Why Am I Getting a ‘C Undefined Reference to Function’ Error and How Can I Fix It?
In the world of C programming, encountering errors is an inevitable part of the development process. Among the myriad of errors that can arise, the dreaded ” reference to function” stands out as a particularly perplexing issue for both novice and seasoned developers alike. This error message often appears during the linking stage of compilation, leaving programmers scratching their heads as they attempt to decipher its meaning and implications. Understanding the root causes of this error is crucial for anyone looking to write efficient, error-free code and successfully navigate the complexities of C programming.
When you see the ” reference to function” error, it typically indicates that the compiler has successfully parsed your code but is unable to find the implementation of a function you’ve declared. This can occur for a variety of reasons, including missing source files, incorrect function signatures, or even simple typographical errors. As you delve deeper into the nuances of C programming, recognizing the common pitfalls that lead to this error will empower you to troubleshoot effectively and enhance your coding skills.
Moreover, addressing this error not only resolves immediate compilation issues but also enriches your understanding of the C language’s compilation and linking processes. By exploring the underlying principles of function declarations, definitions, and the organization of code into separate files, you’ll gain valuable insights that will serve
Understanding the Reference Error
In C programming, encountering an ” reference” error usually indicates that the linker cannot find the definition of a function that has been declared or called in your code. This error typically occurs during the linking phase of compilation, as the compiler successfully compiles individual source files but fails to link them together correctly.
Common reasons for this error include:
- Missing Function Definition: The function may be declared in a header file but not defined in any source file.
- Incorrect Linker Options: The object files or libraries containing the function definitions may not be included in the linking command.
- Typographical Errors: A mismatch in function names, parameters, or signatures between the declaration and definition.
- Scope Issues: The function might be defined in a scope that is not accessible to the calling code.
To address the reference error, consider the following steps:
- Ensure that all functions being called are properly defined.
- Verify that all necessary object files and libraries are linked during the build process.
- Check for spelling errors in function names and ensure that parameter types match.
- If using external libraries, confirm that the library is correctly included and that the linker options are correctly set.
Common Scenarios Leading to Reference Errors
Several scenarios can lead to reference errors. Below are some typical cases and solutions:
Scenario | Solution |
---|---|
Function declared but not defined | Provide the function definition in a source file. |
Typo in function name | Correct the spelling in both declaration and definition. |
Wrong parameters or return type | Ensure that the function signature matches across all declarations and definitions. |
Missing library during linking | Add the required library using the appropriate linker flag. |
Object files not included in the build | Include all relevant object files in the linking command. |
Debugging Reference Errors
When debugging reference errors, a systematic approach can help identify the root cause. Follow these guidelines:
- Check Compiler Output: Review the output from the compiler to identify the specific function causing the error.
- Use Verbose Linker Flags: Compile with verbose flags (e.g., `-Wl,–trace` for GCC) to get detailed information about the linking process.
- Inspect Header Files: Ensure that header files are correctly included and do not contain any inconsistencies.
- Run Dependency Analysis: Tools like `nm` can be used to check the symbols in object files and libraries to verify if the function is present.
By following these practices, developers can effectively diagnose and resolve reference errors, leading to successful compilation and linking of C programs.
Common Causes of References in C
reference errors in C typically occur during the linking phase of the compilation process. This can be attributed to several common issues:
- Missing Function Definitions: If a function is declared in a header file but not defined in any source file, the linker will not find the implementation.
- Incorrect Linkage of Object Files: When compiling multiple source files, if the object file containing the function definition is not included in the linking stage, an reference error will arise.
- Mismatched Function Signatures: A function may be defined with a different signature than its declaration. This includes differences in return type, parameter types, or the number of parameters.
- Static Functions: Functions declared as static in one source file cannot be accessed from other files, leading to references if called externally.
- Conditional Compilation: If a function definition is wrapped in preprocessor conditionals that are not satisfied during compilation, the linker will fail to find the function.
How to Resolve Reference Errors
To address reference errors, follow these steps:
- Check Function Definitions:
- Ensure every function declared in header files has a corresponding definition in at least one source file.
- Verify Object Files:
- When using multiple source files, ensure all necessary object files are included in the linking command. For example, in a command-line compilation:
“`
gcc main.o utility.o -o myprogram
“`
- Confirm Function Signatures:
- Check that function signatures in declarations and definitions match exactly, including return types and parameters.
- Inspect Static Functions:
- If a function is meant to be used across multiple files, avoid declaring it as static. If it must remain static, ensure it is only called within the same source file.
- Review Conditional Compilation:
- Ensure that preprocessor directives are correctly set so that all necessary functions are compiled. This may involve checking macro definitions and flags.
Tools and Techniques for Debugging
Utilizing certain tools and techniques can help troubleshoot reference errors effectively:
- Compiler Warnings: Compile with warnings enabled using flags such as `-Wall` or `-Werror` to catch potential issues early.
- Verbose Linking: Use the `-Wl,–trace` option with the linker to trace the linking process and identify missing references.
- nm Command: Use the `nm` command on object files to inspect symbols:
“`
nm myfile.o
“`
- Dependency Check: Utilize tools like `make` to manage dependencies in large projects, ensuring all files are compiled appropriately.
- Linker Scripts: For complex projects, consider using linker scripts to explicitly manage input and output sections.
Examples of Reference Errors
The following table outlines common scenarios leading to reference errors along with example code snippets:
Scenario | Code Example | Description |
---|---|---|
Missing Definition | `void foo();` | Declaration without a definition causes error. |
Incorrect Linking | `gcc main.o -o myprogram` | Missing `utility.o` where `foo()` is defined. |
Mismatched Signature | `int foo(int);` followed by `float foo(int)` | Function signature mismatch leads to error. |
Static Function Misuse | `static void bar();` in `file1.c` and called in `file2.c` | Static function cannot be accessed externally. |
Conditional Compilation Issue | `ifdef DEBUG void debugFunc(); endif` | `debugFunc` not defined if DEBUG is not set. |
Understanding the ‘ Reference to Function’ Error in C
Dr. Emily Carter (Senior Software Engineer, Code Solutions Inc.). “The ‘ reference to function’ error in C typically arises when the compiler cannot locate the definition of a function that has been declared. This often occurs when the source file containing the function implementation is not linked correctly during the build process.”
Mark Thompson (Lead Developer, Open Source Projects). “One common pitfall developers encounter is forgetting to include the appropriate object files or libraries in the linking stage. Ensuring that all necessary files are included in the compilation command can help mitigate this issue.”
Lisa Nguyen (C Programming Instructor, Tech Academy). “It’s crucial to maintain consistent function declarations and definitions. A mismatch in function signatures or using incorrect namespaces can lead to this error, so developers should always double-check their function prototypes.”
Frequently Asked Questions (FAQs)
What does ” reference to function” mean in C?
This error indicates that the linker cannot find the definition of a function that has been declared and called in your code. It typically occurs when the function is either not implemented or the object file containing the implementation is not linked correctly.
How can I resolve an ” reference” error?
To resolve this error, ensure that the function is implemented in one of your source files. If it is implemented in a separate file, make sure to include that file during the linking process, either by compiling it together or by specifying it in the build command.
Can this error occur due to missing libraries?
Yes, this error can occur if you are using functions from external libraries that are not linked properly. Ensure that you have included the correct library flags in your compilation command, such as `-l
What should I check if I receive this error for a standard library function?
If you receive this error for a standard library function, verify that you are including the correct header files and linking against the appropriate libraries. Ensure your development environment is set up correctly and that the libraries are installed.
Does the order of files in the compile command affect this error?
Yes, the order of files in the compile command can affect linking. The linker processes files in the order they are specified, so if a file that defines a function is placed after a file that calls that function, the linker may not find the definition, resulting in an ” reference” error.
Can I encounter this error when using function pointers?
Yes, if you declare a function pointer without providing a matching function definition, or if the function definition is not linked, you will encounter an ” reference” error when trying to use that function pointer. Ensure that all function pointers point to valid function implementations.
The issue of ” reference to function” in C programming typically arises during the linking stage of the compilation process. This error indicates that the compiler has encountered a function call for which it cannot find a corresponding definition. This situation often occurs due to several common reasons, such as missing function implementations, incorrect function declarations, or improper linkage of source files. Understanding these factors is crucial for effectively resolving the error and ensuring successful compilation of C programs.
One of the primary takeaways is the importance of ensuring that all functions are properly defined and linked. Developers should verify that all source files containing function definitions are included in the compilation command. Additionally, it is essential to check that function prototypes are correctly declared in header files, which can help prevent discrepancies between declarations and definitions. This practice not only aids in avoiding references but also enhances code readability and maintainability.
Moreover, the use of appropriate compilation flags can assist in diagnosing linking issues. For instance, using flags such as `-Wl,–no-as-needed` can help identify missing symbols during the linking stage. Furthermore, adopting a modular programming approach, where functions are organized into separate files with clear interfaces, can reduce the likelihood of encountering references. By adhering to these best practices, developers
Author Profile
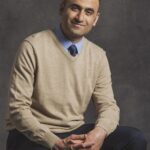
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?