How Can I Read a CSV File in C#?
In the world of data manipulation and analysis, CSV (Comma-Separated Values) files serve as a universal language, bridging the gap between different software applications and programming languages. For Cdevelopers, the ability to read and process CSV files is an essential skill that opens up a plethora of opportunities for data handling, reporting, and integration. Whether you’re working on a small project or a large-scale enterprise application, mastering the techniques to read CSV files can significantly enhance your productivity and efficiency.
As you delve into the intricacies of reading CSV files in C, you’ll discover a range of methods and libraries that simplify this process. From leveraging built-in functionalities to utilizing powerful third-party libraries, Cprovides developers with the tools needed to parse, manipulate, and analyze data seamlessly. Understanding how to effectively read CSV files not only enhances your coding repertoire but also empowers you to tackle real-world data challenges with confidence.
In this article, we will explore the various approaches to reading CSV files in C, highlighting best practices and common pitfalls to avoid. Whether you’re a novice programmer or an experienced developer looking to refresh your skills, this guide will equip you with the knowledge necessary to work with CSV data efficiently, enabling you to unlock the full potential of your applications.
Reading CSV Files in C
To read a CSV file in C, you can utilize various libraries and approaches, each offering different levels of complexity and functionality. Below are some common methods, including using built-in functionalities, third-party libraries, and best practices for handling CSV data.
Using StreamReader
One straightforward way to read a CSV file is by using the `StreamReader` class from the `System.IO` namespace. This method allows you to read the file line by line, making it easy to parse the contents.
“`csharp
using System;
using System.IO;
class Program
{
static void Main()
{
string path = “path_to_your_file.csv”;
using (StreamReader sr = new StreamReader(path))
{
string line;
while ((line = sr.ReadLine()) != null)
{
string[] values = line.Split(‘,’);
// Process the values as needed
}
}
}
}
“`
In this example, each line of the CSV is split into an array of strings using the `Split` method. You can then process these values as needed.
Using CsvHelper Library
For more advanced CSV handling, consider using the CsvHelper library, which simplifies reading and writing CSV files while providing strong typing and additional functionality.
- Install the CsvHelper package via NuGet:
“`
Install-Package CsvHelper
“`
- Use the following code to read a CSV file:
“`csharp
using System.Globalization;
using CsvHelper;
using System.IO;
class Program
{
static void Main()
{
using (var reader = new StreamReader(“path_to_your_file.csv”))
using (var csv = new CsvReader(reader, CultureInfo.InvariantCulture))
{
var records = csv.GetRecords
// Process records as needed
}
}
}
“`
In this example, `YourClass` should be a model class that represents the structure of your CSV data, with properties matching the CSV headers.
Defining a Model Class
When using CsvHelper, it’s essential to define a model class that reflects the structure of your CSV file. Here is an example:
“`csharp
public class YourClass
{
public int Id { get; set; }
public string Name { get; set; }
public string Email { get; set; }
}
“`
This class should have properties that match the columns in your CSV file.
Handling Delimiters and Quoting
CSV files may use different delimiters (such as semicolons or tabs) and can have quoted fields. CsvHelper allows you to customize these settings easily:
“`csharp
var csvConfig = new CsvConfiguration(CultureInfo.InvariantCulture)
{
Delimiter = “;”,
BadDataFound = null, // Ignore bad data
};
using (var reader = new StreamReader(“path_to_your_file.csv”))
using (var csv = new CsvReader(reader, csvConfig))
{
var records = csv.GetRecords
}
“`
Common Issues and Best Practices
When working with CSV files, be aware of the following common issues:
- Inconsistent Data Formats: Ensure that all data adheres to the expected format.
- Special Characters: Handle characters that may disrupt parsing, such as commas within quoted fields.
- Missing Values: Implement error handling for missing or malformed data.
Best practices to follow include:
- Validate the CSV data structure before processing.
- Use appropriate data types in your model class.
- Handle exceptions gracefully to ensure robustness.
Example CSV File Structure
Here is an example of how a CSV file might be structured:
Id | Name | |
---|---|---|
1 | John Doe | [email protected] |
2 | Jane Smith | [email protected] |
This structure can be easily mapped to a corresponding model class in C.
By following these guidelines, you can effectively read and manipulate CSV files in your Capplications.
Using CsvHelper Library
The CsvHelper library is a popular choice for reading and writing CSV files in C. It simplifies the process and provides a robust framework for handling CSV data.
- Installation: First, install the CsvHelper package via NuGet. Use the following command in the Package Manager Console:
“`
Install-Package CsvHelper
“`
- Basic Example:
Here’s a straightforward example of reading a CSV file:
“`csharp
using CsvHelper;
using System.Globalization;
using System.IO;
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
}
public void ReadCsv()
{
using (var reader = new StreamReader(“path/to/file.csv”))
using (var csv = new CsvReader(reader, CultureInfo.InvariantCulture))
{
var records = csv.GetRecords
foreach (var record in records)
{
Console.WriteLine($”{record.Name}, {record.Age}”);
}
}
}
“`
This code snippet demonstrates how to read a CSV file and map its contents to a Cobject. Each row in the CSV corresponds to an instance of the `Person` class.
Reading CSV with Built-in .NET Methods
For simpler cases, especially when you do not need the extensive functionality provided by external libraries, you can use built-in .NET methods to read CSV files.
- Example:
The following code uses `StreamReader` and `Split` method to read a CSV file:
“`csharp
using System;
using System.IO;
public void ReadCsvWithStreamReader()
{
var filePath = “path/to/file.csv”;
using (var reader = new StreamReader(filePath))
{
string line;
while ((line = reader.ReadLine()) != null)
{
var values = line.Split(‘,’);
Console.WriteLine($”Name: {values[0]}, Age: {values[1]}”);
}
}
}
“`
This method splits each line by commas, assuming that the data does not include commas within the values themselves.
Handling Different Delimiters
Sometimes, CSV files may use different delimiters, such as semicolons or tabs. The CsvHelper library allows you to specify a custom delimiter easily.
- Example with Custom Delimiter:
“`csharp
using CsvHelper;
using System.Globalization;
using System.IO;
public void ReadCsvWithCustomDelimiter()
{
using (var reader = new StreamReader(“path/to/file.csv”))
using (var csv = new CsvReader(reader, new CsvConfiguration(CultureInfo.InvariantCulture)
{
Delimiter = “;”, // Specify your custom delimiter here
}))
{
var records = csv.GetRecords
foreach (var record in records)
{
Console.WriteLine($”{record.Name}, {record.Age}”);
}
}
}
“`
This snippet reads a CSV file using a semicolon as the delimiter, showcasing CsvHelper’s flexibility.
Handling Headers and Mapping
When dealing with CSV files, headers often require special handling, especially when they do not match your class properties.
- Example of Custom Mapping:
“`csharp
using CsvHelper.Configuration;
public class PersonMap : ClassMap
{
public PersonMap()
{
Map(m => m.Name).Name(“FullName”); // Maps FullName in CSV to Name property
Map(m => m.Age).Name(“YearsOld”); // Maps YearsOld in CSV to Age property
}
}
public void ReadCsvWithMapping()
{
using (var reader = new StreamReader(“path/to/file.csv”))
using (var csv = new CsvReader(reader, CultureInfo.InvariantCulture))
{
csv.Context.RegisterClassMap
var records = csv.GetRecords
foreach (var record in records)
{
Console.WriteLine($”{record.Name}, {record.Age}”);
}
}
}
“`
This example demonstrates how to use custom mappings to align CSV headers with class properties accurately. The `ClassMap` allows for greater control over data representation.
Error Handling
When reading CSV files, it is crucial to implement error handling to manage potential issues such as malformed data or missing fields.
- Try-Catch Example:
“`csharp
public void ReadCsvWithErrorHandling()
{
try
{
using (var reader = new StreamReader(“path/to/file.csv”))
using (var csv = new CsvReader(reader, CultureInfo.InvariantCulture))
{
var records = csv.GetRecords
foreach (var record in records)
{
Console.WriteLine($”{record.Name}, {record.Age}”);
}
}
}
catch (CsvHelperException ex)
{
Console.WriteLine($”Error reading CSV: {ex.Message}”);
}
}
“`
This implementation ensures that any issues encountered during the reading process are caught, allowing for appropriate error messages to be displayed.
Expert Insights on Reading CSV Files in C
Dr. Emily Chen (Senior Software Engineer, Data Solutions Inc.). “When reading CSV files in C, utilizing the built-in `TextFieldParser` class from the `Microsoft.VisualBasic.FileIO` namespace can significantly simplify the process. This class effectively handles various delimiters and quoted fields, making it a robust choice for parsing complex CSV files.”
Mark Thompson (Lead Developer, Tech Innovations Group). “For developers looking for performance and flexibility, using libraries such as CsvHelper or CsvReader is highly recommended. These libraries not only streamline the reading process but also provide powerful mapping capabilities for converting CSV data directly into Cobjects.”
Linda Martinez (Data Analyst, Insight Analytics). “It is crucial to consider the encoding of the CSV file when reading it in C. Using the correct `StreamReader` encoding ensures that special characters are handled properly, preventing data loss or corruption during the import process.”
Frequently Asked Questions (FAQs)
How can I read a CSV file in C?
You can read a CSV file in Cusing the `StreamReader` class to read the file line by line, then split each line using the `String.Split` method based on the comma delimiter.
What libraries are available for reading CSV files in C?
Several libraries are available, including CsvHelper, FileHelpers, and the built-in `System.IO` namespace. CsvHelper is particularly popular due to its ease of use and robust features.
Can I read a CSV file without using third-party libraries?
Yes, you can read a CSV file without third-party libraries by using `StreamReader` and manually parsing the data. However, using a library can simplify the process and handle edge cases more effectively.
How do I handle different delimiters in a CSV file?
To handle different delimiters, you can specify the delimiter when splitting the string. For example, use `line.Split(‘;’)` for semicolon-delimited files or configure the settings in libraries like CsvHelper.
What should I do if my CSV file contains headers?
If your CSV file contains headers, you can read the first line separately to extract the header names. After that, you can read the remaining lines and map the values to the corresponding headers.
Is it possible to read large CSV files efficiently in C?
Yes, you can read large CSV files efficiently by using `StreamReader` in combination with asynchronous reading methods or by processing the file in chunks to minimize memory usage.
In summary, reading a CSV file in Ccan be accomplished through various methods, each suited to different needs and complexities. The most common approaches include using built-in classes like `StreamReader` for simple file reading, or leveraging libraries such as CsvHelper or Microsoft.VisualBasic.FileIO for more advanced parsing capabilities. These libraries provide robust features, including handling different delimiters, data types, and even mapping CSV data to Cobjects, which can significantly streamline the process of data manipulation.
Another important aspect to consider is error handling when reading CSV files. Implementing proper exception handling ensures that your application can gracefully manage issues such as file not found errors, format mismatches, or data conversion problems. This consideration is crucial for maintaining the reliability and stability of applications that depend on external data sources.
Ultimately, the choice of method for reading CSV files in Cshould align with the specific requirements of the project, including performance considerations, ease of use, and the complexity of the data being processed. By understanding the available options and their respective advantages, developers can effectively integrate CSV file reading into their applications, enhancing data management and processing capabilities.
Author Profile
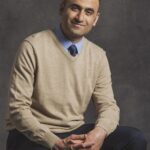
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?