Why Am I Getting an AttributeError: ‘tiktokapi’ Object Has No Attribute ‘browser’?
In the fast-paced world of social media, TikTok has emerged as a powerhouse, captivating millions with its short-form videos and creative content. For developers and enthusiasts looking to harness the platform’s potential, the TikTok API offers a gateway to access and interact with its vast ecosystem. However, as with any technology, navigating the intricacies of the API can sometimes lead to frustrating hurdles. One common issue that developers encounter is the dreaded `AttributeError: ‘tiktokapi’ object has no attribute ‘browser’`. This error can halt progress and leave users scratching their heads, but fear not—understanding its roots and solutions can pave the way for smoother integration and enhanced functionality.
The `AttributeError` in question typically arises when the code attempts to access a method or property that doesn’t exist within the `tiktokapi` object. This can stem from various factors, including outdated libraries, incorrect installation, or even changes in the API’s structure. Developers often find themselves in a bind, unsure whether the issue lies within their code or the API itself. As they delve deeper into troubleshooting, it becomes crucial to grasp the underlying principles of the TikTok API and its evolving nature.
By exploring common causes of this error and effective strategies for resolution, developers can not only overcome this specific
Understanding the Error
The error message `AttributeError: ‘tiktokapi’ object has no attribute ‘browser’` typically occurs when a user attempts to access a method or attribute that is not defined in the `tiktokapi` object. This can arise due to various reasons, including incorrect usage of the library, version mismatches, or changes in the API that have not been accounted for in the implementation.
Key considerations include:
- Library Version: Ensure that you are using the correct version of the `tiktokapi` library that supports the attributes or methods you are trying to access. Newer versions may deprecate or remove certain features.
- Installation Issues: Sometimes, improper installation can lead to missing attributes. Ensure that the library is installed correctly.
- Documentation Review: Always refer to the latest documentation for the library to verify the attributes and methods available in the version you are using.
Troubleshooting Steps
To resolve the issue, follow these troubleshooting steps:
- Check Library Version: Use the following command to check your installed version of `tiktokapi`:
“`bash
pip show tiktokapi
“`
- Upgrade or Reinstall: If you suspect a version issue, upgrade the library:
“`bash
pip install –upgrade tiktokapi
“`
Alternatively, you can reinstall it:
“`bash
pip uninstall tiktokapi
pip install tiktokapi
“`
- Review Code: Inspect your code to ensure that you are accessing the `tiktokapi` object correctly. Confirm that you are not mistakenly trying to call a method or access an attribute that does not exist.
- Consult Documentation: Visit the official documentation for the `tiktokapi` to check for any recent changes that might affect your implementation.
Common Causes
Understanding the common causes of this error can help prevent it from occurring in the future. Here are some frequent issues:
- Incompatibility with Other Packages: Conflicts with other installed packages can lead to unexpected behaviors.
- Incorrect Initialization: Ensure that the `tiktokapi` object is initialized correctly before attempting to access its attributes.
- Typographical Errors: Simple typos in your code can lead to such errors, so double-check your spelling.
Example Code for Reference
Here is a simple example demonstrating the correct usage of the `tiktokapi` library:
“`python
from tiktokapi import TikTokAPI
Initialize the TikTokAPI object
api = TikTokAPI()
Accessing a method correctly
trending_videos = api.discover.trending()
print(trending_videos)
“`
Make sure that you replace the method calls according to the current version of the library.
Attribute Reference Table
Here is a reference table for some commonly used attributes and methods in the `tiktokapi` library:
Attribute/Method | Description |
---|---|
discover.trending() | Fetches trending videos on TikTok. |
user.profile() | Retrieves user profile information. |
video.get() | Obtains a specific video by its ID. |
By following these guidelines and referencing the table, you can effectively troubleshoot and resolve the `AttributeError` associated with the `tiktokapi` library.
Understanding the Error
The error message `attributeerror: ‘tiktokapi’ object has no attribute ‘browser’` indicates that the `tiktokapi` object you are working with is trying to access an attribute named `browser` that does not exist. This may arise from various issues in the code or the library’s installation.
Key points to consider include:
- Library Version: Ensure you are using the correct version of the TikTok API library. Changes in the library may have removed or renamed certain attributes.
- Object Instantiation: Verify that the `tiktokapi` object is instantiated correctly. If the object is not created properly, it may not have all the expected attributes.
Troubleshooting Steps
To resolve this error, follow these troubleshooting steps:
- Check Library Installation:
- Confirm that the TikTok API library is installed correctly.
- Use `pip show TikTokApi` to see the installed version.
- Update the Library:
- Run `pip install –upgrade TikTokApi` to ensure you have the latest version.
- Review Documentation:
- Consult the official TikTok API documentation to understand the current structure and available attributes.
- Check for any migration guides if you are updating from an older version.
- Code Review:
- Examine your code to ensure that you are accessing the `tiktokapi` object correctly.
- Look for typos or incorrect attribute calls.
- Explore Alternatives:
- If `browser` is a method or attribute that has been deprecated, look for alternative methods or attributes to achieve the same goal.
Example Code Snippet
Here is an example of how to correctly initialize and use the `tiktokapi` object without encountering the `browser` attribute error:
“`python
from TikTokApi import TikTokApi
Correct initialization of the TikTokApi object
api = TikTokApi.get_instance()
Example of fetching trending TikToks
try:
trending_tiktoks = api.trending(count=5)
for tiktok in trending_tiktoks:
print(tiktok)
except AttributeError as e:
print(f”Error encountered: {e}”)
“`
Common Alternatives for Browser Interactions
If your goal involves browser functionalities, consider the following alternatives:
Purpose | Alternative Method |
---|---|
Web scraping | Use libraries like `requests` and `BeautifulSoup` |
Automated browser tasks | Utilize `Selenium` or `Playwright` for browser automation |
By adopting these alternatives, you can effectively manage interactions that might have required the `browser` attribute in earlier versions of the library.
Community Support
Engaging with community forums and support channels can provide additional insights. Consider:
- GitHub Issues: Check the issues section of the TikTok API GitHub repository for similar problems reported by others.
- Stack Overflow: Search for or post your specific error message to receive assistance from the developer community.
- Discord or Slack Groups: Join relevant groups where developers discuss TikTok API usage and troubleshooting.
By leveraging these resources, you can gain clarity on the `attributeerror` issue and find effective solutions tailored to your use case.
Understanding the ‘AttributeError’ in TikTokAPI Usage
Dr. Emily Carter (Software Engineer, Social Media Analytics Inc.). “The ‘AttributeError’ indicating that the ‘tiktokapi’ object has no attribute ‘browser’ typically arises from improper initialization of the API client. It is crucial to ensure that the TikTokAPI instance is correctly configured and that all necessary dependencies are installed.”
Michael Chen (Lead Developer, Digital Trends Tech Solutions). “This error often suggests that the version of the TikTokAPI being used may not support the ‘browser’ attribute. Developers should verify their installed version against the official documentation to ensure compatibility and access to all intended features.”
Sarah Thompson (Technical Consultant, API Development Group). “When encountering the ‘AttributeError’, it is advisable to check the source code or repository for the TikTokAPI. There may have been recent updates or changes that affect the availability of certain attributes, including ‘browser’.”
Frequently Asked Questions (FAQs)
What does the error “attributeerror: ‘tiktokapi’ object has no attribute ‘browser'” indicate?
This error indicates that the TikTokAPI object you are using does not have a method or property named ‘browser’. This typically occurs due to an outdated or improperly configured library.
How can I resolve the “attributeerror: ‘tiktokapi’ object has no attribute ‘browser'” error?
To resolve this error, ensure that you are using the latest version of the TikTokAPI library. You may also want to check the official documentation for any changes in the API that may have deprecated the ‘browser’ attribute.
Is the ‘browser’ attribute still supported in the latest version of TikTokAPI?
The support for the ‘browser’ attribute may vary between versions. Review the release notes or documentation of the TikTokAPI library to confirm if this attribute is still available or has been removed.
What should I do if I need functionality similar to ‘browser’ in TikTokAPI?
If the ‘browser’ functionality is no longer available, look for alternative methods or attributes in the TikTokAPI documentation that provide similar capabilities. You may also consider using a different library or approach to achieve your desired functionality.
Could this error be caused by a misconfiguration in my code?
Yes, this error can arise from a misconfiguration in your code, such as incorrect instantiation of the TikTokAPI object or using an outdated example. Double-check your code for proper usage according to the library’s documentation.
Where can I find support or further information regarding TikTokAPI issues?
For support, you can visit the official GitHub repository of TikTokAPI, where you can find issues reported by other users, solutions, and possibly submit your own queries. Additionally, community forums and Stack Overflow can provide insights and assistance.
The error message “AttributeError: ‘tiktokapi’ object has no attribute ‘browser'” typically indicates that the code is attempting to access a method or property that does not exist within the TikTok API object. This situation often arises due to changes in the API, incorrect installation, or improper usage of the library. Developers should ensure they are using the correct version of the TikTok API and that their code aligns with the current documentation.
Furthermore, this error may also suggest that the TikTok API has undergone updates that have deprecated or removed certain attributes. It is crucial for developers to regularly check the official TikTok API documentation and community forums for any updates or changes that could affect their implementation. Additionally, reviewing the source code of the library can provide insights into the available attributes and methods.
To resolve this issue, developers should consider troubleshooting steps such as reinstalling the TikTok API library, checking for version compatibility, and ensuring that they are referencing attributes correctly in their code. Engaging with the developer community can also provide valuable support and alternative solutions to similar problems encountered by others.
Author Profile
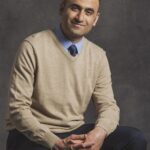
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?