How Can You Resolve the Arithmetic Overflow Error When Converting Varchar to Numeric Data Type?
In the world of database management and programming, data integrity is paramount. However, as systems evolve and data volumes grow, developers often encounter perplexing errors that can disrupt workflows and lead to significant setbacks. One such error is the notorious “arithmetic overflow error converting varchar to data type numeric.” This issue not only highlights the complexities of data types but also underscores the importance of understanding how different formats interact within a database. In this article, we will delve into the intricacies of this error, exploring its causes, implications, and strategies for resolution.
Overview
At its core, the arithmetic overflow error occurs when a system attempts to convert a string representation of a number (varchar) into a numeric data type, but the value exceeds the limits that the numeric type can accommodate. This situation often arises in scenarios where data is imported from external sources, or when user inputs are not properly validated. The error serves as a critical reminder of the need for robust data handling practices, particularly in environments where large datasets are manipulated.
Moreover, understanding the nuances of data types is essential for developers and database administrators alike. Numeric data types come with predefined limits, and failing to account for these can lead to unexpected errors that not only hinder application performance but can also compromise data accuracy. As
Understanding the Arithmetic Overflow Error
An arithmetic overflow error occurs when a calculation exceeds the data type’s storage capacity. In the context of converting `varchar` to `numeric`, this error typically arises when the string contains a numeric value that is too large or too small to fit within the specified numeric data type. For instance, a `numeric(5,2)` type can only store numbers up to 999.99. If an attempt is made to convert a string representing a number larger than this limit, the overflow error will be triggered.
Common Causes of the Error
The following scenarios often lead to arithmetic overflow errors when converting `varchar` to numeric:
- Exceeding Data Type Limits: The numeric value represented by the `varchar` exceeds the defined precision and scale.
- Invalid Characters: The string contains non-numeric characters or is improperly formatted, leading to conversion failures.
- Empty Strings: Attempting to convert an empty string can also result in an error, depending on the context of the conversion.
- Locale Settings: Differences in decimal separators (e.g., comma vs. period) due to regional settings can affect conversion.
Handling the Error in SQL
To effectively manage arithmetic overflow errors during conversions, consider implementing the following strategies:
- Data Validation: Ensure that the `varchar` values are valid numeric strings before attempting conversion.
- Using TRY_CONVERT(): In SQL Server, the `TRY_CONVERT()` function can be used to safely attempt a conversion, returning `NULL` if it fails instead of throwing an error.
Here is an example of how to use `TRY_CONVERT()`:
“`sql
SELECT TRY_CONVERT(numeric(10, 2), your_column) AS SafeConversion
FROM your_table;
“`
- Casting and Rounding: If applicable, round values before conversion to prevent overflow.
Example Scenario
Consider a table containing sales data where the revenue is stored as a `varchar`:
“`sql
CREATE TABLE SalesData (
Revenue varchar(50)
);
“`
Assuming some entries might be outside the valid range for numeric types, you can run a query to identify problematic entries:
“`sql
SELECT Revenue
FROM SalesData
WHERE TRY_CONVERT(numeric(10, 2), Revenue) IS NULL;
“`
This query helps identify any records that would cause an overflow error upon conversion.
Best Practices to Avoid Overflow Errors
Implementing best practices can significantly reduce the likelihood of encountering arithmetic overflow errors:
Practice | Description |
---|---|
Validate Input Data | Ensure data integrity by checking for valid formats. |
Set Appropriate Data Types | Choose suitable data types based on expected values. |
Use Transactions | Wrap conversions in transactions to handle failures gracefully. |
Monitor and Log Errors | Keep track of any errors encountered during conversions for further analysis. |
By adhering to these best practices, you can effectively mitigate the risks associated with arithmetic overflow errors during the conversion from `varchar` to numeric types.
Understanding Arithmetic Overflow Error
Arithmetic overflow occurs when an operation produces a result that exceeds the maximum limit of the data type being used. In the context of converting a `varchar` to a numeric type, this error typically arises when the value in the `varchar` string cannot be accurately represented within the constraints of the numeric data type.
Common Causes
Several scenarios can lead to this error when working with data conversions:
- Exceeding Numeric Precision: The numeric value in the `varchar` string exceeds the defined precision for the target numeric data type.
- Invalid Characters: The presence of non-numeric characters (e.g., letters, symbols) in the `varchar` string can disrupt the conversion process.
- Inadequate Scale: When the numeric value contains decimal places that exceed the allowed scale of the target numeric type.
- Empty or Null Values: Attempting to convert empty strings or `NULL` values can also trigger errors, depending on the context.
Data Type Limits
Understanding the limits of various numeric data types can help prevent overflow errors:
Data Type | Maximum Value | Precision | Scale |
---|---|---|---|
`int` | 2,147,483,647 | 10 | 0 |
`bigint` | 9,223,372,036,854,775,807 | 19 | 0 |
`decimal(p,s)` | 10^p – 1 (for p <= 38) | p | s |
`numeric(p,s)` | Same as decimal | p | s |
`float` | Approx. 1.79E+308 | Varies | Varies |
Troubleshooting Tips
When encountering an arithmetic overflow error during conversion, consider the following strategies:
- Validate Input Data: Ensure that the `varchar` data does not contain invalid characters and is formatted correctly.
- Increase Precision and Scale: Modify the target data type to allow for greater precision and scale, if necessary.
- Use TRY_CONVERT or TRY_CAST: These SQL functions can safely handle conversion attempts and return `NULL` for failed conversions, avoiding errors.
- Check for Nulls and Empties: Implement logic to handle empty or `NULL` values gracefully before conversion.
Example Scenarios
Here are examples that illustrate common situations leading to arithmetic overflow errors:
- Example 1: Trying to convert a `varchar` value “12345678901234567890” to `int` will result in an overflow since the value exceeds `int`’s maximum limit.
- Example 2: A `varchar` value “12345.6789” being converted to `decimal(5,2)` will fail because it exceeds the defined precision and scale.
Preventive Measures
To minimize the likelihood of encountering arithmetic overflow errors:
- Data Validation: Implement robust validation processes for incoming data.
- Type Conversion Awareness: Familiarize yourself with the limitations of data types being used.
- Error Logging: Set up logging mechanisms to capture and analyze errors when they occur. This can help identify patterns and root causes.
By understanding the causes of arithmetic overflow errors and implementing preventive measures, you can effectively mitigate risks associated with converting `varchar` to numeric data types.
Understanding Arithmetic Overflow Errors in Data Conversion
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “Arithmetic overflow errors when converting varchar to numeric data types often arise from improperly formatted data. It is crucial to validate and sanitize input data to ensure it adheres to the expected numeric format before conversion.”
Michael Thompson (Database Administrator, Cloud Solutions Group). “In my experience, these errors can frequently be mitigated by implementing strict data type checks and utilizing error handling mechanisms. Ensuring that the varchar values are within the acceptable range of the numeric type is essential to prevent overflow.”
Sarah Lee (Software Engineer, Data Integrity Experts). “When encountering arithmetic overflow errors, it is important to examine the source of the varchar data. Often, unexpected characters or out-of-range values can lead to conversion issues. Regular expressions can be a helpful tool for pre-validation.”
Frequently Asked Questions (FAQs)
What causes an arithmetic overflow error when converting varchar to numeric?
An arithmetic overflow error occurs when the value being converted from varchar to numeric exceeds the range that the numeric data type can accommodate. This typically happens with large numbers or inappropriate formatting.
How can I identify the specific value causing the overflow error?
You can identify the problematic value by examining the data in the varchar column. Use SQL queries to filter and review values that are either excessively large or improperly formatted, which may lead to the overflow.
What are common solutions to resolve this error?
Common solutions include ensuring that the varchar values are within the acceptable range for the numeric type, using appropriate data type conversions, or adjusting the numeric type to a larger precision and scale.
Can I prevent arithmetic overflow errors in future data imports?
Yes, you can prevent these errors by implementing data validation checks before importing data, ensuring that varchar values conform to the expected numeric format and range, and using error handling in your import scripts.
What SQL functions can help with converting varchar to numeric safely?
Functions such as TRY_CONVERT or TRY_CAST in SQL Server can help safely convert varchar to numeric. These functions return NULL instead of an error if the conversion fails, allowing you to handle problematic values gracefully.
Is it possible to convert varchar to numeric without losing data?
Yes, it is possible to convert varchar to numeric without losing data by ensuring that the values are properly formatted and within the range of the target numeric type. Additionally, using a larger numeric type can help accommodate a wider range of values.
Arithmetic overflow errors that occur during the conversion of varchar to numeric data types are common issues encountered in database management systems. These errors typically arise when the numeric value represented by the varchar exceeds the range that the target numeric data type can accommodate. For instance, attempting to convert a varchar string representing a very large number into a numeric type with limited precision can lead to an overflow, resulting in an error message. Understanding the specific limits of numeric data types in use is crucial for preventing such errors.
To effectively mitigate arithmetic overflow errors, it is essential to validate and sanitize input data before performing conversions. This involves checking the varchar data for valid numeric formats and ensuring that the values fall within acceptable ranges for the target numeric type. Implementing robust error handling mechanisms can also help manage exceptions gracefully, allowing for alternative processing or user notifications when conversion issues arise.
In summary, addressing arithmetic overflow errors during varchar to numeric conversions requires a proactive approach that includes careful data validation and an understanding of data type limitations. By employing best practices in data handling and conversion processes, database administrators and developers can minimize the occurrence of these errors, leading to more reliable and efficient database operations.
Author Profile
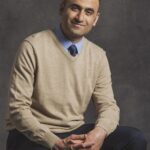
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?