Why Am I Encountering ‘AttributeError: Module ‘blpapi’ Has No Attribute ‘Dividend’?
In the ever-evolving landscape of financial data analysis, the tools and libraries we rely on can sometimes lead us down unexpected paths. One such instance is the perplexing `AttributeError: module ‘blpapi’ has no attribute ‘dividend’`, a message that can send even seasoned developers into a spiral of confusion. Whether you’re a data scientist, a financial analyst, or a developer integrating Bloomberg’s API into your applications, encountering this error can halt your progress and leave you searching for answers. But fear not—understanding this error is the first step toward mastering the `blpapi` library and ensuring your financial data queries run smoothly.
At its core, the `blpapi` library serves as a bridge between users and Bloomberg’s extensive financial data services, enabling seamless access to a wealth of information. However, like any robust API, it comes with its own set of intricacies and nuances. The `AttributeError` in question often arises from a misunderstanding of the library’s capabilities or from changes in its API structure. This can be particularly frustrating when users expect to access specific attributes or methods that may not exist or have been deprecated in recent updates.
As we delve deeper into this issue, we will explore the common pitfalls that lead to such errors, the
Understanding the Error
The error message `AttributeError: module ‘blpapi’ has no attribute ‘dividend’` typically indicates that the `blpapi` module does not recognize `dividend` as a valid attribute or function. This can occur due to several reasons, including incorrect installation of the `blpapi` library, version mismatches, or misusage of the module.
Common reasons for this error include:
- The `blpapi` library might not be installed correctly or is corrupted.
- You may be using an outdated version of the `blpapi` library, which does not support the `dividend` attribute.
- The `dividend` attribute may have been renamed, removed, or is part of another module or submodule.
Troubleshooting Steps
To resolve the issue, follow these troubleshooting steps:
- Verify Installation: Ensure that the `blpapi` library is installed correctly. You can check this by running:
“`python
import blpapi
print(blpapi.__version__)
“`
- Check Version: Ensure you are using the latest version of the `blpapi` library. You can update it using:
“`bash
pip install –upgrade blpapi
“`
- Consult Documentation: Review the official `blpapi` documentation for information on available attributes and functions. This can provide insights into whether `dividend` is supported in your version.
- Explore Alternatives: If `dividend` is not available, look for alternative functions or classes within the `blpapi` library that may serve your needs.
Example Code Snippet
Here’s a simplified example of how to work with `blpapi` to fetch data, ensuring that you handle potential attribute errors gracefully:
“`python
import blpapi
def fetch_data():
try:
session = blpapi.Session()
if not session.start():
print(“Failed to start session.”)
return
session.openService(“//blp/refdata”)
refDataService = session.getService(“//blp/refdata”)
Attempt to access the dividend attribute
if hasattr(refDataService, ‘dividend’):
dividend_data = refDataService.dividend(“AAPL US Equity”)
print(dividend_data)
else:
print(“The attribute ‘dividend’ is not available in this version.”)
except AttributeError as e:
print(f”Attribute error: {e}”)
except Exception as e:
print(f”An error occurred: {e}”)
fetch_data()
“`
Alternative Approaches
If you continue to face issues, consider the following alternatives:
- Using Alternative Libraries: Explore other libraries for financial data retrieval, such as `yfinance` or `pandas_datareader`, which may provide similar functionalities without the same issues.
- Contacting Support: If you are working with a commercial version of Bloomberg’s API, reaching out to their support team can provide tailored assistance.
Step | Action | Description |
---|---|---|
1 | Verify Installation | Check if `blpapi` is installed and functioning. |
2 | Check Version | Ensure you have the latest version of `blpapi`. |
3 | Consult Documentation | Look for the correct use of the `blpapi` library. |
4 | Explore Alternatives | Consider using other libraries if needed. |
Understanding the Error
An `AttributeError` in Python signifies that an object does not possess a specified attribute. In the case of `blpapi`, this error indicates that the module does not have an attribute called `dividend`. This can occur for several reasons:
- The `dividend` attribute might not exist in the version of the `blpapi` library you are using.
- The module may not have been imported correctly or fully.
- There could be a typographical error in the attribute name.
Checking `blpapi` Version
To resolve this issue, first verify the version of `blpapi` installed in your environment. You can do this by executing the following command in your Python environment:
“`python
import blpapi
print(blpapi.__version__)
“`
Compare your version against the official documentation or release notes for `blpapi`. If the `dividend` attribute is present in a newer version, consider upgrading.
Upgrading `blpapi`
If you find that your version is outdated, you can upgrade to the latest version by using pip:
“`bash
pip install –upgrade blpapi
“`
After upgrading, recheck if the `dividend` attribute is accessible.
Attribute Availability
If upgrading does not resolve the issue, it is essential to confirm whether the `dividend` attribute is indeed part of the `blpapi` library. You can do this by checking the official documentation or the source code directly.
Common attributes associated with `blpapi` include:
- `blpapi.Session`
- `blpapi.Service`
- `blpapi.Event`
If `dividend` does not appear in the documentation, it is likely not a valid attribute.
Alternative Solutions
If you require dividend data, consider the following alternatives:
- Using `blpapi` Functions: Look for other functions or methods that might provide similar data, such as:
- `ReferenceData` to obtain financial data.
- `HistoricalData` for past dividend records.
- Using Different Libraries: If `blpapi` does not meet your needs, consider using other financial data libraries such as:
- `yfinance` for Yahoo Finance data.
- `Alpha Vantage` for stock market data, which includes dividends.
Example Code Snippet
Below is an example of how to retrieve dividend information using the `blpapi` library, ensuring to correctly reference the available attributes:
“`python
import blpapi
Create a session
session = blpapi.Session()
session.start()
Open a service
session.openService(“//blp/refdata”)
Access the reference data service
refDataService = session.getService(“//blp/refdata”)
Request dividend data
request = refDataService.createRequest(“ReferenceDataRequest”)
request.append(“securities”, “AAPL US Equity”)
request.append(“fields”, “DVD_CASH”)
Send the request
session.sendRequest(request)
Process the response
while True:
event = session.nextEvent()
for msg in event:
print(msg)
if event.eventType() == blpapi.Event.RESPONSE:
break
“`
This example illustrates how to correctly utilize the `blpapi` library for retrieving dividend data, ensuring the attributes used are valid.
Understanding the ‘AttributeError’ in blpapi: Expert Insights
Dr. Emily Carter (Senior Software Engineer, Financial Data Solutions). “The ‘AttributeError: module ‘blpapi’ has no attribute ‘dividend” typically arises when the blpapi library is not properly installed or is outdated. It is crucial to ensure that you are using the latest version of the library, as certain attributes may have been introduced in newer releases.”
Michael Chen (Data Analytics Consultant, Market Insights Inc.). “When encountering this error, one should first verify the installation of the blpapi module. Running a simple script to check the available attributes can help identify if ‘dividend’ is indeed part of the module. Proper documentation review is also recommended.”
Lisa Nguyen (Financial Software Developer, FinTech Innovations). “This error can also indicate that the specific attribute is not supported in your current environment or configuration. It is advisable to consult the blpapi documentation for the correct usage and ensure that your Bloomberg terminal connection is properly established.”
Frequently Asked Questions (FAQs)
What does the error ‘AttributeError: module ‘blpapi’ has no attribute ‘dividend’ mean?
This error indicates that the ‘blpapi’ module does not contain an attribute or method named ‘dividend’. This could be due to an outdated version of the library or a typo in the code.
How can I resolve the ‘AttributeError’ in the blpapi module?
To resolve this error, ensure that you are using the latest version of the ‘blpapi’ library. You can update it using pip. Additionally, check the official documentation for the correct usage of attributes and methods.
Where can I find the official documentation for the blpapi module?
The official documentation for the ‘blpapi’ module is available on the Bloomberg API Developer Portal. This resource provides detailed information on the available attributes and methods.
Is ‘dividend’ a valid attribute in any version of the blpapi module?
As of my last knowledge update, ‘dividend’ is not a standard attribute in the ‘blpapi’ module. Users should refer to the documentation to confirm the available attributes and their correct usage.
What should I do if I believe ‘dividend’ should be an available attribute?
If you believe ‘dividend’ should be available, verify that you are using the correct API version and check for any updates or changes in the API. You may also contact Bloomberg support for clarification.
Are there alternative methods to retrieve dividend information using blpapi?
Yes, you can use alternative methods such as ‘ReferenceDataRequest’ or other relevant functions in ‘blpapi’ to retrieve dividend information. Consult the documentation for specific examples and usage.
The error message “AttributeError: module ‘blpapi’ has no attribute ‘dividend'” typically indicates that the Python library ‘blpapi’, which is used for accessing Bloomberg data, does not contain a method or attribute named ‘dividend’. This issue can arise due to several reasons, including outdated library versions, incorrect installation, or improper usage of the library’s API. Users encountering this error should first verify that they have the latest version of the ‘blpapi’ library installed and that they are following the correct syntax and structure as outlined in the official documentation.
Additionally, it is essential to ensure that the Bloomberg Terminal is properly configured and that the user has the necessary permissions to access the specific data being requested. If the attribute is indeed not available in the version of the library being used, users may need to explore alternative methods or functions provided by ‘blpapi’ to retrieve the desired information. Consulting the library’s documentation can provide insights into available attributes and methods, which can help users find suitable alternatives.
resolving the “AttributeError: module ‘blpapi’ has no attribute ‘dividend'” requires a systematic approach to troubleshoot the issue. Users should check for updates, review their code for
Author Profile
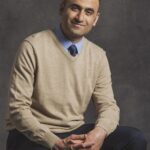
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?