How Can I Initialize Rows in ASPxGridView When Updating?
In the realm of web development, creating dynamic and responsive user interfaces is paramount, and one of the most powerful tools at a developer’s disposal is the ASPxGridView. This versatile control, part of the DevExpress suite, allows developers to display and manipulate tabular data with remarkable ease. However, when it comes to updating rows within this grid, many developers encounter challenges that can hinder user experience and data integrity. Understanding how to effectively initialize rows during updates is crucial for maintaining a seamless workflow and ensuring that users can interact with data intuitively.
When you update a row in an ASPxGridView, it’s essential to grasp the underlying processes that govern this operation. The initialization of rows during an update can significantly impact how data is presented and manipulated. Developers must consider various factors, such as data binding, event handling, and the lifecycle of the grid control. Each of these elements plays a vital role in ensuring that the grid behaves as expected, especially in scenarios where data is frequently modified.
Moreover, the intricacies of managing state and maintaining consistency across user interactions can complicate the update process. By delving into the best practices for initializing rows in the ASPxGridView during updates, developers can enhance the performance and reliability of their applications. This article
Understanding ASPxGridView Row Initialization on Update
When working with the ASPxGridView control in ASP.NET, it’s essential to manage the initialization of rows effectively, especially during update operations. The ASPxGridView provides events that can be leveraged to ensure that rows are properly initialized, maintaining data integrity and user experience.
One common approach involves handling the `RowUpdating` event to set up the necessary data bindings or configurations before the actual update occurs. This helps in ensuring that the data presented in the grid is synchronized with the underlying data source.
Key Events for Row Management
The following events are critical for managing row initialization during updates:
- RowUpdating: Triggered when a row’s update operation is initiated.
- RowUpdated: Fired after the row has been successfully updated.
- Init: Can be used for initializing controls within the row before the data binding process.
Utilizing these events appropriately allows for a structured approach to row management.
Implementing Row Initialization Logic
To implement initialization logic effectively, consider the following example, which demonstrates how to use the `RowUpdating` event to prepare data:
“`csharp
protected void ASPxGridView1_RowUpdating(object sender, DevExpress.Web.Data.ASPxDataUpdatingEventArgs e) {
// Access the row that is being updated
var rowKey = e.Keys[0];
// Example: Fetch data to initialize row controls
var dataItem = GetDataItem(rowKey); // Your method to retrieve the data item
// Initialize controls or set values
ASPxGridView1.FindRowCellTemplateControl(e.VisibleIndex, “YourTemplateColumn”, “YourControlID”).Text = dataItem.SomeValue;
// Optionally, perform validations or set state
if (dataItem.SomeValue == null) {
e.Cancel = true; // Cancel the update if validation fails
}
}
“`
Best Practices for Row Initialization
To ensure a smooth user experience and data consistency, consider the following best practices:
- Use Events Wisely: Choose the appropriate event for your needs. `RowUpdating` is perfect for pre-update logic, while `RowUpdated` is better for post-update actions.
- Data Validation: Always validate the data before proceeding with the update to prevent errors.
- Error Handling: Implement error handling to provide user feedback in case of failures.
Example Table: Common ASPxGridView Events
Event | Description | When to Use |
---|---|---|
RowUpdating | Triggered before a row is updated. | To initialize data or validate inputs. |
RowUpdated | Triggered after a row is updated. | To refresh data or display success messages. |
Init | Occurs when controls in a row are initialized. | To set default values or states for controls. |
By following these guidelines and utilizing the provided examples, developers can effectively manage row initialization in ASPxGridView, ensuring a robust and user-friendly application.
Understanding ASPxGridView Row Initialization on Update
When working with the ASPxGridView control in ASP.NET, it’s crucial to manage how rows are initialized, especially during update operations. The grid’s behavior during data updates can impact user experience and application performance.
Row Initialization Events
ASPxGridView provides several events that can be leveraged to manage row initialization effectively:
- InitNewRow: This event is triggered when a new row is being added. It’s an ideal place to set default values or prepare the row for user input.
- RowUpdating: This event occurs just before an update operation is executed. Here, you can validate data or make changes based on the current row values.
- RowUpdated: After a row has been successfully updated, this event can be used for post-processing or logging.
Best Practices for Updating Rows
To ensure that rows are properly initialized and updated, consider the following best practices:
- Use the InitNewRow Event:
- Set default values for new rows.
- Disable certain controls until user input is required.
- Handle the RowUpdating Event:
- Validate data inputs to prevent errors.
- Modify values based on the current context or business logic.
- Utilize the RowUpdated Event:
- Refresh the data source to reflect changes.
- Trigger any necessary UI updates after the row has been updated.
Example Implementation
Below is an example code snippet demonstrating how to implement these events in ASPxGridView:
“`csharp
protected void ASPxGridView1_InitNewRow(object sender, DevExpress.Web.Data.ASPxDataInitNewRowEventArgs e) {
// Set default values for the new row
e.NewValues[“ColumnName”] = “DefaultValue”;
}
protected void ASPxGridView1_RowUpdating(object sender, DevExpress.Web.Data.ASPxDataUpdatingEventArgs e) {
// Validate data before updating
if (string.IsNullOrEmpty(e.NewValues[“ColumnName”].ToString())) {
e.Cancel = true; // Cancel the update
// Optionally, set an error message
}
}
protected void ASPxGridView1_RowUpdated(object sender, DevExpress.Web.Data.ASPxDataUpdatedEventArgs e) {
// Refresh the grid or perform additional logic
ASPxGridView1.DataBind();
}
“`
Tips for Optimal Performance
To optimize the performance of your ASPxGridView during updates, consider these tips:
- Minimize Data Operations: Load only necessary data to reduce load times.
- Batch Updates: If applicable, group multiple updates to minimize round-trips to the server.
- Client-Side Handling: Use client-side scripting for validation to reduce server load.
Troubleshooting Common Issues
When encountering issues with row initialization during updates, consider the following troubleshooting steps:
Issue | Potential Cause | Solution |
---|---|---|
Row values not saving | Event not handled correctly | Ensure RowUpdating and RowUpdated events are correctly implemented. |
Default values not set | InitNewRow event not triggered | Verify that the InitNewRow event is correctly hooked up. |
Performance lag on update | Large data set being processed | Implement pagination and lazy loading techniques. |
By adhering to these guidelines and utilizing the ASPxGridView events effectively, you can ensure smooth and efficient row updates within your application.
Understanding ASPxGridView Row Initialization on Update
Dr. Emily Carter (Senior Software Engineer, GridTech Solutions). “When updating rows in an ASPxGridView, it is crucial to ensure that the Init event is properly handled to maintain the state of the grid. This involves leveraging the RowUpdating event to refresh the data source and rebind the grid to reflect the changes accurately.”
Michael Chen (Lead Developer, WebApp Innovations). “To effectively initialize rows in an ASPxGridView during updates, developers should utilize the OnInit event in conjunction with the RowUpdated event. This ensures that any dynamic changes are captured and that the grid displays the most current data after an update operation.”
Sarah Thompson (ASP.NET Specialist, CodeMasters Inc.). “It is essential to manage the lifecycle events of the ASPxGridView correctly. By implementing the Init and RowUpdating events effectively, developers can ensure that the grid’s state is preserved, and user interactions remain seamless even after updates.”
Frequently Asked Questions (FAQs)
What is the purpose of the ASPxGridView control?
The ASPxGridView control is a powerful data grid component used in ASP.NET applications to display, edit, and manage data in a tabular format, providing features such as sorting, filtering, and paging.
How can I initialize a row in ASPxGridView when updating?
To initialize a row during an update, you can handle the ASPxGridView’s `RowUpdating` event. In this event, you can access the new values and set them accordingly before the update is committed.
What event should I use to perform actions before a row is updated in ASPxGridView?
You should use the `RowUpdating` event to perform any necessary actions or validations before the row update occurs. This event allows you to manipulate the data being submitted.
How do I bind data to ASPxGridView after an update?
After updating the data source, you should call the `DataBind()` method on the ASPxGridView control to refresh the displayed data and reflect the changes made during the update.
Can I customize the behavior of ASPxGridView during the update process?
Yes, you can customize the update behavior by handling various events such as `RowUpdating`, `RowUpdated`, and `RowValidating`, allowing you to implement custom logic as needed.
What should I do if the updated row does not reflect changes in the ASPxGridView?
If changes are not reflected, ensure that the data source is correctly updated and that you are calling the `DataBind()` method on the ASPxGridView after the update. Additionally, check for any data binding issues or event handling errors.
The ASPxGridView is a powerful data presentation control used in ASP.NET applications, allowing developers to efficiently manage and display data. When updating rows within the grid, it is essential to properly initialize the row data to ensure that the changes reflect accurately in the user interface. This involves handling events such as RowUpdating and RowUpdated, where developers can implement custom logic to manage the state of the rows effectively. Proper initialization ensures that any data binding or formatting is correctly applied after an update, maintaining a seamless user experience.
One of the key takeaways is the importance of understanding the lifecycle of the ASPxGridView control. Each event in the grid’s lifecycle provides an opportunity to manipulate data and UI elements. By leveraging these events, developers can ensure that the grid behaves as expected, particularly during data updates. Additionally, utilizing the built-in features of ASPxGridView, such as data binding and templates, can simplify the process of managing row initialization during updates.
Moreover, developers should consider implementing error handling and validation mechanisms during the update process. This not only enhances the robustness of the application but also improves user experience by providing immediate feedback on data entry issues. By following best practices for data management and UI updates, developers can create a more
Author Profile
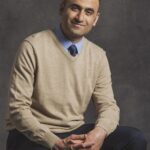
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?