Why Am I Getting ‘AttributeError: ‘connection’ Object Has No Attribute ‘cursor” and How Can I Fix It?
In the world of programming, encountering errors is an inevitable part of the journey, especially when working with databases. One such frustrating error that developers often face is the dreaded `AttributeError: ‘connection’ object has no attribute ‘cursor’`. This seemingly cryptic message can halt your progress and leave you scratching your head, wondering where things went wrong. Whether you’re a seasoned developer or a novice just starting to navigate the complexities of database interactions, understanding this error is crucial for troubleshooting and refining your code.
At its core, this error stems from an attempt to access a method or property that doesn’t exist on the specified object—in this case, a database connection. While it may seem like a simple oversight, the implications can be significant, especially in applications that rely heavily on data manipulation and retrieval. As you dive deeper into the intricacies of database connections and their associated methods, you’ll uncover the common pitfalls that lead to this error and learn how to avoid them in your own projects.
Throughout this article, we will explore the underlying causes of the `AttributeError: ‘connection’ object has no attribute ‘cursor’`, offering insights into the nuances of database handling in various programming environments. By the end, you will not only gain a clearer understanding of this error but also acquire
Understanding the Error
The error message `AttributeError: ‘connection’ object has no attribute ‘cursor’` typically arises in Python applications that utilize database connections, particularly when working with libraries like SQLite or MySQL. This error indicates that the program is trying to call the `cursor()` method on a connection object that does not support it, which can stem from several underlying issues.
Common Causes
Several factors can contribute to this error, including:
- Wrong Library Import: Using a database library that does not have a `cursor` method for its connection object.
- Incorrect Connection Object: Attempting to use a connection object that has not been properly instantiated or is of an unexpected type.
- Library Version Mismatch: Using different versions of the library that may not support the same methods as others.
Troubleshooting Steps
To resolve the issue, consider the following troubleshooting steps:
- Check Library Import: Ensure that you are importing the correct library for your database. For instance, if you’re using SQLite, the import should look like this:
“`python
import sqlite3
“`
- Verify Connection Object: Make sure that the connection object you are working with is created correctly. The syntax for creating a connection in SQLite is:
“`python
connection = sqlite3.connect(‘database_name.db’)
“`
- Inspect Object Type: Before calling the `cursor()` method, print the type of the connection object to verify it is what you expect:
“`python
print(type(connection))
“`
- Consult Documentation: Always check the documentation for the specific database library you are using to confirm that the `cursor()` method is supported and how it should be implemented.
Example of Correct Implementation
Below is an example of how to correctly create a connection and use the `cursor()` method in Python with SQLite:
“`python
import sqlite3
Establish a connection to the database
connection = sqlite3.connect(‘example.db’)
Create a cursor object using the connection
cursor = connection.cursor()
Example of executing a query
cursor.execute(‘SELECT * FROM example_table’)
Fetch results
results = cursor.fetchall()
Close the cursor and connection
cursor.close()
connection.close()
“`
Handling Different Database Libraries
Different database libraries may have varying methods for handling connections and cursors. Below is a comparison table illustrating the differences among some popular database libraries in Python:
Library | Connection Method | Cursor Method |
---|---|---|
SQLite | sqlite3.connect() | connection.cursor() |
MySQL | mysql.connector.connect() | connection.cursor() |
PostgreSQL | psycopg2.connect() | connection.cursor() |
SQLAlchemy | create_engine() | session.execute() |
In summary, understanding the context and specifics of the libraries you are working with is crucial to avoid such errors. Always ensure that the connection and cursor methods align with the expectations of the database library being utilized.
Understanding the Error
An `AttributeError` indicates that you are trying to access an attribute or method that does not exist for a particular object. In the case of the error message `attributeerror: ‘connection’ object has no attribute ‘cursor’`, it suggests that the connection object you are working with does not have a method called `cursor()`.
Common Causes
Several issues can lead to this error:
- Incorrect Library Usage: You might be using a database library that does not provide a `cursor()` method on its connection object. For example, some asynchronous libraries or ORM frameworks may handle connections differently.
- Improper Object Instantiation: The connection object may not have been created or initialized properly, leading to unexpected behavior.
- Namespace Conflicts: If there are multiple libraries imported that define a `connection` object, you may inadvertently be referencing the wrong one.
- Version Incompatibilities: Changes in library versions can lead to deprecated methods or altered interfaces.
Troubleshooting Steps
To resolve the error, follow these steps:
- Check Library Documentation: Verify whether the library you are using supports the `cursor()` method on the connection object.
- Inspect Object Type: Use the `type()` function to ensure that your connection object is indeed what you expect it to be:
“`python
print(type(connection))
“`
- Review Initialization Code: Ensure that the connection is established correctly, following the library’s guidelines. For example:
“`python
import sqlite3
connection = sqlite3.connect(‘example.db’)
cursor = connection.cursor() This should work
“`
- Check for Multiple Imports: Look through your imports to avoid conflicts:
“`python
from library1 import connection as conn1
from library2 import connection as conn2
“`
- Update Your Libraries: Ensure you are using the latest version of the database library, as older versions may have bugs or removed features.
Example of a Proper Connection Usage
Here is a code snippet demonstrating the correct usage of a connection and cursor in Python with SQLite:
“`python
import sqlite3
try:
connection = sqlite3.connect(‘example.db’) Establishing connection
cursor = connection.cursor() Creating a cursor
cursor.execute(‘SELECT * FROM example_table’) Executing a query
results = cursor.fetchall() Fetching results
print(results)
except AttributeError as e:
print(f”Attribute error: {e}”)
finally:
if connection:
connection.close() Closing connection
“`
Alternative Libraries
If the current library continues to cause issues, consider using other libraries that are well-supported and have clear documentation:
Library | Description | Cursor Support |
---|---|---|
SQLite | Lightweight disk-based database | Yes |
psycopg2 | PostgreSQL adapter for Python | Yes |
SQLAlchemy | SQL toolkit and ORM | Yes |
PyMySQL | MySQL database adapter | Yes |
Select a library that aligns with your project needs, ensuring compatibility and proper functionality.
Understanding the ‘AttributeError’ in Database Connections
Dr. Emily Chen (Senior Software Engineer, Data Solutions Inc.). “The error ‘attributeerror: ‘connection’ object has no attribute ‘cursor” typically arises when the database connection object is not properly initialized or when using a library that does not support the cursor method. It is crucial to ensure that the connection is established using the correct database driver.”
Michael Thompson (Database Administrator, TechPro Insights). “In many cases, this error indicates that the connection object is not of the expected type. Developers should verify that they are using the right database API and that the connection object is correctly instantiated before attempting to call cursor methods.”
Lisa Patel (Lead Backend Developer, CodeCraft Labs). “To resolve the ‘attributeerror: ‘connection’ object has no attribute ‘cursor”, one should inspect the connection object and confirm its attributes. It may be beneficial to refer to the documentation of the specific database library being used to ensure compatibility and correct usage.”
Frequently Asked Questions (FAQs)
What does the error ‘AttributeError: ‘connection’ object has no attribute ‘cursor” mean?
This error indicates that the connection object you are using does not have a method or attribute called ‘cursor’. This typically occurs when the connection is not properly established or is of an incorrect type.
How can I resolve the ‘AttributeError: ‘connection’ object has no attribute ‘cursor” error?
To resolve this error, ensure that you are using the correct database connection library and that the connection is successfully established. Verify that the connection object is indeed a database connection and not an alternative object type.
What are common causes of the ‘connection’ object not having a ‘cursor’ attribute?
Common causes include using an incorrect database driver, attempting to use a connection object from a different library, or mistakenly overwriting the connection variable with a non-connection object.
Is this error specific to a particular database system?
No, this error can occur with various database systems, including MySQL, PostgreSQL, and SQLite, depending on the library being used and how the connection is managed.
How can I check if my connection object is valid?
You can check if your connection object is valid by using the `isinstance()` function to confirm it is an instance of the expected connection class from your database library. Additionally, you can handle exceptions during connection creation to ensure it is established correctly.
What should I do if I am using an ORM and encounter this error?
If you are using an ORM (Object-Relational Mapping) tool and encounter this error, ensure that you are accessing the connection object correctly as per the ORM’s documentation. Review the ORM’s methods for obtaining a session or connection to avoid direct manipulation of the connection object.
The error message “AttributeError: ‘connection’ object has no attribute ‘cursor'” typically arises in Python when attempting to access the cursor method on a connection object that does not support it. This issue is often encountered in database interactions, particularly when using libraries such as SQLite, MySQL, or PostgreSQL. It indicates that the connection object being referenced either has not been properly instantiated or is not of the expected type that includes the cursor method.
One common cause of this error is the misuse of the connection object, such as attempting to create a cursor from an incorrect or improperly configured database connection. It is essential to ensure that the connection object is correctly established and that the appropriate database driver is being used. Additionally, developers should verify that they are calling the cursor method on a valid connection instance that supports this functionality.
To resolve this error, developers should review their code to confirm that the connection has been successfully created and that it is of the correct type. Implementing error handling and logging can also assist in diagnosing the issue more effectively. Understanding the specific database library being used and its documentation can provide further clarity on the expected methods and attributes associated with connection objects.
In summary, the “AttributeError: ‘connection’
Author Profile
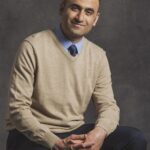
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?