each tab should initialize its own instance of the AG Grid. This can be achieved by creating separate grid configurations and mounting them when the corresponding tab is activated. Here is a basic outline for setting up AG Grid in each tab:
– **Tab Activation**: Use event listeners to trigger the grid initialization when a tab is selected.
– **Unique Grid IDs**: Assign unique IDs to each grid instance to avoid conflicts.
– **Data Handling**: Ensure each grid can load its own data independently.
Example code snippet for initializing grids:
“`javascript
function initializeGrid(tabId, gridOptions) {
const gridDiv = document.querySelector(`${tabId}`);
new agGrid.Grid(gridDiv, gridOptions);
}
// Example of tab selection
document.querySelectorAll(‘.tab’).forEach(tab => {
tab.addEventListener(‘click’, function() {
const gridOptions = {
columnDefs: […],
rowData: fetchDataForTab(tab.id),
};
initializeGrid(tab.id, gridOptions);
});
});
“`
Managing State Across Tabs
Maintaining the state of each grid is crucial for user experience. You can achieve this through various strategies:
- Local Storage: Store grid state in local storage to persist user preferences across sessions.
- React State: If using React, manage the state within components to ensure each tab can update its grid independently.
- Event Emitters: Use event emitters to communicate changes between tabs if they require shared data.
Example of storing grid state:
“`javascript
function saveGridState(tabId, gridOptions) {
const gridState = gridOptions.api.getColumnState();
localStorage.setItem(`${tabId}_gridState`, JSON.stringify(gridState));
}
// To restore state
function restoreGridState(tabId, gridOptions) {
const savedState = localStorage.getItem(`${tabId}_gridState`);
if (savedState) {
gridOptions.api.setColumnState(JSON.parse(savedState));
}
}
“`
Performance Considerations
Using AG Grid in multiple tabs can lead to performance concerns. To optimize performance, consider the following:
- Lazy Loading: Load data only when a tab is activated to reduce initial load times.
- Debouncing Input: For filters or searches, implement debouncing to limit the frequency of updates.
- Grid Refreshing: Use grid API methods judiciously to refresh data only when necessary.
Styling and Responsiveness
Ensure that each AG Grid instance is styled appropriately for a seamless user interface. Utilize CSS frameworks or custom styles to enhance the appearance of the grids across tabs:
- Consistent Design: Maintain a uniform theme for all grids to enhance usability.
- Responsive Design: Ensure grids adapt to various screen sizes, especially in mobile environments.
Example CSS for responsive grids:
“`css
.ag-theme-alpine {
width: 100%;
height: 100%;
}
“`
Example Structure of HTML with Tabs
An example structure for the HTML layout could look like this:
“`html
“`
This structure ensures that each grid is contained within its respective tab, facilitating easier management of events and state transitions.
By following these guidelines, you can effectively implement AG Grid in a multi-tab environment, ensuring a robust and user-friendly application.
Expert Insights on Implementing AG Grid Tables Across Multiple Tabs
Dr. Emily Chen (Senior Software Engineer, DataGrid Solutions). “Implementing AG Grid tables across multiple tabs requires careful management of state and data synchronization. Utilizing a centralized state management system can significantly enhance user experience by ensuring data consistency and reducing redundancy.”
Michael Thompson (Front-End Architect, UI Innovations). “When working with AG Grid in a multi-tab environment, it’s essential to optimize performance by lazy loading grid data. This approach minimizes the initial load time and improves responsiveness, particularly when dealing with large datasets.”
Sarah Patel (UX/UI Designer, Interactive Interfaces). “User experience should be at the forefront when designing AG Grid tables for multiple tabs. Implementing clear navigation and intuitive tab interactions can greatly enhance usability, ensuring that users can easily switch between data views without confusion.”
Frequently Asked Questions (FAQs)
What is AG Grid?
AG Grid is a powerful JavaScript data grid that provides advanced features for displaying and manipulating data in web applications. It supports various frameworks and allows for extensive customization.
Can AG Grid be used across multiple tabs in a web application?
Yes, AG Grid can be utilized across multiple tabs in a web application. Each tab can instantiate its own AG Grid component, allowing for independent data management and display.
How can I share data between AG Grid tables in different tabs?
To share data between AG Grid tables in different tabs, you can use a shared state management solution, such as Redux or Context API, or utilize local storage to persist data across tabs.
Is it possible to synchronize the state of AG Grid tables across multiple tabs?
Yes, it is possible to synchronize the state of AG Grid tables across multiple tabs by implementing event listeners that trigger updates in one tab when changes occur in another, using local storage or session storage.
What are the performance considerations when using AG Grid in multiple tabs?
When using AG Grid in multiple tabs, performance considerations include managing memory usage, optimizing data loading, and ensuring efficient state synchronization to prevent lag or excessive resource consumption.
Are there any limitations when using AG Grid with multiple tabs?
While AG Grid supports multiple tabs, limitations may include complexity in data synchronization, potential for increased resource usage, and the need for careful management of state to avoid conflicts or data inconsistency.
In summary, integrating AG Grid tables across multiple tabs can significantly enhance the user experience by providing a structured and organized way to present data. The flexibility of AG Grid allows developers to create dynamic and responsive tables that can be easily embedded within various tabbed interfaces. This approach not only improves data accessibility but also facilitates better data management and interaction for users navigating through different datasets.
Moreover, utilizing AG Grid in a multi-tab environment can streamline the development process. By leveraging AG Grid’s rich feature set, such as sorting, filtering, and pagination, developers can ensure that each tab remains functional and user-friendly. This integration also promotes consistency in design and functionality across different sections of an application, which is crucial for maintaining a cohesive user interface.
Key takeaways include the importance of optimizing performance when dealing with large datasets across multiple tabs. Implementing lazy loading or virtualization techniques can significantly enhance the responsiveness of the tables. Additionally, ensuring that the state of each tab is preserved during navigation can improve user satisfaction and retention. Overall, the combination of AG Grid and multi-tab layouts presents a powerful solution for modern web applications that require efficient data handling.
Author Profile
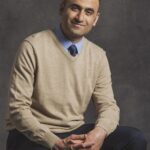
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?