How Can You Call an Async Function from a Non-Async Context?
In the ever-evolving landscape of modern programming, asynchronous programming has emerged as a powerful paradigm, allowing developers to write more efficient and responsive applications. However, as we embrace the benefits of async functions, a common challenge arises: how do we call these async functions from a non-async context? This question is not just a technical hurdle; it represents a fundamental shift in how we think about executing tasks in a non-blocking manner. Whether you’re a seasoned developer or just starting your journey, understanding this concept is crucial for harnessing the full potential of asynchronous programming.
When you delve into the world of async functions, you’ll discover that they are designed to operate independently of the main execution thread, enabling smoother user experiences and better resource management. However, this independence can create complications when trying to invoke these functions from traditional synchronous code. The interplay between async and non-async contexts can lead to confusion, particularly for those unfamiliar with JavaScript’s event loop and promise mechanisms.
Navigating this landscape requires a solid grasp of how to bridge the gap between these two paradigms. By exploring various techniques and best practices, you can learn how to effectively call async functions from non-async code, ensuring that your applications remain responsive and efficient. Join us as we unravel the intricacies of this
Understanding Asynchronous Functions
Asynchronous functions are integral in modern programming, particularly in JavaScript. They allow developers to write non-blocking code, enabling the execution of long-running tasks, such as network requests, without freezing the application. An `async` function always returns a promise, which can be handled with `.then()` or `await` syntax.
To call an async function, the caller must be aware of its asynchronous nature. If a function is defined as `async`, it can utilize the `await` keyword to pause execution until a promise is resolved. However, calling an async function from a non-async context can lead to challenges, as the non-async function will not inherently wait for the promise to resolve.
Calling an Async Function from Non-Async Code
To call an async function from a non-async context, you can use one of the following methods:
– **Using `.then()`**: You can call the async function and chain a `.then()` method to handle the resolved value.
– **Using `Promise` directly**: You can call the async function, which returns a promise, and handle it using `Promise.then()`.
Here’s an example illustrating both methods:
“`javascript
async function asyncFunction() {
return “Hello, World!”;
}
function nonAsyncFunction() {
// Using .then()
asyncFunction().then(result => {
console.log(result); // Output: Hello, World!
});
// Using Promise directly
const promise = asyncFunction();
promise.then(result => {
console.log(result); // Output: Hello, World!
});
}
“`
Considerations for Async Calls
When calling async functions from non-async code, consider the following:
- Error Handling: Use `.catch()` to handle any errors that may arise during the async operation.
- Execution Timing: Be aware that the non-async function will not wait for the async function to complete unless you explicitly handle the promise.
Example of Error Handling
Here is an example of how to implement error handling when calling an async function:
“`javascript
async function fetchData() {
throw new Error(“Data fetch failed”);
}
function nonAsyncFunctionWithErrorHandling() {
fetchData()
.then(data => {
console.log(data);
})
.catch(error => {
console.error(“Error:”, error.message); // Output: Error: Data fetch failed
});
}
“`
Comparison of Methods
The following table summarizes the methods to call async functions from non-async contexts:
Method | Syntax | Use Case |
---|---|---|
.then() | asyncFunction().then(result => { /* handle result */ }); | When you want to handle the result of the async operation directly. |
Promise | const promise = asyncFunction(); promise.then(result => { /* handle result */ }); | When you need to store the promise for further processing. |
By understanding these methods and considerations, you can effectively manage async operations within non-async code, ensuring robust and responsive applications.
Understanding Async Functions
Async functions are a fundamental part of modern JavaScript, allowing developers to write asynchronous code that is easier to read and maintain. These functions return a promise, which is a placeholder for a value that is not yet available. The typical syntax includes the `async` keyword before the function declaration and the use of `await` to pause execution until the promise is resolved.
Invoking Async Functions from Non-Async Contexts
When you need to call an async function from a non-async context, you cannot use the `await` keyword directly, as it requires the surrounding function to be asynchronous. Instead, you have several options:
– **Using `.then()`**: You can call the async function and handle the returned promise using the `.then()` method.
“`javascript
async function fetchData() {
const response = await fetch(‘https://api.example.com/data’);
return response.json();
}
function nonAsyncFunction() {
fetchData().then(data => {
console.log(data);
}).catch(error => {
console.error(‘Error fetching data:’, error);
});
}
“`
– **Using `Promise` constructor**: This method allows wrapping the async call inside a new promise.
“`javascript
function nonAsyncFunction() {
new Promise((resolve, reject) => {
fetchData().then(resolve).catch(reject);
}).then(data => {
console.log(data);
}).catch(error => {
console.error(‘Error:’, error);
});
}
“`
– **Immediate Invocation**: You can also use an immediately invoked async function expression (IIFE) within the non-async function.
“`javascript
function nonAsyncFunction() {
(async () => {
try {
const data = await fetchData();
console.log(data);
} catch (error) {
console.error(‘Error:’, error);
}
})();
}
“`
Handling Errors in Async Calls
When calling async functions from non-async contexts, proper error handling is essential. Use the following methods:
- `.catch()` method: Attach a `.catch()` to handle errors from the promise.
- Try-Catch in IIFE: Using a try-catch block inside the IIFE effectively catches synchronous and asynchronous errors.
Method | Description |
---|---|
`.then()` with `.catch()` | Handles success and failure separately. |
Try-Catch in IIFE | Catches errors within the async function context. |
Performance Considerations
When invoking async functions from non-async contexts, consider the following performance impacts:
- Blocking UI: Although JavaScript is single-threaded, long-running synchronous code can block the UI. Use async functions to avoid this.
- Promise Resolution: Chaining promises can lead to performance overhead if not managed correctly. Aim to keep the promise chains short and efficient.
By understanding these mechanisms, developers can effectively manage async function calls from non-async environments while maintaining code clarity and performance.
Expert Insights on Calling Async Functions from Non-Async Contexts
Jane Thompson (Senior Software Engineer, Tech Innovations Inc.). “In JavaScript, calling an async function from a non-async function requires careful handling of the returned promise. You can invoke the async function and handle the promise using `.then()` and `.catch()`, ensuring that any errors are appropriately managed.”
Michael Chen (Lead Developer, Future Tech Solutions). “While it is technically possible to call an async function from a non-async context, it is crucial to understand that the execution will not wait for the async function to complete. This can lead to unexpected behavior if the non-async function relies on the results of the async call.”
Sarah Patel (JavaScript Architect, CodeCraft Academy). “To effectively call an async function from a non-async context, consider wrapping the async call in an IIFE (Immediately Invoked Function Expression). This allows you to use `await` within the IIFE while keeping the outer function synchronous.”
Frequently Asked Questions (FAQs)
Can I call an async function from a non-async function?
Yes, you can call an async function from a non-async function, but you will not be able to use the `await` keyword. Instead, you should handle the returned promise appropriately.
What happens if I call an async function without awaiting it?
If you call an async function without awaiting it, the function will execute asynchronously, and the calling code will continue executing without waiting for the async function to complete.
How can I handle the result of an async function in a non-async context?
You can handle the result of an async function in a non-async context by using `.then()` to process the promise returned by the async function. This allows you to manage the result once it resolves.
Is it possible to use async/await in a non-async function?
No, you cannot directly use `await` in a non-async function. To utilize `await`, the function must be declared as async.
What is the best practice for calling async functions from non-async functions?
The best practice is to use the async function within a promise chain or to wrap the async call in an immediately invoked async function expression (IIFE) to maintain clarity and manage the asynchronous behavior properly.
Can I catch errors from an async function called in a non-async function?
Yes, you can catch errors by attaching a `.catch()` method to the promise returned by the async function. This allows you to handle any exceptions that may occur during its execution.
Calling an asynchronous function from a non-asynchronous context is a common scenario in programming, particularly in JavaScript. Asynchronous functions, defined with the `async` keyword, return a promise, which allows for non-blocking execution. When invoking these functions from a synchronous context, it is essential to handle the promise appropriately to avoid unhandled promise rejections or unexpected behavior.
To effectively call an async function from a non-async context, developers can use the `.then()` method to handle the promise returned by the async function. This approach allows for chaining additional operations that depend on the result of the async function. Alternatively, using an immediately invoked async function expression (IIFE) can also be a viable solution, enabling the use of `await` within a self-contained block of code.
Key takeaways from this discussion include the importance of understanding the distinction between synchronous and asynchronous code execution. Properly managing promises ensures that the application remains responsive and avoids potential pitfalls associated with unhandled promises. By leveraging techniques such as promise chaining or IIFE, developers can seamlessly integrate asynchronous operations into their synchronous code flows, enhancing the overall functionality of their applications.
Author Profile
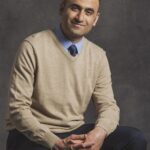
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?