Are Python Lists Mutable? Unraveling the Mystery of List Behavior!
In the world of programming, understanding data structures is crucial, and one of the most versatile and widely used structures in Python is the list. As a beginner or even an experienced coder, you might have come across the term “mutable” and wondered how it applies to Python lists. This fundamental characteristic not only influences how you manipulate data but also shapes the way you design your programs. In this article, we will explore the mutability of Python lists, unraveling the implications of this feature and how it can be leveraged to enhance your coding efficiency.
Python lists are collections that can hold an ordered sequence of items, ranging from numbers and strings to other lists. One of their standout features is their mutability, which means that once a list is created, its contents can be changed without needing to create a new list. This flexibility allows for dynamic data manipulation, making lists an essential tool in a programmer’s toolkit. However, with great power comes great responsibility; understanding how and when to modify lists is key to writing clean, efficient code.
As we delve deeper into the topic, we will examine the practical applications of mutable lists, the differences between mutable and immutable types, and the potential pitfalls that come with their use. Whether you’re looking to optimize your code or simply
Understanding Mutability in Python Lists
Python lists are mutable, which means that their contents can be changed after the list has been created. This characteristic allows for greater flexibility when managing collections of items. You can add, remove, or modify elements within a list without needing to create a new list instance.
The mutability of lists is a fundamental feature in Python, making them suitable for various applications, such as dynamic data storage and manipulation. This contrasts with immutable data types like tuples, where the elements cannot be altered after creation.
Key Features of Mutable Lists
When working with mutable lists, several key features stand out:
- Element Modification: Individual elements can be changed by assigning a new value to a specific index.
- Dynamic Sizing: Lists can grow or shrink in size as elements are added or removed.
- Order Preservation: The order of elements in a list is preserved, allowing for consistent retrieval of items.
Common List Operations
Here are some common operations that demonstrate the mutability of Python lists:
- Appending Elements: Use the `append()` method to add an element to the end of the list.
- Inserting Elements: The `insert(index, value)` method allows you to add an element at a specific position.
- Removing Elements: The `remove(value)` method can be used to delete the first occurrence of a specified value.
- Updating Elements: You can update an element by assigning a new value to its index.
Operation | Method | Example |
---|---|---|
Append | list.append(value) | my_list.append(4) |
Insert | list.insert(index, value) | my_list.insert(1, 5) |
Remove | list.remove(value) | my_list.remove(2) |
Update | list[index] = new_value | my_list[0] = 10 |
Performance Considerations
While mutability offers numerous advantages, it is essential to be aware of performance implications when modifying lists. The following points highlight important considerations:
- Time Complexity: Operations like appending and removing elements generally have an average time complexity of O(1). However, inserting elements can have a time complexity of O(n) due to the need for shifting elements.
- Memory Management: Lists may require additional memory allocation when they grow beyond their current capacity, which can lead to overhead during resizing.
- Copying Behavior: When you assign a list to another variable, you create a reference to the same list, not a copy. This means changes made through one variable will affect the other.
By understanding the mutability of Python lists, developers can leverage their capabilities to create more efficient and effective data structures. This foundational knowledge is critical for mastering Python programming and utilizing its data handling features effectively.
Understanding Python Lists and Their Mutability
Python lists are a fundamental data structure that allows for the storage of ordered collections of items. One of the key characteristics of lists in Python is their mutability.
What Does Mutable Mean?
Mutability refers to the ability of an object to be changed after it has been created. In the context of Python lists, this means that you can modify, add, or remove elements from a list without needing to create a new list.
Characteristics of Mutable Objects
When dealing with mutable objects like lists, consider the following traits:
- In-place Modification: Elements can be changed directly, allowing for efficient memory use.
- Dynamic Size: Lists can grow or shrink in size as elements are added or removed.
- Support for Different Data Types: Lists can hold mixed data types, including other lists.
Examples of List Mutability
To illustrate the mutability of Python lists, consider the following examples:
“`python
Creating a list
my_list = [1, 2, 3]
Modifying an element
my_list[1] = 20
my_list is now [1, 20, 3]
Adding an element
my_list.append(4)
my_list is now [1, 20, 3, 4]
Removing an element
my_list.remove(20)
my_list is now [1, 3, 4]
“`
List Operations That Demonstrate Mutability
The following operations showcase how lists can be modified:
Operation | Example | Result |
---|---|---|
Assigning a new value | `my_list[0] = 10` | Changes first item |
Appending an item | `my_list.append(5)` | Adds 5 to the end |
Inserting an item | `my_list.insert(1, 15)` | Inserts 15 at index 1 |
Extending a list | `my_list.extend([6, 7])` | Adds multiple items |
Removing by index | `del my_list[2]` | Deletes item at index 2 |
Clearing the list | `my_list.clear()` | Empties the list |
Comparison with Immutable Objects
In contrast to mutable lists, Python also has immutable types, such as tuples. The main differences are:
Feature | Mutable (List) | Immutable (Tuple) |
---|---|---|
Change after creation | Yes | No |
Size modification | Yes | No |
Syntax | `[]` | `()` |
Performance impact | Generally slower | Generally faster |
Conclusion on List Mutability
Python lists are indeed mutable, allowing for a wide range of operations that modify their contents. This flexibility makes them a popular choice for developers when working with collections of data that require dynamic manipulation.
Understanding the Mutability of Python Lists
Dr. Emily Carter (Computer Science Professor, Tech University). “Python lists are indeed mutable, which means that their contents can be changed without creating a new list. This characteristic allows for efficient data manipulation, making lists a fundamental data structure in Python programming.”
James Liu (Senior Software Engineer, CodeCraft Solutions). “The mutability of Python lists is one of their most powerful features. Developers can add, remove, or modify elements in place, which is crucial for dynamic data handling in applications.”
Maria Gonzalez (Data Scientist, Insight Analytics). “Understanding that Python lists are mutable is essential for managing memory effectively. It allows for in-place updates, which can significantly enhance performance in data processing tasks.”
Frequently Asked Questions (FAQs)
Are Python lists mutable?
Yes, Python lists are mutable, meaning their elements can be changed, added, or removed after the list has been created.
What does it mean for a data structure to be mutable?
A mutable data structure allows modifications to its contents without changing its identity. This includes adding, removing, or altering elements.
Can I change an element in a Python list?
Yes, you can change an element in a Python list by accessing it via its index and assigning a new value.
How do I add elements to a Python list?
You can add elements to a Python list using methods such as `append()`, `extend()`, or `insert()`, depending on how you want to add the elements.
Are there any immutable data structures in Python?
Yes, tuples and strings are examples of immutable data structures in Python, meaning their contents cannot be altered after creation.
What are the implications of using mutable data structures like lists?
Using mutable data structures can lead to unintended side effects if the same list is referenced in multiple places. Care should be taken to avoid modifying shared lists inadvertently.
In summary, Python lists are indeed mutable, which means that their contents can be modified after the list has been created. This mutability allows for a wide range of operations, such as adding, removing, or changing elements within the list without the need to create a new list. This characteristic is fundamental to how lists function in Python, providing flexibility and efficiency in data manipulation.
One of the key takeaways is that mutability in Python lists enables developers to perform in-place modifications, which can enhance performance by reducing the overhead associated with creating new objects. For example, methods like `append()`, `extend()`, and `remove()` allow for dynamic changes to the list, making it a powerful tool for handling collections of data.
Additionally, understanding the mutability of lists is crucial for avoiding common pitfalls, especially when dealing with references to lists. Since lists are mutable, changes made to a list through one reference will be reflected in all references to that list. This behavior can lead to unintended consequences if not properly managed, emphasizing the importance of careful programming practices when working with mutable data structures.
Author Profile
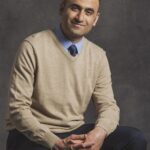
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?