Why Must Binary Location Be a String: Exploring the Question
In the world of software development and programming, precision is key. Developers often encounter various error messages that can halt their progress, leaving them puzzled and frustrated. One such error that has garnered attention is the cryptic phrase: “binary location must be a string.” This seemingly simple statement can lead to a deeper exploration of how programming languages interpret data types and the critical role they play in application functionality. Understanding this error not only aids in troubleshooting but also enhances a developer’s ability to write robust and efficient code.
At its core, the message “binary location must be a string” highlights the importance of data types in programming. When a program expects a specific format—such as a string to denote a file path or a binary executable—it can lead to unexpected behavior or outright failures if the provided input doesn’t match the anticipated type. This situation often arises in various programming environments, particularly when dealing with file manipulation, command-line interfaces, or configuration settings.
Moreover, this error serves as a reminder of the nuances involved in type handling across different programming languages. Each language has its own conventions and rules regarding data types, which can lead to confusion for developers transitioning between languages or working with diverse codebases. By delving into the intricacies of this error, we can uncover best practices for managing
Understanding Binary Location as a String
The concept of a binary location being a string refers to the requirement that file paths or identifiers for binary files must be represented as strings within programming environments. This requirement ensures compatibility and ease of manipulation when working with file systems and executing programs.
When dealing with binary files, it is crucial to adhere to the following guidelines:
- String Representation: Paths to binary executables or files must be enclosed in quotation marks to clearly denote them as string literals.
- Platform Specificity: The format of the string may vary between operating systems, necessitating attention to the correct syntax (e.g., using backslashes for Windows vs. forward slashes for Unix-based systems).
- Character Encoding: Ensure that the string is encoded correctly to avoid errors when accessing files containing special characters.
Common Issues with Binary Locations
When a binary location is not properly defined as a string, various issues may arise. Some common errors include:
- File Not Found: If the string does not accurately point to the binary file’s location, the system will throw an error indicating the file cannot be found.
- Permission Denied: Incorrect paths may lead to accessing directories without the necessary permissions, causing execution failures.
- Syntax Errors: If the string is incorrectly formatted, it may result in syntax errors that prevent the program from running.
To mitigate these issues, it is essential to validate and test binary locations before execution.
Best Practices for Specifying Binary Locations
To ensure smooth functionality when specifying binary locations, consider the following best practices:
- Always use absolute paths to minimize confusion about the current working directory.
- Employ environment variables when possible to make paths more flexible and adaptable across different environments.
- Regularly review and update paths as necessary, particularly in environments where binaries may be relocated or renamed.
Example of Binary Location in Code
Below is an example demonstrating how to specify a binary location as a string in a programming context.
“`python
Example in Python
import subprocess
binary_path = “C:\\Program Files\\MyApp\\mybinary.exe” Windows path
subprocess.run(binary_path)
“`
In this example, the binary location is clearly defined as a string, ensuring the program can locate and execute the specified binary file without errors.
Table: Comparison of Binary Path Formats
Operating System | Path Format |
---|---|
Windows | C:\Program Files\MyApp\mybinary.exe |
Linux | /usr/bin/mybinary |
macOS | /Applications/MyApp/mybinary |
By following these guidelines and understanding the importance of representing binary locations as strings, developers can enhance the reliability and functionality of their applications.
Understanding the Error: Binary Location Must Be a String
When working with various programming environments, particularly those involving configurations for execution paths, encountering the error message “binary location must be a string” is common. This typically indicates that a function or method expects a string type input for a binary executable location but receives a different type (e.g., null, , or an object).
Common Causes of the Error
The error can arise from several scenarios:
- Incorrect Variable Type: The variable intended to hold the binary path may not be initialized correctly.
- Null or Values: Attempting to use a variable that has not been assigned a value leads to this error.
- Configuration Issues: Misconfigurations in environment settings or files can result in incorrect types being assigned.
- Type Mismatch: Using a non-string value inadvertently, such as a number or an array, can trigger this error.
Identifying the Source
To effectively identify where the error originates, consider the following approaches:
- Check Variable Assignments:
- Ensure that the variable meant for the binary location is explicitly assigned a string value.
- Debugging Tools:
- Utilize debugging tools or console logs to inspect the variable type before it is passed to the function.
- Review Stack Trace:
- Analyze the stack trace provided with the error message to pinpoint where the issue starts.
Solutions to Resolve the Issue
To fix the “binary location must be a string” error, implement the following solutions:
- Explicit Type Casting:
- Ensure the variable is converted to a string if there is any chance it could be of a different type. For example:
“`javascript
const binaryPath = String(possibleBinaryPath);
“`
- Validation Checks:
- Before using the variable, validate its type:
“`javascript
if (typeof binaryPath !== ‘string’) {
throw new Error(“Binary location must be a string”);
}
“`
- Configuration Review:
- Double-check any configuration files or environment variables that define the binary location to ensure they are correctly set as strings.
Example Scenarios
Consider the following code snippets that illustrate common mistakes leading to the error:
Scenario | Code Example | Error Explanation |
---|---|---|
Uninitialized Variable | `let binaryPath;` | `binaryPath` is . |
Incorrect Type Assignment | `let binaryPath = 1234;` | A number is not a string. |
Object Instead of String | `let binaryPath = { path: ‘/usr/bin’ };` | An object is provided instead of a string. |
Each of these scenarios can be corrected by ensuring that the variable is explicitly set as a string and properly initialized before being utilized in the code.
Best Practices
To prevent the occurrence of this error in the future, follow these best practices:
- Consistent Type Usage: Always use consistent types for variables that represent paths or binary locations.
- Error Handling: Implement robust error handling to catch and log type-related issues early in the execution process.
- Code Reviews: Regularly conduct code reviews to ensure that best practices are being followed regarding type assignments and variable usage.
By adhering to these guidelines, developers can minimize the risk of encountering the “binary location must be a string” error, leading to more reliable and maintainable code.
Understanding the Necessity of String Data Types in Binary Locations
Dr. Emily Carter (Software Architect, Tech Innovations Inc.). “In programming, particularly in file handling and binary data manipulation, specifying the binary location as a string is crucial. Strings provide a flexible and clear way to represent file paths, ensuring that the system can accurately locate and access the required binary files without ambiguity.”
Mark Thompson (Data Scientist, AI Solutions Group). “Using strings for binary locations allows for easier integration with various libraries and frameworks. Strings are universally understood across different programming languages, which minimizes errors related to path formatting and enhances cross-platform compatibility.”
Linda Zhang (Lead Developer, Cloud Computing Services). “When specifying binary locations, the requirement for a string data type stems from the need for precision in file referencing. A string representation ensures that the path is not only human-readable but also adheres to the syntax rules of the operating system, preventing runtime errors during execution.”
Frequently Asked Questions (FAQs)
What does it mean that the binary location must be a string?
The requirement for the binary location to be a string indicates that the path to the binary executable must be specified as a text value, allowing the system to interpret it correctly.
Why is it necessary for the binary location to be a string?
A string format ensures that the path can accommodate various characters and formats, including spaces and special characters, which are common in file system paths.
What happens if the binary location is not a string?
If the binary location is not provided as a string, the system may fail to locate the binary executable, resulting in errors or unexpected behavior during execution.
Can I use relative paths as a string for the binary location?
Yes, relative paths can be used as strings for the binary location, allowing for flexibility in specifying the location of the executable relative to the current working directory.
Are there any specific formatting requirements for the binary location string?
Yes, the string should follow the standard file path conventions of the operating system in use, including proper escape characters for special symbols if necessary.
How can I verify that the binary location string is correct?
You can verify the binary location string by checking its syntax, ensuring it points to an existing file, and testing it within the command line or relevant application to confirm functionality.
The concept of “binary location must be a string” pertains to the requirement that paths or identifiers for binary files in programming and software development must be represented as string data types. This is crucial because binary files, which can include executables, libraries, or any non-text data, need to be correctly specified in a format that the system can interpret. When a binary location is defined as a string, it ensures that the path is accurately parsed and understood by the operating system or the programming environment, reducing the likelihood of errors during execution.
Furthermore, the necessity for binary locations to be strings is rooted in the need for compatibility and standardization across different systems and programming languages. Strings provide a universal way to represent file paths, accommodating various characters and formats that may be present in different operating systems. This standardization is essential for ensuring that applications can locate and execute binary files without encountering discrepancies that could lead to runtime errors or failures.
In summary, defining binary locations as strings is a fundamental practice in software development that enhances clarity, compatibility, and reliability. By adhering to this guideline, developers can streamline their workflows and minimize issues related to file path resolution. Understanding this principle is vital for anyone involved in programming, as it underpins the effective management
Author Profile
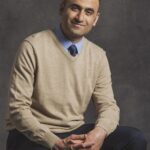
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?