Can You Upload Features as a Client-Side Function?
In the ever-evolving landscape of web development, the way we handle data and user interactions is constantly being redefined. One of the most pressing questions for developers today is whether certain functionalities, particularly those involving file uploads, can be effectively managed on the client side. As applications become more dynamic and user-centric, understanding the implications and possibilities of client-side file uploads is crucial for creating seamless user experiences. This article delves into the intricacies of client-side functions, examining their capabilities, limitations, and the potential they hold for modern web applications.
The concept of client-side file uploads revolves around the ability to manage file selection, validation, and even preliminary processing directly within the user’s browser. This approach not only enhances the responsiveness of applications but also reduces server load by minimizing unnecessary data transfers. By leveraging technologies such as JavaScript and HTML5, developers can create intuitive interfaces that empower users to interact with their files before any data is sent to the server. However, this method also raises important questions about security, compatibility, and performance that must be carefully considered.
As we explore the feasibility of client-side upload features, we will touch upon the various tools and frameworks available to developers, as well as the best practices that can help mitigate potential risks. Understanding the balance between client-side convenience and
Understanding Client-Side Upload Features
Client-side upload features allow users to select and send files directly from their devices to a server without needing to refresh the page or perform server-side processing for each file. Implementing these features effectively enhances user experience, as they provide immediate feedback and reduce latency.
Advantages of Client-Side Uploads
Utilizing client-side functions for file uploads comes with several advantages:
- Instant Feedback: Users receive immediate validation and feedback on their file selections.
- Reduced Server Load: Offloading initial file handling to the client can decrease server processing time and resource usage.
- Better User Experience: Users can preview files before uploading, which enhances engagement and satisfaction.
Key Technologies for Client-Side Uploads
Several technologies are pivotal in creating efficient client-side upload features:
- HTML5 File API: Enables web applications to access files stored on the user’s device.
- JavaScript: Facilitates file reading, validation, and asynchronous uploads.
- AJAX: Allows for the sending of files to the server without reloading the page.
Implementation Example
Below is a simple example of how client-side file uploads can be implemented using HTML and JavaScript.
“`html
```
This code allows users to select multiple files, preview them as thumbnails, and prepares them for upload.
Challenges and Considerations
When implementing client-side upload features, it’s crucial to consider potential challenges:
- Browser Compatibility: Different browsers may have varying levels of support for the File API.
- File Size Limitations: Users might attempt to upload files larger than the allowed size; implementing checks is essential.
- Security Concerns: Ensure that file validation and sanitization are done to avoid security vulnerabilities, such as file type spoofing.
Best Practices for Client-Side Uploads
To ensure successful implementation, follow these best practices:
- Validate file types and sizes before upload.
- Provide clear user feedback throughout the upload process.
- Implement progress indicators for large file uploads.
File Type | Max Size | Notes |
---|---|---|
Image (JPEG, PNG) | 5 MB | Commonly used for profile pictures and galleries. |
Document (PDF, DOCX) | 10 MB | Used for resumes, reports, etc. |
Video (MP4) | 100 MB | Streaming or uploading video content. |
By adhering to these guidelines and considering the technological landscape, developers can successfully implement client-side file upload features that are efficient, secure, and user-friendly.
Understanding Client-Side Upload Features
The ability to upload files through a client-side function is a common requirement in web applications. This functionality can greatly enhance user experience by allowing users to interactively select and upload files without the need for server round trips until the actual upload is initiated.
Mechanisms for Client-Side File Uploads
Client-side file uploads can be achieved primarily through HTML and JavaScript. The main components involved include:
- HTML Input Elements: `` allows users to select files from their local system.
- JavaScript APIs: The File API and XMLHttpRequest (or Fetch API) facilitate reading files and sending them to the server.
Implementation Steps
- HTML Structure: Create a file input element.
```html
```
- JavaScript Handling:
- Capture the file selection.
- Read the file content (optional).
- Upload the file to the server.
Example code snippet:
```javascript
document.getElementById('fileInput').addEventListener('change', function(event) {
const files = event.target.files;
const formData = new FormData();
for (let i = 0; i < files.length; i++) {
formData.append('files[]', files[i]);
}
fetch('/upload', {
method: 'POST',
body: formData
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
});
```
Advantages of Client-Side Uploads
- User Experience: Immediate feedback and interactivity.
- Reduced Server Load: Only sends data to the server when necessary.
- File Validation: Can perform checks (e.g., file type, size) before sending data.
Considerations for Implementation
When implementing client-side uploads, consider the following:
Aspect | Details |
---|---|
Browser Compatibility | Ensure compatibility across modern browsers. |
Security | Implement security measures to prevent malicious uploads. |
Progress Feedback | Use progress bars to enhance user experience during uploads. |
File Size Limits | Implement limits to avoid excessive load on the server. |
Best Practices
- Use Asynchronous Uploads: This keeps the user interface responsive.
- Validate Files: On both client and server sides to prevent errors.
- Optimize File Handling: Consider using libraries for handling uploads, such as Dropzone.js or Fine Uploader.
Client-side file uploads are not only feasible but are a best practice in modern web development, enhancing user interaction while optimizing server resources. By leveraging HTML and JavaScript effectively, developers can create robust applications that manage file uploads efficiently.
Client-Side Functionality in Feature Uploads: Expert Insights
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). Client-side functions can indeed handle feature uploads, provided that the necessary security protocols are in place. This approach can enhance user experience by allowing immediate feedback and reducing server load.
Michael Thompson (Lead Front-End Developer, Web Solutions Group). Implementing upload features on the client side is feasible and often beneficial. However, developers must consider browser compatibility and the limitations of local storage when designing these functionalities.
Sarah Patel (Cloud Computing Specialist, Future Tech Labs). While client-side upload features can improve performance, they should not replace server-side validations. A hybrid approach ensures data integrity and security, making it essential for robust applications.
Frequently Asked Questions (FAQs)
Can you upload features be a client side function?
Yes, upload features can be implemented as client-side functions, allowing users to select and upload files directly from their devices to a server without extensive server-side processing.
What technologies are commonly used for client-side file uploads?
Common technologies include HTML5, JavaScript, and frameworks such as React or Angular, which facilitate user interactions and file handling in the browser.
What are the advantages of client-side file uploads?
Client-side uploads reduce server load, enhance user experience by providing immediate feedback, and allow for pre-upload validations, such as file type and size checks.
Are there any limitations to client-side file uploads?
Yes, limitations include reliance on the user's browser capabilities, potential security risks if not properly handled, and restrictions on file size imposed by the browser or server.
How can security be ensured during client-side uploads?
Security can be enhanced by implementing measures such as file type validation, size limits, and using secure protocols (like HTTPS) to encrypt data during transmission.
Can client-side uploads work with large files?
While client-side uploads can handle large files, it is important to implement chunked uploads or resumable uploads to manage large files effectively and avoid timeouts or failures.
In the context of web development, the ability to upload features on the client side refers to the process where users can select and send files from their local devices to a server without requiring a page refresh. This functionality is typically implemented using HTML5 and JavaScript, which facilitate a more interactive and responsive user experience. Client-side file uploads are often achieved through the use of APIs such as the File API and XMLHttpRequest, as well as modern alternatives like the Fetch API. These technologies allow for asynchronous uploads, enabling users to continue interacting with the application while files are being processed.
One of the primary advantages of client-side file uploads is the reduction in server load and bandwidth usage, as files can be processed directly in the user's browser before being sent to the server. This can lead to improved performance and faster upload times. Additionally, client-side validation can be implemented to ensure that the files meet specific criteria (e.g., file type, size) before they are uploaded, enhancing the overall user experience and reducing unnecessary server requests.
However, it is essential to consider security implications when implementing client-side upload features. Proper validation and sanitization of files are crucial to prevent vulnerabilities such as file injection attacks. Furthermore, developers should ensure that the application adher
Author Profile
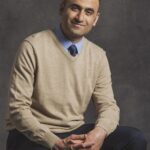
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?