Why Am I Getting ‘AttributeError: module ‘matplotlib.cm’ has no attribute ‘get_cmap’?’ and How to Fix It?
If you’ve ever delved into the world of data visualization using Python, chances are you’ve encountered the powerful library known as Matplotlib. This versatile tool allows users to create stunning visual representations of data, making complex information more accessible and understandable. However, as with any robust software, users may occasionally stumble upon cryptic error messages that can leave them scratching their heads. One such message that has puzzled many is the infamous `AttributeError: module ‘matplotlib.cm’ has no attribute ‘get_cmap’`. This error not only interrupts the flow of your coding but also raises questions about your understanding of the library’s functionalities and updates.
In this article, we will explore the nuances of this specific error, shedding light on the underlying reasons for its occurrence. We’ll delve into the intricacies of Matplotlib’s colormap module and how changes in library versions can lead to unexpected issues. By understanding the context and potential solutions, you’ll be better equipped to navigate the challenges of data visualization and enhance your coding experience. Whether you are a seasoned data scientist or a novice programmer, this discussion will provide valuable insights to help you troubleshoot and refine your skills in using Matplotlib effectively.
Join us as we unravel the mystery behind this common error, equipping you with the knowledge to
Understanding the Error
The error message `AttributeError: module ‘matplotlib.cm’ has no attribute ‘get_cmap’` typically indicates that the code is trying to access a method or attribute that does not exist in the `matplotlib.cm` module. This can arise from a variety of issues, including changes in the library’s API, version mismatches, or incorrect usage of the functions.
Several common reasons for this error include:
- Incorrect Matplotlib Version: The method `get_cmap` may not be available in the version of Matplotlib you are using. Make sure you are using a version that supports this function.
- Improper Import Statements: Ensure that the import statements are correct and that you are not accidentally overriding the `matplotlib.cm` module.
- Typographical Errors: A simple typo in the method name can lead to this error, so double-check the spelling and casing.
Resolving the Error
To resolve the `AttributeError`, consider the following steps:
- Check Matplotlib Version: Ensure you are using a compatible version of Matplotlib. You can check your version by running:
“`python
import matplotlib
print(matplotlib.__version__)
“`
- Upgrade or Downgrade Matplotlib: If your version is outdated or incompatible, you might need to upgrade or downgrade it. Use the following commands:
“`bash
Upgrade
pip install –upgrade matplotlib
Downgrade (example to a specific version)
pip install matplotlib==3.4.3
“`
- Correct Imports: Make sure to import the colormap correctly. Instead of directly using `matplotlib.cm`, you can use:
“`python
from matplotlib import cm
“`
- Use Alternative Methods: If `get_cmap` is not available, consider using alternative methods such as `cm.get_cmap()` directly from the `cm` module.
Alternative Solutions
If the above methods do not resolve the issue, here are some alternative approaches you can take:
- Check Documentation: Verify the official Matplotlib documentation for the correct usage of colormaps. This can help ensure that you are utilizing the library as intended.
- Consult Community Forums: Platforms like Stack Overflow or GitHub issues for Matplotlib can provide insights from other users who may have encountered similar problems.
- Fallback to Basic Colormaps: If specific colormaps are not essential, you can use the basic colormaps provided by Matplotlib without needing `get_cmap`.
Colormap Name | Description |
---|---|
viridis | A perceptually-uniform colormap ideal for displaying information. |
plasma | A vibrant colormap that is suitable for many types of data visualizations. |
inferno | A colormap designed for better visibility in dark environments. |
magma | A colormap that offers a smooth gradient from dark to light. |
cividis | A colorblind-friendly colormap, useful for accessibility. |
By addressing the underlying causes and implementing these solutions, you should be able to resolve the `AttributeError` and effectively utilize colormaps in your Matplotlib visualizations.
Understanding the Error
The error message `AttributeError: module ‘matplotlib.cm’ has no attribute ‘get_cmap’` typically arises when there’s a mismatch between the expected API of the `matplotlib` library and the version installed in your environment. This issue can occur for several reasons, including:
- Version Incompatibility: The function `get_cmap` may not exist in earlier versions of `matplotlib`. The most common version where this function is available is `matplotlib 1.5` and later.
- Import Errors: If the library is not imported correctly, or if there is a naming conflict with a local file named `matplotlib.py`, this error may be triggered.
Troubleshooting Steps
To resolve this error, follow these steps:
- Check Matplotlib Version:
- Open a Python environment and run:
“`python
import matplotlib
print(matplotlib.__version__)
“`
- Ensure the version is `1.5` or newer.
- Update Matplotlib:
- If your version is outdated, update it using pip:
“`bash
pip install –upgrade matplotlib
“`
- Verify Imports:
- Ensure you’re importing `matplotlib.cm` correctly:
“`python
import matplotlib.cm as cm
cmap = cm.get_cmap(‘viridis’)
“`
- Check for Local Files:
- Ensure there is no local file named `matplotlib.py` in the same directory as your script, as this can shadow the actual library.
- Explore Alternative Methods:
- If you are using a version that does not support `get_cmap`, consider using the following alternative:
“`python
from matplotlib import colormaps
cmap = colormaps[‘viridis’]
“`
Common Solutions
Here are some common solutions that users have found helpful:
Solution | Description |
---|---|
Upgrade Matplotlib | Ensure you are using a compatible version. |
Correct Import Statements | Verify that imports are done correctly without conflicts. |
Check for Local File Conflicts | Ensure there are no naming conflicts with local scripts. |
Further Considerations
If the problem persists even after following the above steps, consider the following:
- Reinstall Matplotlib: Sometimes a clean install can resolve underlying issues:
“`bash
pip uninstall matplotlib
pip install matplotlib
“`
- Virtual Environments: Use a virtual environment to isolate dependencies and avoid conflicts:
“`bash
python -m venv myenv
source myenv/bin/activate On Windows use `myenv\Scripts\activate`
pip install matplotlib
“`
- Consult Documentation: Always refer to the [official Matplotlib documentation](https://matplotlib.org/stable/users/index.html) for the latest updates and best practices regarding functions and attributes.
By following these guidelines, you should be able to resolve the `AttributeError` related to `matplotlib.cm` effectively.
Understanding the AttributeError in Matplotlib
Dr. Emily Chen (Data Visualization Specialist, Graphical Insights Inc.). “The error ‘attributeerror: module ‘matplotlib.cm’ has no attribute ‘get_cmap” typically arises when there is a version mismatch in the Matplotlib library. Users should ensure they are using a compatible version that supports the ‘get_cmap’ function, as it may have been deprecated or altered in recent updates.”
Michael Tran (Senior Software Engineer, Data Science Solutions). “When encountering this error, it is crucial to verify the import statements in your code. If you are using a custom module or have redefined ‘cm’, it could lead to this issue. Always check for namespace conflicts that might obscure the default Matplotlib functionality.”
Lisa Patel (Python Programming Instructor, Tech Academy). “This error can also occur if the Matplotlib library is not correctly installed. I recommend reinstalling the library using pip and ensuring that all dependencies are satisfied. A clean environment can often resolve such attribute errors effectively.”
Frequently Asked Questions (FAQs)
What does the error “AttributeError: module ‘matplotlib.cm’ has no attribute ‘get_cmap'” mean?
This error indicates that the Python interpreter cannot find the `get_cmap` function within the `matplotlib.cm` module. This can occur due to version discrepancies or incorrect usage of the library.
How can I resolve the “AttributeError: module ‘matplotlib.cm’ has no attribute ‘get_cmap'”?
To resolve this error, ensure that you are using a compatible version of Matplotlib. Update Matplotlib using pip with the command `pip install –upgrade matplotlib`, and check the documentation for the correct usage of colormap functions.
Is `get_cmap` deprecated in recent versions of Matplotlib?
No, `get_cmap` is not deprecated, but its usage may differ based on the version of Matplotlib. In some versions, you can use `plt.get_cmap()` instead of directly accessing it from `matplotlib.cm`.
What is the correct way to access colormaps in Matplotlib?
The recommended way to access colormaps is by using `matplotlib.pyplot.get_cmap()` or `matplotlib.cm.get_cmap()`. Ensure you import the necessary modules correctly.
Can I use an alternative method to get colormaps in Matplotlib?
Yes, you can directly access colormaps via `matplotlib.cm` by using `matplotlib.cm.
What should I check if I still encounter the error after updating Matplotlib?
If the error persists, verify that there are no conflicting installations of Matplotlib, check your import statements for correctness, and ensure that your Python environment is properly configured.
The error message “AttributeError: module ‘matplotlib.cm’ has no attribute ‘get_cmap'” typically indicates that the user is attempting to access a function or attribute that is not available in the version of the Matplotlib library being used. This can arise from various reasons, such as using an outdated version of Matplotlib, incorrect import statements, or changes in the library’s API in newer releases. Users should ensure that they are using the correct syntax and that their Matplotlib installation is up to date to avoid such issues.
One key takeaway is the importance of checking the version of Matplotlib installed in your environment. The `get_cmap` function is indeed a valid method in Matplotlib, but if the library is not properly installed or is an older version, the function may not be accessible. Users can update Matplotlib using package managers like pip or conda to ensure they have the latest features and bug fixes.
Additionally, it is essential to verify the import statements in your code. Properly importing the required modules is crucial to accessing their functionalities. Instead of directly accessing `get_cmap` from `matplotlib.cm`, users should ensure they are using it correctly, possibly by importing it directly or checking the documentation for any
Author Profile
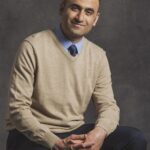
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?