How Can I Get the Directory of a Bash Script?
When working with Bash scripts, understanding the environment in which your script operates is crucial for effective coding. One common challenge that many developers face is determining the directory in which their script resides. This seemingly simple task can have significant implications, especially when your script relies on external files or resources located in the same directory. Whether you’re a seasoned developer or just starting your journey in shell scripting, mastering this technique will enhance your scripting skills and streamline your workflow.
In Bash, the ability to retrieve the directory of the currently executing script opens up a world of possibilities. It allows you to create scripts that are more portable and adaptable, as they can dynamically reference paths relative to their own location. This is particularly useful when distributing scripts across different systems or when working in environments where absolute paths may vary. By leveraging built-in variables and commands, you can easily access the script’s directory and use it to manage file paths, execute other scripts, or organize your project structure.
As we delve deeper into this topic, we will explore various methods to obtain the directory of a Bash script, highlighting best practices and potential pitfalls. Understanding these techniques will not only empower you to write more robust scripts but also enhance your ability to troubleshoot and maintain your code effectively. So, let’s unlock the secrets of script directories
Getting the Directory of a Bash Script
When working with Bash scripts, it is often necessary to determine the directory in which the script resides. This can be useful for various purposes, such as accessing other files relative to the script’s location. Below are methods to achieve this.
Using `$BASH_SOURCE`
The `$BASH_SOURCE` variable provides the path of the currently executing script. To obtain the directory, you can combine this variable with the `dirname` command. Here’s how you can do it:
“`bash
SCRIPT_DIR=$(dirname “$BASH_SOURCE”)
“`
This command extracts the directory part of the script’s path. If you want the absolute path, you can use the following code:
“`bash
SCRIPT_DIR=$(dirname “$(realpath “$BASH_SOURCE”)”)
“`
This ensures that you get the full path, resolving any symbolic links that may be present.
Using `$0`
Another common approach is using the special variable `$0`, which holds the name of the script as it was called. Similar to the previous method, you can obtain the directory with:
“`bash
SCRIPT_DIR=$(dirname “$0”)
“`
However, it’s essential to note that `$0` can sometimes return a relative path depending on how the script was executed. To ensure you get the absolute path, you may use:
“`bash
SCRIPT_DIR=$(dirname “$(realpath “$0″)”)
“`
Considerations for Different Execution Methods
The behavior of the above methods can vary based on how the script is executed. Here are some scenarios:
- Direct Execution: When running the script directly from the command line.
- Sourcing the Script: Using the `source` or `.` command will make `$BASH_SOURCE` point to the current shell, and `$0` might not reflect the script’s path.
- Symbolic Links: If the script is invoked through a symlink, using `realpath` will help resolve the actual path.
Comparison Table
Method | Variable Used | Returns | Notes |
---|---|---|---|
Using `$BASH_SOURCE` | $BASH_SOURCE | Script’s directory | Best for scripts called directly |
Using `$0` | $0 | Path from command line | May return relative path |
By understanding these methods and considerations, you can effectively manage file paths in your Bash scripts, enhancing portability and reliability in your scripting tasks.
Retrieve the Directory of a Script in Bash
When working with Bash scripts, determining the directory in which the script resides can be crucial for various tasks, such as accessing files or resources relative to the script’s location. Below are methods to achieve this.
Using `$0` and `dirname` Command
The most common way to get the directory of the currently executing script is by utilizing the special variable `$0`, which contains the path of the script. You can then combine it with the `dirname` command to extract the directory.
“`bash
SCRIPT_DIR=$(dirname “$0”)
“`
This command will return the directory path of the script when executed.
Resolving Absolute Paths
In some cases, the script may be executed with a relative path. To ensure you get the absolute path, use the following combination:
“`bash
SCRIPT_DIR=$(dirname “$(realpath “$0″)”)
“`
This command uses `realpath`, which resolves any symbolic links and returns the absolute path, ensuring accuracy regardless of how the script is called.
Handling Symbolic Links
If your script is executed via a symbolic link, you may want to get the directory of the actual script file. The `readlink` command can help in this situation:
“`bash
SCRIPT_DIR=$(dirname “$(readlink -f “$0″)”)
“`
This approach is particularly useful when scripts may be linked from different locations.
Example Script
Here’s a simple example that demonstrates how to retrieve and print the directory of a script:
“`bash
!/bin/bash
Get the script directory
SCRIPT_DIR=$(dirname “$(realpath “$0″)”)
Output the directory
echo “The script is located in: $SCRIPT_DIR”
“`
This script will output the directory in which it resides, utilizing the methods outlined above.
Considerations
When using these methods, be aware of the following:
- Execution Context: The value of `$0` can vary depending on how the script is invoked. Always use `realpath` or `readlink` for consistent results.
- Permissions: Ensure that the script has permission to access the directories involved.
- Portability: These commands are generally available in most Unix-like environments, but always test in your target environment for compatibility.
By employing these methods, you can reliably ascertain the directory of your Bash scripts, allowing for more robust and flexible script development.
Understanding Script Directory Retrieval in Bash
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “To obtain the directory of a script in Bash, one can utilize the special variable `$(dirname “$0″)`. This command effectively extracts the directory path of the currently executing script, which is essential for managing relative paths and ensuring that resources are accessed correctly.”
Michael Chen (Linux Systems Administrator, OpenSource Innovations). “When writing Bash scripts, it’s crucial to remember that using `$0` may yield unexpected results if the script is called through a symlink. To avoid this, it is advisable to use `$(dirname “$(readlink -f “$0″)”)`, which resolves the absolute path and provides the correct directory regardless of how the script is invoked.”
Sarah Thompson (DevOps Engineer, Cloud Solutions Inc.). “In complex Bash environments, understanding the context of script execution is vital. Using `$(cd “$(dirname “$0″)”; pwd)` not only retrieves the script directory but also changes the current directory to that of the script, which can be particularly useful when subsequent commands need to operate relative to the script’s location.”
Frequently Asked Questions (FAQs)
How can I get the directory of the currently executing script in Bash?
You can use the command `dirname “$0″` to obtain the directory of the currently executing script. This command returns the path of the script’s directory.
What if I want the absolute path of the script’s directory?
To get the absolute path, use `$(cd “$(dirname “$0″)”; pwd)`. This command changes to the script’s directory and prints the absolute path.
Does the method of obtaining the script directory differ when using sourced scripts?
Yes, when sourcing a script, `$0` refers to the parent script, not the sourced one. Instead, use `BASH_SOURCE` array: `dirname “${BASH_SOURCE[0]}”` for the directory of the sourced script.
Can I store the script directory in a variable for later use?
Yes, you can store the directory in a variable like this: `SCRIPT_DIR=$(dirname “$0”)`. This allows you to reference the script’s directory throughout your script.
What if the script is called from a different directory?
Using `dirname “$0″` will still return the path relative to the current working directory. To ensure you always get the absolute path, use `$(cd “$(dirname “$0″)”; pwd)`.
Are there any considerations for symbolic links when getting the script directory?
Yes, if your script is executed via a symbolic link, `$0` will reflect the link’s path. To resolve to the actual script location, use `readlink -f “$0″` combined with `dirname`.
In summary, obtaining the directory of a script in Bash is a fundamental task that can enhance the functionality and portability of shell scripts. The most common method to achieve this is by using the special variable `$(dirname “$0”)`, which returns the directory of the script being executed. This approach is particularly useful when scripts need to reference files or other resources located in the same directory or when they need to be executed from different locations without altering their behavior.
Additionally, it is important to consider the context in which the script is being executed. Using `$0` directly may yield unexpected results if the script is called through a symlink or from a different directory. To mitigate this issue, a more robust solution involves resolving the absolute path using `readlink` or `realpath`, ensuring that the script reliably identifies its directory regardless of how it is invoked.
mastering the techniques to get the directory of a script in Bash not only improves script reliability but also enhances user experience by allowing scripts to function correctly in diverse environments. By implementing best practices and understanding the nuances of path resolution, developers can create more adaptable and efficient shell scripts.
Author Profile
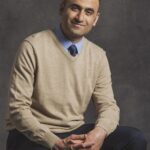
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?