Why Am I Seeing ‘AttributeError: ‘engine’ object has no attribute ‘cursor” and How Can I Fix It?
In the realm of programming, particularly when working with databases in Python, encountering errors can be both frustrating and enlightening. One common error that developers may stumble upon is the infamous `AttributeError: ‘engine’ object has no attribute ‘cursor’`. This seemingly cryptic message can halt your progress, leaving you scratching your head and questioning your code. Understanding the roots of this error not only helps in troubleshooting but also deepens your grasp of how database connections and object-oriented programming work in Python.
As you delve into the intricacies of this error, you’ll discover that it often arises from a misunderstanding of the libraries and frameworks involved in database interactions. Many developers rely on SQLAlchemy for ORM (Object-Relational Mapping), but the nuances of its architecture can lead to confusion, especially when it comes to handling connections and executing queries. The `engine` object, a cornerstone of SQLAlchemy, plays a pivotal role in establishing connections to the database, yet it does not provide a direct method for creating cursors, which can be a source of frustration for those accustomed to traditional database libraries.
By examining the underlying principles of the `engine` object and the correct methodologies for executing SQL commands, you can not only resolve this error but also enhance your overall coding proficiency. This
Common Causes of AttributeError in Database Connections
When encountering the error message `AttributeError: ‘engine’ object has no attribute ‘cursor’`, it is crucial to understand the context in which it arises. This error typically occurs in Python applications that interact with databases using libraries like SQLAlchemy. The underlying cause often relates to the misuse of the database connection object.
Key reasons for this error include:
- Incorrect Object Usage: The `engine` object in SQLAlchemy does not directly provide a cursor method. Instead, it is used to establish a connection to the database.
- Misunderstanding of Library Functions: Users may confuse the roles of the engine and connection objects, leading to incorrect method calls.
- Version Compatibility Issues: Certain versions of SQLAlchemy or other database libraries might have deprecated or altered functionalities, causing the code to break.
Understanding SQLAlchemy Engine and Connection
In SQLAlchemy, the `engine` serves as the starting point for any interaction with the database. It encapsulates the database connection details, while the `connection` object is responsible for executing SQL commands.
Object Type | Description |
---|---|
Engine | Handles the database connection, but does not execute SQL commands directly. |
Connection | Represents an active connection to the database, allowing for execution of SQL commands. |
To properly execute SQL statements, one must obtain a `Connection` object from the `Engine`. This can be achieved using the `connect()` method, as shown below:
“`python
from sqlalchemy import create_engine
engine = create_engine(‘database_url’)
with engine.connect() as connection:
result = connection.execute(“SELECT * FROM table_name”)
“`
Resolving the AttributeError
To resolve the `AttributeError: ‘engine’ object has no attribute ‘cursor’`, the following steps can be taken:
- Use the Correct Object: Ensure that you are using a `Connection` object for executing SQL commands rather than the `Engine`.
- Check Your SQLAlchemy Version: Ensure that you are using a compatible version of SQLAlchemy, as function signatures and capabilities may change over time.
- Refer to Documentation: Always refer to the official SQLAlchemy documentation for the most accurate and up-to-date guidance on object methods and best practices.
By ensuring you are properly utilizing the connection object, you can avoid common pitfalls associated with database interactions in Python applications.
Understanding the Error
The error message `AttributeError: ‘engine’ object has no attribute ‘cursor’` typically arises when working with database connections in Python, particularly with libraries such as SQLAlchemy. This error indicates that the code is attempting to access a cursor method on an `engine` object, which does not exist.
In SQLAlchemy, the `engine` is primarily used to establish connections to a database and execute SQL statements. Unlike some other database libraries, SQLAlchemy’s `engine` does not provide direct access to a cursor. Instead, the correct approach involves creating a connection from the engine.
Common Causes
Several scenarios can lead to this error:
- Incorrect Object Type: Attempting to call `cursor()` on an SQLAlchemy `engine` object instead of a connection object.
- Misunderstanding of SQLAlchemy Design: Using SQLAlchemy with a mindset similar to other libraries such as SQLite or MySQL Connector.
- Version Mismatch: Using outdated documentation or examples that do not align with the installed version of SQLAlchemy.
Correct Usage of SQLAlchemy Engine
To effectively use the SQLAlchemy engine, follow these guidelines:
- Establish a Connection:
Use the `connect()` method or a context manager to create a connection object from the engine.
“`python
from sqlalchemy import create_engine
engine = create_engine(‘sqlite:///example.db’)
with engine.connect() as connection:
result = connection.execute(“SELECT * FROM users”)
for row in result:
print(row)
“`
- Execute Queries:
Utilize the connection object to execute SQL queries directly without calling `cursor()`.
- Use a Session:
For ORM operations, prefer using a session object instead of directly executing raw SQL.
“`python
from sqlalchemy.orm import sessionmaker
Session = sessionmaker(bind=engine)
session = Session()
users = session.query(User).all()
“`
Differences Between SQLAlchemy and Other Libraries
Understanding the distinctions between SQLAlchemy and other database libraries can help prevent this error. Below is a comparison:
Feature | SQLAlchemy | SQLite/MySQL Connector |
---|---|---|
Cursor Access | No direct cursor | Direct cursor access |
Connection Handling | Use `connect()` | Use `connect()` |
Execution of Queries | Use connection object | Use cursor object |
ORM Support | Yes | Limited or no ORM |
Troubleshooting Steps
If you encounter this error, consider the following steps:
- Check Documentation: Review the official SQLAlchemy documentation to ensure correct usage of `engine` and connection.
- Debug the Code: Print the type of the object being used to verify it is indeed an `engine` and not a connection or session.
- Update SQLAlchemy: Ensure you are using the latest version of SQLAlchemy, as features and best practices may change over time.
- Consult Community Forums: Platforms like Stack Overflow can provide insights from other developers who may have faced similar issues.
By adhering to these guidelines and understanding the underlying principles of SQLAlchemy’s design, you can effectively avoid the `AttributeError` and enhance your database interaction in Python.
Understanding the ‘AttributeError’ in Database Engines
Dr. Emily Carter (Senior Software Engineer, Data Solutions Inc.). “The error ‘attributeerror: ‘engine’ object has no attribute ‘cursor” typically arises when the database engine is improperly instantiated. It is crucial to ensure that the engine is created using the correct database connection string and that the relevant database library is properly imported and utilized.”
Michael Chen (Database Administrator, Tech Innovations Corp.). “This error can also indicate that the object you are trying to use does not support the cursor method. In many cases, developers may confuse the engine object with a connection object. It is essential to call the connection method on the engine to obtain a connection that has the cursor attribute.”
Sarah Johnson (Lead Python Developer, CodeCraft Solutions). “When encountering ‘attributeerror: ‘engine’ object has no attribute ‘cursor”, it is advisable to review the documentation of the database library being used. Different libraries have varying methods for executing SQL commands, and understanding these nuances can prevent such errors from occurring in the first place.”
Frequently Asked Questions (FAQs)
What does the error ‘attributeerror: ‘engine’ object has no attribute ‘cursor” mean?
This error indicates that the code is attempting to call a method or access an attribute named ‘cursor’ on an object of type ‘engine’, which does not possess such an attribute. This typically occurs in database connection contexts, particularly when using SQLAlchemy.
How can I resolve the ‘attributeerror: ‘engine’ object has no attribute ‘cursor” error?
To resolve this error, ensure you are using the correct method to interact with the database. Instead of calling ‘cursor’ on the engine object, you should create a connection using `engine.connect()` and then utilize that connection for executing queries.
Is the ‘cursor’ method available in all database engines?
No, the ‘cursor’ method is not universally available across all database engines. In SQLAlchemy, for instance, the ‘engine’ object does not have a ‘cursor’ method; instead, you should use the connection object to execute SQL commands.
What is the correct way to execute a query using SQLAlchemy?
To execute a query using SQLAlchemy, first create a connection with `engine.connect()`, then use the connection to execute the desired SQL statement, typically through methods like `execute()` or by using a session object for ORM operations.
Can this error occur in other programming languages or frameworks?
Yes, similar errors can occur in other programming languages or frameworks when attempting to access non-existent attributes or methods on objects. The underlying principle remains the same: ensure that the object being used supports the intended operations.
What should I check if I encounter this error in my code?
Check the documentation for the database library you are using to confirm the correct methods for executing queries. Additionally, verify that you are correctly initializing and using the database connection and that you are not mistakenly trying to access attributes that do not exist on the object.
The error message “AttributeError: ‘engine’ object has no attribute ‘cursor'” typically arises in Python when working with database connections, particularly in libraries such as SQLAlchemy. This error indicates that the code is attempting to access a cursor method on an engine object, which is not supported. In SQLAlchemy, the engine is responsible for managing connections to the database, but it does not provide a cursor directly. Instead, developers should use the connection object obtained from the engine to execute SQL statements.
To resolve this issue, it is crucial to understand the correct usage of SQLAlchemy’s API. Instead of calling a cursor on the engine, one should first create a connection using the engine’s `connect()` method. Once a connection is established, SQL statements can be executed using the connection object. This approach aligns with SQLAlchemy’s design, which abstracts the underlying database interaction and promotes a more efficient and manageable way to handle database operations.
In summary, the “AttributeError: ‘engine’ object has no attribute ‘cursor'” serves as a reminder of the importance of understanding the specific methods and attributes associated with different objects in a library. By adhering to the correct usage patterns, developers can avoid common pitfalls and ensure that their database interactions are both
Author Profile
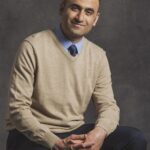
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?