How Can I Resolve the ‘An Error Occurred While Calling o123.save’ Issue?
In the world of software development and data management, errors are an inevitable part of the journey. Among the myriad of issues developers encounter, one particularly perplexing error message stands out: “an error occurred while calling o123.save?” This seemingly innocuous line can send even seasoned programmers into a spiral of confusion, prompting a quest for clarity and resolution. Understanding the nuances behind this error not only aids in troubleshooting but also enhances overall coding practices and application reliability.
This article delves into the intricacies of this error message, exploring its common causes and implications in various programming environments. From database interactions to API calls, the challenges associated with saving data can manifest in numerous ways, each requiring a unique approach to diagnosis and resolution. By examining the underlying factors that contribute to this error, developers can better equip themselves with the knowledge needed to prevent it from disrupting their workflow.
As we navigate through the complexities of this error, we will highlight practical strategies for troubleshooting and offer insights into best practices for data handling. Whether you’re a novice coder or an experienced developer, understanding the context and solutions surrounding “an error occurred while calling o123.save?” will empower you to tackle similar challenges with confidence and finesse.
Error Description
When encountering the message “an error occurred while calling o123.save,” it indicates an issue during the execution of a save operation on an object or record identified by `o123`. This error can arise from various underlying problems related to the data being saved, the state of the application, or the environment in which the operation is being executed.
Common causes of this error may include:
- Validation Failures: The data being saved does not meet the required validation rules defined within the application.
- Database Connection Issues: The application may be unable to connect to the database where the data is being stored.
- Concurrency Conflicts: If multiple processes are attempting to modify the same data simultaneously, this can lead to conflicts and errors.
- Insufficient Permissions: The user or process executing the save operation may not have the necessary permissions to perform this action.
Troubleshooting Steps
To effectively troubleshoot the error, consider following these steps:
- Review Error Logs: Check the application and database error logs for any specific messages related to the save operation.
- Validate Data: Ensure that all required fields are populated and conform to the defined validation rules.
- Check Database Connection: Verify that the database server is operational and that the application can connect to it.
- Assess Permissions: Confirm that the user or application has the appropriate permissions to perform the save operation.
- Test for Concurrency: Determine if there are other operations trying to modify the same record and address any conflicts.
Best Practices for Prevention
Implementing best practices can help prevent the occurrence of this error in the future:
- Comprehensive Input Validation: Always validate user inputs before attempting to save them.
- Error Handling Mechanisms: Implement robust error handling to gracefully manage exceptions and provide informative feedback to users.
- Transaction Management: Use transactions to ensure that save operations are atomic and can be rolled back in case of an error.
- Logging: Maintain detailed logs of application activities, especially for data modifications, to facilitate easier troubleshooting.
Error Handling Example
To manage the error effectively, it’s beneficial to include error handling in the code. Below is a simple example in pseudocode:
“`pseudocode
try {
o123.save();
} catch (Error e) {
log(e.message);
notifyUser(“An error occurred while saving the data. Please try again.”);
}
“`
This example captures the error and logs it while notifying the user, which can help in understanding the context of the failure.
Common Error Codes
The following table summarizes some common error codes associated with save operations and their meanings:
Error Code | Description |
---|---|
400 | Bad Request – Validation error occurred. |
500 | Internal Server Error – General failure in processing the request. |
403 | Forbidden – Insufficient permissions to perform the operation. |
409 | Conflict – Concurrency issue detected. |
By following these guidelines and understanding the potential causes of the error, you can streamline the troubleshooting process and enhance the stability of your application’s data handling capabilities.
Understanding the Error
The error message `an error occurred while calling o123.save?` typically indicates a failure during the execution of a save operation in a software application. This issue may arise from various underlying causes, which can be categorized into several key areas.
- Database Connection Issues:
- The application may not be able to connect to the database, leading to failed save attempts.
- Possible causes include incorrect connection strings, unresponsive database servers, or network issues.
- Data Validation Failures:
- The data being saved might not meet the required validation rules.
- Common validation failures include:
- Missing required fields.
- Data types not matching expected formats.
- Unique constraints being violated.
- Permissions and Access Control:
- Insufficient permissions for the user or application attempting the save can trigger this error.
- Access control lists (ACLs) or role-based access control (RBAC) settings may need to be reviewed.
Troubleshooting Steps
To resolve the error effectively, follow these troubleshooting steps:
- Check Database Connection:
- Verify the connection string in the application configuration.
- Ensure that the database server is running and accessible.
- Review Application Logs:
- Examine log files for detailed error messages or stack traces.
- Look for patterns or specific error codes that can guide further investigation.
- Validate Input Data:
- Ensure that all required fields are populated.
- Confirm that the data types and formats adhere to expected standards.
- Check Permissions:
- Review user roles and permissions related to the save operation.
- Modify permissions if necessary to grant access.
- Test Save Operation:
- Attempt to save a simplified version of the data to isolate the issue.
- Gradually reintroduce complexity to pinpoint the failure source.
Common Solutions
Depending on the identified cause, several solutions may be applicable:
Cause | Solution |
---|---|
Database Connection Issues | Update the connection string or settings. Restart the database service if necessary. |
Data Validation Failures | Adjust the input data to meet validation criteria. Implement proper error handling to catch validation errors before the save attempt. |
Permissions Issues | Update user roles or permissions in the database or application settings. Ensure that the user has the required access rights. |
Preventative Measures
To minimize the occurrence of this error in the future, consider implementing the following measures:
- Input Validation:
- Implement comprehensive input validation to catch errors early in the process.
- Enhanced Logging:
- Use detailed logging to capture information about the save operations, which can aid in diagnosing future errors.
- Regular Audits:
- Perform regular audits of database schemas and application permissions to ensure compliance with expected configurations.
- Monitoring Tools:
- Utilize monitoring tools to track database performance and connection health, allowing for proactive intervention before issues arise.
Understanding the Error: Insights on `an error occurred while calling o123.save?`
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error message `an error occurred while calling o123.save?` typically indicates an issue with the data being processed or a failure in the underlying service. It is crucial to check the parameters being passed to the `save` function and ensure that they meet the expected format and data types.”
Michael Chen (Lead Database Administrator, Data Solutions Group). “This error can often arise from database constraints or connection issues. It is essential to review the database logs for any related errors and ensure that the database service is running optimally. Additionally, validating the integrity of the data before calling the save function can prevent such errors.”
Sarah Johnson (Application Development Consultant, CodeWise Solutions). “In many cases, `an error occurred while calling o123.save?` can be attributed to permission issues or misconfigured API endpoints. Developers should ensure that the application has the necessary permissions to execute the save operation and that the endpoint is correctly set up to handle the request.”
Frequently Asked Questions (FAQs)
What does the error “an error occurred while calling o123.save?” mean?
This error typically indicates a failure in the execution of the save operation within the o123 system, often due to issues such as invalid data, system configuration errors, or connectivity problems.
What are common causes for the “an error occurred while calling o123.save?” error?
Common causes include incorrect data formats, missing required fields, insufficient user permissions, or server-side issues that prevent the save operation from completing successfully.
How can I troubleshoot the “an error occurred while calling o123.save?” error?
To troubleshoot, verify the data being submitted for correctness, check user permissions, review server logs for additional error details, and ensure that all required fields are populated.
Is there a way to prevent the “an error occurred while calling o123.save?” error from occurring?
Preventative measures include implementing thorough data validation before submission, ensuring user permissions are correctly set, and regularly monitoring server performance and configurations.
Where can I find more information about resolving the “an error occurred while calling o123.save?” error?
Detailed documentation and troubleshooting guides are often available in the system’s support portal or knowledge base. Additionally, consulting with technical support can provide tailored assistance.
What should I do if the error persists despite troubleshooting?
If the error continues, escalate the issue to your technical support team with detailed information about the error, steps taken during troubleshooting, and any relevant logs or screenshots for further analysis.
In addressing the issue of the error message “an error occurred while calling o123.save,” it is essential to recognize the potential causes that may lead to this error. Commonly, such errors arise from misconfigurations in the code, issues with data validation, or problems related to the database connection. Understanding the context in which this error occurs can significantly aid in troubleshooting and resolving the underlying issues.
Furthermore, it is important to consider the role of proper error handling and logging in software development. Implementing robust error handling mechanisms can provide developers with clearer insights into the nature of the error, allowing for more efficient debugging. Additionally, logging relevant details during the execution of the save operation can help identify patterns or recurring issues that may not be immediately apparent.
addressing the error “an error occurred while calling o123.save” requires a systematic approach that includes examining the code for potential issues, ensuring proper data handling, and leveraging effective error logging practices. By doing so, developers can enhance the reliability of their applications and improve the overall user experience.
Author Profile
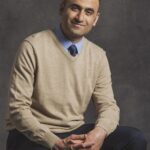
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?