What Does the C# Question Mark After a Type Mean?
In the world of Cprogramming, understanding data types is fundamental to writing efficient and effective code. Among the many nuances of C, one symbol stands out for its significance: the question mark (`?`). This seemingly simple character can transform the way developers handle data, particularly when it comes to dealing with null values. As software applications become increasingly complex, mastering the use of the question mark can be a game changer, allowing for more robust and error-resistant coding practices.
At its core, the question mark in Cserves a critical purpose in defining nullable types. By appending a question mark to a value type, developers can indicate that the variable is allowed to hold a null value, expanding the flexibility of data handling. This feature is particularly useful in scenarios where a value may not always be present, such as in database interactions or when dealing with optional parameters. Understanding how to effectively utilize nullable types can lead to cleaner, more maintainable code and can help prevent common pitfalls associated with null references.
Moreover, the question mark is not just limited to defining nullable types; it also plays a key role in the null-coalescing operator and conditional expressions. These constructs enable developers to write more concise and expressive code, reducing the verbosity often associated with traditional null checks. As we delve deeper
Understanding the Question Mark in C
In C, the question mark `?` signifies that a type is nullable. This means that a value type can also hold a null value, which is particularly useful for representing or missing data. This feature is primarily applied to value types, such as integers, doubles, and structs, which by default cannot be assigned null.
When using the `?` syntax, a value type is transformed into a nullable type. For instance, an `int` type can be made nullable by declaring it as `int?`. Here are some key points regarding nullable types:
- Declaration: To declare a nullable type, simply append the question mark to the type name, e.g., `int? myNullableInt;`.
- Assignment: You can assign a value to a nullable type just like a regular type, but you can also assign `null`, e.g., `myNullableInt = 5;` or `myNullableInt = null;`.
- Checking for null: Use the `HasValue` property to check if the nullable type has a value, and the `Value` property to access the value itself.
Working with Nullable Types
Utilizing nullable types enables more expressive and safer code when dealing with databases or data that might not be present. Below is a table summarizing the properties and methods associated with nullable types:
Property/Method | Description |
---|---|
HasValue | Returns true if the nullable type has a value; otherwise, . |
Value | Gets the value of the nullable type, throwing an exception if it is null. |
GetValueOrDefault() | Returns the value if it has one; otherwise, returns the default value of the underlying type. |
Here is a code example demonstrating the use of nullable types:
“`csharp
int? myNullableInt = null;
if (myNullableInt.HasValue)
{
Console.WriteLine($”The value is: {myNullableInt.Value}”);
}
else
{
Console.WriteLine(“The value is null.”);
}
“`
In this example, since `myNullableInt` is null, the output will indicate that the value is null. This illustrates how nullable types can help prevent exceptions and provide cleaner code when dealing with optional data.
Common Use Cases for Nullable Types
Nullable types are particularly useful in several scenarios:
- Database Operations: When working with databases, certain fields might not have values (e.g., a date of birth for an unspecified user). Using nullable types allows you to handle such cases seamlessly.
- Optional Parameters: When defining methods that accept optional parameters, nullable types can indicate that a parameter is not required.
- Data Transfer Objects (DTOs): When transferring data between layers or systems, nullable types can reflect the optional nature of certain fields.
By understanding and utilizing nullable types effectively, developers can write more robust and error-resistant Capplications.
Understanding the Question Mark in C
In C, the question mark (`?`) has specific meanings depending on its context, particularly when used with data types. This operator is primarily associated with nullable types and the conditional operator.
Nullable Types
A nullable type can represent all the values of its underlying type plus an additional `null` value. This is particularly useful for value types such as `int`, `double`, or `bool`, which normally cannot hold a `null` value.
- Declaration: To declare a nullable type, append a question mark to the type name.
“`csharp
int? nullableInt = null; // This can hold either an int value or null.
“`
- Usage: Nullable types are often used in database operations where a field can be empty.
- Properties:
- The `HasValue` property indicates whether the variable holds a value.
- The `Value` property retrieves the actual value, but it will throw an `InvalidOperationException` if `HasValue` is .
Nullable Type | Description |
---|---|
`int?` | Nullable integer |
`double?` | Nullable double |
`bool?` | Nullable boolean |
Conditional Operator
The question mark is also used in the conditional (ternary) operator, which provides a shorthand way to perform an if-else operation.
– **Syntax**:
“`csharp
condition ? trueExpression : Expression;
“`
– **Example**:
“`csharp
int a = 5;
int b = 10;
int max = (a > b) ? a : b; // max will be 10
“`
- Use Cases:
- Simplifies code by reducing the number of lines.
- Enhances readability for simple conditional assignments.
Null Coalescing Operator
Calso utilizes the `??` operator, known as the null coalescing operator, which can be used with nullable types to provide a default value.
- Syntax:
“`csharp
var result = nullableValue ?? defaultValue;
“`
- Example:
“`csharp
int? nullableInt = null;
int nonNullableInt = nullableInt ?? 0; // nonNullableInt will be 0
“`
Practical Considerations
When working with nullable types and the question mark operator, consider the following:
- Performance: Nullable types incur a slight performance overhead due to additional checks for null values.
- Error Handling: Always check `HasValue` before accessing the `Value` property to avoid exceptions.
- Readability: Use nullable types judiciously to maintain code clarity and prevent confusion.
Understanding the use of the question mark in Cis crucial for effective programming, especially when dealing with nullable types and conditional expressions. Familiarity with these concepts enhances the ability to write robust and error-free code.
Understanding the Significance of the CQuestion Mark in Type Definitions
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). The question mark in Csignifies a nullable type, allowing developers to assign null values to value types. This feature enhances flexibility in handling database operations and user inputs, where nullability is often a requirement.
Michael Zhang (CDeveloper Advocate, CodeCraft Academy). Utilizing the question mark after a type in Cis crucial for ensuring that your applications can gracefully handle scenarios where data may not be present. It promotes safer code practices by reducing the risk of null reference exceptions.
Sarah Thompson (Lead Architect, FutureTech Solutions). The question mark in Cnot only indicates that a value type can be null but also plays a significant role in LINQ queries and data manipulation. Understanding how to leverage nullable types effectively can lead to more robust and maintainable code.
Frequently Asked Questions (FAQs)
What does the question mark after a type mean in C?
The question mark indicates that the type is a nullable type. It allows value types, such as integers or booleans, to also represent a null value.
How do I declare a nullable type in C?
To declare a nullable type, append a question mark to the value type. For example, `int? myNullableInt = null;` allows `myNullableInt` to hold an integer or null.
Can I use nullable types with reference types in C?
No, reference types in Care inherently nullable. The question mark is only applicable to value types, such as `int`, `double`, or `bool`.
How do I check if a nullable type has a value?
You can use the `HasValue` property or compare the nullable type to null. For example, `if (myNullableInt.HasValue)` or `if (myNullableInt != null)` checks for a value.
What happens if I try to access the value of a null nullable type?
Attempting to access the value of a null nullable type using the `.Value` property will throw an `InvalidOperationException`. Always check for a value before accessing it.
Are there any performance implications of using nullable types in C?
Nullable types may introduce slight overhead due to additional memory allocation for the null state. However, the impact is generally minimal and often outweighed by the benefits of type safety and clarity in code.
The use of the question mark after a type in Csignifies that the type is a nullable type. This feature allows value types, such as integers and booleans, to also hold a null value, which is particularly useful when dealing with databases or scenarios where a value might be absent. By declaring a variable with a question mark, developers can differentiate between a variable that has a valid value and one that is intentionally left uninitialized or .
Nullable types are especially beneficial in situations where data integrity is crucial. For instance, when working with databases, certain fields may not always have a value. Using nullable types enables developers to represent these scenarios accurately without resorting to workarounds like using sentinel values. This enhances code clarity and reduces the risk of errors associated with uninitialized variables.
Additionally, nullable types come with built-in methods and properties that facilitate the handling of null values. For example, the `GetValueOrDefault()` method can be used to provide a default value if the nullable variable is null. This feature simplifies code and improves maintainability, allowing developers to focus on core logic without constantly checking for null references.
In summary, the question mark after a type in Cis a powerful feature that
Author Profile
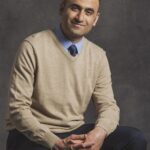
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?