How Can You Call a PowerShell Script from Another PowerShell Script?
In the world of automation and system administration, PowerShell stands out as a powerful tool for managing and configuring Windows environments. As scripts become more complex and multifaceted, the need to modularize code and enhance reusability becomes paramount. One effective way to achieve this is by calling a PowerShell script from another PowerShell script. This technique not only streamlines workflows but also promotes cleaner, more organized code, making it easier to maintain and update over time.
Calling a PowerShell script from another script allows you to break down tasks into manageable components, enabling you to build a library of reusable scripts that can be invoked as needed. This modular approach not only saves time but also reduces the likelihood of errors by allowing you to test individual scripts in isolation. Whether you’re automating routine tasks, managing system configurations, or orchestrating complex deployments, understanding how to effectively call scripts can significantly enhance your scripting capabilities.
As we delve deeper into this topic, we will explore the various methods available for invoking scripts, the parameters that can be passed between them, and best practices for ensuring seamless execution. By mastering this skill, you’ll empower yourself to create more efficient and robust PowerShell solutions that can adapt to the ever-evolving demands of your IT environment.
Methods to Call a PowerShell Script from Another PowerShell Script
Calling a PowerShell script from another PowerShell script can be achieved through various methods, each suitable for different scenarios. Understanding these methods will allow you to integrate scripts effectively, promoting code reuse and modularity.
Using the & Call Operator
The simplest way to call another PowerShell script is by using the call operator (`&`). This operator allows you to run a script or a command within the current scope.
“`powershell
& “C:\Path\To\Your\Script.ps1”
“`
This method can be particularly useful when you want to execute a script and immediately use its output or effects in the calling script.
Using the Dot Sourcing Method
Dot sourcing is another technique that runs a script in the current scope, allowing any variables or functions defined in the called script to be available in the calling script.
“`powershell
. “C:\Path\To\Your\Script.ps1”
“`
In this case, the dot followed by a space (`. `) before the script path is crucial. This allows for better integration of scripts, especially when you need to manipulate or access variables defined in the sourced script.
Passing Arguments to Scripts
When calling a script, it’s often necessary to pass arguments to it. This can be done by specifying the parameters after the script path.
“`powershell
& “C:\Path\To\Your\Script.ps1” -Param1 “Value1” -Param2 “Value2”
“`
Ensure that the called script is designed to accept parameters, which can be defined at the beginning of the script using the `param` block:
“`powershell
param (
[string]$Param1,
[string]$Param2
)
“`
Using Invoke-Expression
The `Invoke-Expression` cmdlet can also be used to run scripts dynamically. This method evaluates a string as a command, which can be useful in situations where the script path is constructed at runtime.
“`powershell
Invoke-Expression “C:\Path\To\Your\Script.ps1”
“`
However, this method is generally less preferred due to security concerns related to executing arbitrary strings as commands.
Table of Method Comparisons
Method | Scope | Parameter Passing | Use Case |
---|---|---|---|
& Call Operator | Current | Yes | Simple execution |
Dot Sourcing | Current | Yes | Variable/function reuse |
Invoke-Expression | Current | Yes | Dynamic execution |
Selecting the appropriate method depends on the requirements of your scripts, including the need for variable scope, the complexity of parameter passing, and security considerations.
Methods to Call a PowerShell Script from Another PowerShell Script
To execute a PowerShell script from another script, several methods are available, each with its own use cases and considerations. Below are the most common methods.
Using the Dot Sourcing Method
Dot sourcing allows you to run a script in the current scope, meaning any variables or functions defined in the called script are available in the calling script.
“`powershell
. “C:\Path\To\Your\Script.ps1”
“`
- Pros:
- Variables and functions are retained in the current session.
- Useful for modular scripting where shared functions are needed.
- Cons:
- Can lead to namespace pollution if not managed properly.
Using the Call Operator (&)
The call operator (`&`) executes a script in its own scope, meaning it does not inherit variables from the calling script.
“`powershell
& “C:\Path\To\Your\Script.ps1”
“`
- Pros:
- Keeps the scopes separate, minimizing variable conflicts.
- Ideal for scripts that do not need to share state.
- Cons:
- Variables defined in the called script will not be accessible afterward.
Passing Parameters to Scripts
When calling another script, you can pass parameters to it, allowing for more dynamic behavior.
“`powershell
& “C:\Path\To\Your\Script.ps1” -Param1 “Value1” -Param2 “Value2”
“`
- Defining Parameters in the Called Script:
In the called script, define parameters at the beginning:
“`powershell
param (
[string]$Param1,
[string]$Param2
)
“`
Using Start-Process
The `Start-Process` cmdlet is useful when you want to run a script in a new process, which can be beneficial for scripts that require isolation or when you want to run scripts asynchronously.
“`powershell
Start-Process powershell -ArgumentList “-File ‘C:\Path\To\Your\Script.ps1′”
“`
- Pros:
- Runs the script in a separate PowerShell session.
- Allows for asynchronous execution.
- Cons:
- No shared variables or state between processes.
Example Scenario: Calling Scripts with Parameters
Consider you have two scripts: `MainScript.ps1` and `ChildScript.ps1`.
ChildScript.ps1:
“`powershell
param (
[string]$Name
)
Write-Host “Hello, $Name!”
“`
MainScript.ps1:
“`powershell
& “C:\Path\To\ChildScript.ps1” -Name “World”
“`
This will output: `Hello, World!`.
Best Practices
- Always use full paths to avoid ambiguity, especially in larger scripts.
- Use consistent naming conventions for scripts and parameters.
- Consider error handling with `try/catch` blocks when calling scripts to manage execution failures gracefully.
- Document the purpose and parameters of each script to enhance maintainability.
By employing these methods, you can effectively manage the execution and interaction of multiple PowerShell scripts, enhancing your scripting capabilities and overall efficiency.
Expert Insights on Calling PowerShell Scripts from Another PowerShell Script
Jessica Lee (Senior Systems Administrator, Tech Solutions Inc.). “When calling a PowerShell script from another script, it is crucial to ensure that the execution policy allows for script execution. Using the `&` operator is a straightforward method to invoke another script, which can help maintain modularity and reusability in your code.”
Michael Chen (PowerShell Automation Specialist, Cloud Innovations). “Utilizing the `Start-Process` cmdlet can be beneficial when you need to run a script asynchronously. This approach allows for better resource management and can enhance the performance of your automation tasks, especially in larger environments.”
Sarah Thompson (IT Security Consultant, SecureTech Advisors). “It is essential to consider security implications when calling scripts. Always validate the scripts being executed and ensure they come from trusted sources to mitigate risks associated with script injection or execution of malicious code.”
Frequently Asked Questions (FAQs)
How can I call a PowerShell script from another PowerShell script?
You can call a PowerShell script from another script by using the `&` (call operator) followed by the path to the script. For example, `& “C:\Path\To\YourScript.ps1″`.
Can I pass parameters to a PowerShell script when calling it from another script?
Yes, you can pass parameters by including them after the script path. For example, `& “C:\Path\To\YourScript.ps1” -Param1 Value1 -Param2 Value2`.
What happens if the called script has its own parameters defined?
The called script will execute with the parameters you provide. Ensure that the parameter names match those defined in the called script to avoid errors.
Is it possible to call a script located on a remote machine?
Yes, you can call a script on a remote machine using PowerShell Remoting. Use the `Invoke-Command` cmdlet, specifying the remote machine and the script path.
What should I do if the called script fails to execute?
Check for errors in the script path, ensure the script has execution permissions, and verify that any required modules or dependencies are available. Use `Try-Catch` blocks for error handling.
Can I call a script and capture its output in the calling script?
Yes, you can capture the output by assigning the call to a variable. For example, `$output = & “C:\Path\To\YourScript.ps1″`. This allows you to use the output further in your calling script.
Calling a PowerShell script from another PowerShell script is a straightforward process that enhances modularity and reusability in scripting. This technique allows developers to break down complex tasks into smaller, manageable scripts, which can be executed independently or in conjunction with other scripts. By utilizing the `&` operator or the `Invoke-Expression` cmdlet, users can effectively execute external scripts while maintaining the context of the calling script.
Moreover, passing parameters between scripts is a crucial aspect of this process. By defining parameters in the called script, users can customize its behavior based on the input provided from the calling script. This not only promotes flexibility but also allows for dynamic script execution tailored to specific needs. Implementing error handling and ensuring that scripts are executed in the correct context further enhances the reliability of this approach.
mastering the technique of calling one PowerShell script from another is essential for efficient script management and execution. By leveraging this capability, users can create more organized and maintainable codebases. Additionally, understanding how to pass parameters and handle errors will significantly improve the robustness of automation tasks in PowerShell, ultimately leading to better productivity and streamlined workflows.
Author Profile
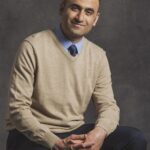
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?