Can You Connect React to Node.js? Exploring the Seamless Integration!
In the ever-evolving landscape of web development, the combination of React and Node.js has emerged as a powerful duo that can elevate your applications to new heights. As developers seek to create dynamic, responsive user interfaces alongside robust server-side functionalities, the ability to seamlessly connect these two technologies becomes essential. Whether you’re building a single-page application or a full-fledged web platform, understanding how to integrate React with Node.js can unlock a world of possibilities, enhancing both performance and user experience.
At its core, React is a JavaScript library designed for building user interfaces, while Node.js serves as a runtime environment that allows you to execute JavaScript on the server side. This synergy enables developers to use a single programming language throughout the entire stack, streamlining development processes and fostering greater collaboration between frontend and backend teams. By connecting React to Node.js, you can efficiently manage data flow, handle API requests, and create a cohesive environment where client and server communicate effortlessly.
In this article, we will explore the fundamental concepts and techniques for integrating React with Node.js, highlighting the benefits of this connection and providing insights into best practices. As we delve deeper, you’ll discover how to set up your development environment, manage state effectively, and leverage the strengths of both technologies to create high-performing applications that resonate with
Setting Up the Environment
To effectively connect React to Node.js, it is essential to set up both environments correctly. This typically involves installing Node.js and npm (Node Package Manager), which are crucial for managing packages and running your backend server. On the React side, create a new project using Create React App for a streamlined setup.
- Install Node.js and npm:
- Download the installer from the [official Node.js website](https://nodejs.org/).
- Follow the installation instructions for your operating system.
- Create a new React application:
“`bash
npx create-react-app my-app
cd my-app
“`
- Set up a Node.js server:
- Create a new directory for the server.
- Initialize a new Node.js project:
“`bash
mkdir my-server
cd my-server
npm init -y
“`
Building the Node.js Backend
Once the environment is set up, you can start building the Node.js backend. The backend will handle HTTP requests from the React frontend. Here’s how to create a simple Express server:
– **Install Express and other dependencies:**
“`bash
npm install express cors body-parser
“`
– **Create a basic server setup:**
“`javascript
const express = require(‘express’);
const cors = require(‘cors’);
const bodyParser = require(‘body-parser’);
const app = express();
app.use(cors());
app.use(bodyParser.json());
app.get(‘/api/data’, (req, res) => {
res.json({ message: ‘Hello from Node.js!’ });
});
const PORT = process.env.PORT || 5000;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
“`
This code sets up a simple server that responds with a JSON object when the `/api/data` endpoint is accessed.
Connecting React to Node.js
To connect your React application to the Node.js backend, you will need to make HTTP requests from the React components. This can be done using the Fetch API or libraries like Axios.
– **Using Fetch API:**
“`javascript
useEffect(() => {
fetch(‘http://localhost:5000/api/data’)
.then(response => response.json())
.then(data => console.log(data));
}, []);
“`
– **Using Axios:**
First, install Axios:
“`bash
npm install axios
“`
Then, use it in your React component:
“`javascript
import axios from ‘axios’;
useEffect(() => {
axios.get(‘http://localhost:5000/api/data’)
.then(response => {
console.log(response.data);
});
}, []);
“`
Proxy Configuration
To simplify API calls during development, you can set up a proxy in your React application. This allows you to avoid CORS issues and makes the API calls cleaner.
– **Add a proxy to `package.json` in the React project:**
“`json
“proxy”: “http://localhost:5000”,
“`
By configuring this proxy, you can change your API requests in React to relative paths, eliminating the need to specify the full URL:
“`javascript
fetch(‘/api/data’)
.then(response => response.json())
.then(data => console.log(data));
“`
Common Issues and Solutions
While connecting React to Node.js, you may encounter several common issues. Here are some potential problems and their solutions:
Issue | Solution |
---|---|
CORS errors | Ensure you have the CORS middleware set up in your Express server. |
Server not responding | Verify that the Node.js server is running and the correct port is being used. |
404 not found | Check that the API endpoint is correctly defined and matches the request URL. |
Connecting React to Node.js
To connect a React application to a Node.js backend, you typically establish a RESTful API. This allows the front-end React application to communicate with the back-end Node.js server, enabling data exchange and functionality.
Setting Up the Node.js Server
- **Initialize Your Node.js Project**:
- Create a new directory for your server.
- Run `npm init -y` to generate a `package.json` file.
- **Install Required Packages**:
- Install Express.js for routing and middleware handling:
“`bash
npm install express cors body-parser
“`
- Optionally, install nodemon for auto-restarting the server during development:
“`bash
npm install –save-dev nodemon
“`
- **Create a Basic Server**:
- Create an `index.js` file and set up a simple Express server:
“`javascript
const express = require(‘express’);
const cors = require(‘cors’);
const bodyParser = require(‘body-parser’);
const app = express();
const PORT = process.env.PORT || 5000;
app.use(cors());
app.use(bodyParser.json());
app.get(‘/api/data’, (req, res) => {
res.json({ message: ‘Hello from Node.js!’ });
});
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
“`
Creating the React Application
- **Set Up the React App**:
- Use Create React App for easy setup:
“`bash
npx create-react-app my-app
cd my-app
“`
- **Install Axios for API Requests**:
- Axios is a promise-based HTTP client for making requests:
“`bash
npm install axios
“`
- **Fetch Data from the Node.js Server**:
- In one of your components (e.g., `App.js`), import Axios and fetch data:
“`javascript
import React, { useEffect, useState } from ‘react’;
import axios from ‘axios’;
function App() {
const [data, setData] = useState(null);
useEffect(() => {
axios.get(‘http://localhost:5000/api/data’)
.then(response => {
setData(response.data.message);
})
.catch(error => {
console.error(‘There was an error fetching the data!’, error);
});
}, []);
return (
{data}
);
}
export default App;
“`
Handling CORS Issues
When connecting React and Node.js, you may encounter Cross-Origin Resource Sharing (CORS) issues. This occurs when the front-end and back-end are served from different origins.
- Solution:
- Use the `cors` middleware in your Node.js server. This allows requests from different origins. The configuration can be as simple as:
“`javascript
app.use(cors());
“`
Environment Variables
To manage configurations such as API URLs effectively, use environment variables:
- Create a `.env` file in your React app directory:
“`
REACT_APP_API_URL=http://localhost:5000/api/data
“`
- Access the variable in your React app:
“`javascript
axios.get(process.env.REACT_APP_API_URL)
“`
Deployment Considerations
When deploying your applications, consider the following:
Aspect | Node.js Backend | React Frontend |
---|---|---|
Hosting | Heroku, AWS, DigitalOcean | Netlify, Vercel, GitHub Pages |
Build Process | Use `npm run build` for production | Use `npm run build` for production |
Environment Variables | Configure through hosting platform | Use `.env` for local development |
Properly connecting React to Node.js involves understanding both the front-end and back-end setup, ensuring smooth communication and data exchange while handling issues like CORS and environment configurations effectively.
Connecting React and Node.js: Perspectives from Leading Developers
Dr. Emily Carter (Full-Stack Developer, Tech Innovations Inc.). “Connecting React to Node.js is not only feasible but also a common practice in modern web development. React serves as an excellent front-end library for building user interfaces, while Node.js provides a powerful backend environment. By using RESTful APIs or GraphQL, developers can seamlessly integrate these technologies to create dynamic and responsive applications.”
Michael Chen (Senior Software Engineer, CodeCraft Solutions). “The synergy between React and Node.js lies in their JavaScript foundation, which allows for a unified development experience. This connection enables developers to share code between the client and server, streamlining the development process and enhancing maintainability. Utilizing tools like Express.js on the Node.js side can further simplify the integration.”
Sarah Patel (Tech Lead, Digital Future Labs). “To effectively connect React with Node.js, it is crucial to understand the architecture of your application. Using a client-server model, where React handles the front end and Node.js manages the backend, allows for efficient data flow. Implementing WebSockets can also facilitate real-time communication between the two, enhancing user experience.”
Frequently Asked Questions (FAQs)
Can you connect React to Node.js?
Yes, you can connect React to Node.js. React serves as the front-end framework, while Node.js can be used for the back-end server. They communicate through APIs, typically using REST or GraphQL.
What is the best way to connect React to Node.js?
The best way to connect React to Node.js is by creating a RESTful API using Node.js and Express. You can then use the Fetch API or Axios in React to make HTTP requests to this API.
Do I need to use a database with Node.js when connecting to React?
Using a database is not mandatory, but it is highly recommended for storing and retrieving data. Common choices include MongoDB, PostgreSQL, or MySQL, depending on your application’s requirements.
How do I handle CORS when connecting React to Node.js?
To handle CORS (Cross-Origin Resource Sharing), you can use the `cors` middleware in your Node.js application. This allows you to specify which domains are permitted to access your API.
Can I deploy React and Node.js together?
Yes, you can deploy React and Node.js together. You can serve the React app from the same Node.js server, allowing for a seamless integration and reducing the complexity of managing separate deployments.
What are some common issues when connecting React to Node.js?
Common issues include CORS errors, incorrect API endpoints, and data format mismatches. Debugging these issues often involves checking network requests in the browser’s developer tools and ensuring proper API responses from the Node.js server.
In summary, connecting React to Node.js is a powerful approach for developing full-stack applications. React, as a front-end library, excels in creating dynamic user interfaces, while Node.js serves as an efficient back-end runtime environment that allows for server-side scripting. The integration of these two technologies enables developers to build seamless applications that leverage the strengths of both the client and server sides.
The process of connecting React to Node.js typically involves setting up an API with Node.js that communicates with the React front end. This is often achieved through RESTful services or GraphQL, allowing for efficient data exchange. By using tools like Axios or Fetch API in React, developers can make HTTP requests to the Node.js server, facilitating a smooth flow of data between the client and server.
Moreover, utilizing technologies such as Express.js for routing and MongoDB or other databases for data storage enhances the overall architecture of the application. This combination not only improves performance but also ensures scalability and maintainability. As a result, developers can create robust applications that meet user demands while maintaining a clean separation of concerns between the front end and back end.
the synergy between React and Node.js offers a comprehensive solution for modern web development. By leveraging the
Author Profile
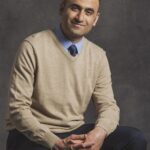
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?