Why Can’t an Insert Exec Statement Be Nested?
In the world of database management, executing commands efficiently is crucial for maintaining data integrity and performance. However, developers often encounter various challenges when working with SQL statements, particularly when it comes to executing nested commands. One such common pitfall is the error message: “an insert exec statement cannot be nested.” This seemingly straightforward issue can lead to confusion and frustration, especially for those new to SQL or those who are integrating complex procedures into their applications. Understanding the underlying reasons for this restriction is essential for anyone looking to streamline their database operations and avoid potential pitfalls.
The error message highlights a fundamental limitation in SQL Server’s handling of certain types of commands. When developers attempt to execute an `INSERT` statement that calls a stored procedure (using `EXEC`) within the same scope, they may inadvertently trigger this error. This restriction is rooted in the way SQL Server processes transactions and manages resources, which can complicate the execution of nested commands. As a result, it becomes imperative for developers to grasp the nuances of SQL execution flow and the implications of nesting commands to ensure their queries run smoothly.
Navigating this error requires a blend of understanding SQL Server’s architecture and employing best practices in query design. By recognizing the scenarios that lead to this error, developers can adopt alternative strategies to achieve
An Insert Exec Statement Cannot Be Nested
The error message “an insert exec statement cannot be nested” typically arises when attempting to execute an `INSERT` statement that includes a nested `EXEC` command. This situation often occurs when dealing with stored procedures or dynamic SQL in SQL Server. Understanding the limitations of nesting these statements is crucial for effective database management.
When you execute a stored procedure that performs an `INSERT` operation inside another stored procedure, SQL Server restricts such actions. This restriction is in place to prevent complex execution contexts that could lead to unexpected behaviors or data integrity issues.
Key Points to Consider
- Stored Procedures: A stored procedure is a precompiled collection of one or more SQL statements that can be executed as a single unit. When a stored procedure attempts to call another stored procedure that includes an `INSERT` statement, SQL Server does not allow this nesting.
- Dynamic SQL: If you use dynamic SQL to construct an `INSERT` statement, ensure that it is not nested within another `EXEC` call. SQL Server requires that the outer context be stable to avoid execution errors.
- Transaction Management: Nested transactions can complicate error handling and rollback procedures. SQL Server treats all transactions at the same nesting level, which can lead to confusion if not managed properly.
Solutions and Workarounds
To resolve the issue of nested `INSERT EXEC` statements, consider the following approaches:
- Refactor Stored Procedures: Avoid nesting by restructuring your stored procedures to eliminate the need for an `INSERT EXEC` operation. Instead, break down the logic into separate procedures that handle individual tasks.
- Use Temporary Tables: Instead of inserting directly from an `EXEC` statement, you can insert data into a temporary table first and then perform the `INSERT` operation from that table. This can help maintain clarity in your code.
- Utilize Table Variables: Table variables can be used to store intermediate results that can be subsequently manipulated without causing nesting issues.
Example Scenario
Here’s an example illustrating how to avoid the nested `INSERT EXEC` error:
“`sql
— This will cause an error due to nesting
CREATE PROCEDURE OuterProcedure AS
BEGIN
EXEC InnerProcedure; — InnerProcedure contains an INSERT EXEC
END;
— Refactored approach using a temporary table
CREATE PROCEDURE OuterProcedure AS
BEGIN
CREATE TABLE TempResults (Column1 INT, Column2 VARCHAR(100));
INSERT INTO TempResults EXEC InnerProcedure; — Safe to do
INSERT INTO FinalTable (Column1, Column2)
SELECT Column1, Column2 FROM TempResults;
DROP TABLE TempResults;
END;
“`
Summary Table of Solutions
Method | Description |
---|---|
Refactor Stored Procedures | Eliminate nested execution by restructuring stored procedure calls. |
Use Temporary Tables | Store results in a temporary table before further manipulation. |
Utilize Table Variables | Use table variables to hold intermediate data securely. |
By understanding these strategies and the context of the “insert exec statement cannot be nested” error, database administrators and developers can design more efficient and error-free SQL code.
Understanding the Error Message
The error message “an insert exec statement cannot be nested” typically occurs in SQL Server when an `INSERT…EXEC` command is executed within a context that does not allow it to be nested. This situation arises due to the restrictions SQL Server places on certain operations.
Key points to consider regarding this error include:
- Context of Execution: The error often emerges when attempting to execute a stored procedure that itself performs an `INSERT…EXEC` statement while already within another `INSERT…EXEC`.
- Transaction Management: SQL Server may restrict nested execution to prevent conflicts and ensure data integrity during transactions.
- Compatibility: The error can arise in various SQL Server versions, but understanding the specific version in use can be crucial for troubleshooting.
Common Scenarios Leading to the Error
Several scenarios can trigger this error message:
- Nested Procedures: When a stored procedure calls another stored procedure that uses `INSERT…EXEC`, it can lead to nesting issues.
- Triggers: If a trigger on a table executes an `INSERT…EXEC`, it can conflict with another `INSERT…EXEC` being executed.
- Dynamic SQL: Using dynamic SQL to call an `INSERT…EXEC` within another `INSERT…EXEC` can also result in this error.
Strategies to Resolve the Error
To resolve the “an insert exec statement cannot be nested” error, consider the following strategies:
- Refactor Code:
- Avoid nesting `INSERT…EXEC` statements.
- Separate the logic into different stored procedures or use temporary tables.
- Use Temporary Tables:
- Instead of inserting directly from an `EXEC`, insert results into a temporary table first, then perform the insert operation.
- Example SQL:
“`sql
CREATE TABLE TempResults (Column1 INT, Column2 VARCHAR(50));
INSERT INTO TempResults EXEC YourStoredProcedure;
INSERT INTO YourFinalTable SELECT * FROM TempResults;
DROP TABLE TempResults;
“`
- Check for Triggers:
- Review any triggers on the table to ensure they do not contain nested `INSERT…EXEC` operations.
- Review Transaction Logic:
- Ensure that your transaction logic does not inadvertently cause nested executions.
Best Practices to Prevent the Error
Implementing best practices can help avoid encountering the nesting error in SQL Server:
- Modular Design: Break down complex stored procedures into smaller, manageable ones that do not call `INSERT…EXEC` within each other.
- Clear Naming Conventions: Use descriptive names for stored procedures to clarify their purpose and avoid confusion regarding nesting.
- Thorough Testing: Regularly test stored procedures in isolation before integrating them into larger scripts or applications.
- Documentation: Keep detailed documentation of stored procedures and their interactions to identify potential nesting issues early.
By understanding the underlying causes and implementing effective strategies, database developers can mitigate the risks associated with the “an insert exec statement cannot be nested” error. Employing best practices in stored procedure design and transaction management will lead to more robust and maintainable SQL code.
Understanding the Limitations of Nested Insert Exec Statements
Dr. Emily Carter (Database Architect, Tech Innovations Inc.). “The restriction against nesting `INSERT EXEC` statements is primarily designed to maintain data integrity and prevent unexpected behavior during transaction processing. When a stored procedure is executed within an `INSERT` statement, it can lead to complex dependencies that are difficult to manage and debug.”
Michael Thompson (Senior SQL Developer, Data Solutions Group). “In SQL Server, the inability to nest `INSERT EXEC` statements is a known limitation that developers must account for. This design choice helps avoid issues such as deadlocks and performance degradation that can arise from executing multiple layers of commands simultaneously.”
Sarah Patel (Lead Database Consultant, Enterprise Data Strategies). “Understanding the implications of not being able to nest `INSERT EXEC` statements is crucial for database optimization. Developers should consider alternative approaches, such as using temporary tables or restructuring their queries, to achieve the desired outcomes without running into this limitation.”
Frequently Asked Questions (FAQs)
What does the error “an insert exec statement cannot be nested” mean?
This error occurs when you attempt to execute an `INSERT EXEC` statement within another `INSERT EXEC` statement. SQL Server does not allow nesting of these commands due to potential conflicts and resource management issues.
What are the common scenarios that lead to this error?
Common scenarios include executing a stored procedure that contains an `INSERT EXEC` within another stored procedure or batch that also uses `INSERT EXEC`. This nesting is not permitted in SQL Server.
How can I resolve the “an insert exec statement cannot be nested” error?
To resolve this error, you can refactor your code to avoid nesting. Consider using temporary tables or table variables to store results from the first `INSERT EXEC` before passing them to the second operation.
Can I use a transaction with an `INSERT EXEC` statement?
Yes, you can use a transaction with an `INSERT EXEC` statement. However, ensure that the transaction does not include another `INSERT EXEC` statement within it, as this will trigger the nesting error.
Are there any performance implications of using `INSERT EXEC`?
Yes, using `INSERT EXEC` can have performance implications, especially if the executed stored procedure returns a large dataset. It is advisable to analyze the execution plan and optimize the procedure for better performance.
Is there a workaround to achieve similar functionality without nesting?
Yes, you can achieve similar functionality by first executing the stored procedure and storing the results in a temporary table. Subsequently, you can perform any required operations on that temporary table without nesting `INSERT EXEC` statements.
The statement “an insert exec statement cannot be nested” refers to a limitation in SQL Server regarding the execution of stored procedures and the use of the INSERT command. This restriction arises when attempting to execute an INSERT statement that calls another stored procedure, which in turn attempts to perform an INSERT operation. SQL Server does not allow this nesting due to potential conflicts and complications that may arise during transaction management and resource allocation.
This limitation is significant for database developers and administrators as it necessitates careful planning and structuring of SQL code. Understanding this restriction helps in avoiding runtime errors and ensures smoother execution of database operations. Developers may need to consider alternative approaches, such as restructuring the logic of their stored procedures or using temporary tables to manage data before performing the final INSERT operations.
In summary, recognizing the constraints associated with nested INSERT EXEC statements is crucial for effective database management. By adhering to best practices and understanding the implications of this limitation, developers can enhance the reliability and performance of their SQL Server applications. This knowledge ultimately contributes to more robust and maintainable database solutions.
Author Profile
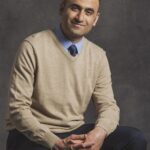
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?