Can We Use Partial Classes for XML Serialization in C#?
In the world of Cprogramming, the ability to serialize and deserialize objects into XML format is a powerful feature that facilitates data interchange and storage. As developers strive for cleaner, more maintainable code, the concept of partial classes emerges as a compelling solution. But can these partial classes be effectively utilized in the context of XML serialization? This question opens the door to a deeper exploration of how C’s features can enhance the serialization process, allowing for more organized and modular code structures.
XML serialization in Cenables developers to convert objects into XML format, making it easier to store and transmit data. Partial classes, which allow the definition of a class across multiple files, can significantly improve code organization and readability. By separating the concerns of data structure and serialization logic, developers can create a more maintainable codebase. This approach not only fosters collaboration among team members but also simplifies the management of large codebases.
Understanding how to leverage partial classes in XML serialization can lead to more efficient coding practices. As we delve into the nuances of this topic, we will uncover the benefits, potential challenges, and best practices for implementing partial classes in your XML serialization strategies. Whether you’re a seasoned developer or just starting your journey with C, the intersection of these two powerful features promises
Understanding Partial Classes in XML Serialization
Partial classes in Callow developers to split the definition of a class into multiple files. This feature can enhance code organization and maintainability, especially in large projects. When it comes to XML serialization, the use of partial classes can be particularly beneficial.
XML serialization converts an object into an XML format, allowing data to be easily transferred or stored. For partial classes, each part of the class can define members that are serialized or deserialized, providing flexibility in how you manage your data models.
Benefits of Using Partial Classes for XML Serialization
Utilizing partial classes for XML serialization offers several advantages:
- Separation of Concerns: You can separate the generated code from the custom logic. This is particularly useful when working with tools that generate code, such as Entity Framework or Visual Studio’s designer.
- Ease of Maintenance: Changes in one part of a class do not require modification of the entire class definition, making it easier to update and maintain.
- Enhanced Readability: By distributing the class definition across multiple files, you can enhance the readability of your code, making it easier for multiple developers to work on different aspects of the class concurrently.
Implementing Partial Classes with XML Serialization
To implement XML serialization with partial classes, follow these steps:
- Define the Partial Class: Create multiple files with the same class name marked as partial.
- Use XML Attributes: In one of the partial classes, apply the necessary XML serialization attributes to control the serialization behavior.
- Serialize and Deserialize: Utilize the `XmlSerializer` class to perform serialization and deserialization.
For example:
“`csharp
// File: Person.Part1.cs
[XmlRoot(“Person”)]
public partial class Person
{
[XmlElement(“Name”)]
public string Name { get; set; }
}
// File: Person.Part2.cs
public partial class Person
{
[XmlElement(“Age”)]
public int Age { get; set; }
}
“`
XML Serialization Example with Partial Classes
Below is a concise example demonstrating how to serialize and deserialize a partial class.
“`csharp
public void SerializePerson()
{
Person person = new Person { Name = “John Doe”, Age = 30 };
XmlSerializer serializer = new XmlSerializer(typeof(Person));
using (StringWriter writer = new StringWriter())
{
serializer.Serialize(writer, person);
string xmlOutput = writer.ToString();
Console.WriteLine(xmlOutput);
}
}
public Person DeserializePerson(string xml)
{
XmlSerializer serializer = new XmlSerializer(typeof(Person));
using (StringReader reader = new StringReader(xml))
{
return (Person)serializer.Deserialize(reader);
}
}
“`
Considerations When Using Partial Classes
While there are numerous benefits to using partial classes with XML serialization, some considerations include:
- Consistency: Ensure that all partial class definitions maintain consistent member names and types.
- Serialization Attributes: Only one partial class should define serialization attributes to avoid conflicts.
- Testing: Thoroughly test the serialization and deserialization processes to ensure data integrity.
Incorporating partial classes into your XML serialization strategy can lead to cleaner, more maintainable code. This approach allows developers to manage complex data structures effectively while leveraging the power of XML serialization in C.
Advantage | Description |
---|---|
Separation of Concerns | Clear distinction between generated and custom code. |
Ease of Maintenance | Modifications can be localized to individual parts. |
Enhanced Readability | Improved organization of class definitions. |
Using Partial Classes with XML Serialization in C
Partial classes in Callow you to split the definition of a class into multiple files. This feature can be particularly useful for XML serialization, as it enables the separation of auto-generated code from user-defined code. When working with XML serialization, certain attributes and methods can be defined in one part of the class, while the data properties can reside in another.
Benefits of Using Partial Classes
- Organization: Keeps auto-generated code separate from custom logic, improving maintainability.
- Collaboration: Multiple developers can work on different parts of the same class without causing merge conflicts.
- Extensibility: Allows you to extend functionality without modifying the generated code.
Implementing XML Serialization with Partial Classes
To use partial classes with XML serialization, follow these steps:
- Define the Main Class: Create a partial class in one file that includes properties for serialization.
“`csharp
[XmlRoot(“Person”)]
public partial class Person
{
[XmlElement(“Name”)]
public string Name { get; set; }
[XmlElement(“Age”)]
public int Age { get; set; }
}
“`
- Add Custom Logic: Create another partial class in a separate file to include methods or custom logic related to serialization.
“`csharp
public partial class Person
{
public string GetDetails()
{
return $”{Name}, Age: {Age}”;
}
}
“`
- Serialization Process: Use the `XmlSerializer` class to serialize or deserialize the instance of the partial class.
“`csharp
var person = new Person { Name = “John Doe”, Age = 30 };
var serializer = new XmlSerializer(typeof(Person));
using (var writer = new StringWriter())
{
serializer.Serialize(writer, person);
string xmlOutput = writer.ToString();
}
“`
Considerations and Best Practices
- Attribute Placement: Ensure that XML serialization attributes are placed in the partial class that contains the properties to be serialized.
- Consistency: Maintain consistent naming conventions across partial classes to avoid confusion.
- Visibility: Be mindful of access modifiers (public, private) when defining properties in partial classes to ensure they are accessible as intended.
Limitations
While using partial classes provides many benefits, there are some limitations to consider:
Limitation | Description |
---|---|
Single Assembly | Partial classes must be defined within the same assembly. |
Inheritance Constraints | Inheritance cannot be split across partial classes. |
Serialization Behavior | Ensure that all required properties are included across the partial class files. |
Conclusion on XML Serialization with Partial Classes
Using partial classes for XML serialization in Cis a practical approach that enhances code organization and maintainability. By following best practices and understanding the limitations, developers can effectively leverage this feature to create clean and efficient serialization processes.
Expert Insights on Using Partial Classes for XML Serialization in C
Dr. Emily Carter (Senior Software Architect, Tech Innovations Inc.). Partial classes can indeed be utilized for XML serialization in C. They allow developers to separate the auto-generated code from custom logic, enhancing maintainability. However, it is crucial to ensure that all properties intended for serialization are defined in the same partial class or are accessible to the serializer.
Michael Thompson (Lead Developer, CodeCraft Solutions). Using partial classes for XML serialization can streamline the development process. By splitting the class definition, you can manage complex data structures more effectively. Just remember that the XML serializer will only serialize properties that are public and have both getters and setters, regardless of how they are organized across partial classes.
Sarah Lee (CProgramming Expert, Software Development Journal). Partial classes offer a flexible approach to XML serialization in C. They allow for a clean separation of concerns, especially when dealing with large classes. Nevertheless, developers should be cautious about the visibility of properties and ensure that all necessary attributes for serialization are properly applied across the partial definitions.
Frequently Asked Questions (FAQs)
Can we use partial classes for XML serialization in C?
Yes, partial classes can be used for XML serialization in C. The XML serializer can serialize and deserialize properties defined in any part of the partial class, as long as they are accessible.
What are the benefits of using partial classes with XML serialization?
Using partial classes allows developers to separate the auto-generated code from custom logic, improving maintainability. It also enables easier collaboration in larger projects by allowing multiple developers to work on different parts of the same class.
Do all members of a partial class need to be public for XML serialization?
Not all members need to be public; however, only public properties and fields are serialized by default. Private members will not be included in the serialization process unless specified otherwise.
Can attributes be applied to properties in a partial class for XML serialization?
Yes, attributes such as `[XmlElement]`, `[XmlAttribute]`, and `[XmlIgnore]` can be applied to properties in any part of the partial class. These attributes control the behavior of the XML serialization process.
Is it possible to override serialization behavior in a partial class?
Yes, you can override serialization behavior by implementing the `IXmlSerializable` interface in any part of the partial class. This allows for custom serialization logic beyond the default behavior.
Are there any limitations when using partial classes with XML serialization?
While there are no inherent limitations with partial classes, developers must ensure that all parts of the class are correctly defined and that the XML serialization attributes are consistently applied across all parts for expected behavior.
In the context of XML serialization in C, partial classes can indeed be utilized effectively. A partial class allows a class definition to be split across multiple files, which can enhance organization and maintainability, especially in large projects. When it comes to XML serialization, the attributes and methods related to serialization can be defined in one part of the partial class, while the remaining functionality can be implemented in another. This separation can lead to clearer code and easier debugging.
Moreover, using partial classes for XML serialization enables developers to extend or modify the serialization behavior without altering the original class structure. This is particularly beneficial when working with auto-generated classes, such as those created by tools like Visual Studio’s dataset designer. By defining serialization attributes in a separate file, developers can ensure that their custom logic remains intact even if the auto-generated code changes.
Another important aspect to consider is that when using partial classes for XML serialization, all parts of the class must be in the same assembly and namespace. This requirement ensures that the XML serializer can access all the necessary members of the class. Additionally, developers should be mindful of the visibility of class members, as only public or internal members are serialized by default.
In summary, utilizing partial classes in C
Author Profile
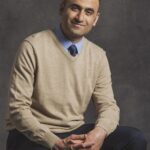
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?