Are Dictionaries Mutable in Python? Exploring Their Flexibility and Usage
In the world of Python programming, data structures play a pivotal role in how we store and manipulate information. Among these structures, dictionaries stand out due to their unique ability to associate keys with values, enabling efficient data retrieval and organization. However, a common question that arises among both novice and experienced programmers is: Are dictionaries mutable in Python? Understanding the mutability of dictionaries is crucial for effective coding, as it influences how we manage data, modify existing entries, and handle potential errors in our programs.
Dictionaries in Python are indeed mutable, meaning that their contents can be changed after they are created. This characteristic allows developers to add, remove, or modify key-value pairs without needing to create a new dictionary. The mutability of dictionaries provides flexibility and efficiency, making them a popular choice for various applications, from simple data storage to complex data management tasks.
Moreover, the mutable nature of dictionaries comes with its own set of considerations. While it offers the convenience of dynamic data handling, it also requires careful management to avoid unintended side effects, especially when dictionaries are passed around in functions or shared across different parts of a program. As we delve deeper into this topic, we will explore the implications of dictionary mutability, practical examples, and best practices to harness their full potential in your Python
Understanding Mutability in Python
In Python, mutability refers to the ability of an object to be modified after it has been created. Objects that can be changed in place are considered mutable, while those that cannot be changed are immutable. This distinction is crucial when working with data structures, as it affects how they behave and interact with other components in your programs.
Dictionaries as Mutable Objects
Dictionaries in Python are indeed mutable. This means that you can modify a dictionary after it has been created. You can add, remove, or change items without needing to create a new dictionary.
Key characteristics of mutable dictionaries include:
- Adding Items: New key-value pairs can be added using assignment.
- Updating Items: Existing key-value pairs can be modified by assigning a new value to an existing key.
- Removing Items: Key-value pairs can be removed using methods such as `del` or `.pop()`.
Examples of Mutability in Dictionaries
To illustrate the mutability of dictionaries, consider the following examples:
“`python
Creating a dictionary
my_dict = {‘a’: 1, ‘b’: 2, ‘c’: 3}
Adding a new key-value pair
my_dict[‘d’] = 4
Updating an existing value
my_dict[‘a’] = 10
Removing a key-value pair
del my_dict[‘b’]
Current state of my_dict
print(my_dict) Output: {‘a’: 10, ‘c’: 3, ‘d’: 4}
“`
The above example demonstrates how the dictionary is modified in various ways, showcasing its mutability.
Comparison of Mutable and Immutable Types
Understanding the differences between mutable and immutable types is essential for effective programming in Python. Below is a comparison table that highlights these differences:
Feature | Mutable | Immutable |
---|---|---|
Modification | Can be changed in place | Cannot be changed in place |
Examples | Dictionaries, Lists, Sets | Tuples, Strings, Frozensets |
Memory Efficiency | More efficient for large data structures | Less efficient due to creation of new instances |
Usage | Ideal for dynamic data | Ideal for fixed data |
This table underscores the important distinctions between mutable and immutable types, emphasizing the practical implications of using dictionaries in Python programming.
Mutability of Dictionaries in Python
Dictionaries in Python are indeed mutable, which means that their contents can be modified after creation. This mutability is a key feature that allows for dynamic data manipulation, making dictionaries highly versatile for various applications.
Characteristics of Mutable Objects
When an object is mutable, it allows for changes in its state or contents without requiring the creation of a new object. The following characteristics define mutable objects, including dictionaries:
- In-Place Modification: Mutable objects can be changed directly without creating a new object.
- Dynamic Resizing: They can grow or shrink in size as items are added or removed.
- Reference-Based: Multiple references can point to the same object, and changes made through one reference will reflect in others.
Operations Demonstrating Mutability
Several operations can demonstrate the mutability of dictionaries:
- Adding Items: New key-value pairs can be added using the assignment operator.
“`python
my_dict = {‘a’: 1, ‘b’: 2}
my_dict[‘c’] = 3 Adds a new key ‘c’ with value 3
“`
- Updating Existing Items: The value of an existing key can be updated.
“`python
my_dict[‘a’] = 10 Updates the value of key ‘a’ to 10
“`
- Removing Items: Items can be removed using the `del` statement or the `pop()` method.
“`python
del my_dict[‘b’] Removes the key ‘b’
my_dict.pop(‘c’) Removes the key ‘c’
“`
Examples of Dictionary Mutability
Below is a table illustrating various operations on dictionaries that highlight their mutable nature.
Operation | Example Code | Result |
---|---|---|
Initial Creation | `my_dict = {‘x’: 1, ‘y’: 2}` | `{‘x’: 1, ‘y’: 2}` |
Add Item | `my_dict[‘z’] = 3` | `{‘x’: 1, ‘y’: 2, ‘z’: 3}` |
Update Item | `my_dict[‘x’] = 5` | `{‘x’: 5, ‘y’: 2, ‘z’: 3}` |
Remove Item | `my_dict.pop(‘y’)` | `{‘x’: 5, ‘z’: 3}` |
Clear All Items | `my_dict.clear()` | `{}` |
Comparison with Immutable Types
In contrast to mutable types, immutable types such as tuples and strings cannot be modified once created. This distinction leads to different behaviors in operations:
- Tuples: Attempting to change a tuple results in a `TypeError`.
“`python
my_tuple = (1, 2, 3)
my_tuple[0] = 4 Raises TypeError
“`
- Strings: Strings cannot be altered; instead, a new string must be created.
“`python
my_string = “hello”
my_string[0] = ‘H’ Raises TypeError
new_string = “H” + my_string[1:] Creates a new string
“`
Understanding the mutability of dictionaries is essential for effective programming in Python, as it allows for efficient data handling and manipulation.
Understanding the Mutability of Dictionaries in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Dictionaries in Python are indeed mutable, which means that you can change their content without creating a new object. This mutability allows developers to efficiently manage and update key-value pairs during runtime, making dictionaries a powerful data structure for dynamic applications.”
Michael Chen (Python Developer and Author, Python Programming Weekly). “The mutability of dictionaries is one of their defining characteristics. Unlike tuples or strings, dictionaries allow for modifications such as adding, removing, or updating entries, which is essential for applications that require dynamic data handling.”
Linda Patel (Data Scientist, AI Solutions Group). “In Python, the mutable nature of dictionaries facilitates a range of operations that can enhance performance and flexibility. This feature is particularly advantageous in data manipulation tasks, where the ability to change the dictionary’s contents on-the-fly can lead to more efficient algorithms.”
Frequently Asked Questions (FAQs)
Are dictionaries mutable in Python?
Yes, dictionaries in Python are mutable, meaning their contents can be changed after creation. You can add, remove, or modify key-value pairs.
What does it mean for a data structure to be mutable?
A mutable data structure allows for modifications without creating a new object. This includes changing elements, adding new ones, or deleting existing ones.
How can I add a key-value pair to a Python dictionary?
You can add a key-value pair by using the assignment operator. For example, `my_dict[‘new_key’] = ‘new_value’` adds a new entry to the dictionary.
Can I remove a key from a dictionary in Python?
Yes, you can remove a key using the `del` statement or the `pop()` method. For example, `del my_dict[‘key’]` or `my_dict.pop(‘key’)` will remove the specified key.
Are there any restrictions on the types of keys in a Python dictionary?
Yes, dictionary keys must be immutable types, such as strings, numbers, or tuples. Mutable types like lists cannot be used as keys.
What happens if I try to access a key that does not exist in a dictionary?
If you attempt to access a non-existent key, Python raises a `KeyError`. You can avoid this by using the `get()` method, which returns `None` or a specified default value if the key is absent.
In Python, dictionaries are indeed mutable data structures. This means that once a dictionary is created, its contents can be modified without the need to create a new dictionary. Users can add, remove, or change key-value pairs at any time, which provides a high degree of flexibility when managing collections of data.
The mutability of dictionaries allows for dynamic data manipulation, making them particularly useful in various programming scenarios. For instance, developers can efficiently update configurations, store user information, or manage data that requires frequent changes. This characteristic distinguishes dictionaries from immutable data types, such as tuples or strings, which cannot be altered after their creation.
It is also important to note that while the dictionary itself is mutable, the values it holds can be of any data type, including mutable types like lists or other dictionaries. This means that the overall structure can be complex, and understanding how to manage mutability within these nested structures is crucial for effective programming in Python.
In summary, the mutability of dictionaries in Python enhances their usability and adaptability in various applications. This feature, along with their key-value pairing system, makes dictionaries an essential tool for developers when handling dynamic datasets.
Author Profile
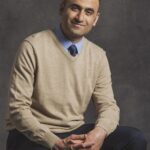
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?