Understanding the BrokenPipeError: What Causes Errno 32 and How Can You Fix It?
In the world of programming and system operations, encountering errors is an inevitable part of the journey. Among the myriad of issues that developers face, the `BrokenPipeError: [Errno 32] Broken pipe` stands out as a particularly perplexing challenge. This error, often encountered in network programming or inter-process communication, can disrupt the flow of data and halt processes, leading to frustration and delays. Understanding the underlying causes and solutions to this error is crucial for developers and system administrators alike, as it can significantly impact the reliability and efficiency of applications.
At its core, the `BrokenPipeError` occurs when one end of a communication channel attempts to write data while the other end has already closed the connection. This can happen in various scenarios, such as when a client disconnects unexpectedly or when a server fails to handle requests properly. The implications of this error extend beyond mere annoyance; they can lead to data loss and degraded user experiences. As we delve deeper into this topic, we will explore the common situations that trigger this error, the mechanisms behind it, and effective strategies for troubleshooting and prevention.
By equipping yourself with a comprehensive understanding of the `BrokenPipeError`, you can enhance your programming skills and improve the resilience of your applications. Whether you’re a seasoned developer or
Understanding BrokenPipeError
BrokenPipeError occurs in Unix-like operating systems when a process attempts to write to a pipe while the other end of the pipe has been closed. This error is typically represented by the error code `errno 32`. It is often encountered in scenarios involving inter-process communication or when dealing with network sockets.
When a program writes data to a pipe, it expects the receiving end to be active. If the receiver has terminated or closed the connection, the sending process receives a BrokenPipeError upon attempting to send more data. This can happen in various contexts, such as:
- Writing to a closed socket
- Sending data through a subprocess that has exited
- Using output streams that have been closed
Common Scenarios Leading to BrokenPipeError
There are several situations where you might encounter a BrokenPipeError. Understanding these can help in troubleshooting and preventing the error in future applications:
- Network Programming: When a client disconnects unexpectedly from a server, and the server tries to send data to the client.
- Piping Commands: In shell scripting, if a command in a pipeline exits before the preceding command finishes writing.
- File Operations: Writing to a file descriptor that has been closed or is no longer valid.
Handling BrokenPipeError in Python
When working with Python, it is essential to handle BrokenPipeError gracefully to prevent your application from crashing. You can manage this error using exception handling.
Example code snippet:
“`python
import socket
try:
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.connect((‘localhost’, 8080))
s.sendall(b’Hello, World!’)
except BrokenPipeError:
print(“Caught BrokenPipeError: The receiver has closed the connection.”)
finally:
s.close()
“`
In this example, if the connection is closed before the send operation, the exception is caught, allowing the program to respond appropriately.
Best Practices to Avoid BrokenPipeError
To minimize the likelihood of encountering a BrokenPipeError, consider the following best practices:
- Check Connection Status: Before writing data, ensure that the connection is still active.
- Use Non-blocking Sockets: Implement non-blocking sockets to handle connections more effectively.
- Set Socket Options: Adjust socket options such as `SO_SNDTIMEO` to define send timeouts.
- Implement Retry Logic: When a BrokenPipeError occurs, implement a retry mechanism with appropriate backoff strategies.
Summary of Key Points
Scenario | Action |
---|---|
Network disconnection | Handle with try-except |
Closed file descriptor | Check before write |
Pipeline command failure | Monitor command exits |
By following these guidelines and understanding the conditions that lead to BrokenPipeError, developers can better manage their applications and ensure smoother execution without abrupt interruptions.
Understanding Broken Pipe Errors
A Broken Pipe Error occurs when a process attempts to write to a pipe while the reading end has been closed. This error is represented by the error code `errno 32` in Unix-based systems.
Causes of Broken Pipe Errors
- Process Termination: The reading process terminates before the writing process completes its operation.
- Network Issues: In socket programming, network disconnections can lead to a broken pipe.
- Timeouts: Long operations without data transfer can cause timeouts, resulting in the reading end closing the connection.
Symptoms of Broken Pipe Errors
- Error messages indicating `BrokenPipeError` or `errno 32`.
- Unexpected termination of processes.
- Data not being sent or received as intended.
Handling Broken Pipe Errors
To effectively manage Broken Pipe Errors, implement the following strategies:
- Error Handling: Use try-except blocks in your code to catch exceptions related to broken pipes.
- Graceful Shutdown: Ensure that processes are designed to handle shutdown signals gracefully, allowing for proper closing of pipes.
- Retries: Implement retry logic for transient errors, especially in network communication.
Example Code Snippet
Here is a Python example demonstrating how to handle a Broken Pipe Error:
“`python
import os
import signal
import sys
def signal_handler(signum, frame):
print(“Received signal:”, signum)
sys.exit(0)
signal.signal(signal.SIGINT, signal_handler)
try:
Simulate writing to a pipe
os.write(pipe_fd, b”data”)
except BrokenPipeError:
print(“Caught Broken Pipe Error. The other end has closed.”)
“`
Debugging Broken Pipe Errors
When troubleshooting Broken Pipe Errors, consider the following:
- Check Process Status: Verify the status of both the writing and reading processes to ensure they are alive.
- Log Messages: Implement logging to capture the sequence of operations leading up to the error.
- Use Tools: Utilize tools such as `strace` or `tcpdump` to monitor system calls and network traffic.
Prevention Techniques
To minimize the occurrence of Broken Pipe Errors, employ the following techniques:
- Keep Alive Mechanisms: Use keep-alive messages in network applications to maintain connections.
- Timeout Configuration: Adjust timeout settings for socket connections to allow longer operations before considering the connection lost.
- Buffering: Implement buffering strategies to handle bursts of data, preventing premature closure of pipes.
By understanding the underlying causes and implementing robust error handling, you can effectively manage Broken Pipe Errors in your applications.
Understanding the Broken Pipe Error: Insights from Professionals
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘broken pipe’ error, specifically errno 32, typically occurs when one end of a communication channel is closed while the other end is still attempting to send data. This situation often arises in network programming or inter-process communication, where proper error handling is crucial to avoid unexpected crashes.”
Mark Thompson (Network Systems Analyst, CyberSecure Solutions). “In my experience, a broken pipe error can indicate that the application is trying to write to a socket that has been closed by the peer. It is essential to implement robust connection management and to monitor the state of the connection actively to mitigate such issues.”
Linda Garcia (DevOps Specialist, CloudTech Enterprises). “To effectively address the broken pipe error, developers should consider using retries with exponential backoff strategies. This approach allows for temporary network issues to resolve before attempting to resend data, thereby reducing the likelihood of encountering errno 32.”
Frequently Asked Questions (FAQs)
What does the error “broken pipe” mean?
The “broken pipe” error indicates that a process is trying to write to a pipe that has no reader. This typically occurs when the reading end of the pipe has closed before the writing process attempts to send data.
What causes the “errno 32 broken pipe” error?
The “errno 32 broken pipe” error is caused by a situation where a process attempts to write data to a socket or pipe after the other end has been closed. This can happen in network communications or inter-process communications.
How can I troubleshoot a broken pipe error?
To troubleshoot a broken pipe error, check if the receiving end of the pipe or socket is still open. Ensure that both processes are synchronized and consider implementing error handling to manage unexpected closures.
Is the broken pipe error specific to certain programming languages?
No, the broken pipe error is not specific to any programming language. It can occur in various programming environments, including Python, C, and Java, whenever inter-process communication is involved.
Can I prevent the broken pipe error in my application?
Yes, you can prevent the broken pipe error by implementing proper error handling, checking the status of the connection before writing data, and ensuring that the reading process is active and ready to receive data.
What should I do if I encounter a broken pipe error in a production environment?
In a production environment, investigate the source of the error by reviewing logs and monitoring the communication between processes. Implement retry mechanisms and graceful error handling to minimize disruptions.
The “BrokenPipeError: [Errno 32] Broken pipe” is a common error encountered in programming, particularly in network communications and inter-process communications. This error typically arises when one end of a pipe or socket attempts to write data while the other end has already closed the connection. As a result, the writing process fails, leading to this specific error message. Understanding the context in which this error occurs is crucial for effective debugging and resolution.
One significant aspect of this error is its occurrence in various programming environments, including Python, where it is frequently reported when dealing with socket programming or subprocesses. Developers need to be aware of the lifecycle of their connections and ensure that they handle exceptions properly. Implementing robust error handling strategies, such as using try-except blocks, can mitigate the impact of this error on applications.
Another key takeaway is the importance of monitoring the state of connections. Regularly checking whether a connection is still open before attempting to send data can prevent the “BrokenPipeError” from occurring. Additionally, employing techniques such as graceful shutdowns and proper resource management can enhance the reliability of applications and reduce the likelihood of encountering this error in the first place.
Author Profile
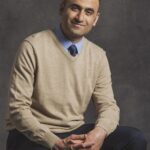
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?