Can a REP Prefix Stand Alone in Assembly Language: Understanding Its Usage?
In the intricate world of assembly language programming, every detail counts, and understanding the nuances of instruction prefixes can significantly impact the efficiency and functionality of your code. Among these prefixes, the REP (repeat) prefix stands out as a powerful tool that can enhance the performance of string operations and loops. However, a common question arises among both novice and seasoned programmers: can a REP prefix be used by itself in assembly? This inquiry delves into the fundamental aspects of assembly language design and the intended use of prefixes, setting the stage for a deeper exploration of their roles and limitations.
The REP prefix is primarily designed to modify the behavior of certain string manipulation instructions, allowing them to execute repeatedly until a specified condition is met. This functionality is particularly useful in operations that involve moving or comparing blocks of data, where efficiency is paramount. However, the question of whether the REP prefix can stand alone, without an accompanying instruction, raises important considerations about the structure and syntax of assembly language.
As we navigate through the intricacies of assembly programming, we will explore the implications of using prefixes like REP, their intended applications, and the potential pitfalls of misusing them. By understanding these concepts, programmers can harness the full power of assembly language, ensuring that their code is not only functional but
Understanding REP Prefix in Assembly
The REP prefix in assembly language is used to indicate that a specific instruction should be repeated a certain number of times, as defined by the CX (or ECX/RCX) register. However, the use of the REP prefix by itself is not valid in assembly programming. It must always be used in conjunction with certain string operations.
Usage of REP Prefix
When the REP prefix is employed, it modifies the behavior of string instructions, allowing them to process multiple elements in a single operation. The following instructions can utilize the REP prefix:
- MOVS: Move string data.
- CMPS: Compare string data.
- SCAS: Scan string data.
- LODS: Load string data.
- STOS: Store string data.
The REP prefix will repeat the operation until the CX register reaches zero.
Instruction | Description |
---|---|
MOVS | Moves data from the source address to the destination address. |
CMPS | Compares data at the source and destination addresses. |
SCAS | Scans through the string for a specific value. |
LODS | Loads a string from a specified address into the accumulator. |
STOS | Stores a string from the accumulator to a specified address. |
Examples of REP Prefix Usage
Here are some examples illustrating how the REP prefix is used in assembly language:
“`assembly
; Example of using REP with MOVSB
MOV CX, 5 ; Set the count to 5
LEA SI, Source ; Load effective address of source
LEA DI, Dest ; Load effective address of destination
REP MOVSB ; Repeat MOVSB instruction 5 times
“`
In the example above, the REP prefix allows the MOVSB instruction to execute five times, transferring a byte from the source to the destination each time.
Limitations of Using REP Prefix
While the REP prefix is powerful, it has specific limitations:
- Requires String Instructions: The prefix cannot be used independently; it must be paired with a string instruction.
- CX/ECX Dependency: The number of repetitions is dependent on the CX or ECX register, which must be initialized beforehand.
- Overhead: The prefix might introduce overhead in situations where a small number of repetitions is required, as the instruction must still check the count after each iteration.
Understanding the proper context and application of the REP prefix is crucial for effective assembly programming, as it enhances data processing capabilities while also requiring careful management of registers.
Usage of REP Prefix in Assembly
The REP prefix in assembly language is a powerful tool used primarily with string manipulation instructions. Its main purpose is to control the repetition of these instructions, allowing for efficient processing of arrays or strings. However, the question arises whether the REP prefix can be used by itself, without any accompanying instruction.
Understanding the REP Prefix
The REP prefix modifies specific string operations to repeat them based on the value in the CX register (or ECX/R8C for 32-bit or 64-bit modes respectively). The operations that can use the REP prefix include:
- MOVS: Move string
- LODS: Load string
- STOS: Store string
- SCAS: Scan string
- CMPS: Compare string
When the instruction is executed, the REP prefix decrements the CX register and continues executing the instruction until CX reaches zero.
Can REP Be Used Alone?
The REP prefix cannot be used by itself as a standalone instruction. It is designed to work in conjunction with specific string operations. Here are key points regarding its usage:
- Must Accompany an Instruction: The REP prefix requires a valid instruction to operate on; it cannot function independently.
- Syntax Requirement: The correct syntax must include both the REP prefix and the appropriate instruction. For example:
“`assembly
REP MOVSB
“`
- Error Handling: Attempting to use REP without an instruction will result in an assembler error, as the assembler expects a valid operation to follow the prefix.
Examples of REP Usage
Here are some examples that illustrate the correct use of the REP prefix in assembly:
Example Instruction | Description |
---|---|
`REP MOVSB` | Repeats the MOVSB instruction CX times. |
`REP LODSB` | Repeats the LODSB instruction CX times. |
`REP STOSB` | Repeats the STOSB instruction CX times. |
In each example, the REP prefix enhances the instruction’s functionality by controlling the number of repetitions based on the count in the CX register.
Performance Considerations
Utilizing the REP prefix can yield performance benefits, particularly in scenarios involving large data sets. Key considerations include:
- Efficiency: By using REP, the processor can execute multiple operations in a single instruction cycle, reducing the overhead of looping constructs in high-level languages.
- Code Size: The use of REP can significantly reduce code size compared to manually coding loops.
Conclusion on REP Usage
In summary, the REP prefix is a highly efficient assembly instruction modifier that cannot stand alone. It requires a corresponding operation to effectively function, thus forming an integral part of string manipulation in assembly programming. Understanding how to leverage this prefix can lead to optimized performance in assembly language applications.
Understanding the Use of REP Prefix in Assembly Language
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The REP prefix in assembly is specifically designed to enhance the performance of string operations. However, using it by itself, without the accompanying instruction, is not valid and will result in an error. It must always be paired with an instruction that supports repetition, such as MOVS or STOS.”
Michael Tran (Assembly Language Expert, CodeCraft Magazine). “While the REP prefix can technically be present in the code, it cannot function independently. It requires a corresponding instruction to dictate its behavior. Therefore, it is essential to understand the context in which REP is utilized.”
Lisa Chen (Computer Architecture Professor, University of Technology). “In assembly language programming, the REP prefix is a powerful tool for optimizing loops. However, it is not meaningful on its own; it must always be used in conjunction with a valid instruction to execute the intended repetitive action.”
Frequently Asked Questions (FAQs)
Can a rep prefix be used by itself in assembly?
No, a rep prefix cannot be used by itself; it must be combined with an instruction to indicate repetition of that instruction.
What is the purpose of the rep prefix in assembly language?
The rep prefix is used to repeat string operations, allowing for efficient processing of arrays or strings by executing the associated instruction multiple times.
Which instructions can be used with the rep prefix?
The rep prefix can be used with string manipulation instructions such as MOVS, CMPS, SCAS, and STOS, among others.
Are there different types of rep prefixes?
Yes, there are different types of rep prefixes, including REP, REPE (or REPZ), and REPNE (or REPNZ), each serving specific conditions for repetition.
How does the rep prefix affect the instruction pointer in assembly?
The rep prefix does not directly affect the instruction pointer; however, it influences the execution flow by repeating the associated instruction until a specified condition is met.
Can the rep prefix be used in conjunction with other prefixes?
Yes, the rep prefix can be combined with other instruction prefixes, such as operand size or address size prefixes, to modify the behavior of the instruction being repeated.
In assembly language programming, the use of a REP prefix is a common practice to streamline the execution of certain string operations. The REP prefix, which stands for “repeat,” is specifically designed to enhance the efficiency of instructions like MOVS, CMPS, SCAS, and others by allowing them to be executed multiple times in a single command. However, it is important to note that the REP prefix cannot be used in isolation; it must precede a valid instruction that supports repetition. This means that while the REP prefix is a powerful tool for optimizing code, it cannot function independently without an accompanying instruction.
The functionality of the REP prefix is contingent upon the state of the CX or ECX register, which dictates the number of repetitions for the operation. If the register is zero, the operation will not execute, highlighting the necessity of proper setup before employing the REP prefix. Additionally, the context in which the REP prefix is used is crucial, as it is primarily applicable to string operations that manipulate data in memory. Understanding these limitations is essential for effective assembly programming.
while the REP prefix is a valuable feature in assembly language for enhancing the performance of specific operations, it cannot be utilized by itself. It requires a valid instruction to function
Author Profile
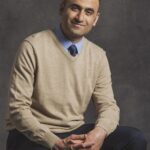
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?