Can You Really Code a Website Using Python? Exploring the Possibilities!
In the ever-evolving landscape of web development, the question of whether you can code a website in Python has gained significant traction. Traditionally associated with data science and machine learning, Python has emerged as a versatile language capable of powering dynamic web applications. As the demand for robust, scalable, and efficient web solutions continues to rise, developers are increasingly turning to Python to harness its simplicity and rich ecosystem of frameworks. But how does Python stack up against more conventional web development languages, and what tools are available to help you create a stunning website?
At its core, Python offers a plethora of frameworks and libraries that streamline the web development process, making it accessible for both seasoned developers and newcomers alike. Frameworks like Django and Flask provide powerful tools for building everything from simple blogs to complex e-commerce platforms, allowing developers to focus on creating engaging user experiences rather than getting bogged down in the minutiae of coding. With Python’s clean syntax and strong community support, coding a website in Python is not only feasible but can also be an enjoyable experience.
Moreover, the flexibility of Python means that it can integrate seamlessly with various technologies, making it an appealing choice for full-stack development. Whether you’re looking to implement RESTful APIs, manage databases, or handle user authentication, Python’s extensive libraries and modules
Frameworks for Building Websites in Python
Python offers several robust frameworks that simplify the process of web development. Each framework has its unique features, catering to various project requirements. Here are some of the most popular frameworks:
- Django: A high-level framework that encourages rapid development and clean, pragmatic design. It comes with built-in features such as an ORM (Object-Relational Mapping), authentication, and an admin panel.
- Flask: A lightweight and flexible micro-framework that allows developers to create web applications quickly. It is ideal for smaller projects or when a high degree of customization is needed.
- FastAPI: A modern framework for building APIs with Python 3.6+ based on standard Python type hints. It is known for its speed and performance.
- Pyramid: A versatile framework that can be used for both small and large applications. It allows developers to start small and scale up as needed.
Choosing the Right Framework
When selecting a framework, consider the following criteria:
- Project Size: Larger projects may benefit from Django’s features, while smaller applications might be better suited for Flask.
- Development Speed: If time is a constraint, Django’s built-in features can accelerate development.
- Scalability: Choose a framework that can grow with your project. Pyramid and FastAPI are designed with scalability in mind.
- Community and Support: A strong community can provide valuable resources and support. Django and Flask have large communities, making them easier to work with.
Framework | Type | Best For | Key Features |
---|---|---|---|
Django | High-level | Full-stack applications | ORM, Admin panel, Authentication |
Flask | Micro | Small to medium applications | Flexibility, Minimalism |
FastAPI | Modern | APIs | Speed, Asynchronous support |
Pyramid | Versatile | Scalable applications | Flexible, Modular |
Development Process Overview
Building a website with Python typically involves the following steps:
- Setting Up the Environment: Install Python and the desired framework. Use virtual environments to manage dependencies.
- Creating the Application Structure: Organize your project files according to the conventions of the chosen framework.
- Routing: Define URLs and map them to functions or classes that handle requests.
- Creating Templates: Use templating engines like Jinja2 (for Flask) or Django’s templating system to create dynamic HTML pages.
- Database Integration: Utilize the framework’s ORM to connect to databases and perform CRUD operations.
- Implementing Business Logic: Write the core functionality of your application, ensuring it meets the requirements.
- Testing: Conduct tests to ensure that your application is robust and functional. Most frameworks come with built-in testing tools.
- Deployment: Choose a hosting solution and deploy your web application.
In summary, the development process is systematic and can be tailored according to the project needs. Each framework provides tools and libraries that facilitate these steps, enabling developers to focus on building quality applications.
Frameworks for Web Development in Python
Python offers several frameworks that facilitate web development, each with its strengths and use cases. The most notable frameworks include:
- Django
- Full-stack framework that follows the “batteries included” philosophy.
- Features include an ORM, authentication system, and an admin panel.
- Suitable for large applications and rapid development.
- Flask
- A micro-framework that provides flexibility and simplicity.
- Ideal for small to medium applications and APIs.
- Allows developers to choose components as needed, promoting modularity.
- FastAPI
- Asynchronous framework designed for high-performance APIs.
- Utilizes type hints for automatic generation of interactive documentation.
- Great for applications requiring speed and scalability.
- Pyramid
- A flexible framework that can scale from small to large applications.
- Offers a minimalistic approach, giving developers the freedom to structure their projects.
Setting Up a Basic Web Application
To create a simple web application using Flask, follow these steps:
- Install Flask:
“`bash
pip install Flask
“`
- Create the Application:
- Create a new Python file (e.g., `app.py`).
- Add the following code:
“`python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def home():
return “Hello, World!”
if __name__ == ‘__main__’:
app.run(debug=True)
“`
- Run the Application:
- Execute the command:
“`bash
python app.py
“`
- Access the Web Application:
- Open a web browser and navigate to `http://127.0.0.1:5000/`.
Database Integration
Integrating a database into a Python web application can be achieved using various libraries. Common choices include:
Library | Description |
---|---|
SQLAlchemy | ORM that supports multiple database backends. |
SQLite | Lightweight database that comes built-in with Python. |
PostgreSQL | Advanced relational database suitable for complex queries. |
MySQL | Popular open-source database, often used in web applications. |
Example using SQLAlchemy with Flask:
- Install SQLAlchemy:
“`bash
pip install Flask-SQLAlchemy
“`
- Set Up Database:
- Modify `app.py` to include:
“`python
from flask_sqlalchemy import SQLAlchemy
app.config[‘SQLALCHEMY_DATABASE_URI’] = ‘sqlite:///site.db’
db = SQLAlchemy(app)
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(150), nullable=, unique=True)
db.create_all()
“`
Deployment Options
Deploying a Python web application can be done via various platforms. Some popular choices include:
- Heroku:
- Easy deployment for small to medium applications.
- Supports add-ons for databases and other services.
- AWS Elastic Beanstalk:
- Scalable deployment option for larger applications.
- Manages the infrastructure and scaling.
- DigitalOcean:
- Virtual private servers (Droplets) for full control.
- Suitable for developers who want to manage their environments.
- PythonAnywhere:
- Simplified deployment platform specifically for Python applications.
- Offers a free tier for small projects.
By choosing the appropriate framework, integrating a database, and selecting a deployment option, you can effectively build and launch a web application using Python.
Can You Build a Website Using Python? Insights from Experts
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Yes, you can code a website in Python. Frameworks like Django and Flask provide robust tools for web development, allowing developers to create dynamic and scalable web applications efficiently.”
James Lin (Lead Developer, Web Solutions Group). “Python is an excellent choice for web development, especially for back-end services. Its simplicity and readability enhance productivity, making it easier to maintain and scale web applications.”
Linda Gomez (Web Development Instructor, Code Academy). “While Python is not traditionally associated with front-end development, it can be effectively used in conjunction with JavaScript and HTML to create full-stack applications, demonstrating its versatility in web development.”
Frequently Asked Questions (FAQs)
Can you code a website in Python?
Yes, you can code a website in Python using various web frameworks such as Django, Flask, and FastAPI, which facilitate the development of dynamic web applications.
What are the advantages of using Python for web development?
Python offers simplicity and readability, extensive libraries, a strong community, and frameworks that streamline web development, making it an excellent choice for developers.
Which Python framework is best for building a website?
The best framework depends on the project requirements. Django is ideal for large applications with complex functionalities, while Flask is suitable for smaller, simpler applications.
Can Python handle front-end development?
Python is primarily a back-end language. However, it can be integrated with front-end technologies like HTML, CSS, and JavaScript to create a full-stack web application.
Is Python suitable for high-traffic websites?
Yes, Python can handle high-traffic websites, especially when using scalable frameworks like Django and optimizing the code and database queries for performance.
What are common databases used with Python web applications?
Common databases include PostgreSQL, MySQL, SQLite, and MongoDB, all of which can be easily integrated with Python web frameworks for data management.
it is indeed possible to code a website using Python. The language offers several frameworks and libraries, such as Django and Flask, which facilitate the development of robust and scalable web applications. These frameworks provide essential tools and features that streamline the coding process, allowing developers to focus on building functionality rather than dealing with the intricacies of web protocols and server management.
Moreover, Python’s simplicity and readability make it an attractive choice for both novice and experienced developers. The vast ecosystem of third-party packages and modules available through Python’s package manager, pip, further enhances its capabilities for web development. This flexibility allows developers to integrate various functionalities, such as database management, user authentication, and API development, seamlessly into their projects.
Key takeaways from the discussion highlight that while Python may not be the traditional choice for front-end development, it excels in back-end development. Combining Python with front-end technologies like HTML, CSS, and JavaScript can lead to the creation of dynamic and interactive web applications. Overall, Python’s versatility and strong community support make it a valuable tool for web development.
Author Profile
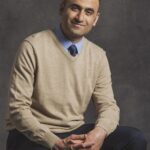
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?