Are Sets Immutable in Python? Exploring the Nature of Python Sets
In the dynamic world of Python programming, understanding the nuances of data structures is crucial for efficient coding and problem-solving. One question that often arises among developers, both novice and experienced, is whether sets in Python are immutable. This inquiry not only touches on the fundamental characteristics of sets but also delves into the broader concepts of mutability and immutability in Python. As we explore this topic, we will uncover the nature of sets, their behavior in various contexts, and how this impacts your coding practices.
At its core, the concept of immutability refers to the inability to change an object after it has been created. In Python, this characteristic applies to certain data types, while others are designed to be mutable, allowing for modifications. Sets, which are collections of unique elements, present an intriguing case in this discussion. While they offer flexibility in terms of adding and removing elements, the question remains: does this mean they are inherently mutable or can they be considered immutable in any sense?
As we dive deeper, we will clarify the distinction between mutable and immutable types, examine the properties of sets, and explore how these characteristics influence their use in programming. By the end of this article, you will have a comprehensive understanding of sets in Python and how their mutability affects your coding
Understanding Immutability in Python Sets
In Python, the term “immutable” refers to an object whose state cannot be modified after it has been created. However, when it comes to sets, the situation is nuanced. Sets themselves are mutable, meaning that their contents can be changed. You can add or remove elements from a set without creating a new set.
For instance, you can perform the following operations:
- Adding elements: You can use the `add()` method to include a new element.
- Removing elements: You can remove an element using the `remove()` or `discard()` methods.
Here’s a simple example demonstrating this:
“`python
my_set = {1, 2, 3}
my_set.add(4) my_set is now {1, 2, 3, 4}
my_set.remove(2) my_set is now {1, 3, 4}
“`
However, the elements contained within a set must be immutable. This means you cannot have mutable types such as lists or dictionaries as elements of a set. If you attempt to include a mutable object, Python will raise a `TypeError`.
Mutable vs. Immutable Types
To clarify the distinction between mutable and immutable objects in Python, consider the following table:
Type | Mutability | Examples |
---|---|---|
Mutable | Can be changed | Lists, Dictionaries, Sets |
Immutable | Cannot be changed | Tuples, Strings, Frozensets |
Creating Immutable Sets
If you need a set that cannot be altered, Python provides a built-in type called `frozenset`. A `frozenset` behaves like a regular set but is immutable. Once created, you cannot add or remove elements from a `frozenset`.
Example of creating a `frozenset`:
“`python
my_frozenset = frozenset([1, 2, 3])
“`
Attempting to modify a `frozenset`, such as adding or removing elements, will result in an `AttributeError`.
While sets in Python are mutable and allow for dynamic modification of their contents, the elements within those sets must be immutable. For scenarios requiring immutability, `frozenset` serves as an effective alternative, ensuring the integrity of the data structure. Understanding these distinctions is crucial for effective data management in Python programming.
Understanding Set Mutability in Python
In Python, sets are mutable data structures. This means that you can add or remove elements from a set after it has been created. However, the elements that can be added to a set must be immutable themselves.
Mutable vs Immutable Types
- Mutable Types: These are objects that can be modified after their creation. Examples include:
- Lists
- Dictionaries
- Sets
- Immutable Types: These objects cannot be altered once created. Examples include:
- Tuples
- Strings
- Frozensets
Characteristics of Sets
Sets in Python have several important characteristics:
- Unordered: The elements in a set do not maintain a specific order.
- Unique Elements: Sets automatically discard duplicate entries, ensuring all elements are unique.
- Dynamic Size: Sets can grow or shrink as elements are added or removed.
Operations on Sets
You can perform various operations on sets due to their mutable nature:
- Adding Elements:
- Use `add()` method to insert a single element.
- Use `update()` method to add multiple elements from an iterable.
- Removing Elements:
- Use `remove()` method to delete a specific element; raises a KeyError if the element is not found.
- Use `discard()` method to delete an element without raising an error if it doesn’t exist.
- Use `pop()` method to remove and return an arbitrary element from the set.
- Clear the Set:
- Use `clear()` method to remove all elements from the set.
Immutable Set: Frozenset
While regular sets are mutable, Python provides a variant called `frozenset`, which is immutable. This means once a frozenset is created, its elements cannot be changed, added, or removed.
Feature | Set | Frozenset |
---|---|---|
Mutability | Mutable | Immutable |
Methods | add(), remove(), update(), clear() | No add/remove methods |
Use Cases | Dynamic collections | Hashable collections for use as dictionary keys or set elements |
Best Practices
When working with sets, consider the following best practices:
- Always ensure that elements added to a set are hashable (i.e., immutable types).
- Use frozensets when you need a collection of unique items that should not change throughout the program.
- Take advantage of set operations like union, intersection, and difference to perform mathematical set operations efficiently.
By understanding the mutability of sets and their operations, you can effectively utilize them in your Python programming tasks.
Understanding the Immutability of Sets in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In Python, sets are mutable, meaning that the elements within a set can be changed after its creation. However, the set itself cannot contain mutable elements like lists or dictionaries, which can lead to confusion regarding the concept of immutability.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “While sets in Python are mutable, it is important to note that once a set is created, you cannot change its identity. You can add or remove elements, but the set will always be a collection of unique, unordered items.”
Sarah Thompson (Python Educator, LearnPython.org). “The misconception about sets being immutable often arises from their behavior in relation to other data structures. Sets provide a unique feature set that allows for dynamic changes, but they must always consist of immutable items, reinforcing the importance of understanding how mutability works in Python.”
Frequently Asked Questions (FAQs)
Are sets immutable in Python?
No, sets in Python are mutable. You can add or remove elements from a set after it has been created.
What is the difference between a set and a frozenset in Python?
A set is mutable, allowing modifications, while a frozenset is immutable and cannot be altered once created.
Can you store mutable objects in a set?
You can store immutable objects in a set, but mutable objects (like lists or dictionaries) cannot be added to a set since they are not hashable.
How do you create a set in Python?
A set can be created using curly braces `{}` or the `set()` function. For example, `my_set = {1, 2, 3}` or `my_set = set([1, 2, 3])`.
What happens if you try to modify a frozenset?
Attempting to modify a frozenset will result in a `AttributeError` since frozensets do not support item assignment or any method that alters their contents.
Are sets ordered in Python?
Sets are unordered collections, meaning that the elements do not maintain any specific order. However, starting from Python 3.7, the insertion order is preserved in the implementation of sets.
In Python, sets are mutable collections, meaning that their contents can be changed after the set has been created. This mutability allows for the addition and removal of elements, providing flexibility in managing collections of unique items. However, it is important to note that while the set itself can be modified, the elements contained within a set must be immutable types. This means that you cannot include lists or other sets as elements within a set, but you can include immutable types such as integers, strings, and tuples.
The distinction between the mutability of sets and the immutability of their elements is crucial for developers working with Python. Understanding this concept helps prevent errors related to type compatibility when attempting to include various data types in sets. Additionally, the mutable nature of sets allows for efficient operations such as union, intersection, and difference, which are essential for various programming tasks that involve collection manipulation.
In summary, sets in Python are mutable, allowing for dynamic changes to their contents, while the elements within them must be immutable. This duality provides both flexibility and constraints, making sets a powerful tool for managing unique collections of data in Python programming. Developers should leverage this understanding to effectively utilize sets in their applications, ensuring they adhere to the rules governing
Author Profile
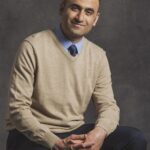
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?