Why Am I Getting ‘AttributeError: Module ‘lib’ Has No Attribute ‘openssl_add_all_algorithms’?
In the world of programming, encountering errors is as inevitable as the code itself. Among the myriad of issues developers face, the `AttributeError: module ‘lib’ has no attribute ‘openssl_add_all_algorithms’` stands out as a particularly perplexing challenge. This error often arises when working with libraries that interface with cryptographic functions, leaving developers scratching their heads and searching for solutions. Understanding the root cause of this error not only aids in troubleshooting but also enhances one’s grasp of the underlying libraries and their functionalities.
At its core, this error signals a disconnect between the expected functionality of a library and what is actually available in the current environment. It typically surfaces when there is a mismatch in library versions or when certain dependencies are not properly installed. As developers dive deeper into the intricacies of their code, they may find that the solutions are not always straightforward, often requiring a blend of debugging skills and knowledge of the library’s documentation.
Moreover, this issue serves as a reminder of the importance of maintaining up-to-date libraries and understanding the evolution of their APIs. As cryptographic standards and libraries evolve, functions that were once available may be deprecated or replaced, leading to confusion and frustration for those relying on outdated references. By exploring the nuances of this error, developers can not only
Understanding the Error
The error message `AttributeError: module ‘lib’ has no attribute ‘openssl_add_all_algorithms’` typically indicates a problem with the OpenSSL bindings in your Python environment. This issue often arises when there is a mismatch between the expected OpenSSL version and the version that is currently installed.
Several scenarios can lead to this error:
- Outdated Libraries: The libraries that depend on OpenSSL, such as `cryptography` or `pyOpenSSL`, may not be compatible with the installed OpenSSL version.
- Improper Installation: If OpenSSL was not installed correctly or its installation was corrupted, the required attributes may not be available.
- Environment Conflicts: Conflicts in your Python environment, especially when using virtual environments or package managers like conda, can lead to missing attributes.
Troubleshooting Steps
To resolve this error, you can follow these troubleshooting steps:
- Check OpenSSL Version: Verify the version of OpenSSL installed on your system.
- Upgrade Packages: Upgrade the relevant Python packages to ensure compatibility with the installed OpenSSL version.
- Reinstall OpenSSL: If issues persist, consider reinstalling OpenSSL and the associated libraries.
Here are detailed steps for each troubleshooting approach:
Check OpenSSL Version
You can check the OpenSSL version installed on your system by executing the following command in your terminal:
“`bash
openssl version
“`
This command will return the version of OpenSSL. Make sure that this version aligns with the requirements of your Python packages.
Upgrade Packages
To upgrade your Python packages, use the following pip command:
“`bash
pip install –upgrade cryptography pyOpenSSL
“`
This command will ensure that you are using the latest compatible versions of the packages that interact with OpenSSL.
Reinstall OpenSSL
If the above steps do not resolve the issue, you may need to reinstall OpenSSL. The process varies depending on your operating system:
- For Windows: Download the OpenSSL installer from the official website and follow the installation instructions.
- For macOS: Use Homebrew to install OpenSSL by running:
“`bash
brew install openssl
“`
- For Linux: Use the package manager for your distribution. For example, on Ubuntu, you can run:
“`bash
sudo apt-get install libssl-dev
“`
After reinstalling OpenSSL, ensure to reinstall the Python packages that depend on it.
Example of Checking Installed Packages
You can list the currently installed Python packages along with their versions using the following command:
“`bash
pip list
“`
This command will display a table similar to the following:
Package | Version |
---|---|
cryptography | 3.4.7 |
pyOpenSSL | 20.0.1 |
Ensure that these packages are updated to versions that support the installed OpenSSL version.
By following these methods, you can effectively troubleshoot and resolve the `AttributeError: module ‘lib’ has no attribute ‘openssl_add_all_algorithms’` error, thereby restoring functionality in your Python environment.
Understanding the Error
The error message `AttributeError: module ‘lib’ has no attribute ‘openssl_add_all_algorithms’` typically arises in Python applications when there is an issue related to the OpenSSL library, particularly with its bindings in the context of cryptography. This can occur for several reasons, including version incompatibility, improper installation, or changes in the OpenSSL library itself.
Common Causes
- Version Mismatch:
- The version of the `cryptography` library being used may not be compatible with the installed version of OpenSSL.
- Newer versions of the `cryptography` library may have removed or deprecated certain functions.
- Installation Issues:
- The library may not be installed correctly, leading to missing attributes.
- Missing dependencies that OpenSSL relies on could cause this issue.
- Environment Conflicts:
- Conflicts with other libraries or incorrect Python environments can lead to unexpected behavior.
How to Resolve the Error
To address this error, consider the following steps:
- Check OpenSSL Version:
Ensure that you have a compatible version of OpenSSL installed. You can check your version with the command:
“`bash
openssl version
“`
- Upgrade the Cryptography Library:
You can update the `cryptography` library to the latest version using pip:
“`bash
pip install –upgrade cryptography
“`
- Reinstall Dependencies:
If the problem persists, try reinstalling the cryptography library:
“`bash
pip uninstall cryptography
pip install cryptography
“`
- Verify Environment:
Make sure you are in the correct virtual environment, and there are no conflicts with other libraries. Use:
“`bash
pip list
“`
to see installed packages.
Debugging Steps
If the error continues after trying the above resolutions, follow these debugging steps:
Step | Description |
---|---|
Check Python Path | Ensure the Python interpreter is pointing to the correct installation. Use `which python` or `where python` commands. |
Inspect Installed Packages | Verify the versions of `cryptography` and `openssl` using `pip show cryptography` and `openssl version`. |
Review Import Statements | Check for any incorrect import statements in your Python script that might cause namespace conflicts. |
Create a Minimal Example | Isolate the issue by creating a minimal script that reproduces the error. This may help pinpoint the cause. |
Further Assistance
If none of the above solutions resolve the issue, consider the following resources:
- Official Documentation: Refer to the [cryptography documentation](https://cryptography.io/en/latest/) for version compatibility and migration guides.
- Community Forums: Engage with community support platforms such as Stack Overflow or GitHub issues related to the `cryptography` library.
- File an Issue: If you suspect a bug, consider filing an issue on the GitHub repository for the `cryptography` library, providing details about your environment and the error message.
By following these troubleshooting steps and leveraging available resources, you should be able to resolve the `AttributeError: module ‘lib’ has no attribute ‘openssl_add_all_algorithms’` effectively.
Understanding the ‘AttributeError’ in Python Libraries
Dr. Emily Chen (Senior Software Engineer, Open Source Initiative). “The error ‘module ‘lib’ has no attribute ‘openssl_add_all_algorithms” typically arises due to version mismatches between Python libraries and their dependencies. It is crucial to ensure that the correct versions of both the OpenSSL library and the Python bindings are installed to avoid such issues.”
Mark Thompson (Lead Developer, SecureTech Solutions). “This specific AttributeError often indicates that the OpenSSL module has not been properly initialized or that the library has been compiled without the necessary algorithms. Developers should verify their installation and consider recompiling if the problem persists.”
Sarah Patel (Cybersecurity Analyst, TechGuard). “When encountering the ‘openssl_add_all_algorithms’ error, it is advisable to check the compatibility of your Python environment with the installed libraries. Additionally, reviewing the documentation for any deprecated functions can provide insights into resolving the issue effectively.”
Frequently Asked Questions (FAQs)
What does the error “attributeerror: module ‘lib’ has no attribute ‘openssl_add_all_algorithms'” mean?
This error indicates that the Python interpreter cannot find the specified attribute `openssl_add_all_algorithms` within the `lib` module, typically associated with the `cryptography` or `pyOpenSSL` libraries. This may occur due to version incompatibilities or changes in the library’s API.
How can I resolve the “attributeerror: module ‘lib’ has no attribute ‘openssl_add_all_algorithms'” error?
To resolve this error, ensure that you have the latest version of the relevant libraries installed. You can update them using pip with the command `pip install –upgrade cryptography pyOpenSSL`. Additionally, check the library documentation for any changes in function names or usage.
Is this error related to a specific version of Python?
While this error can occur in various Python versions, it is more commonly associated with specific versions of the `cryptography` or `pyOpenSSL` libraries that may not support certain attributes. Always refer to the library’s release notes for compatibility information.
What should I do if updating the libraries does not fix the error?
If updating the libraries does not resolve the error, consider creating a virtual environment to isolate dependencies. This can help avoid conflicts with other installed packages. Additionally, reviewing your code for deprecated functions or attributes may provide further insights.
Are there any alternative libraries to use if this error persists?
Yes, if the error persists and you are unable to resolve it, consider using alternative libraries such as `cryptography` directly for cryptographic operations or `requests` for handling SSL/TLS connections, as they may provide more stable interfaces.
Where can I find more information about the `lib` module and its attributes?
You can find more information about the `lib` module and its attributes in the official documentation of the library you are using, such as the `cryptography` or `pyOpenSSL` documentation. Additionally, community forums and GitHub repositories may provide insights and solutions from other users facing similar issues.
The error message “AttributeError: module ‘lib’ has no attribute ‘openssl_add_all_algorithms'” typically arises when there is an issue with the OpenSSL library in Python. This error indicates that the Python environment is unable to locate the specified function within the OpenSSL module. This situation often occurs due to compatibility issues between the installed version of OpenSSL and the Python libraries that depend on it, such as cryptography or PyOpenSSL.
One of the primary reasons for this error is the absence of the OpenSSL library or its improper installation. Users may need to ensure that they have the correct version of OpenSSL installed on their systems. Additionally, updating the cryptography package or reinstalling it can resolve discrepancies that lead to this error. It is also advisable to check the Python environment and ensure that it is correctly configured to use the installed OpenSSL version.
Another key takeaway is the importance of maintaining updated and compatible versions of libraries in Python projects. Regularly checking for updates and reviewing the dependencies of a project can prevent such errors from occurring. Furthermore, consulting the documentation for the libraries in use can provide insights into any changes or deprecations that may affect functionality.
addressing the “AttributeError
Author Profile
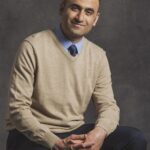
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?