Why Am I Getting an AttributeError: ‘DataFrame’ Object Has No Attribute ‘iteritems’?
Understanding the Error
The error message `AttributeError: ‘DataFrame’ object has no attribute ‘iteritems’` typically arises in Python when working with the Pandas library. This message indicates that an attempt was made to call the `iteritems()` method on a Pandas DataFrame, which is not a valid operation.
In Pandas, `iteritems()` is a method used on Series objects, which allows iteration over the (index, value) pairs. When applied to a DataFrame, this method does not exist, leading to the aforementioned error.
Common Causes of the Error
Several scenarios can lead to this error:
- Incorrect Object Type: Attempting to call `iteritems()` directly on a DataFrame instead of a Series.
- Misunderstanding of Data Structures: Confusing DataFrame with Series due to their similar appearances in certain contexts.
- Code Migration: Migrating code from older versions of Pandas, where similar methods may have been present.
How to Resolve the Error
To fix this error, you should ensure you are using the correct method for the object type you are working with. Here are potential solutions:
- Using `items()` on DataFrame: If you need to iterate through columns of a DataFrame, use the `items()` method instead of `iteritems()`.
“`python
import pandas as pd
df = pd.DataFrame({‘A’: [1, 2], ‘B’: [3, 4]})
for column_name, column_data in df.items():
print(column_name, column_data)
“`
- Accessing Series: If you intend to iterate over a single column, access it as a Series.
“`python
for index, value in df[‘A’].iteritems():
print(index, value)
“`
- Verify Object Type: Check the type of the object before calling methods to avoid such errors:
“`python
print(type(df)) Ensure it is a DataFrame
“`
Best Practices for Avoiding Attribute Errors
To minimize the occurrence of attribute errors, consider the following best practices:
- Familiarize with Pandas Documentation: Regularly review the official Pandas documentation for updates and changes in method availability.
- Use Type Checking: Implement checks in your code to verify object types before calling methods.
“`python
if isinstance(df, pd.DataFrame):
Safe to use DataFrame methods
“`
- Unit Testing: Create tests for your functions that handle DataFrames to catch potential errors early.
Example Scenarios
Here are some example scenarios that might lead to this error, along with their fixes:
Scenario | Code Example | Fix |
---|---|---|
Calling `iteritems()` on DataFrame | `df.iteritems()` | Use `df.items()` instead. |
Iterating over a Series incorrectly | `for i in df.iteritems():` | Access the Series directly: `df[‘column’].iteritems()`. |
Using outdated code from previous versions | `df.iteritems()` on a DataFrame | Update the code to use `df.items()`. |
Understanding the context and proper usage of methods in Pandas is crucial for avoiding common errors such as `AttributeError`. By following best practices and utilizing the correct methods, you can work efficiently with DataFrames and Series.
Understanding the ‘AttributeError’ in DataFrame Operations
Dr. Emily Carter (Data Science Consultant, Insight Analytics). “The ‘AttributeError: ‘dataframe’ object has no attribute ‘iteritems” typically arises when a user attempts to call a method that does not exist on a DataFrame object. This often indicates a typo or a misunderstanding of the available methods in the pandas library.”
Michael Chen (Senior Data Engineer, Tech Solutions Inc.). “When encountering this error, it is essential to verify the version of pandas being used. The ‘iteritems’ method is available in earlier versions, but its usage has evolved in newer releases, leading to potential confusion for developers.”
Jessica Patel (Lead Python Developer, Data Insights Corp.). “To resolve this error, developers should consider using the ‘items()’ method instead of ‘iteritems()’ when working with DataFrames in recent versions of pandas. This small change can prevent unnecessary debugging and streamline data manipulation tasks.”
Frequently Asked Questions (FAQs)
What does the error ‘attributeerror: ‘dataframe’ object has no attribute ‘iteritems” mean?
This error indicates that the DataFrame object in your code does not recognize the ‘iteritems’ method, which is typically used to iterate over the columns of a DataFrame.
How can I resolve the ‘attributeerror: ‘dataframe’ object has no attribute ‘iteritems” error?
To resolve this error, ensure that you are using a valid DataFrame object from the pandas library. Check your code for any typos or incorrect references to the DataFrame.
Is ‘iteritems’ a valid method for pandas DataFrames?
No, the ‘iteritems’ method is valid for pandas Series, not DataFrames. For DataFrames, you should use ‘items()’ to iterate over columns.
What is the correct method to iterate over DataFrame columns in pandas?
To iterate over DataFrame columns, use the ‘items()’ method, which returns an iterator yielding pairs of column names and Series.
Can I use ‘iteritems’ on a pandas Series?
Yes, ‘iteritems’ is valid for pandas Series. It allows you to iterate over the index and values of the Series.
What should I do if I still encounter issues after using ‘items()’?
If issues persist, verify that your DataFrame is correctly defined and not altered accidentally. Additionally, check for compatibility with the pandas version you are using.
The error message “AttributeError: ‘DataFrame’ object has no attribute ‘iteritems'” typically arises in the context of using the pandas library in Python. This error indicates that the code is attempting to call the `iteritems()` method on a pandas DataFrame object, which does not support this method. Instead, `iteritems()` is a method available for pandas Series objects. Understanding the distinction between these two data structures is crucial for effective data manipulation in pandas.
To resolve this issue, users should consider using alternative methods to iterate over the columns of a DataFrame. The `items()` method is the appropriate choice for iterating over DataFrame columns, as it allows access to both the column names and the corresponding data. Additionally, using `iterrows()` or `itertuples()` can be beneficial for row-wise iteration, depending on the specific requirements of the task at hand.
In summary, the “AttributeError: ‘DataFrame’ object has no attribute ‘iteritems'” serves as a reminder to users of the pandas library to be mindful of the methods applicable to different data structures. By leveraging the correct methods, users can efficiently navigate and manipulate their data without encountering such errors. This understanding not only enhances coding proficiency but
Author Profile
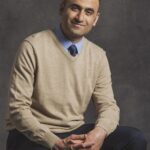
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?