How Can I Implement a Camera Using Quaternions in C with OpenGL?
In the realm of computer graphics and game development, the quest for smooth and realistic camera movements is paramount. Traditional methods of camera control often fall short when it comes to representing complex rotations without suffering from issues like gimbal lock. Enter quaternions—a mathematical construct that not only simplifies the representation of 3D rotations but also enhances the overall fluidity of camera dynamics in OpenGL applications. This article delves into the fascinating world of quaternion-based camera systems, exploring their advantages, implementation techniques, and how they can elevate your graphics projects to new heights.
Quaternions, often regarded as the unsung heroes of 3D mathematics, provide a robust framework for handling rotations in a way that is both efficient and intuitive. Unlike Euler angles, which can lead to confusing rotational anomalies, quaternions offer a seamless solution that allows developers to interpolate between orientations smoothly. This makes them particularly well-suited for camera applications, where maintaining a natural perspective is crucial for user immersion. By leveraging quaternions, developers can create cameras that not only follow objects with precision but also respond dynamically to user inputs, resulting in a more engaging experience.
As we journey deeper into the mechanics of quaternion-based cameras in OpenGL, we will uncover the core principles that underpin this approach.
Understanding Quaternion Basics
Quaternions are a number system that extends complex numbers and are widely used in 3D graphics to represent rotations. A quaternion is typically expressed as:
\[ q = w + xi + yj + zk \]
where \( w \) is the scalar part and \( x, y, z \) are the vector components. Quaternions offer several advantages over traditional Euler angles and rotation matrices, including:
- No Gimbal Lock: Quaternions avoid the singularities associated with Euler angles.
- Smooth Interpolation: Quaternions enable smooth transitions between orientations, commonly utilized in animations and camera movements.
- Compact Representation: They require less memory and are computationally efficient for representing rotations.
Implementing a Quaternion-Based Camera in OpenGL
To implement a camera based on quaternions in OpenGL, the essential steps involve defining the camera’s position, direction, up vector, and the quaternion representing its orientation. The following components are pivotal in this setup:
- Camera Position: A 3D vector indicating the camera’s location in the scene.
- Camera Direction: A normalized vector pointing in the direction the camera is facing.
- Up Vector: A normalized vector defining the upward direction relative to the camera.
The transformation from the camera’s local space to world space can be achieved using a view matrix, which can be computed using the quaternion for rotation.
Quaternion to Rotation Matrix Conversion
To apply a quaternion for transforming the camera’s view, it must be converted to a rotation matrix. The conversion from a quaternion \( q = (w, x, y, z) \) to a rotation matrix can be computed using the following formula:
\[
\begin{bmatrix}
1 – 2y^2 – 2z^2 & 2xy – 2wz & 2xz + 2wy \\
2xy + 2wz & 1 – 2x^2 – 2z^2 & 2yz – 2wx \\
2xz – 2wy & 2yz + 2wx & 1 – 2x^2 – 2y^2
\end{bmatrix}
\]
This matrix can then be used to create the view matrix for OpenGL.
Sample Implementation in C
The following code snippet demonstrates how to implement a quaternion-based camera in C with OpenGL:
“`c
include
include
// Define the quaternion structure
typedef struct {
float w, x, y, z;
} Quaternion;
// Function to normalize the quaternion
void normalize(Quaternion* q) {
float norm = sqrt(q->w * q->w + q->x * q->x + q->y * q->y + q->z * q->z);
q->w /= norm;
q->x /= norm;
q->y /= norm;
q->z /= norm;
}
// Function to create a rotation quaternion
Quaternion createRotationQuaternion(float angle, float x, float y, float z) {
Quaternion q;
float halfAngle = angle * 0.5f;
q.w = cos(halfAngle);
q.x = x * sin(halfAngle);
q.y = y * sin(halfAngle);
q.z = z * sin(halfAngle);
normalize(&q);
return q;
}
// View matrix generation
void updateViewMatrix(Quaternion q, float posX, float posY, float posZ) {
// Convert quaternion to rotation matrix here and set the view matrix
// Use glLoadIdentity(), glRotatef(), glTranslatef() as needed
}
“`
Benefits of Using Quaternion-Based Camera
Using a quaternion-based approach for camera manipulation in OpenGL provides significant benefits:
- Enhanced Performance: Quaternions reduce the computational overhead associated with rotation calculations.
- Robust Handling of Rotations: Quaternions facilitate seamless and intuitive camera rotations, especially when combined with input devices.
- Flexibility in Implementation: Easily adaptable to various camera styles and behaviors, such as first-person or third-person perspectives.
This methodology allows for sophisticated camera control, providing a more immersive experience in 3D applications.
Aspect | Euler Angles | Quaternions |
---|---|---|
Gimbal Lock | Yes | No |
Smooth Interpolation | Complex | Simple |
Memory Usage | More | Less |
Quaternion Fundamentals for Camera Rotation
Quaternions are a mathematical representation that efficiently handles 3D rotations, avoiding gimbal lock and providing smooth interpolations. In the context of OpenGL and camera movement, they serve as a robust method for orienting the camera in a scene.
- Components of a Quaternion:
- w (real part): Scalar component.
- x, y, z (imaginary parts): Vector component representing the axis of rotation.
- Quaternion Representation:
- A quaternion \( q \) can be represented as:
\( q = w + xi + yj + zk \)
- Normalization:
- Quaternions must be normalized to maintain valid rotations. The normalization formula is:
\( \text{normalized}(q) = \frac{q}{||q||} \)
where \( ||q|| = \sqrt{w^2 + x^2 + y^2 + z^2} \)
Creating a Quaternion for Camera Orientation
To create a quaternion representing a rotation, you typically start with an angle-axis representation or Euler angles.
- Angle-Axis Representation:
- Given an axis of rotation \( \mathbf{v} \) (a unit vector) and an angle \( \theta \), the quaternion is calculated as follows:
\[
q = \left( \cos\left(\frac{\theta}{2}\right), v_x \sin\left(\frac{\theta}{2}\right), v_y \sin\left(\frac{\theta}{2}\right), v_z \sin\left(\frac{\theta}{2}\right) \right)
\]
- From Euler Angles:
- Convert Euler angles \( (\phi, \psi, \theta) \) to a quaternion using the following formulas:
\[
q_w = \cos\left(\frac{\phi}{2}\right) \cos\left(\frac{\psi}{2}\right) \cos\left(\frac{\theta}{2}\right) + \sin\left(\frac{\phi}{2}\right) \sin\left(\frac{\psi}{2}\right) \sin\left(\frac{\theta}{2}\right)
\]
\[
q_x = \sin\left(\frac{\phi}{2}\right) \cos\left(\frac{\psi}{2}\right) \cos\left(\frac{\theta}{2}\right) – \cos\left(\frac{\phi}{2}\right) \sin\left(\frac{\psi}{2}\right) \sin\left(\frac{\theta}{2}\right)
\]
\[
q_y = \cos\left(\frac{\phi}{2}\right) \sin\left(\frac{\psi}{2}\right) \cos\left(\frac{\theta}{2}\right) + \sin\left(\frac{\phi}{2}\right) \cos\left(\frac{\psi}{2}\right) \sin\left(\frac{\theta}{2}\right)
\]
\[
q_z = \cos\left(\frac{\phi}{2}\right) \cos\left(\frac{\psi}{2}\right) \sin\left(\frac{\theta}{2}\right) – \sin\left(\frac{\phi}{2}\right) \sin\left(\frac{\psi}{2}\right) \cos\left(\frac{\theta}{2}\right)
\]
Applying Quaternions to Camera Movement in OpenGL
The camera’s view matrix can be constructed using quaternions to set its orientation. This can be achieved through the following steps:
- Update the Camera Rotation:
- Use user inputs or predefined movements to adjust the camera’s quaternion.
- Convert Quaternion to Rotation Matrix:
- To apply the quaternion, convert it to a rotation matrix, which can be used in OpenGL:
\[
R = \begin{bmatrix}
1 – 2(q_y^2 + q_z^2) & 2(q_x q_y – q_z q_w) & 2(q_x q_z + q_y q_w) & 0 \\
2(q_x q_y + q_z q_w) & 1 – 2(q_x^2 + q_z^2) & 2(q_y q_z – q_x q_w) & 0 \\
2(q_x q_z – q_y q_w) & 2(q_y q_z + q_x q_w) & 1 – 2(q_x^2 + q_y^2) & 0 \\
0 & 0 & 0 & 1
\end{bmatrix}
\]
- Set the View Matrix:
- Pass the rotation matrix to OpenGL’s view matrix calculation to update the camera’s orientation:
“`c
glm::mat4 viewMatrix = glm::toMat4(cameraQuaternion);
glLoadMatrixf(glm::value_ptr(viewMatrix));
“`
By utilizing quaternions for camera orientation in OpenGL, you achieve smoother and more efficient rotations, enhancing the overall user experience in 3D environments.
Expert Insights on Camera Implementation Using Quaternions in C with OpenGL
Dr. Emily Carter (Computer Graphics Researcher, Visual Computing Institute). Quaternions provide a robust way to represent rotations in 3D space, which is essential for camera implementations in OpenGL. They avoid the gimbal lock issue that can occur with Euler angles, allowing for smooth and continuous camera movements, especially in complex scenes.
Michael Chen (Lead Software Engineer, Interactive Graphics Solutions). When implementing a camera system based on quaternions in C with OpenGL, it’s crucial to understand how to convert quaternion rotations into transformation matrices. This conversion is key to integrating the camera’s orientation into the rendering pipeline effectively.
Sarah Thompson (3D Graphics Programmer, GameDev Studios). Utilizing quaternions for camera control not only enhances performance but also simplifies the mathematics involved in 3D rotations. By leveraging libraries that support quaternion operations, developers can focus more on creative aspects rather than getting bogged down by complex calculations.
Frequently Asked Questions (FAQs)
What is a quaternion and how is it used in camera transformations?
A quaternion is a mathematical representation that extends complex numbers, consisting of one real part and three imaginary parts. In camera transformations, quaternions are used to represent rotations efficiently, avoiding issues like gimbal lock that can occur with Euler angles.
How do I implement a camera based on quaternions in OpenGL?
To implement a quaternion-based camera in OpenGL, you need to define the camera’s orientation using quaternions. You can convert the quaternion to a rotation matrix and apply it to the view matrix. This involves updating the camera’s position and orientation based on user input and then recalculating the view matrix for rendering.
What are the advantages of using quaternions over Euler angles for camera rotation?
Quaternions provide several advantages, including smooth interpolation between orientations (slerp), no gimbal lock, and more compact representation. They also allow for more efficient calculations when combining multiple rotations.
How can I interpolate between two quaternions for smooth camera movement?
To interpolate between two quaternions, use spherical linear interpolation (slerp). This method calculates intermediate orientations based on the shortest path on the unit sphere, ensuring smooth transitions between the two orientations.
What libraries can assist in quaternion operations for OpenGL?
Several libraries can assist with quaternion operations in OpenGL, including GLM (OpenGL Mathematics), Eigen, and DirectXMath. These libraries provide built-in support for quaternion manipulation, making it easier to integrate into your OpenGL projects.
Can I convert a quaternion to a rotation matrix in OpenGL?
Yes, you can convert a quaternion to a rotation matrix. The conversion involves using the quaternion components to populate a 4×4 rotation matrix, which can then be used to transform vertices in OpenGL. This is essential for applying the camera’s orientation to the scene.
In the context of computer graphics, particularly when using OpenGL, implementing a camera system based on quaternions offers several advantages over traditional Euler angles. Quaternions provide a robust way to represent rotations without suffering from gimbal lock, which can occur with Euler angles. This characteristic is crucial for applications requiring smooth and continuous camera movements, such as in 3D simulations and games.
Quaternions allow for efficient interpolation between orientations, enabling smooth transitions and animations. Techniques such as spherical linear interpolation (slerp) can be utilized to achieve fluid camera movements, enhancing the overall user experience. Additionally, quaternions are computationally efficient, which is essential for real-time rendering applications where performance is a key consideration.
When implementing a camera based on quaternions in OpenGL, it is important to understand how to convert quaternion representations into transformation matrices that OpenGL can utilize. This involves creating a rotation matrix from the quaternion and applying it to the view matrix. Properly managing the camera’s position and orientation using quaternions can lead to more intuitive controls and a more immersive experience for the user.
In summary, using quaternions for camera representation in OpenGL provides significant benefits in terms of rotation representation
Author Profile
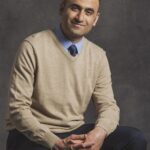
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?