How Can You Troubleshoot an AxiosError: Request Failed with Status Code 500?
When developing web applications, encountering errors is an inevitable part of the process. One such error that developers often face is the dreaded `AxiosError: Request failed with status code 500`. This error can be particularly frustrating, as it indicates a server-side issue that can stem from a variety of underlying problems. In this article, we will delve into the nuances of this error, exploring its causes, implications, and effective strategies for troubleshooting and resolution. Whether you’re a seasoned developer or just starting your journey in web development, understanding this error is crucial for maintaining robust and resilient applications.
At its core, a status code 500 signifies an internal server error, suggesting that something has gone awry on the server while processing the request. This can result from a range of issues, including misconfigurations, unhandled exceptions in server-side code, or even resource limitations. The challenge lies not only in identifying the root cause but also in implementing effective solutions to prevent its recurrence. As we navigate through the complexities of this error, we will highlight common scenarios that lead to a 500 status code and provide insights into best practices for error handling and debugging.
Moreover, understanding the context in which `AxiosError` occurs can significantly enhance your ability to diagnose and fix issues efficiently. By leveraging tools and
Understanding AxiosError
AxiosError is a specific error object returned by the Axios library when a request fails. This object provides detailed information about what went wrong during the HTTP request. When you encounter an error such as “request failed with status code 500,” it indicates that the server has encountered an unexpected condition that prevented it from fulfilling the request.
Key properties of the AxiosError object include:
- message: A human-readable description of the error.
- name: The error name, typically “AxiosError.”
- code: A string representing the error code.
- config: The configuration object used for the request.
- response: The response object that contains the status code and data returned from the server.
Common Causes of 500 Status Code
A 500 Internal Server Error is a generic error message indicating that something has gone wrong on the server side. It does not provide specific details about the issue. Common causes include:
- Server Misconfiguration: Issues in the server configuration files can lead to unexpected behaviors.
- Uncaught Exceptions: If the server application encounters an unhandled exception, it may result in a 500 error.
- Resource Limitations: Insufficient server resources, such as memory or CPU, can cause the server to fail when processing requests.
- Database Errors: Problems with the database connection or queries can lead to server errors.
Troubleshooting AxiosError with Status Code 500
To effectively troubleshoot and resolve issues related to AxiosError with status code 500, consider the following steps:
- Check Server Logs: Review server logs for error messages or stack traces that provide insight into what went wrong.
- Inspect the Request: Ensure that the request parameters, headers, and payload are correctly formatted and valid.
- Validate API Endpoints: Confirm that the API endpoint being accessed is correct and operational.
- Test with Tools: Use tools like Postman or curl to replicate the request and analyze the server’s response.
- Review Application Code: Examine the application code for potential bugs or logic errors that could lead to unhandled exceptions.
Example of AxiosError Handling
When handling AxiosError in your application, you can implement a structured error handling mechanism. Here’s an example of how you can catch and manage Axios errors:
“`javascript
axios.get(‘/api/endpoint’)
.then(response => {
// Handle successful response
console.log(response.data);
})
.catch(error => {
if (error.response) {
// Server responded with a status code outside the 2xx range
console.error(‘Error Status:’, error.response.status);
console.error(‘Error Data:’, error.response.data);
} else if (error.request) {
// Request was made but no response was received
console.error(‘No response received:’, error.request);
} else {
// Something else caused the error
console.error(‘Error Message:’, error.message);
}
});
“`
AxiosError Response Table
The following table summarizes the possible responses and respective actions when encountering AxiosError:
Status Code | Meaning | Recommended Action |
---|---|---|
500 | Internal Server Error | Check server logs for details |
404 | Not Found | Verify the endpoint URL |
403 | Forbidden | Check permissions and authentication |
400 | Bad Request | Inspect request payload and parameters |
By systematically analyzing the response and server conditions, developers can effectively address issues related to AxiosError and ensure smoother application performance.
Understanding AxiosError
Axios is a popular promise-based HTTP client for JavaScript, used to make requests to APIs. When using Axios, developers may encounter various errors, one of which is the AxiosError with a response status code of 500. This indicates an internal server error.
Causes of AxiosError with Status Code 500
An AxiosError with a status code of 500 signifies that the server encountered an unexpected condition that prevented it from fulfilling the request. The causes can vary, including but not limited to:
- Server Misconfiguration: Errors in server settings or deployment configurations can lead to a 500 status.
- Application Bugs: Unhandled exceptions or logical errors in the application code can trigger this response.
- Database Issues: Problems with database connections or queries can result in a server error.
- Resource Limitations: Exceeding memory or CPU limits can cause the server to fail in processing requests.
Debugging Steps
To effectively troubleshoot a 500 internal server error when using Axios, consider the following steps:
- Check Server Logs: Review the server logs for any error messages or stack traces that provide insights into the issue.
- Inspect API Endpoint: Verify that the API endpoint is correct and accessible. Test it using tools like Postman or cURL.
- Review Code Changes: If the error started occurring after recent changes, examine those modifications for potential issues.
- Test in Development: Replicate the issue in a development environment to identify the root cause without affecting production.
- Increase Logging: Temporarily enhance logging in the server application to capture more details around the error.
Handling AxiosError in Code
When handling AxiosError in your code, it is crucial to implement robust error handling mechanisms. Here’s an example of how to manage errors effectively:
“`javascript
axios.get(‘/api/endpoint’)
.then(response => {
// Handle successful response
console.log(response.data);
})
.catch(error => {
if (error.response) {
// The request was made, and the server responded with a status code
console.error(‘Error Status:’, error.response.status);
console.error(‘Error Data:’, error.response.data);
} else if (error.request) {
// The request was made but no response was received
console.error(‘No response received:’, error.request);
} else {
// Something else caused the error
console.error(‘Error Message:’, error.message);
}
});
“`
Best Practices for Avoiding 500 Errors
To reduce the occurrence of 500 errors, implement the following best practices:
- Input Validation: Ensure that all incoming data is validated to prevent errors caused by invalid data types or formats.
- Error Handling: Implement comprehensive error handling in your application logic to catch and manage exceptions.
- Monitoring and Alerts: Use monitoring tools to track server performance and set up alerts for unexpected issues.
- Load Testing: Perform load testing to identify potential bottlenecks or limitations in your application before it goes live.
By understanding the nature of AxiosError with status code 500, employing effective debugging strategies, and implementing best practices, developers can significantly minimize the impact of these errors on their applications.
Understanding AxiosError: Insights into Status Code 500
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “A status code 500 indicates an internal server error, suggesting that something has gone wrong on the server side. It’s crucial to examine server logs to identify the root cause, as this error can arise from unhandled exceptions or misconfigurations in the server environment.”
Michael Chen (Lead Backend Developer, Cloud Solutions Corp.). “When encountering an AxiosError with a status code 500, developers should first ensure that the API endpoint is functioning correctly. This may involve testing the endpoint independently and checking for any recent changes in the server code that could lead to such failures.”
Sarah Thompson (Technical Support Specialist, WebDev Helpdesk). “It’s essential to implement robust error handling in your Axios requests. Catching errors and providing informative feedback can help users understand the issue better. Additionally, consider implementing retries for transient errors that may cause a 500 status code intermittently.”
Frequently Asked Questions (FAQs)
What does the error “axioserror request failed with status code 500” mean?
This error indicates that the server encountered an unexpected condition that prevented it from fulfilling the request. A status code of 500 signifies an internal server error.
What are common causes of a 500 status code when using Axios?
Common causes include server misconfigurations, unhandled exceptions in server-side code, database errors, or issues with third-party services that the server relies on.
How can I troubleshoot a 500 status code error in my Axios request?
To troubleshoot, check the server logs for detailed error messages, ensure the server is running correctly, validate the request payload, and confirm that the endpoint is functioning as expected.
Can I handle a 500 status code error in my Axios code?
Yes, you can handle this error using a `.catch` block in your Axios request. This allows you to manage the error gracefully and provide feedback to users or log the error for further investigation.
What should I do if the 500 error persists despite troubleshooting?
If the error persists, consider reaching out to the server administrator or support team for assistance, as they may need to investigate server-side issues that are not visible from the client side.
Are there any specific Axios configurations that can help prevent 500 errors?
While Axios configurations cannot directly prevent 500 errors, ensuring proper request formatting, using timeouts, and implementing error handling can improve the robustness of your application and provide better user experiences.
The error message “axios error request failed with status code 500” indicates that a server-side issue has occurred while processing a request made using Axios, a popular JavaScript library for making HTTP requests. A status code of 500 signifies an internal server error, which means that the server encountered an unexpected condition that prevented it from fulfilling the request. This type of error can stem from various issues, including server misconfigurations, unhandled exceptions in server-side code, or problems with the database or other backend services.
When encountering this error, it is essential to investigate the server logs to identify the root cause of the issue. Developers should ensure that the server is properly configured and that all dependencies are functioning correctly. Additionally, implementing error handling in the server-side code can help catch exceptions and provide more informative error messages, which can assist in diagnosing the problem. Testing the API endpoints independently using tools like Postman can also help determine if the issue is specific to the Axios request or if it is a broader problem with the server.
In summary, a 500 status code error when using Axios is indicative of a significant issue on the server side that requires careful examination. By analyzing server logs, improving error handling, and conducting thorough testing, developers
Author Profile
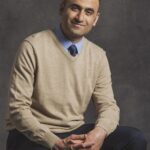
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?