Are Python Dictionaries Mutable? Unpacking the Flexibility of Key-Value Pairs!
In the world of programming, understanding data structures is crucial for efficient code development. Among the myriad of options available, Python dictionaries stand out as one of the most versatile and widely used structures. But what makes them so special? One key aspect that often piques the interest of both novice and seasoned developers alike is their mutability. Are Python dictionaries mutable? This question not only touches on the fundamental nature of dictionaries but also opens the door to a deeper exploration of how we can manipulate data in Python. Join us as we delve into the fascinating characteristics of dictionaries, their mutable nature, and the implications it has for your coding practices.
Overview
At its core, a Python dictionary is a collection of key-value pairs that allows for efficient data retrieval and organization. Unlike some other data structures, dictionaries offer the ability to update, add, or remove entries after their creation. This dynamic capability is what we refer to as mutability. Understanding this trait is essential for developers who want to leverage dictionaries effectively in their applications.
The mutability of Python dictionaries not only enhances their functionality but also influences how they interact with other data types within the language. As we explore this topic further, we will uncover the advantages and potential pitfalls of working with mutable objects, providing you with the
Understanding Mutability in Python Dictionaries
Dictionaries in Python are indeed mutable, which means that their contents can be changed after the dictionary has been created. This characteristic is fundamental to the way dictionaries operate, allowing for flexible data management. Mutability in dictionaries allows you to add, remove, or modify key-value pairs without needing to create a new dictionary.
Key Characteristics of Mutable Dictionaries
- Dynamic Size: You can change the size of a dictionary by adding or removing items.
- Key-Value Pair Modification: Existing keys can have their associated values updated.
- Item Deletion: You can remove items using methods like `del` or `pop()`.
Example of Mutability in Action
To illustrate mutability, consider the following example:
“`python
my_dict = {‘name’: ‘Alice’, ‘age’: 30}
my_dict[‘age’] = 31 Modifying an existing key’s value
my_dict[‘city’] = ‘New York’ Adding a new key-value pair
del my_dict[‘name’] Removing a key-value pair
“`
After executing these statements, the contents of `my_dict` would be:
“`python
{‘age’: 31, ‘city’: ‘New York’}
“`
This demonstrates how you can change a dictionary’s content without creating a new instance.
Comparison with Immutable Data Types
To further understand the concept of mutability, it’s useful to compare dictionaries with immutable data types, such as tuples and strings.
Data Type | Mutability | Example |
---|---|---|
Dictionary | Mutable | {‘key’: ‘value’} |
Tuple | Immutable | (1, 2, 3) |
String | Immutable | ‘hello’ |
This table highlights the differences in mutability among various data types in Python, emphasizing the flexibility of dictionaries.
Practical Implications of Mutability
The mutability of dictionaries has several practical implications in programming:
- In-Place Updates: You can modify dictionaries in place, which can lead to more efficient memory usage.
- Dynamic Data Structures: Mutable dictionaries are particularly useful in situations where the data is subject to frequent changes, such as caching results or maintaining state.
- Concurrency Considerations: In multi-threaded environments, mutable structures can lead to issues if not managed correctly, necessitating synchronization mechanisms.
In summary, understanding the mutability of dictionaries is crucial for effective programming in Python, enabling developers to leverage their dynamic nature for a variety of applications.
Understanding the Mutability of Python Dictionaries
Python dictionaries are indeed mutable, which means that they can be changed after their creation. This characteristic allows for various operations that can modify the content of a dictionary, such as adding, removing, or updating key-value pairs.
Key Characteristics of Mutable Objects
- Dynamic Size: The size of a dictionary can change as you add or remove key-value pairs.
- In-place Modification: Changes to a dictionary can be made without creating a new object.
- Non-ordered Until Python 3.7: Although dictionaries maintain insertion order from Python 3.7 onwards, their mutability allows for rearranging elements through updates.
Common Operations Demonstrating Mutability
The following operations highlight the mutable nature of dictionaries:
- Adding Items: You can add new key-value pairs to a dictionary.
“`python
my_dict = {‘key1’: ‘value1’}
my_dict[‘key2’] = ‘value2’ Adding a new key-value pair
“`
- Updating Items: Existing values can be updated using their keys.
“`python
my_dict[‘key1’] = ‘new_value1’ Updating the value of ‘key1’
“`
- Removing Items: Key-value pairs can be removed from a dictionary.
“`python
del my_dict[‘key2’] Removing the key ‘key2’ and its value
“`
- Clearing a Dictionary: All items can be removed at once.
“`python
my_dict.clear() This will empty the dictionary
“`
Implications of Mutability
The mutability of dictionaries offers both flexibility and certain risks:
Advantages | Disadvantages |
---|---|
Easy to update data | Accidental data loss possible |
Efficient memory usage | Potential for unintended changes |
Allows for dynamic data structures | Complexity in managing state |
Conclusion on Mutability
The mutable nature of Python dictionaries makes them a powerful tool for developers, enabling dynamic data handling. Understanding their properties and operations is crucial for effective programming in Python.
Understanding the Mutability of Python Dictionaries
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Corp). “Python dictionaries are indeed mutable, which means that their contents can be changed without creating a new dictionary. This property allows for efficient data manipulation, making dictionaries a powerful tool for developers working with dynamic datasets.”
Michael Chen (Data Scientist, Analytics Solutions). “The mutability of Python dictionaries is a fundamental aspect that distinguishes them from immutable data structures like tuples. This feature enables programmers to add, remove, or modify key-value pairs on the fly, which is essential for many data processing tasks.”
Sarah Patel (Python Instructor, Code Academy). “Understanding that dictionaries in Python are mutable is crucial for beginners. It impacts how they handle data and manage memory, as mutable objects can lead to unintended side effects if not properly managed during function calls or data assignments.”
Frequently Asked Questions (FAQs)
Are Python dictionaries mutable?
Yes, Python dictionaries are mutable, meaning their contents can be changed after creation. You can add, remove, or modify key-value pairs without creating a new dictionary.
What does it mean for an object to be mutable?
An object is considered mutable if its state or contents can be altered after it has been created. This contrasts with immutable objects, which cannot be changed once defined.
Can you give an example of modifying a Python dictionary?
Certainly. You can modify a dictionary by adding a new key-value pair using the syntax `dict[key] = value`, or by updating an existing key with `dict[key] = new_value`.
Are there any performance implications of using mutable objects like dictionaries?
Yes, mutable objects can lead to performance overhead in certain situations, particularly when they are copied or passed around in functions. Careful management of mutable objects is essential to avoid unintended side effects.
How do Python dictionaries compare to other mutable data types?
Python dictionaries are similar to lists and sets in terms of mutability. However, dictionaries are key-value pairs, while lists are ordered collections of items, and sets are unordered collections of unique items.
Can you use mutable objects as dictionary keys?
No, mutable objects cannot be used as dictionary keys because they can change state, which would affect their hash value. Only immutable objects, such as strings, numbers, and tuples, can serve as dictionary keys.
In summary, Python dictionaries are indeed mutable data structures. This means that once a dictionary is created, its contents can be modified. Users can add new key-value pairs, update existing ones, or remove pairs altogether without needing to create a new dictionary. This mutability is a fundamental characteristic that allows for dynamic data management and manipulation within Python programming.
One of the key advantages of mutable dictionaries is their flexibility. Developers can efficiently manage collections of data that require frequent updates, making dictionaries an ideal choice for scenarios where the data set is subject to change. This contrasts with immutable data structures, such as tuples, which do not allow for modification after creation.
Furthermore, understanding the mutability of dictionaries is crucial for effective memory management and performance optimization in Python. Since dictionaries can grow and shrink in size as needed, they provide a robust solution for applications that require dynamic data handling. However, it is essential to be cautious when using mutable objects as keys in dictionaries, as this can lead to unpredictable behavior if the object is altered after being used as a key.
Author Profile
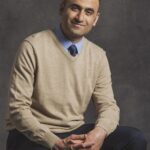
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?