How Can I Resolve the ‘Broken Pipe: java.io.IOException’ Error in My Java Application?
In the world of Java programming, encountering exceptions is an inevitable part of the development process. Among the myriad of exceptions that developers may face, the `java.io.IOException: Broken pipe` stands out as a particularly perplexing issue. This exception often signals a disruption in communication between a client and a server, leaving developers scratching their heads as they attempt to decipher its underlying causes and implications. Understanding this exception is crucial for anyone working with networked applications, as it can lead to significant disruptions in data flow and user experience.
The `broken pipe` error typically arises when one end of a connection attempts to write data to a stream that has already been closed by the other end. This can happen in various scenarios, such as when a client unexpectedly disconnects or when network interruptions occur. The result is a frustrating halt in operations, prompting developers to investigate the reasons behind the disconnection and how to handle it gracefully. As we delve deeper into this topic, we will explore the common causes of the `java.io.IOException: Broken pipe`, effective strategies for troubleshooting, and best practices for preventing this issue from derailing your applications.
By equipping yourself with a solid understanding of this exception, you can enhance the robustness of your Java applications and improve their resilience against unexpected network behavior
Understanding the IOException: Broken Pipe
When dealing with input and output operations in Java, encountering an `IOException` with the message “broken pipe” can be a common issue, particularly when working with sockets or file streams. This error typically indicates that an application tried to write to a stream that has been closed on the other end. Understanding the causes and implications of a broken pipe can help in effectively managing and debugging these scenarios.
Common Causes of Broken Pipe Errors
Several scenarios can lead to a broken pipe exception. Identifying these can assist developers in preventing or mitigating the issue:
- Socket Disconnection: When a client disconnects from a server or vice versa while the server is still attempting to send data.
- Timeouts: Network timeouts can occur, especially in long-running applications, which may lead to the disconnection of streams.
- Resource Management: Improper handling of streams, such as closing a stream that is still in use, can also result in a broken pipe.
Best Practices to Avoid Broken Pipe Exceptions
To minimize the chances of encountering broken pipe exceptions, consider implementing the following best practices:
- Check Connection Status: Before writing data to a socket, ensure that the connection is still alive.
- Handle Exceptions Gracefully: Implement robust exception handling to manage `IOExceptions` effectively.
- Use Heartbeat Mechanisms: For long-lived connections, use a heartbeat mechanism to detect dead connections early.
- Close Streams Properly: Always ensure that streams are closed only when they are no longer needed.
Handling Broken Pipe in Code
In Java, handling an `IOException` due to a broken pipe can be achieved using proper try-catch blocks. Below is an example of how to handle such exceptions:
“`java
try {
OutputStream outputStream = socket.getOutputStream();
outputStream.write(data);
} catch (IOException e) {
if (e.getMessage().contains(“broken pipe”)) {
System.err.println(“Error: Broken pipe. The remote side has closed the connection.”);
// Additional handling logic
} else {
// Handle other IOExceptions
}
}
“`
Error Handling Strategy
To effectively manage errors related to broken pipes, consider adopting an error handling strategy that includes:
- Logging: Maintain logs for occurrences of broken pipe errors for analysis.
- Retries: Implement a retry mechanism for transient errors if applicable.
- User Notifications: Inform users of connectivity issues in real-time to improve user experience.
Summary of Key Points
Cause | Prevention |
---|---|
Socket Disconnection | Check connection status before writing data |
Timeouts | Use heartbeat mechanisms |
Poor Resource Management | Close streams properly |
By understanding the underlying issues and implementing preventive measures, developers can significantly reduce the occurrence of broken pipe exceptions in their Java applications.
Understanding `java.io.IOException: Broken Pipe`
The `java.io.IOException: Broken Pipe` error occurs primarily in network programming scenarios. It indicates that a process attempted to write to a socket that has been closed by the peer. This typically arises when the other end of the connection has terminated unexpectedly or has closed the connection.
Common Causes of Broken Pipe Error
Several factors can contribute to this error:
- Network Disruptions: Temporary network failures or interruptions can cause socket connections to drop.
- Timeouts: If a connection remains idle for a prolonged period, it may lead to a timeout, resulting in one side closing the connection.
- Application Logic Errors: The application may attempt to write to a socket after it has been closed, either due to logical flaws in the code or improper error handling.
- Resource Limitations: Running out of system resources, such as file descriptors, can also lead to broken pipes.
How to Handle Broken Pipe Exception
Effective handling of the `Broken Pipe` exception involves several strategies:
- Exception Handling: Implement robust exception handling to catch `IOException` and respond appropriately.
“`java
try {
outputStream.write(data);
} catch (IOException e) {
if (e.getMessage().contains(“Broken pipe”)) {
// Handle broken pipe scenario
}
}
“`
- Connection Checks: Before writing to a socket, check if the connection is still open. This can prevent attempts to write to a closed socket.
“`java
if (!socket.isClosed()) {
outputStream.write(data);
}
“`
- Retries: Implement a retry mechanism for transient network issues. If a write fails due to a broken pipe, retry after a short delay.
- Graceful Shutdown: Ensure that both sides of the communication close their connections gracefully to avoid abrupt terminations.
Best Practices for Socket Programming
To minimize the occurrence of broken pipe errors, consider the following best practices:
Best Practice | Description |
---|---|
Keep Connections Alive | Use keep-alive settings to maintain long-lived connections. |
Timeout Settings | Set appropriate read and write timeouts for sockets. |
Error Logging | Log errors for better debugging and monitoring. |
Connection Pooling | Utilize connection pools to manage socket connections efficiently. |
Protocol Design | Design protocols that handle connection loss gracefully. |
Debugging Broken Pipe Issues
When encountering a `Broken Pipe` error, debugging can involve several steps:
- Check Logs: Review application and server logs for error messages or warnings preceding the broken pipe error.
- Network Monitoring: Use network monitoring tools to identify connectivity issues or unexpected disconnections.
- Test Under Load: Simulate high-load conditions to see if the issue is related to resource exhaustion.
- Review Code: Inspect the application’s logic, particularly around socket management and error handling.
Implementing these strategies can help in effectively managing and debugging the `java.io.IOException: Broken Pipe` error, leading to more resilient network applications.
Understanding the Causes and Solutions for Java’s IOException: Broken Pipe
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘broken pipe’ error in Java typically arises when a process attempts to write to a socket that has been closed on the other end. This can often occur in network programming when the client disconnects unexpectedly. To mitigate this, developers should implement proper error handling and ensure that connections are gracefully closed.”
Michael Chen (Lead Developer, Cloud Solutions Group). “In my experience, the ‘java.io.IOException: Broken pipe’ error is frequently encountered in high-load environments. It is essential to monitor the health of your network connections and employ timeout settings. Additionally, using asynchronous I/O can help in managing connections more effectively and reduce the likelihood of such errors.”
Sarah Johnson (Network Architect, Global Tech Networks). “Addressing a ‘broken pipe’ exception requires a comprehensive understanding of the underlying network architecture. It is crucial to analyze the server and client interactions, ensuring that both sides of the connection are synchronized. Implementing retry logic and connection pooling can also significantly improve resilience against this type of error.”
Frequently Asked Questions (FAQs)
What does a “broken pipe” error mean in Java?
A “broken pipe” error in Java typically indicates that an attempt was made to write to a socket that has been closed by the other end. This occurs when the receiving end of the communication has terminated the connection unexpectedly.
What causes a java.io.IOException: Broken pipe?
This exception is usually caused by network issues, such as the remote host closing the connection before the data can be sent. It can also occur if there is a timeout on the connection or if the application attempts to write to a socket after it has been closed.
How can I handle a broken pipe exception in Java?
To handle a broken pipe exception, implement proper error handling using try-catch blocks around your socket write operations. Additionally, ensure that you check the connection status before attempting to write data.
What are the common scenarios that lead to a broken pipe in network programming?
Common scenarios include abrupt termination of the client or server application, network failures, or exceeding the maximum allowed data transmission size, which can lead to socket closure.
Can a broken pipe error be prevented in Java applications?
While it may not be entirely preventable, you can minimize the occurrence by implementing robust connection management, using keep-alive options, and properly handling socket timeouts and closures.
Is a broken pipe exception specific to Java, or can it occur in other programming languages?
A broken pipe exception is not specific to Java; it can occur in any programming language that utilizes socket communication. The underlying concept remains the same across different platforms and languages.
The “broken pipe” error in Java, specifically represented as `java.io.IOException: Broken pipe`, typically occurs when a program attempts to write data to a socket or a stream that has been closed by the other end. This situation often arises in network programming, where one side of the communication may terminate unexpectedly or intentionally, leading to the disruption of the data flow. Understanding the context and causes of this exception is crucial for developers to implement robust error handling and maintain the stability of their applications.
Key takeaways from the discussion include the importance of proper resource management in Java applications, particularly when dealing with I/O operations. Developers should ensure that they handle exceptions gracefully and implement retry mechanisms when appropriate. Additionally, monitoring the state of connections and implementing timeouts can help prevent scenarios where a broken pipe may occur, thus enhancing the overall reliability of networked applications.
Furthermore, it is essential for developers to be aware of the implications of the broken pipe error on user experience and application performance. By proactively addressing potential issues related to socket communication and stream management, developers can minimize disruptions and provide a seamless experience for users. Continuous testing and logging can also aid in identifying patterns that lead to such exceptions, allowing for more informed decisions in application design and architecture.
Author Profile
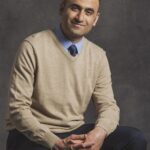
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?