How Can Async JavaScript Enhance Your Interaction with REST APIs?
In the ever-evolving landscape of web development, the ability to handle asynchronous operations has become a cornerstone of building responsive and efficient applications. As developers increasingly turn to REST APIs for seamless data integration, mastering asynchronous JavaScript is essential for creating dynamic user experiences. Whether you’re fetching user data, submitting forms, or interacting with third-party services, understanding how to effectively manage asynchronous requests can set your applications apart. This article delves into the synergy between async JavaScript and REST APIs, guiding you through the principles and practices that will enhance your coding toolkit.
Asynchronous JavaScript allows developers to execute tasks without blocking the main thread, enabling smoother interactions and faster load times. By leveraging modern features such as `async` and `await`, developers can write cleaner, more readable code that simplifies the process of handling API requests. REST APIs, with their standardized approach to data exchange, provide a powerful mechanism for accessing and manipulating resources over the web. Together, these technologies empower developers to create applications that not only perform well but also offer a superior user experience.
In this exploration, we will uncover the fundamentals of async programming in JavaScript and how it interfaces with RESTful services. From understanding the nuances of promises to implementing error handling and optimizing performance, you’ll gain insights that will elevate your
Understanding Async JavaScript
Asynchronous JavaScript is a programming paradigm that allows developers to write code that performs non-blocking operations. This means that while a long-running task is being processed, other operations can continue executing, improving the responsiveness of applications. Promises, async/await syntax, and callbacks are the primary mechanisms for handling asynchronous code.
– **Promises**: Objects that represent the eventual completion (or failure) of an asynchronous operation and its resulting value.
– **Async/Await**: Syntactic sugar built on top of promises, allowing for cleaner and more readable asynchronous code.
For example, a simple promise might look like this:
“`javascript
let myPromise = new Promise((resolve, reject) => {
// Simulate an asynchronous operation
setTimeout(() => {
resolve(“Operation Successful!”);
}, 2000);
});
myPromise.then(result => console.log(result));
“`
In this code, the promise will resolve after 2 seconds, demonstrating how other code can run in the meantime.
Making REST API Calls
REST (Representational State Transfer) APIs provide a standardized way for applications to communicate over HTTP. They allow developers to retrieve, create, update, and delete resources. In JavaScript, especially with async/await, making REST API calls can be straightforward and efficient.
Here’s how you can make a GET request to a REST API using async/await:
“`javascript
async function fetchData(url) {
try {
const response = await fetch(url);
if (!response.ok) {
throw new Error(‘Network response was not ok’);
}
const data = await response.json();
console.log(data);
} catch (error) {
console.error(‘There was a problem with the fetch operation:’, error);
}
}
fetchData(‘https://api.example.com/data’);
“`
In this example, the `fetchData` function retrieves data from the specified URL. It uses the `fetch` API to initiate the request and handles errors gracefully.
Combining Async JavaScript with REST APIs
Integrating async JavaScript with REST APIs allows developers to create dynamic web applications that can update content without refreshing the page. This is particularly useful for single-page applications (SPAs) where user experience is paramount.
When working with REST APIs asynchronously, consider the following best practices:
- Error Handling: Always include error handling to manage network issues or unexpected responses.
- Loading Indicators: Show a loading indicator during API calls to enhance user experience.
- Rate Limiting: Be mindful of API rate limits; implement throttling when necessary.
Method | Description | Use Case |
---|---|---|
GET | Retrieve data from the server | Fetching user profiles |
POST | Send data to the server | Creating a new user |
PUT | Update existing data | Editing user information |
DELETE | Remove data from the server | Deleting a user account |
This table provides a quick overview of common HTTP methods used in RESTful APIs and their typical use cases. Understanding these methods is crucial for effective API interaction in your JavaScript applications.
Understanding Asynchronous JavaScript
Asynchronous JavaScript allows for non-blocking operations, enabling developers to perform tasks such as fetching data from APIs without freezing the user interface. This is achieved through various mechanisms, primarily Promises and the async/await syntax.
- Promises: Objects that represent the eventual completion (or failure) of an asynchronous operation, allowing for cleaner handling of asynchronous tasks.
- Callbacks: Functions passed as arguments to handle asynchronous results but can lead to callback hell when nested excessively.
- Async/Await: A syntactic sugar built on Promises, making asynchronous code look synchronous and more readable.
Example of using async/await:
“`javascript
async function fetchData(url) {
try {
const response = await fetch(url);
if (!response.ok) {
throw new Error(‘Network response was not ok’);
}
const data = await response.json();
return data;
} catch (error) {
console.error(‘Fetch error:’, error);
}
}
“`
Interacting with REST APIs
REST (Representational State Transfer) APIs are widely used for web services, allowing clients to interact with servers using standard HTTP methods. Key operations include:
- GET: Retrieve data from the server.
- POST: Submit data to be processed.
- PUT: Update existing data.
- DELETE: Remove data from the server.
To interact with a REST API in JavaScript, the Fetch API is commonly used. It provides a simple interface for making HTTP requests.
Using Fetch API with Async JavaScript
The Fetch API returns a Promise, making it straightforward to use with async/await. Below is an example of how to perform a GET request to fetch data from a REST API.
“`javascript
async function getUserData(userId) {
const apiUrl = `https://jsonplaceholder.typicode.com/users/${userId}`;
const userData = await fetch(apiUrl);
const user = await userData.json();
return user;
}
“`
For a POST request to send data, the Fetch API can be configured with additional options:
“`javascript
async function createUser(user) {
const apiUrl = ‘https://jsonplaceholder.typicode.com/users’;
const response = await fetch(apiUrl, {
method: ‘POST’,
headers: {
‘Content-Type’: ‘application/json’
},
body: JSON.stringify(user)
});
const newUser = await response.json();
return newUser;
}
“`
Handling Errors in Async Operations
Error handling is crucial when working with asynchronous operations. Using try/catch blocks ensures that errors are caught gracefully. Here’s an example:
“`javascript
async function getData(url) {
try {
const response = await fetch(url);
if (!response.ok) {
throw new Error(‘Network response was not ok’);
}
return await response.json();
} catch (error) {
console.error(‘Error fetching data:’, error);
}
}
“`
Best Practices
When working with asynchronous JavaScript and REST APIs, consider the following best practices:
- Use Async/Await: Prefer async/await for cleaner, more readable code.
- Error Handling: Always implement error handling for network requests.
- Avoid Blocking Calls: Ensure that no synchronous operations hinder the responsiveness of your application.
- Limit Concurrent Requests: Manage the number of concurrent requests to avoid overwhelming the server.
- Caching: Implement caching strategies to enhance performance and reduce server load.
By adhering to these practices, developers can create efficient, robust applications that leverage the full potential of asynchronous JavaScript and RESTful APIs.
Expert Insights on Async JavaScript and REST APIs
Dr. Emily Carter (Lead Software Engineer, Tech Innovations Inc.). “Asynchronous JavaScript is crucial for enhancing user experience when interacting with REST APIs. By using async/await syntax, developers can write cleaner and more readable code, allowing for better error handling and improved performance in web applications.”
Michael Chen (Senior Web Architect, Digital Solutions Group). “Incorporating async JavaScript with REST APIs enables developers to make non-blocking calls, which is essential for modern web applications. This approach not only optimizes the loading times but also ensures that the UI remains responsive, providing a seamless experience for users.”
Sarah Patel (Full Stack Developer, CodeCraft Labs). “Utilizing async functions when working with REST APIs allows for better management of asynchronous operations. By leveraging Promises, developers can avoid callback hell and write more maintainable code, ultimately leading to more efficient and scalable applications.”
Frequently Asked Questions (FAQs)
What is async JavaScript?
Async JavaScript refers to the use of asynchronous programming techniques in JavaScript, allowing code to execute without blocking the main thread. This enables non-blocking operations, such as fetching data from a server, which enhances performance and user experience.
How do I use async/await with a REST API?
To use async/await with a REST API, define an asynchronous function using the `async` keyword. Inside this function, use the `await` keyword before calling the `fetch` function to retrieve data from the API. This allows the code to pause until the promise is resolved, simplifying the handling of asynchronous operations.
What is the difference between fetch and axios for REST APIs?
Fetch is a built-in JavaScript function for making network requests, while Axios is a third-party library that simplifies HTTP requests. Axios automatically transforms JSON data, handles timeouts, and provides better error handling compared to the native fetch API.
Can I make concurrent requests to a REST API using async JavaScript?
Yes, you can make concurrent requests using async JavaScript by utilizing `Promise.all()`. This method allows you to execute multiple asynchronous operations simultaneously and wait for all of them to complete, improving efficiency when dealing with multiple API calls.
What are common errors when using async JavaScript with REST APIs?
Common errors include network issues, incorrect API endpoints, and improper handling of promises. Additionally, forgetting to use `await` when calling asynchronous functions can lead to unexpected results, as the code may execute before the promise is resolved.
How can I handle errors in async JavaScript when calling a REST API?
To handle errors in async JavaScript, use a try-catch block around your await calls. This allows you to catch any exceptions that occur during the fetch operation and handle them appropriately, such as logging the error or displaying a message to the user.
Asynchronous JavaScript plays a crucial role in enhancing the performance and user experience of web applications, particularly when interacting with REST APIs. By utilizing asynchronous programming techniques, developers can make non-blocking calls to APIs, allowing for smoother user interfaces and faster data retrieval. This approach is essential in today’s web development landscape, where responsiveness and efficiency are paramount.
REST APIs, which adhere to the principles of Representational State Transfer, facilitate seamless communication between client and server. They allow developers to access and manipulate resources over the web using standard HTTP methods. When combined with asynchronous JavaScript, such as through the use of Promises, async/await syntax, or libraries like Axios, developers can easily handle API requests and responses without freezing the user interface.
Key takeaways from the discussion include the importance of understanding asynchronous programming concepts in JavaScript, as they are vital for effective API integration. Furthermore, leveraging tools like Fetch API or Axios can simplify the process of making API calls. Finally, mastering error handling and response management is essential for building robust applications that can gracefully handle unexpected issues during API interactions.
Author Profile
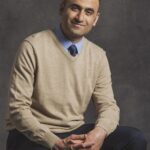
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?