How Can You Call a Python Script from Another Python Script?
In the world of programming, efficiency and modularity are key to developing robust applications. Python, with its simplicity and versatility, allows developers to create scripts that can perform a multitude of tasks. However, as projects grow in complexity, the need to organize and reuse code becomes increasingly important. This is where the ability to call one Python script from another comes into play. Whether you’re looking to streamline your workflow, enhance code reusability, or simply manage larger projects, understanding how to effectively invoke one script from another can significantly elevate your programming prowess.
Calling a Python script from another script is not only a practical approach to code organization but also a powerful technique that can help you leverage existing functionalities without reinventing the wheel. By breaking your code into smaller, manageable components, you can enhance readability and maintainability, making it easier to debug and update your projects. This practice is especially beneficial in larger applications where different modules may need to interact with one another.
Moreover, this capability opens up a world of possibilities for collaboration and integration. By structuring your code into separate scripts, you can work on different components simultaneously, allowing for a more efficient development process. Additionally, it enables you to share and reuse code across various projects, fostering a more collaborative environment among developers. As we delve deeper
Methods to Call a Python Script from Another Python Script
There are several effective methods to call a Python script from another Python script, each suitable for different use cases. Here are the most commonly used approaches:
Using the `import` Statement
The simplest way to call a function or a class from another Python script is to use the `import` statement. This method allows you to access specific functions or classes defined in another script, provided that both scripts are in the same directory or the path to the script is included in the Python path.
Example:
Suppose you have two scripts: `script1.py` and `script2.py`.
“`python
script1.py
def hello():
print(“Hello from script1!”)
script2.py
import script1
script1.hello()
“`
This will output:
“`
Hello from script1!
“`
Using the `subprocess` Module
When you want to execute a Python script as a separate process, the `subprocess` module is the ideal choice. This method allows you to run scripts that might not be structured for import, or when you need to run a script in a different environment.
Example:
“`python
script2.py
import subprocess
subprocess.run([‘python’, ‘script1.py’])
“`
This will execute `script1.py` as a separate process. You can also pass arguments to the script as follows:
“`python
subprocess.run([‘python’, ‘script1.py’, ‘arg1’, ‘arg2’])
“`
Using `exec()` Function
The `exec()` function allows you to execute Python code dynamically. This can be useful for small scripts or snippets, but it is generally not recommended for larger scripts due to potential security risks and maintainability issues.
Example:
“`python
script1.py
code = “print(‘Hello from script1!’)”
script2.py
with open(‘script1.py’) as f:
exec(f.read())
“`
This will execute the content of `script1.py` directly within the context of `script2.py`.
Comparison of Methods
Method | Use Case | Pros | Cons |
---|---|---|---|
Import | Reusing functions or classes | Simple and clean | Requires proper structure and path |
Subprocess | Running scripts as separate processes | Independent execution | More overhead |
exec() | Dynamic execution of code | Flexible for small snippets | Security risks, harder to debug |
Each method outlined above has its advantages and disadvantages depending on your specific requirements. It is crucial to select the appropriate method based on factors such as the complexity of the scripts, the need for modularity, and performance considerations.
Using the `import` Statement
One of the most straightforward methods to call a Python script from another is by using the `import` statement. This approach allows you to access functions, classes, and variables defined in one script from another.
- Basic Import Syntax:
“`python
import script_name
“`
- Calling Functions:
If you have a function defined in `script_name.py`, you can call it as follows:
“`python
script_name.function_name()
“`
- Example:
Suppose you have a script named `math_operations.py` with a function called `add`. You can call it like this:
“`python
In main_script.py
import math_operations
result = math_operations.add(5, 3)
print(result) Output: 8
“`
Using the `exec()` Function
The `exec()` function can execute Python code dynamically. This method is less common but useful for specific scenarios where you need to run code from a script as a string.
- Example:
“`python
with open(‘script_name.py’) as file:
exec(file.read())
“`
This will execute all the code present in `script_name.py` in the current script’s context.
Using the `subprocess` Module
For cases where you need to run a script as a separate process, the `subprocess` module is ideal. This is particularly useful for scripts that might be larger or require their own execution environment.
- Basic Usage:
“`python
import subprocess
subprocess.run([‘python’, ‘script_name.py’])
“`
- Example with Arguments:
If you need to pass arguments to the script:
“`python
subprocess.run([‘python’, ‘script_name.py’, ‘arg1’, ‘arg2’])
“`
Using `from … import` Syntax
This variant of the import statement allows you to import specific functions or classes directly, making it easier to use them without prefixing the module name.
- Syntax:
“`python
from script_name import function_name
“`
- Example:
“`python
from math_operations import add
result = add(5, 3)
print(result) Output: 8
“`
Setting Up the Python Path
If the script you want to import is located in a different directory, you may need to adjust the Python path.
- Modifying `sys.path`:
“`python
import sys
sys.path.append(‘/path/to/directory’)
import script_name
“`
- Example:
“`python
import sys
sys.path.append(‘/path/to/my_scripts’)
import my_script
“`
Using Package Structure
For larger projects, consider organizing your scripts into packages. This allows for better modularity and reusability.
- Structure Example:
“`
my_project/
├── __init__.py
├── script_one.py
└── script_two.py
“`
- Importing from a Package:
“`python
from my_project import script_one
“`
By employing these methods, you can efficiently call Python scripts from one another, enhancing code organization and functionality.
Expert Insights on Calling Python Scripts from Another Python Script
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Utilizing the `import` statement is the most straightforward method to call a Python script from another. This allows for modular programming, where functions and classes can be reused efficiently across different scripts, enhancing code maintainability and readability.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “For more complex scenarios, such as needing to execute scripts in different environments or managing dependencies, using the `subprocess` module is advisable. This method provides greater control over the execution of the script and allows for capturing output and errors effectively.”
Sarah Thompson (Data Scientist, Analytics Hub). “When calling a Python script from another, it’s crucial to consider the context in which the scripts run. If they share the same environment, `import` is ideal. However, if they require separate environments, leveraging virtual environments along with `subprocess` can prevent conflicts and maintain clean dependencies.”
Frequently Asked Questions (FAQs)
How can I call a Python script from another Python script?
You can call a Python script from another script using the `import` statement or by using the `subprocess` module. The `import` statement allows you to access functions and classes defined in another script, while `subprocess` enables you to run the script as a separate process.
What is the difference between using `import` and `subprocess`?
Using `import` allows you to directly access and utilize functions and variables defined in the other script within the same Python runtime. In contrast, `subprocess` runs the script as a separate process, which is useful for executing scripts independently or when you need to run a script that is not structured as a module.
Can I pass arguments to the script being called?
Yes, you can pass arguments to a script using the `subprocess` module by providing a list of arguments. When using `import`, you can define functions in the called script that accept parameters and invoke them with the desired arguments.
What should I do if the script I want to call is not in the same directory?
If the script is not in the same directory, you can modify the Python path using `sys.path.append(‘/path/to/script’)` before importing it. Alternatively, you can specify the full path in the `subprocess` call.
Is it possible to capture the output of the called script?
Yes, when using the `subprocess` module, you can capture the output by setting the `stdout` parameter to `subprocess.PIPE`. This allows you to retrieve the output of the script after it has executed.
What are some common errors to watch for when calling scripts?
Common errors include `ModuleNotFoundError` when using `import`, which indicates that the script cannot be found, and `FileNotFoundError` when using `subprocess`, which suggests that the specified script path is incorrect. Additionally, ensure that the called script has the appropriate permissions to execute.
In summary, calling a Python script from another Python script is a common practice that can enhance modularity and reusability in programming. There are several methods to achieve this, including using the `import` statement, the `subprocess` module, and executing scripts through the command line. Each method serves different use cases and offers unique advantages, depending on the complexity and requirements of the task at hand.
Importing a script allows for direct access to its functions and classes, promoting code organization and reducing redundancy. This approach is particularly effective when the scripts are closely related and require frequent interaction. On the other hand, using the `subprocess` module provides a way to run scripts as separate processes, which can be beneficial for tasks that need to run independently or require different environments.
Key takeaways from this discussion include the importance of understanding the context in which one intends to call another script. Factors such as the need for data sharing, performance considerations, and error handling should guide the choice of method. Additionally, leveraging the command line can be useful for quick executions and testing, making it a versatile option for developers. Ultimately, selecting the appropriate method will lead to more efficient and maintainable code.
Author Profile
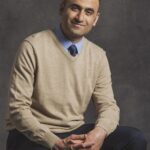
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?