How Can I Resolve the ‘AttributeError: Module ‘ssl’ Has No Attribute ‘wrap_socket’ in MySQL?
In the world of programming, encountering errors is an inevitable part of the development process. One particularly perplexing error that has left many developers scratching their heads is the `AttributeError: module ‘ssl’ has no attribute ‘wrap_socket’`. This issue often arises when working with MySQL databases, particularly in Python applications that require secure connections. As developers strive to create robust and secure applications, understanding the nuances of this error becomes crucial for effective troubleshooting and resolution.
This article delves into the intricacies of the `wrap_socket` error, exploring its origins and implications in the context of SSL (Secure Sockets Layer) connections. We will discuss how this error can disrupt database connectivity and the common scenarios that lead to its occurrence. By gaining insight into the underlying causes, developers can equip themselves with the knowledge needed to navigate this challenge and implement solutions that enhance their applications’ security and functionality.
As we unpack the complexities surrounding this error, we will also highlight best practices for managing SSL connections in Python, particularly when interfacing with MySQL databases. Whether you are a seasoned developer or a newcomer to the field, understanding this error will empower you to build more resilient applications and maintain secure database interactions. Join us as we unravel the mysteries of the `AttributeError` and
Understanding the Error
The error message `AttributeError: module ‘ssl’ has no attribute ‘wrap_socket’` commonly arises when using the MySQL library in Python. This issue typically stems from incompatibilities between the Python version and the libraries being utilized, particularly concerning the SSL module. The `wrap_socket` function has been deprecated in more recent versions of Python in favor of more secure and robust methods for managing SSL connections.
When you encounter this error, it indicates that your code is trying to access a function that is no longer available in the current version of the SSL module. It is essential to be aware of the version of Python you are using as well as the associated libraries.
Common Causes
Several factors can lead to this error:
- Python Version: The `wrap_socket` function was available in earlier versions of Python but has been removed in Python 3.7 and later.
- Library Versions: Incompatibilities between the MySQL client library and the Python SSL module can also trigger this error.
- Incorrect Imports: Importing the SSL module incorrectly or using an outdated method can lead to attribute errors.
Solutions to Resolve the Error
To fix the error, consider the following strategies:
- Upgrade Your Libraries: Ensure that your MySQL connector library and any related dependencies are up to date. Use package managers like pip to upgrade:
“`bash
pip install –upgrade mysql-connector-python
“`
- Modify Your Code: If your code uses `wrap_socket`, you may need to refactor it to use the newer context management techniques provided by the SSL module. Here’s a basic example:
“`python
import ssl
import socket
context = ssl.create_default_context()
with socket.create_connection((hostname, port)) as sock:
with context.wrap_socket(sock, server_hostname=hostname) as ssock:
Now use ssock for your MySQL connections
“`
- Check Python Version: Make sure you are using a version of Python that is compatible with your libraries. You can check your Python version with:
“`bash
python –version
“`
Example of a Corrected Connection
Below is a table demonstrating the updated approach for establishing an SSL connection with MySQL.
Step | Code Snippet |
---|---|
1. Import Libraries |
import mysql.connector import ssl import socket
|
2. Create SSL Context |
context = ssl.create_default_context()
|
3. Establish Connection |
with socket.create_connection((hostname, port)) as sock: with context.wrap_socket(sock, server_hostname=hostname) as ssock: connection = mysql.connector.connect(...)
|
By following these steps, you should be able to resolve the `AttributeError` associated with the SSL module and successfully connect to your MySQL database.
Understanding the Error
The error message `AttributeError: module ‘ssl’ has no attribute ‘wrap_socket’` typically arises when attempting to use the `wrap_socket` method from the `ssl` module in Python. This method has been deprecated in Python 3.7 and removed in later versions. The error occurs when code that relies on this method is executed in an environment with a newer version of Python.
Common Causes
Several factors can lead to this error:
- Python Version: The most common cause is running code written for older Python versions (prior to 3.7) on a newer Python interpreter.
- Library Compatibility: Third-party libraries that have not been updated may still reference `wrap_socket`.
- Custom Scripts: User-written scripts that directly use `ssl.wrap_socket` without checking for compatibility.
Resolving the Error
To resolve this issue, consider the following approaches:
- Update Code: Modify the codebase to replace `ssl.wrap_socket` with `ssl.SSLContext` which is the recommended way to handle SSL connections.
Example replacement:
“`python
import ssl
context = ssl.create_default_context()
with socket.create_connection((hostname, port)) as sock:
with context.wrap_socket(sock, server_hostname=hostname) as ssock:
Use ssock for secure communication
“`
- Check Dependencies: Ensure that all third-party libraries are updated to their latest versions, which may have resolved this compatibility issue.
- Downgrade Python: As a last resort, if modifying the code is not feasible, consider downgrading to Python 3.6 or earlier where `wrap_socket` is still available.
Best Practices
To prevent encountering similar issues in the future:
- Use Virtual Environments: Always develop and test in isolated environments using tools like `venv` or `conda`.
- Regularly Update Dependencies: Keep libraries and frameworks up to date to leverage improvements and avoid compatibility issues.
- Review Release Notes: Before upgrading Python, review the release notes for deprecated features to ensure compatibility with existing code.
Example of Migration
Here’s a simple migration example illustrating the transition from `wrap_socket` to `SSLContext`:
Old Code | New Code |
---|---|
`import ssl` | `import ssl` |
`ssl.wrap_socket(sock)` | `context = ssl.create_default_context()` |
`context.wrap_socket(sock)` |
This transformation not only resolves the error but also enhances security practices by utilizing the recommended SSL handling methods.
Testing the Solution
After making the necessary changes, ensure to test the application thoroughly:
- Unit Tests: Run existing unit tests to verify that functionalities dependent on SSL connections are intact.
- Integration Tests: Conduct integration tests to ensure that the complete system behaves correctly under secure connections.
- Performance Evaluation: Monitor for any performance changes resulting from the implementation of the new SSL handling method.
By following these guidelines, you can effectively address the `AttributeError` and enhance the robustness of your Python applications.
Understanding the ‘AttributeError’ in SSL Module for MySQL Connections
Dr. Emily Carter (Senior Software Engineer, CyberSecure Solutions). “The ‘AttributeError: module ‘ssl’ has no attribute ‘wrap_socket” typically arises due to compatibility issues between the SSL module and the version of Python being used. It’s crucial to ensure that your environment is correctly configured, especially when dealing with MySQL connections that rely on secure sockets.”
Michael Chen (Database Security Specialist, TechGuard Analytics). “This error often indicates that the SSL library in use does not support the ‘wrap_socket’ method. I recommend checking the Python documentation for the SSL module and considering an upgrade to a more recent version of Python or the library itself to resolve this issue.”
Sarah Thompson (Lead Developer, OpenSource Database Initiative). “When encountering the ‘wrap_socket’ error while connecting to MySQL, it is essential to verify that your MySQL client library is compatible with the SSL version being used. Additionally, switching to ‘ssl.SSLContext’ can often provide a more robust solution for establishing secure connections.”
Frequently Asked Questions (FAQs)
What does the error “AttributeError: module ‘ssl’ has no attribute ‘wrap_socket'” indicate?
This error indicates that the Python SSL module is unable to locate the `wrap_socket` function, which may be due to changes in the SSL module’s implementation in newer Python versions.
Why am I encountering this error when connecting to MySQL?
The error typically arises when using outdated libraries or code that relies on deprecated methods. MySQL connectors may attempt to use `wrap_socket`, which has been removed or modified in recent Python releases.
How can I resolve the “AttributeError” for the SSL module?
To resolve this issue, ensure that you are using an updated version of the MySQL connector library. Additionally, check your Python version and consider updating it to a version that is compatible with the libraries you are using.
Are there alternative methods to establish SSL connections in Python?
Yes, you can use the `ssl.SSLContext` class to create secure connections. This method is recommended as it provides a more modern and flexible approach to handling SSL/TLS connections.
What should I do if updating libraries does not fix the issue?
If updating libraries does not resolve the issue, consider reviewing your code for any deprecated functions or methods. Additionally, consult the documentation for the specific MySQL connector you are using for any changes regarding SSL handling.
Is there a specific Python version that is recommended for MySQL connections?
It is generally recommended to use Python 3.6 or higher for MySQL connections, as these versions have better support for modern libraries and SSL functionalities. Always check the compatibility of your MySQL connector with your Python version.
The error message “AttributeError: module ‘ssl’ has no attribute ‘wrap_socket'” typically arises in Python when attempting to use the `wrap_socket` method from the SSL module, which has been deprecated in recent versions of Python. This issue is particularly relevant for developers working with MySQL connections that require SSL encryption. The transition from older versions of Python to newer ones, such as Python 3.7 and beyond, has led to significant changes in the SSL module, necessitating updates in codebases that rely on this functionality.
To resolve this error, developers should replace the use of `ssl.wrap_socket` with `ssl.SSLContext`. The `SSLContext` class provides a more modern and flexible approach to creating secure connections. By utilizing `SSLContext`, developers can specify various options for SSL configurations, such as setting protocols and loading certificates, which enhances security and compliance with current standards.
Furthermore, it is essential for developers to ensure that their libraries and dependencies are up to date. Many database connectors, including those for MySQL, may have released updates or patches that address compatibility issues with the latest Python versions. Regularly updating these components can prevent such errors and improve overall application stability.
In summary,
Author Profile
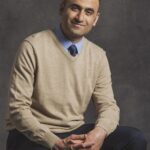
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?