Can I Program Arduino in Python? Exploring the Possibilities!
In the world of electronics and programming, Arduino has carved out a niche as a versatile platform for hobbyists and professionals alike. Traditionally, Arduino programming has been dominated by C/C++, but as the tech landscape evolves, so too do the tools and languages available to developers. One question that often arises among aspiring makers and seasoned engineers is: “Can I program Arduino in Python?” This inquiry opens up a realm of possibilities, blending the simplicity and readability of Python with the robust capabilities of Arduino hardware. Join us as we explore the intersection of these two powerful tools and uncover how Python can enhance your Arduino projects.
As the popularity of Python continues to soar, many users are eager to leverage its strengths in their Arduino endeavors. While the native Arduino IDE is designed for C/C++, various libraries and frameworks have emerged that allow for Python programming on Arduino boards. This shift not only makes programming more accessible to beginners but also empowers experienced developers to harness Python’s extensive libraries and community support for rapid prototyping and complex applications.
Moreover, the integration of Python with Arduino opens doors to exciting projects that involve data analysis, machine learning, and even web connectivity. By understanding how to bridge the gap between these two platforms, you can unlock new potential for your projects, whether you’re building a
Python Libraries for Arduino
Programming Arduino in Python typically involves using specific libraries that facilitate communication between Python and the Arduino hardware. One of the most popular libraries is `pySerial`, which allows for serial communication between Python scripts and Arduino boards.
- pySerial: This library is used to read and write data to the serial port. It is essential for sending commands and receiving data from the Arduino.
- Firmata: An open-source protocol that allows for communication between microcontrollers and software on a host computer. Using the `pyFirmata` library, you can control the Arduino board directly from Python without needing to upload custom sketches.
Setting Up Python for Arduino Programming
To begin programming an Arduino with Python, follow these steps:
- Install Python: Ensure that Python is installed on your computer. The latest version can be downloaded from the official Python website.
- Install Libraries: Use pip to install the necessary libraries:
“`bash
pip install pyserial pyfirmata
“`
- Connect Your Arduino: Use a USB cable to connect your Arduino board to your computer. Identify the COM port (Windows) or /dev/tty device (Linux/Mac) associated with the Arduino.
Basic Example of Python Code for Arduino
Here is a simple example of how to blink an LED connected to pin 13 of the Arduino using the `pyFirmata` library:
“`python
import pyfirmata
import time
Replace ‘COM3’ with your Arduino’s port
board = pyfirmata.Arduino(‘COM3’)
Start the iterator to avoid timeouts
it = pyfirmata.util.Iterator(board)
it.start()
Define the LED pin
led = board.get_pin(‘d:13:o’) digital pin 13 as output
while True:
led.write(1) Turn LED on
time.sleep(1) Wait for 1 second
led.write(0) Turn LED off
time.sleep(1) Wait for 1 second
“`
Advantages of Using Python with Arduino
Utilizing Python for Arduino programming offers several advantages:
- Ease of Use: Python’s syntax is generally simpler and more readable compared to C/C++, making it more accessible for beginners.
- Rapid Development: Python allows for quicker prototyping and testing of ideas due to its interpreted nature.
- Rich Ecosystem: Access to a vast array of Python libraries for data processing, machine learning, and more, which can be integrated into Arduino projects.
Limitations to Consider
While programming Arduino with Python is advantageous, there are limitations to be aware of:
- Performance: Python may not execute as quickly as C/C++, which could be critical for timing-sensitive applications.
- Real-Time Processing: Arduino sketches in C/C++ can interact with hardware in real-time, while Python relies on the underlying operating system, which may introduce delays.
Aspect | C/C++ Programming | Python Programming |
---|---|---|
Speed | Faster execution | Slower execution |
Ease of Learning | Steeper learning curve | More accessible |
Control | Full control over hardware | Limited control, dependent on libraries |
Programming Arduino with Python
Arduino is traditionally programmed using C/C++ via the Arduino IDE. However, there are several ways to integrate Python into the development process, allowing for scripting and rapid prototyping.
Methods to Use Python with Arduino
There are multiple approaches to utilize Python for Arduino programming:
- MicroPython: A lean implementation of Python 3 designed to run on microcontrollers. It allows you to write Python code directly on supported boards.
- Firmata Protocol: A protocol that allows communication between Arduino and Python via a serial connection. The Arduino board runs a Firmata sketch, while Python scripts control it using libraries such as `pyFirmata`.
- PyMata: A Python library that communicates with Arduino using the Firmata protocol but offers additional features and functionalities.
- Arduino-Python3: A library that allows Python scripts to control Arduino devices over serial communication, enabling a more straightforward control mechanism.
Using MicroPython with Arduino
MicroPython is suitable for boards that support it, such as the ESP8266 or ESP32. Here’s how to get started:
- Install MicroPython on your board.
- Use a compatible IDE like Thonny or uPyCraft.
- Write and upload Python scripts directly to the board.
Example code to blink an LED:
“`python
from machine import Pin
import time
led = Pin(2, Pin.OUT)
while True:
led.value(1) Turn LED on
time.sleep(1) Wait for 1 second
led.value(0) Turn LED off
time.sleep(1) Wait for 1 second
“`
Communicating with Firmata
To use Firmata for Python-Arduino communication, follow these steps:
- Upload the Firmata sketch to your Arduino from the Arduino IDE.
- Install the `pyFirmata` library using pip:
“`bash
pip install pyfirmata
“`
- Write a Python script to control the Arduino:
“`python
import pyfirmata
import time
board = pyfirmata.Arduino(‘/dev/ttyACM0’) Adjust for your port
led = board.get_pin(‘d:13:o’) Pin 13 as output
while True:
led.write(1) Turn LED on
time.sleep(1)
led.write(0) Turn LED off
time.sleep(1)
“`
Pros and Cons of Using Python with Arduino
Pros | Cons |
---|---|
Higher-level syntax for rapid development | Limited libraries compared to C/C++ |
Easier to learn for beginners | Performance overhead due to interpreted nature |
Great for prototyping | Not all Arduino boards support MicroPython |
Rich ecosystem of Python libraries | Some functionalities may not be available |
Conclusion on Python and Arduino Integration
While Arduino is primarily C/C++ based, Python can be effectively utilized through various methods, enhancing accessibility and ease of use for developers. Depending on project requirements and board capabilities, you can choose the most suitable approach for integrating Python with Arduino.
Can Python Be Used for Arduino Programming? Expert Insights
Dr. Emily Carter (Embedded Systems Engineer, Tech Innovations Inc.). “While Arduino is primarily designed to be programmed in C/C++, there are libraries such as MicroPython and CircuitPython that allow developers to write code in Python. This opens up Arduino programming to a wider audience, particularly those who are more familiar with Python.”
Mark Thompson (Senior Software Developer, IoT Solutions Group). “Using Python with Arduino can simplify the development process, especially for rapid prototyping. However, it is essential to understand the limitations in terms of performance and hardware access compared to traditional C/C++ programming.”
Linda Zhang (Educational Technology Specialist, MakerSpace Academy). “Integrating Python into Arduino projects can enhance learning experiences for students. It allows them to focus on programming logic and creativity without getting bogged down by the complexities of C/C++. However, educators should ensure that students grasp the fundamentals of both languages.”
Frequently Asked Questions (FAQs)
Can I program Arduino in Python?
Yes, you can program Arduino using Python through libraries such as PyMata, PySerial, or Firmata. These libraries facilitate communication between Python and Arduino, allowing you to control the hardware.
What is the Firmata protocol?
Firmata is a protocol that allows for communication between Arduino and software on a host computer. It enables users to write scripts in Python or other languages to control Arduino without needing to upload a separate sketch each time.
Do I need to install additional software to use Python with Arduino?
Yes, you need to install the relevant Python libraries, such as PySerial or PyMata. Additionally, you may need to upload the Firmata firmware to your Arduino board to enable communication.
Can I access all Arduino features using Python?
While many features are accessible, certain low-level functionalities may not be fully supported in Python. Complex tasks might still require programming in C/C++ directly on the Arduino IDE for optimal performance.
Is programming Arduino in Python suitable for beginners?
Yes, programming Arduino in Python can be suitable for beginners, especially those familiar with Python. It provides a more accessible syntax and allows users to leverage existing Python libraries for various tasks.
What are the advantages of using Python with Arduino?
Using Python with Arduino offers advantages such as rapid prototyping, access to extensive libraries for data analysis and visualization, and the ability to integrate with other Python applications easily.
In summary, while the primary programming language for Arduino is C/C++, it is indeed possible to program Arduino boards using Python through various methods. One popular approach is to use the MicroPython firmware, which allows users to run Python code directly on compatible microcontrollers. Additionally, tools like Firmata enable communication between Python scripts running on a host computer and the Arduino board, allowing for control and interaction without directly programming the Arduino in Python.
Moreover, the use of libraries such as PyMata and pyFirmata facilitates the integration of Python with Arduino, making it easier for developers familiar with Python to leverage their skills in hardware projects. This cross-language capability broadens the accessibility of Arduino programming, allowing a wider audience to engage with microcontroller projects.
Ultimately, while the traditional approach to Arduino programming remains rooted in C/C++, the advent of Python-compatible solutions provides an alternative for those who prefer Python’s syntax and features. This flexibility underscores the evolving nature of programming in the embedded systems landscape, catering to diverse user preferences and project requirements.
Author Profile
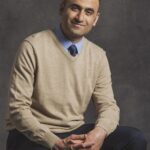
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?