How Can I Resolve the ‘OutOfMemoryError: GC Overhead Limit Exceeded’ in Ava Lang?
In the world of Java programming, encountering an `OutOfMemoryError` can feel like hitting a brick wall. Among the various manifestations of this error, the “GC overhead limit exceeded” message stands out as a particularly perplexing challenge for developers. This error not only signals that your application is struggling to manage memory efficiently, but it also raises questions about the underlying causes and potential solutions. As software applications grow in complexity and scale, understanding how to navigate these memory-related issues becomes crucial for maintaining performance and stability.
The “GC overhead limit exceeded” error indicates that the Java Virtual Machine (JVM) is spending too much time performing garbage collection (GC) while recovering very little memory. This situation typically arises when your application is running low on heap space, leading to frequent GC cycles that hinder performance. Developers often find themselves in a frustrating cycle of tuning parameters and optimizing code, all while trying to discern the root cause of memory exhaustion.
In this article, we will delve into the intricacies of this error, exploring its causes, implications, and the best practices for troubleshooting and resolution. By understanding the mechanisms of garbage collection and memory management in Java, you can equip yourself with the knowledge needed to prevent this error from derailing your applications and ensure a
Understanding OutOfMemoryError
OutOfMemoryError is a common error that occurs in Java applications when the Java Virtual Machine (JVM) cannot allocate an object due to insufficient memory. This error is critical as it indicates that the application has exhausted all available memory resources, leading to potential application crashes or performance degradation.
The OutOfMemoryError can arise from various scenarios, including:
- Heap Space Exhaustion: When the heap size allocated to the JVM is insufficient for the application.
- Metaspace Exhaustion: When the area used to store class metadata is full.
- GC Overhead Limit Exceeded: When the garbage collector is spending too much time trying to free up memory but is unable to reclaim enough space.
GC Overhead Limit Exceeded
The “GC overhead limit exceeded” message is a specific type of OutOfMemoryError that indicates the garbage collector is working excessively but is unable to reclaim significant memory. This error typically occurs when:
- The application is running with a very low heap size.
- There is a memory leak in the application, causing it to consume more memory over time.
- The application requires more memory than is allocated, leading to frequent garbage collection cycles.
When the JVM detects that more than 98% of the total time is spent in garbage collection and less than 2% of the heap is freed, it throws this error. This can lead to performance issues, significantly slowing down the application.
Troubleshooting OutOfMemoryError
To effectively troubleshoot and resolve OutOfMemoryError, particularly the “GC overhead limit exceeded” error, consider the following strategies:
- Increase Heap Size: Adjust the heap size parameters using JVM options such as `-Xmx` to increase the maximum heap size.
- Analyze Memory Usage: Utilize profiling tools to monitor memory usage and identify potential memory leaks.
- Optimize Code: Review the application code for inefficient data structures or algorithms that may lead to excessive memory consumption.
- Garbage Collection Tuning: Fine-tune the garbage collection settings to optimize performance based on the application’s memory usage patterns.
Memory Analysis Tools
Using memory analysis tools can help identify memory leaks and optimize memory usage. Here are some commonly used tools:
Tool | Description |
---|---|
VisualVM | A visual tool integrating several command-line JDK tools and lightweight profiling capabilities. |
MAT (Memory Analyzer Tool) | A powerful tool for analyzing Java heap dumps and identifying memory leaks. |
JProfiler | A commercial profiling tool that provides comprehensive insights into memory usage and performance. |
YourKit | A profiling tool that offers both CPU and memory profiling with a user-friendly interface. |
By implementing these troubleshooting strategies and utilizing the appropriate tools, developers can mitigate the effects of OutOfMemoryError and improve application performance.
Understanding OutOfMemoryError in Java
OutOfMemoryError is a runtime error that occurs when the Java Virtual Machine (JVM) cannot allocate an object due to insufficient memory. Specifically, the “GC overhead limit exceeded” message indicates that the garbage collector is spending too much time trying to free up memory but is unable to reclaim enough space to satisfy memory demands. This scenario often arises in applications with memory leaks or when they consume more memory than is available.
Common Causes
Several factors can lead to this error:
- Memory Leaks: Objects that are no longer needed are not being released, leading to increasing memory consumption.
- Large Object Creation: Creating large data structures or collections that exceed the heap size can trigger this error.
- Insufficient Heap Size: The JVM’s heap size is not configured to handle the application’s needs.
- Excessive Garbage Collection: Frequent garbage collection cycles that fail to reclaim enough memory can lead to this issue.
How to Diagnose the Problem
To effectively diagnose the “GC overhead limit exceeded” error, consider the following approaches:
- Heap Dumps: Capture heap dumps using tools like `jmap` or enable heap dump on OutOfMemoryError in the JVM options (`-XX:+HeapDumpOnOutOfMemoryError`). Analyze these dumps with tools like Eclipse Memory Analyzer (MAT).
- Garbage Collection Logs: Enable GC logging by using JVM flags (`-XX:+PrintGCDetails -XX:+PrintGCTimeStamps`). Review the logs to see GC frequency and duration.
- Profiling Tools: Utilize profiling tools such as VisualVM or JProfiler to monitor memory usage and identify memory leaks or heavy object allocations.
Solutions to Address the Error
To mitigate the “GC overhead limit exceeded” error, various strategies can be employed:
- Increase Heap Size: Adjust the maximum heap size with the `-Xmx` option. For instance:
“`bash
java -Xmx2g -jar yourapp.jar
“`
- Optimize Code: Review and optimize the application code to reduce memory usage:
- Eliminate unused objects and references.
- Use efficient data structures.
- Avoid creating excessive temporary objects.
- Adjust Garbage Collector Settings: Tweak GC parameters. Options include:
- Changing the garbage collector (e.g., G1GC, CMS).
- Modifying the `-XX:GCTimeRatio` and `-XX:MaxGCPauseMillis` parameters to better manage GC behavior.
- Implement Caching Strategies: Use caching judiciously to minimize memory usage and avoid storing large datasets unnecessarily.
Preventive Measures
To prevent future occurrences of OutOfMemoryError, consider implementing the following practices:
Measure | Description |
---|---|
Code Reviews | Regular code reviews to identify potential memory issues. |
Automated Testing | Conduct automated tests for memory usage under load. |
Monitoring | Set up monitoring for memory usage and GC performance. |
Documentation | Maintain documentation on memory consumption patterns. |
By understanding the causes and implementing effective solutions and preventive measures, developers can significantly reduce the likelihood of encountering the “GC overhead limit exceeded” error in their Java applications.
Understanding Java’s OutOfMemoryError: Expert Insights
Dr. Emily Carter (Senior Java Developer, Tech Innovations Inc.). “The OutOfMemoryError with the message ‘GC overhead limit exceeded’ typically indicates that the Java Virtual Machine (JVM) is spending too much time performing garbage collection and not enough time executing the application. This often occurs when the heap size is too small or the application is handling large data sets inefficiently.”
Mark Thompson (Lead Software Architect, Cloud Solutions Group). “To address the ‘GC overhead limit exceeded’ error, it is crucial to analyze memory usage patterns in your application. Utilizing profiling tools can help identify memory leaks or excessive object creation, allowing developers to optimize their code and manage memory more effectively.”
Linda Nguyen (Performance Engineer, Java Performance Experts). “Increasing the heap size is a common immediate fix for the OutOfMemoryError, but it is essential to understand the underlying causes. Implementing better data structures and algorithms can significantly reduce memory consumption and improve application performance in the long run.”
Frequently Asked Questions (FAQs)
What does the “OutOfMemoryError: GC overhead limit exceeded” error indicate?
This error indicates that the Java Virtual Machine (JVM) is spending too much time performing garbage collection and is unable to free up enough memory. It typically occurs when the application is running low on heap space.
What are the common causes of the “GC overhead limit exceeded” error?
Common causes include insufficient heap memory allocated to the JVM, memory leaks in the application, or processing large datasets that exceed the available memory limits.
How can I resolve the “OutOfMemoryError: GC overhead limit exceeded” error?
To resolve this error, consider increasing the heap size by adjusting the JVM options (e.g., `-Xmx`), optimizing your code to reduce memory usage, or identifying and fixing memory leaks in your application.
What JVM options can I use to increase heap memory?
You can use the `-Xms` option to set the initial heap size and the `-Xmx` option to set the maximum heap size. For example, `-Xms512m -Xmx2048m` allocates a minimum of 512 MB and a maximum of 2048 MB.
How can I diagnose memory issues in my Java application?
You can use profiling tools such as VisualVM, JProfiler, or Eclipse Memory Analyzer to monitor memory usage, identify memory leaks, and analyze heap dumps for better insights into memory allocation patterns.
Is it advisable to disable the GC overhead limit check?
Disabling the GC overhead limit check is generally not recommended as it may allow your application to run indefinitely in a low-memory state, leading to performance degradation and potential crashes. Instead, focus on optimizing memory usage.
The “OutOfMemoryError: GC overhead limit exceeded” error in Java is a common issue that arises when the Java Virtual Machine (JVM) is spending too much time performing garbage collection (GC) but is unable to reclaim enough memory. This situation indicates that the application is running low on heap space, leading to performance degradation. The JVM’s garbage collector is designed to free up memory by removing objects that are no longer in use; however, when it fails to do so efficiently, it results in this specific error. Understanding the underlying causes of this error is crucial for developers aiming to optimize their applications and ensure smooth performance.
One of the primary reasons for encountering this error is the allocation of insufficient heap memory to the JVM. When an application requires more memory than what is allocated, the garbage collector may struggle to keep up, leading to excessive GC activity. Additionally, memory leaks, where objects are unintentionally retained in memory, can exacerbate the problem. Developers should regularly monitor memory usage and utilize profiling tools to identify memory leaks and optimize memory allocation effectively.
To mitigate the “GC overhead limit exceeded” error, several strategies can be implemented. Increasing the heap size allocated to the JVM is a common solution, as it allows for more
Author Profile
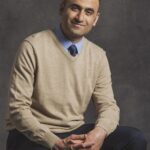
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?